1.
创建继承至AttributeSet C++类,命名为MMOARPGAttributeSet
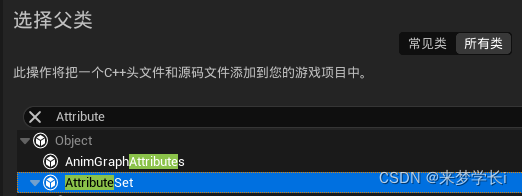
2.?创建属性定义通用宏
?MMOARPGGameplayAbilityMacros.h,它可以自动生成属性的所有样板代码
#pragma once
#include "AttributeSet.h"
//定义一个通用宏,用于定义属性集
#define ATTRIBUTE_ACCESSORS(ClassName, PropertyName) \
GAMEPLAYATTRIBUTE_PROPERTY_GETTER(ClassName, PropertyName) \
GAMEPLAYATTRIBUTE_VALUE_GETTER(PropertyName) \
GAMEPLAYATTRIBUTE_VALUE_SETTER(PropertyName) \
GAMEPLAYATTRIBUTE_VALUE_INITTER(PropertyName)
3.属性创建,
打开MMOARPGAttributeSet.h
#pragma once
#include "CoreMinimal.h"
#include "AttributeSet.h"
#include "AbilitySystemComponent.h"
#include "Net/UnrealNetwork.h"
#include "MMOARPGGameplayAbilityMacros.h"
#include "MMOARPGAttributeSet.generated.h"
/**
*
*/
UCLASS()
class MMOARPGGAME_API UMMOARPGAttributeSet : public UAttributeSet
{
GENERATED_BODY()
public:
UMMOARPGAttributeSet();
virtual void GetLifetimeReplicatedProps(TArray<FLifetimeProperty>& OutLifetimeProps) const override;
virtual void PostGameplayEffectExecute(const struct FGameplayEffectModCallbackData& Data) override;
public:
UPROPERTY(BlueprintReadOnly, Category = "Attribute", ReplicatedUsing = OnRep_Level)
FGameplayAttributeData Level;//角色等级
ATTRIBUTE_ACCESSORS(UMMOARPGAttributeSet, Level)
UPROPERTY(BlueprintReadOnly, Category = "Attribute", ReplicatedUsing = OnRep_Health)
FGameplayAttributeData Health;//生命值
ATTRIBUTE_ACCESSORS(UMMOARPGAttributeSet, Health)
UPROPERTY(BlueprintReadOnly, Category = "Attribute", ReplicatedUsing = OnRep_MaxHealth)
FGameplayAttributeData MaxHealth;//最大生命值
ATTRIBUTE_ACCESSORS(UMMOARPGAttributeSet, MaxHealth)
UPROPERTY(BlueprintReadOnly, Category = "Attribute", ReplicatedUsing = OnRep_Mana)
FGameplayAttributeData Mana;//魔法值
ATTRIBUTE_ACCESSORS(UMMOARPGAttributeSet, Mana)
UPROPERTY(BlueprintReadOnly, Category = "Attribute", ReplicatedUsing = OnRep_MaxMana)
FGameplayAttributeData MaxMana;//最大魔法值
ATTRIBUTE_ACCESSORS(UMMOARPGAttributeSet, MaxMana)
protected:
UFUNCTION()
virtual void OnRep_Level(const FGameplayAttributeData& OldValue);
UFUNCTION()
virtual void OnRep_Health(const FGameplayAttributeData& OldValue);
UFUNCTION()
virtual void OnRep_MaxHealth(const FGameplayAttributeData& OldValue);
UFUNCTION()
virtual void OnRep_Mana(const FGameplayAttributeData& OldValue);
UFUNCTION()
virtual void OnRep_MaxMana(const FGameplayAttributeData& OldValue);
};
MMOARPGAttributeSet.cpp的实现
#include "MMOARPGAttributeSet.h"
#include "GameplayEffect.h"
#include "GameplayEffectExtension.h"
UMMOARPGAttributeSet::UMMOARPGAttributeSet()
:Level(1)
, Health(100.f)
, MaxHealth(100.f)
, Mana(0.f)
, MaxMana(0.f)
{
}
void UMMOARPGAttributeSet::GetLifetimeReplicatedProps(TArray<FLifetimeProperty>& OutLifetimeProps) const
{
Super::GetLifetimeReplicatedProps(OutLifetimeProps);
DOREPLIFETIME(UMMOARPGAttributeSet, Level);
DOREPLIFETIME(UMMOARPGAttributeSet, Health);
DOREPLIFETIME(UMMOARPGAttributeSet, MaxHealth);
DOREPLIFETIME(UMMOARPGAttributeSet, Mana);
DOREPLIFETIME(UMMOARPGAttributeSet, MaxMana);
}
void UMMOARPGAttributeSet::PostGameplayEffectExecute(const struct FGameplayEffectModCallbackData& Data)
{
//数值计算,例如受到伤害后扣血就是写在此处
}
void UMMOARPGAttributeSet::OnRep_Level(const FGameplayAttributeData& OldValue)
{
GAMEPLAYATTRIBUTE_REPNOTIFY(UMMOARPGAttributeSet, Level, OldValue);
}
void UMMOARPGAttributeSet::OnRep_Health(const FGameplayAttributeData& OldValue)
{
GAMEPLAYATTRIBUTE_REPNOTIFY(UMMOARPGAttributeSet, Health, OldValue);
}
void UMMOARPGAttributeSet::OnRep_MaxHealth(const FGameplayAttributeData& OldValue)
{
GAMEPLAYATTRIBUTE_REPNOTIFY(UMMOARPGAttributeSet, MaxHealth, OldValue);
}
void UMMOARPGAttributeSet::OnRep_Mana(const FGameplayAttributeData& OldValue)
{
GAMEPLAYATTRIBUTE_REPNOTIFY(UMMOARPGAttributeSet, Mana, OldValue);
}
void UMMOARPGAttributeSet::OnRep_MaxMana(const FGameplayAttributeData& OldValue)
{
GAMEPLAYATTRIBUTE_REPNOTIFY(UMMOARPGAttributeSet, MaxMana, OldValue);
}
4.属性生成
打开CharacterBase.h
class UMMOARPGAttributeSet;//前置声明
UPROPERTY(Category = MMOARPGCharacterBase, VisibleAnywhere, BlueprintReadOnly, meta = (AllowPrivateAccess = "true"))
TObjectPtr<UMMOARPGAttributeSet>AttributeSet;
打开CharacterBase.cpp,在构造函数中创建属性对象组件,在Beginplay中生成
AttributeSet = CreateDefaultSubobject<UMMOARPGAttributeSet>(TEXT("AttributeSet"));
void AMMOARPGCharacterBase::BeginPlay()
{
Super::BeginPlay();
if (GetWorld())
{
//生成角色属性
TArray<UAttributeSet*> AttributeSets;
AttributeSets.Add(AttributeSet);
AbilitySystemComponent->SetSpawnedAttributes(AttributeSets);
}
}
|