Java黑皮书15.3(移动小球)
package sample;
import javafx.application.Application;
import javafx.event.ActionEvent;
import javafx.event.EventHandler;
import javafx.geometry.Pos;
import javafx.scene.Scene;
import javafx.scene.layout.BorderPane;
import javafx.scene.layout.Pane;
import javafx.scene.shape.Circle;
import javafx.scene.control.Button;
import javafx.scene.layout.HBox;
import javafx.scene.paint.Color;
import javafx.stage.Stage;
public class Main extends Application {
public static void main(String[] args) {
launch(args);
}
Ball ball = new Ball(200, 100, 30);
@Override
public void start(Stage primaryStage) {
Board board = new Board(ball);
Frame frame = new Frame();
BorderPane borderPane = new BorderPane();
borderPane.setCenter(board);
borderPane.setBottom(frame);
Scene scene = new Scene(borderPane, 600, 300);
primaryStage.setTitle("移动小球");
primaryStage.setScene(scene);
primaryStage.show();
frame.btLeft.setOnAction(new Left());
frame.btRight.setOnAction(new Right());
frame.btUp.setOnAction(new Up());
frame.btDown.setOnAction(new Down());
}
class Left implements EventHandler<ActionEvent> {
@Override
public void handle(ActionEvent e) {
ball.Left();
ball.checkBall();
}
}
class Right implements EventHandler<ActionEvent> {
@Override
public void handle(ActionEvent e) {
ball.Right();
ball.checkBall();
}
}
class Up implements EventHandler<ActionEvent> {
@Override
public void handle(ActionEvent e) {
ball.Up();
ball.checkBall();
}
}
class Down implements EventHandler<ActionEvent> {
@Override
public void handle(ActionEvent e) {
ball.Down();
ball.checkBall();
}
}
class Ball extends Circle {
public Ball() {
}
public Ball(double x, double y, double r) {
super(x, y, r);
setStroke(Color.BLACK);
setFill(Color.WHITE);
}
public void Left() {
setCenterX(getCenterX() - 10);
}
public void Right() {
setCenterX(getCenterX() + 10);
}
public void Up() {
setCenterY(getCenterY() - 10);
}
public void Down() {
setCenterY(getCenterY() + 10);
}
public void checkBall() {
if (getCenterX() < 0) Right();
if (getCenterX() > 400) Left();
if (getCenterY() < 0) Down();
if (getCenterY() > 220) Up();
}
}
class Board extends Pane {
public Board() {
}
public Board(Ball ball) {
getChildren().add(ball);
}
}
class Frame extends HBox {
public Button btLeft = new Button("左");
public Button btRight = new Button("右");
public Button btUp = new Button("上");
public Button btDown = new Button("下");
public Frame() {
this.getChildren().add(btLeft);
this.getChildren().add(btRight);
this.getChildren().add(btUp);
this.getChildren().add(btDown);
setAlignment(Pos.CENTER);
}
}
}
运行结果
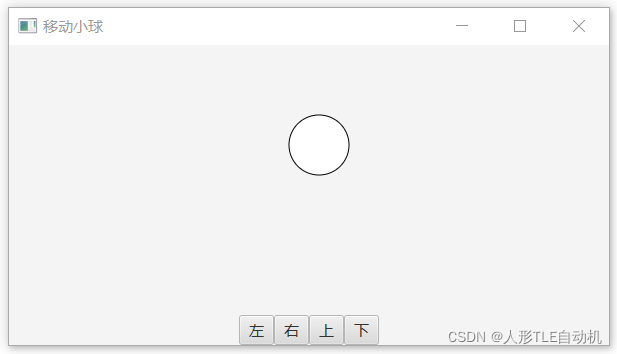
|