HTML5游戏引擎(十七)-egret引擎实战——踩格子游戏
第六章 踩格子游戏
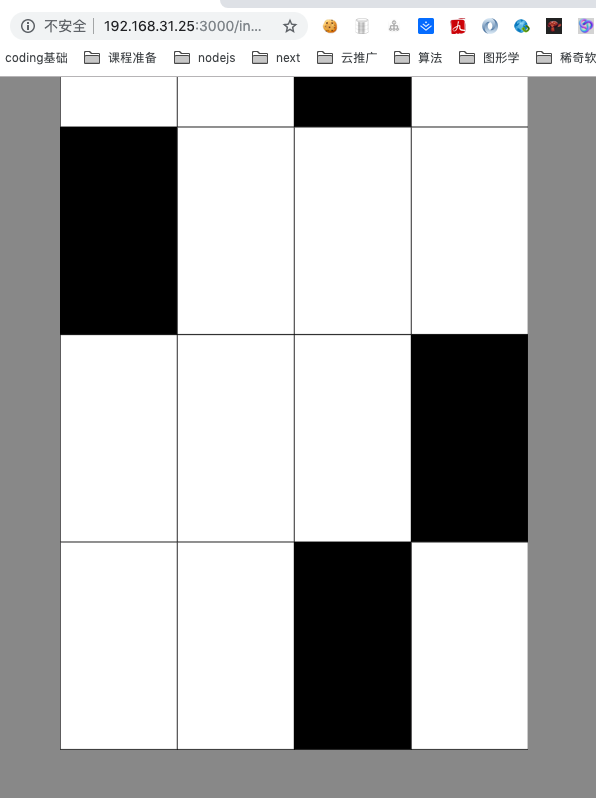
逻辑分析
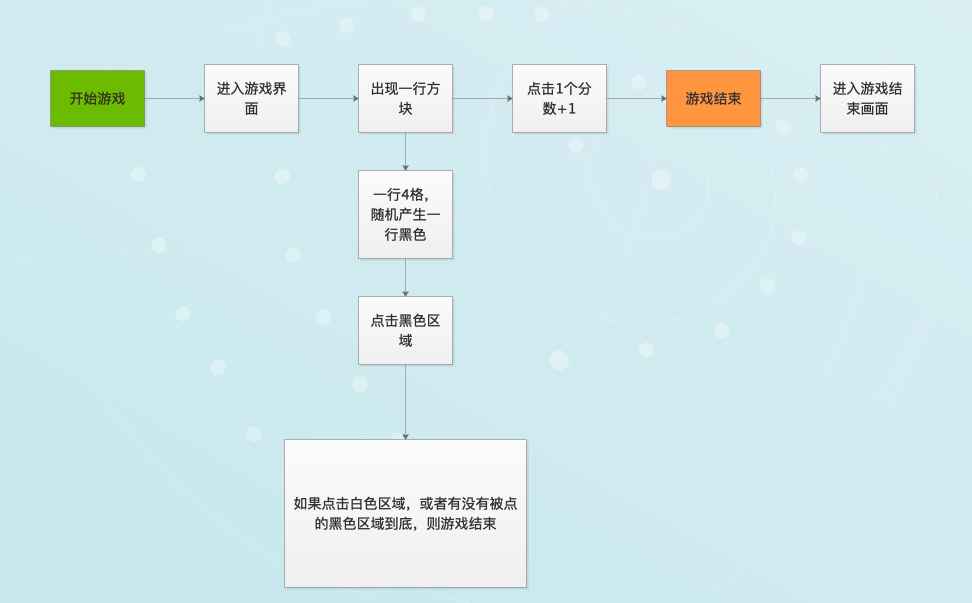
游戏配置
class GameData {
private static score:number = 0;
public static row:number = 4;
public static column:number = 4;
public static speed:number = 10;
private static _boxWidth:number = 0;
private static _boxHeight:number = 0;
public static getScore():number {
return GameData.score;
}
public static setScore(val:number) {
GameData.score = val;
GameData.speed = 10+GameData.score;
}
public static getBoxWidth():number {
if( GameData._boxWidth == 0) {
GameData._boxWidth = egret.MainContext.instance.stage.stageWidth / GameData.column;
}
return GameData._boxWidth;
}
public static getBoxHeight():number {
if( GameData._boxHeight == 0) {
GameData._boxHeight = egret.MainContext.instance.stage.stageHeight / GameData.row;
}
return GameData._boxHeight;
}
public static getStageHeight():number {
return egret.MainContext.instance.stage.stageHeight;
}
public static getStageWidth():number {
return egret.MainContext.instance.stage.stageWidth;
}
}
入口文件
Main.ts作为项目入口文件,在构造函数中启动init方法
class Main extends egret.DisplayObjectContainer {
public constructor() {
super();
console.log('start');
this.addEventListener(egret.Event.ADDED_TO_STAGE, this.addStage, this);
}
private addStage() {
this.removeEventListener(egret.Event.ADDED_TO_STAGE, this.addStage, this);
this.init()
}
private gameview:GameView ;
private timer:egret.Timer;
private gameoverPanel:GameOverPanel;
private startgamePanel:StartGamePanel;
private init():void {
this.gameview = new GameView();
this.addChild(this.gameview);
this.gameview.addEventListener(GameEvent.GAME_OVER, this.gameover,this);
this.timer = new egret.Timer(20,0);
this.timer.addEventListener(egret.TimerEvent.TIMER, this.timers, this);
this.gameoverPanel = new GameOverPanel();
this.gameoverPanel.addEventListener(GameEvent.GAME_START,this.startgame,this);
this.startgamePanel = new StartGamePanel();
this.startgamePanel.addEventListener(GameEvent.GAME_START, this.startgame, this);
this.addChild(this.startgamePanel);
}
private timers() {
this.gameview.move();
}
private gameover(evt:GameEvent):void {
this.timer.stop();
this.gameoverPanel.update();
this.addChild(this.gameoverPanel);
}
private startgame(evt:GameEvent):void {
GameData.speed = 10;
GameData.setScore(0);
this.gameview.startgame();
if(this.startgamePanel.parent) {
this.removeChild(this.startgamePanel);
}
if(this.gameoverPanel.parent) {
this.removeChild(this.gameoverPanel);
}
this.timer.start();
}
}
游戏场景GameView
游戏中的整体逻辑
class GameView extends egret.Sprite {
public constructor() {
super();
this.init();
}
private _boxGroups:Array<GroupRect>;
private scoreText:egret.BitmapText;
private init():void {
this._boxGroups = [];
var len:number = GameData.row+1;
for(var i:number=0;i<len;i++) {
var boxg:GroupRect = new GroupRect();
this._boxGroups.push(boxg);
this.addChild(boxg);
boxg.addEventListener(GameEvent.GAME_OVER, this.gameOver, this);
boxg.addEventListener(GameEvent.GAME_HIT, this.clickRight, this);
}
this.scoreText = new egret.BitmapText();
this.scoreText.x = 180;
this.scoreText.y = 50;
this.scoreText.text = String(0);
this.addChild(this.scoreText);
}
public startgame():void {
this.scoreText.text = String(0);
var len:number = GameData.row+1;
for(var i:number=0;i<len;i++) {
this._boxGroups[i].create();
this._boxGroups[i].y = 0-GameData.getBoxHeight()*(1+i);
}
}
public move() {
var len:number = GameData.row+1;
for(var i:number=0;i<len;i++) {
this._boxGroups[i].y += GameData.speed;
if(this._boxGroups[i].y>=GameData.getStageHeight()){
if(!this._boxGroups[i].isHit) {
this.gameOver();
return;
}
if(i==0) {
this._boxGroups[i].y = this._boxGroups[4].y - GameData.getBoxHeight();
} else {
this._boxGroups[i].y = this._boxGroups[i-1].y - GameData.getBoxHeight();
}
this._boxGroups[i].create();
}
}
}
private gameOver(evt:GameEvent=null):void {
var event:GameEvent = new GameEvent(GameEvent.GAME_OVER);
this.dispatchEvent(event);
}
private clickRight(evt:GameEvent):void {
GameData.setScore(GameData.getScore()+1);
this.scoreText.text = String(GameData.getScore());
}
}
一行格子GroupRect
每一排格子的抽象类,会生成4个格子。
class GroupRect extends egret.Sprite {
public constructor() {
super();
this.init();
}
private _boxs:Array<BoxGraphics>;
private init():void {
this._boxs = [];
for(var i:number=0;i<GameData.column;i++) {
var box:BoxGraphics = new BoxGraphics();
this._boxs.push(box);
box.addEventListener(GameEvent.GAME_HIT, this.clickRight, this);
box.addEventListener(GameEvent.GAME_OVER, this.boxGameOver, this);
this.addChild(box);
box.x = GameData.getBoxWidth()*i;
}
}
public create():void {
this._isHit = false;
var touchIndex:number = Math.floor(Math.random()*4);
var len:number = this._boxs.length;
for(var i:number=0;i<len;i++) {
if(i==touchIndex) {
this._boxs[i].drawBox(true);
} else {
this._boxs[i].drawBox();
}
}
}
private _isHit:boolean = false;
public get isHit():boolean {
return this._isHit;
}
private clickRight(evt:GameEvent):void {
if(!this._isHit) {
this._isHit = true;
var event:GameEvent = new GameEvent(GameEvent.GAME_HIT);
this.dispatchEvent(event);
}
}
private boxGameOver(evt:GameEvent):void {
var event:GameEvent = new GameEvent(GameEvent.GAME_OVER);
this.dispatchEvent(event);
}
}
一个格子BoxGraphics
每一个小格子,可以绑定点击事件,决定游戏的成功与失败。
class BoxGraphics extends egret.Shape {
public constructor() {
super();
this.init();
}
private init() {
this.touchEnabled = true;
this.width = GameData.getBoxWidth();
this.height = GameData.getBoxHeight();
this.addEventListener(egret.TouchEvent.TOUCH_BEGIN, this.click, this);
}
private _canTouch:boolean = false;
public drawBox(canTouch:boolean=false) {
this._canTouch = canTouch;
this.graphics.clear();
if(canTouch) {
this.graphics.beginFill(0);
} else {
this.graphics.beginFill(0xffffff);
}
this.graphics.lineStyle(1, 0);
this.graphics.drawRect(0,0,GameData.getBoxWidth(),GameData.getBoxHeight());
this.graphics.endFill();
}
private click(evt:egret.TouchEvent):void {
this.graphics.clear();
if(this._canTouch) {
this.graphics.beginFill(0xcccccc);
} else {
this.graphics.beginFill(0xff0000);
}
this.graphics.lineStyle(1, 0);
this.graphics.drawRect(0,0,GameData.getBoxWidth(),GameData.getBoxHeight());
this.graphics.endFill();
var event:GameEvent;
if(!this._canTouch) {
event = new GameEvent(GameEvent.GAME_OVER);
} else {
event = new GameEvent(GameEvent.GAME_HIT);
}
this.dispatchEvent(event);
}
}
事件绑定
游戏开始与结束的自定义事件
class GameEvent extends egret.Event {
public static GAME_OVER:string = "game_over_event";
public static GAME_HIT:string = "game_hit_event";
public static GAME_START:string = "game_start_event";
public constructor(type:string, bubbles:boolean = false, cancelable:boolean = false) {
super(type,bubbles,cancelable);
}
}
游戏开始场景
class StartGamePanel extends egret.Sprite {
public constructor() {
super();
this.init();
}
private _background:egret.Shape;
private _startBtn:egret.TextField;
private init() {
this._background = new egret.Shape();
this._background.graphics.beginFill(0);
this._background.graphics.drawRect(0,0,GameData.getStageWidth(),GameData.getStageHeight());
this._background.graphics.endFill();
this.addChild( this._background );
this._startBtn = new egret.TextField();
this._startBtn.text = "开始";
this._startBtn.size = 50;
this._startBtn.x = 180;
this._startBtn.y = 200;
this.addChild( this._startBtn );
this._startBtn.touchEnabled = true;
this._startBtn.addEventListener(egret.TouchEvent.TOUCH_BEGIN, this.click, this);
}
private click(evt:egret.TouchEvent):void {
var event:GameEvent = new GameEvent(GameEvent.GAME_START);
this.dispatchEvent(event);
}
}
游戏结束场景
class GameOverPanel extends egret.Sprite {
public constructor() {
super();
this.init();
}
private _background:egret.Shape;
private _score:egret.TextField;
private _startGameBtn:egret.TextField;
private init():void {
this._background = new egret.Shape();
this._background.graphics.beginFill(0);
this._background.graphics.drawRect(0,0,GameData.getStageWidth(),GameData.getStageHeight());
this._background.graphics.endFill();
this.addChild( this._background );
this._score = new egret.TextField();
this._score.textColor = 0xffffff;
this._score.text = "分数:" + GameData.getScore();
this._score.size = 50;
this._score.x = 150;
this._score.y = 50;
this.addChild( this._score );
this._startGameBtn = new egret.TextField();
this._startGameBtn.text = "重玩";
this._startGameBtn.size = 40;
this._startGameBtn.textColor = 0xffffff;
this._startGameBtn.x = 180;
this._startGameBtn.y = 200;
this.addChild( this._startGameBtn );
this._startGameBtn.touchEnabled = true;
this._startGameBtn.addEventListener(egret.TouchEvent.TOUCH_BEGIN, this.restartGame,this);
}
public update() {
this._score.text = "分数:" + GameData.getScore();
}
private restartGame(evt:egret.TouchEvent) {
var event:GameEvent = new GameEvent(GameEvent.GAME_START);
this.dispatchEvent(event);
}
}
|