【千锋合集】史上最全Unity3D全套教程|匠心之作_哔哩哔哩_bilibili
《设计模式》一书
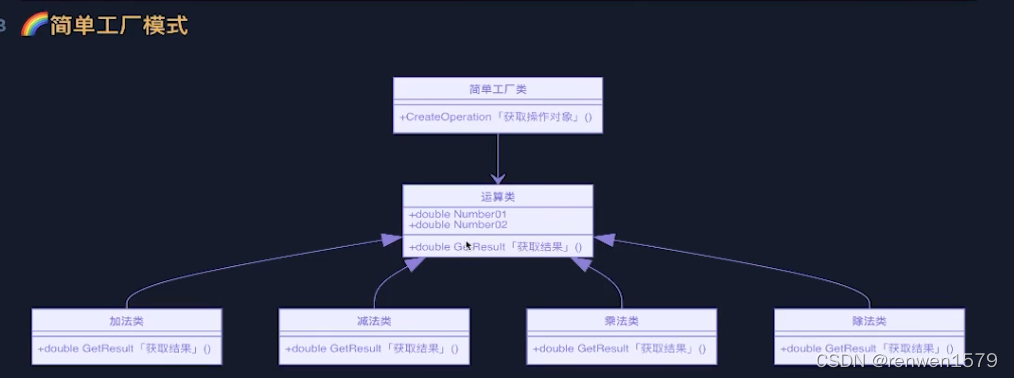
?UML类图------StarUML
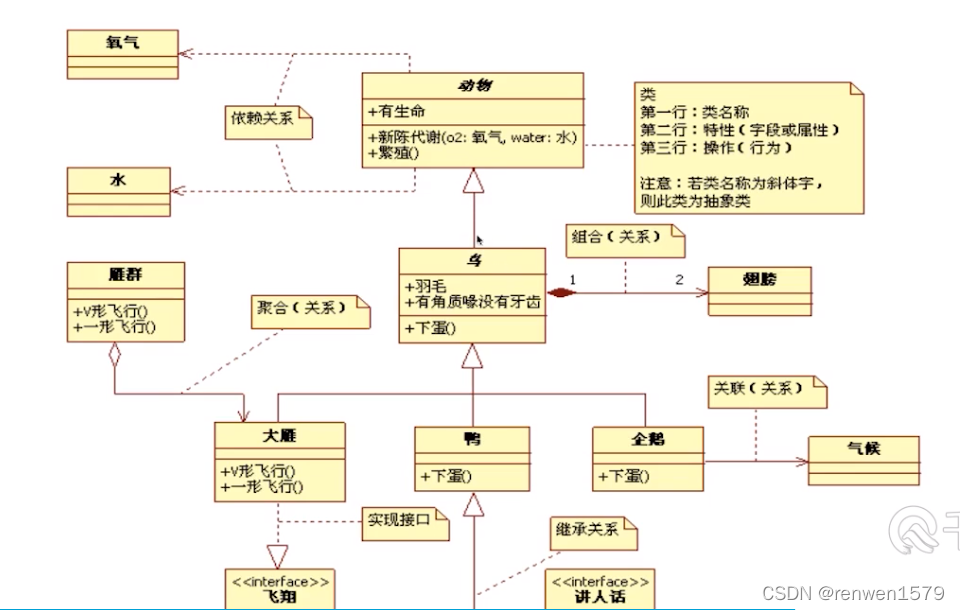
?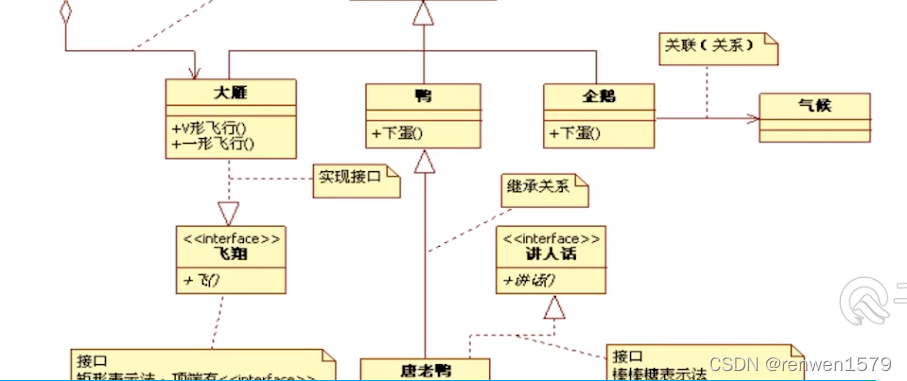
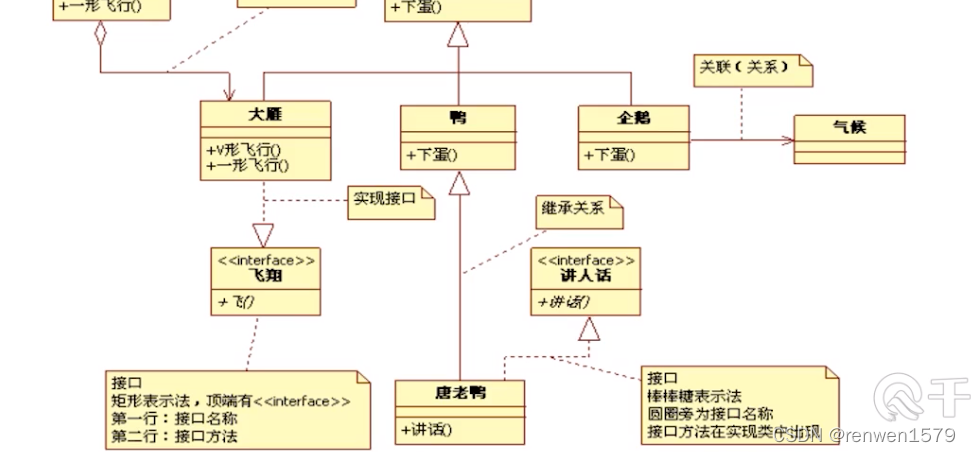
using System.Collections; using System.Collections.Generic; using UnityEngine;
public class LegencyFunction : MonoBehaviour {
? ? private void Start() ? ? { ? ? ? ? float? result=Count(10,21,"*"); ? ? ? ? Debug.Log(result); ? ? ? ? //新模式 ? ? ? ? BinaryOperation operation = OperationFactory.CreateOperation(1,2,"+"); ? ? ? ? operation.SetNumber(2,3); ? ? ? ? float newresult=operation.GetResult(); ? ? ? ? Debug.Log(newresult); ? ? } ? ? public float? Count(float num01,float num02,string sign) { ? ? ? ? switch (sign) { ? ? ? ? ? ? case "+": ? ? ? ? ? ? ? ? return num01 + num02; ? ? ? ? ? ? case "-": ? ? ? ? ? ? ? ? return num01 - num02; ? ? ? ? ? ? case "*": ? ? ? ? ? ? ? ? return num01 * num02; ? ? ? ? ? ? case "/": ? ? ? ? ? ? ? ? return num01 / num02; ? ? ? ? ? ? default: ? ? ? ? ? ? ? ? return null; ? ? ? ? }? ? ? }
? ? /// <summary> ? ? /// /工厂模式 ? ? /// </summary> ? ? public abstract class BinaryOperation { ? ? ? ? protected float number01; ? ? ? ? protected float number02;
? ? ? ? public BinaryOperation() { }
? ? ? ? public void SetNumber(float number01,float number02) { ? ? ? ? ? ? this.number01 = number01; ? ? ? ? ? ? this.number02 = number02; ? ? ? ? }
? ? ? ? public BinaryOperation(float number01, float number02) ? ? ? ? { ? ? ? ? ? ? this.number01 = number01; ? ? ? ? ? ? this.number02 = number02; ? ? ? ? } ? ? ? ? public abstract float GetResult(); ? ? } ? ? public class PlusOperation : BinaryOperation ? ? { ? ? ? ? public PlusOperation(float number01, float number02) : base(number01, number02) ? ? ? ? { ? ? ? ? }
? ? ? ? public override float GetResult() ? ? ? ? { ? ? ? ? ? ? return number01 + number02; ? ? ? ? } ? ? }
? ? public class SubtractionOperation : BinaryOperation ? ? { ? ? ? ? public SubtractionOperation(float number01, float number02) : base(number01, number02) ? ? ? ? { ? ? ? ? }
? ? ? ? public override float GetResult() ? ? ? ? { ? ? ? ? ? ? return number01 - number02; ? ? ? ? } ? ? }
? ? public class MultiplicationOperation : BinaryOperation ? ? { ? ? ? ? public MultiplicationOperation(float number01, float number02) : base(number01, number02) ? ? ? ? { ? ? ? ? }
? ? ? ? public override float GetResult() ? ? ? ? { ? ? ? ? ? ? return number01*number02; ? ? ? ? } ? ? }
? ? public class OperationFactory { ? ? ? ? public static BinaryOperation CreateOperation(float number01, float number02, string sign) { ? ? ? ? ? ? switch (sign) ? ? ? ? ? ? { ? ? ? ? ? ? ? ? case "+": ? ? ? ? ? ? ? ? ? ? return new PlusOperation(number01,number02); ? ? ? ? ? ? ? ? case "-": ? ? ? ? ? ? ? ? ? ? return new SubtractionOperation(number01, number02); ? ? ? ? ? ? ? ? case "*": ? ? ? ? ? ? ? ? ? ? return new MultiplicationOperation(number01,number02); ? ? ? ? ? ? ? ? default: ? ? ? ? ? ? ? ? ? ? return null; ? ? ? ? ? ? } ? ? ? ? } ? ? } }
拆分、隔离
using System.Collections; using System.Collections.Generic; using UnityEngine;
public class UnityAssetsFactory : MonoBehaviour { ? ? public static UnityAsset CreateUnityAsset(string assetType) { ? ? ? ? switch (assetType) { ? ? ? ? ? ? case "GameObject": ? ? ? ? ? ? ? ? return new PrefabAsset(""); ? ? ? ? ? ? case "Sprite": ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? return new SpriteAsset(""); ? ? ? ? ? ? default: ? ? ? ? ? ? ? ? return null; ? ? ? ? } ? ? }?? ? }
public abstract class UnityAsset { ? ? public string path; ? ? public UnityAsset(string path) { ? ? ? ? this.path=path; ? ? } ? ? protected object asset; ? ? public void Load() { asset = Resources.Load(path); } ? ? public abstract void Instantiate(); ? ? public abstract void Show(); }
public class PrefabAsset : UnityAsset {
? ? public PrefabAsset(string path) : base(path) ? ? { ? ? }
? ? public override void Instantiate() ? ? { ? ? ? ? GameObject go = GameObject.Instantiate(asset as GameObject); ? ? }
? ? public override void Show() ? ? { ? ? ? ?? ? ? } }
public class SpriteAsset : UnityAsset {
? ? public SpriteAsset(string path) : base(path) ? ? { ? ? }
? ? public override void Instantiate() ? ? { ? ? ? ? GameObject go = new GameObject("Sprite"); ? ? ? ? SpriteRenderer spriteRenderer=go.AddComponent<SpriteRenderer>(); ? ? ?? ? ? ? ? Sprite sprite = Sprite.Create(asset as Texture2D,new Rect(0,0,100,100),Vector2.zero); ? ? ? ? sprite.name = "sprite"; ? ? ? ? spriteRenderer.sprite = sprite; ? ? }
? ? public override void Show() ? ? { ? ? ? ? ? ? } } ?
using System.Collections; using System.Collections.Generic; using UnityEngine;
public class UnityFactoryConsole : MonoBehaviour { ? ? private void Start() ? ? { ? ? ? ? //第一步,工厂生产对象 ? ? ? ? //UnityAsset unityAsset =UnityAssetsFactory.CreateUnityAsset("GameObject","Cube"); ? ? ? ? UnityAsset unityAsset = UnityAssetsFactory.CreateUnityAsset("Sprite"); ? ? ? ? unityAsset.path = "Pig"; ? ? ? ? //第二步,对象加载资源 ? ? ? ? unityAsset.Load(); ? ? ? ? //第三步,生产该资源 ? ? ? ? unityAsset.Instantiate(); ? ?? ? ? }
} ?
using System.Collections; using System.Collections.Generic; using UnityEngine;
public class UnitySingletonFactory : MonoBehaviour { ? ? public static UnitySingletonFactory instance;
? ? private void Awake() ? ? { ? ? ? ? instance = this; ? ? ? ? DontDestroyOnLoad(gameObject);//单例永久存在 ? ? }
? ? public T GetAsset<T>(string assetPath) where T:Object { ? ? ? ? return Resources.Load<T>(assetPath); ? ? } ? ? public T[] GetAssets<T>(string assetsPath) where T:Object { ? ? ? ? return Resources.LoadAll<T>(assetsPath); ? ? }
? ? } ?
using System.Collections; using System.Collections.Generic; using UnityEngine;
public class UnityFactoryConsole : MonoBehaviour { ? ? private void Start() ? ? { ? ? ? ? //第一步,工厂生产对象 ? ? ? ? //UnityAsset unityAsset =UnityAssetsFactory.CreateUnityAsset("GameObject","Cube"); ? ? ? ? UnityAsset unityAsset = UnityAssetsFactory.CreateUnityAsset("Sprite"); ? ? ? ? unityAsset.path = "Pig"; ? ? ? ? //第二步,对象加载资源 ? ? ? ? unityAsset.Load(); ? ? ? ? //第三步,生产该资源 ? ? ? ? unityAsset.Instantiate();
? ? ? ? //单例工厂--->直接获取资源
? ? ? ? GameObject cubePrefab=UnitySingletonFactory.instance.GetAsset<GameObject>("Cube"); ? ? ? ? Instantiate(cubePrefab); ? ?? ? ? }
} ?
|