这次有三个项目可以选择,我将其中的公告牌和血条两个项目放在一起完成了。我把这次做的项目命名为小企鹅钓鱼,主要是可以用滑动公告牌选择鱼的种类,通过按键控制血量条中血量的多少。
公告牌部分
UI部分
- 新建一个Canvas,其配置如下图。在Canvas下创建BigPanel、Toggle
? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ??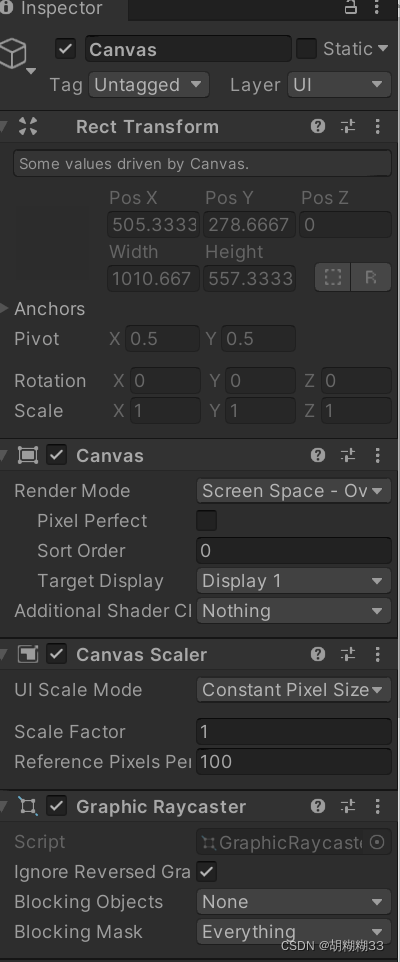
- BigPanel
????????在Canvas下创建BigPanel、Toggle。在BigPanel中主要是对整个项目背景的设置,将Backgroud中的Source Image对象选为海底图片(所用图片在Resource\Textures中,并且将图片的Texture Type设为Script(2D and UI)),在Title中设置标题的图片背景和文字,选择钓鱼(2)作为背景图片,在其Text中将标题设为小企鹅钓鱼。BigPanel中Backgroud、Borad、Title的配置如下图——? ? ?
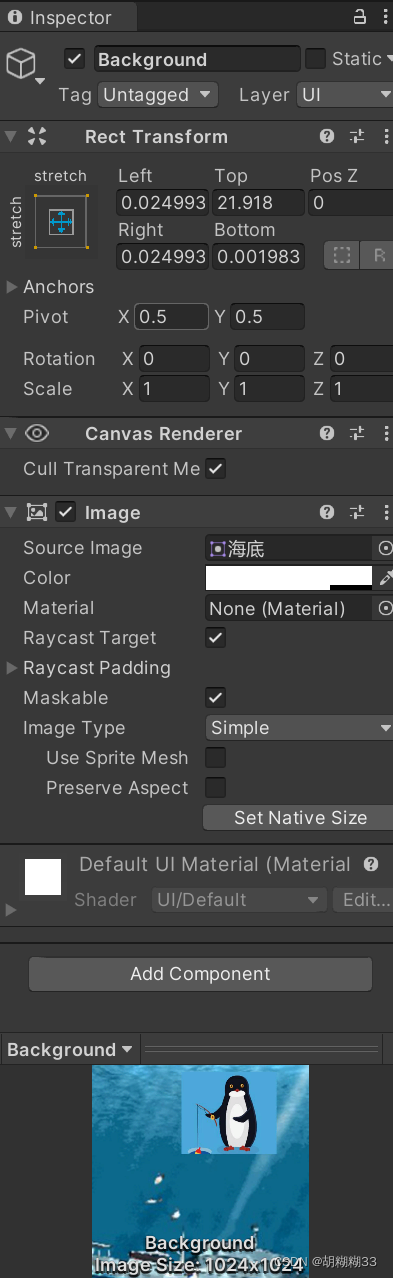
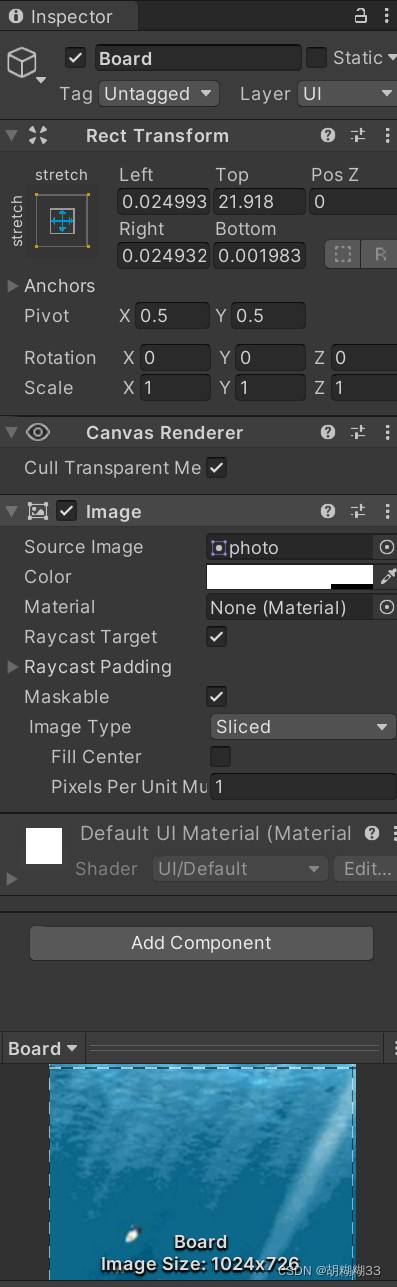
?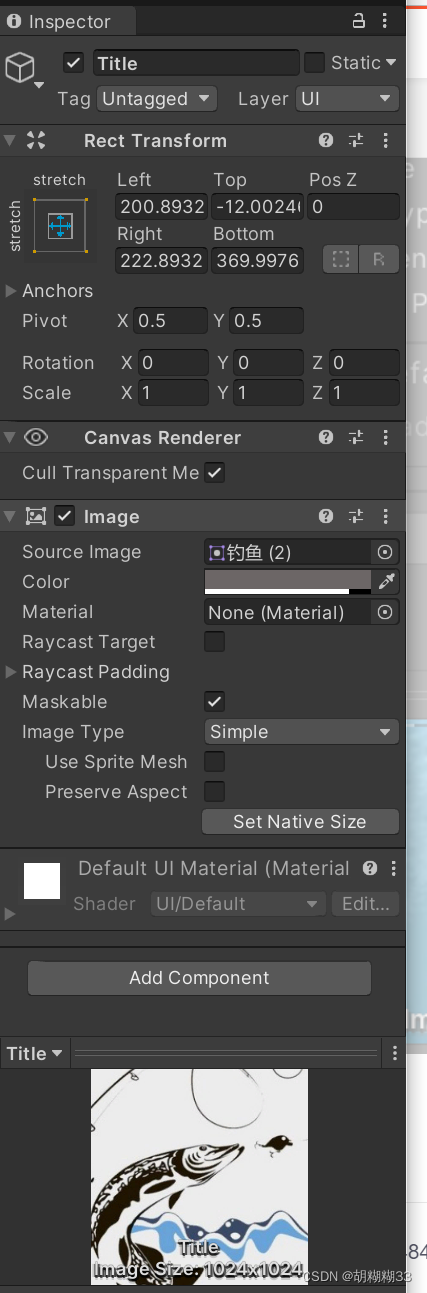 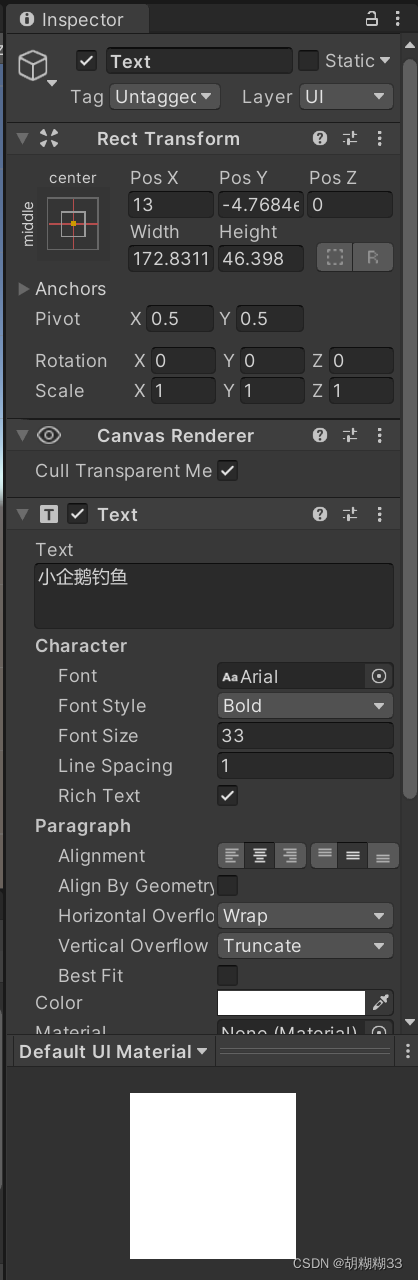
?????????
????????在Canvas一个比较重要的部分是Scroll View,将ScrollRect的Anchors设置在中心,Vertical Scrollbar为None。在Scroll View的Viewport的Content下添加Grid Layout Group组件,并添加Image组件(10个)。在Scroll View下的Scrollbar Horizontal设置一个公告框,其下的Sliding Area组件是放置滑动条的框,在Sliding Area下的Handle设置滑动条。在Scroll View添加一个Panel,作为中心选中的边框。Scroll View中的设置如下——?
???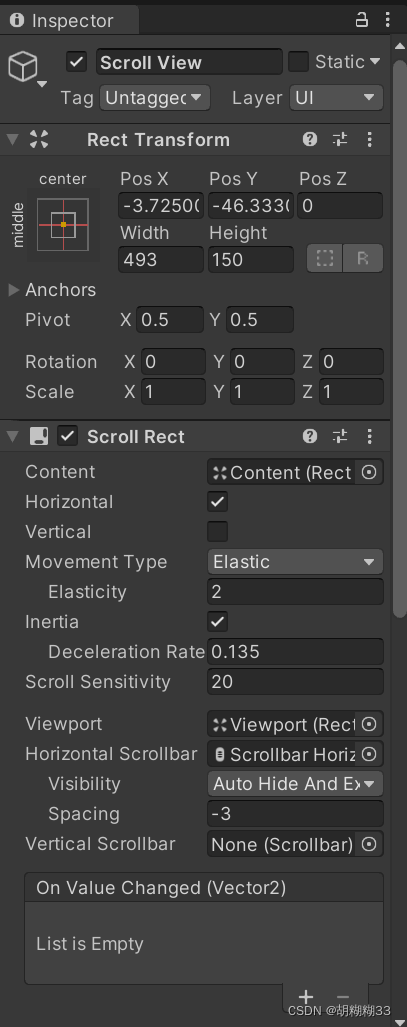 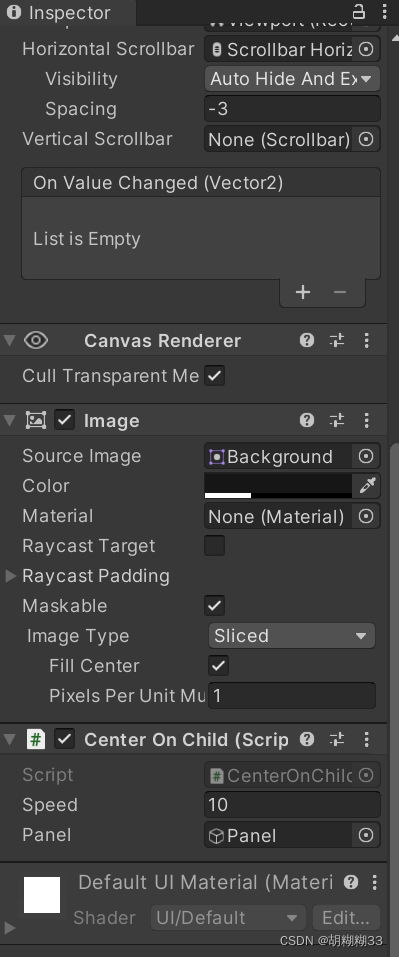 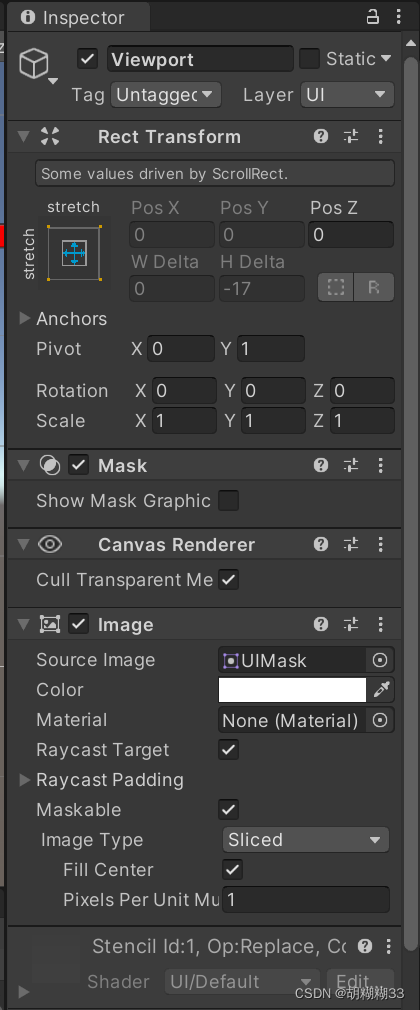 ? ? ? ? ? ? ? ? ?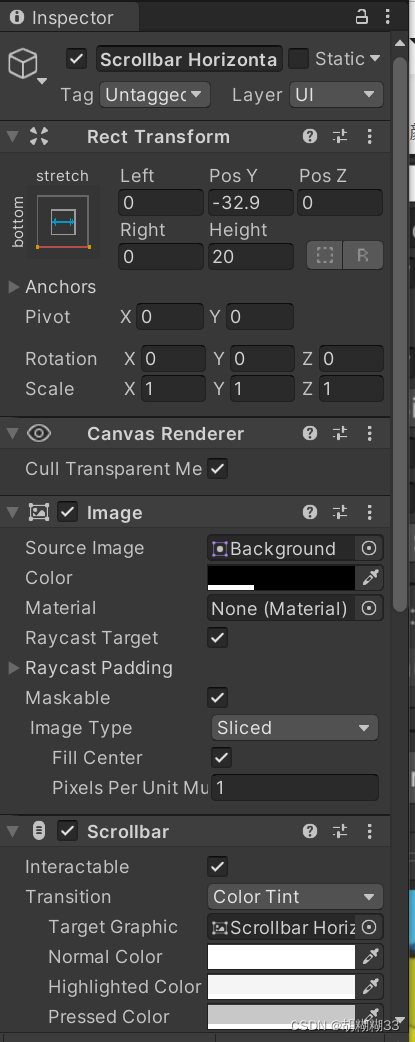 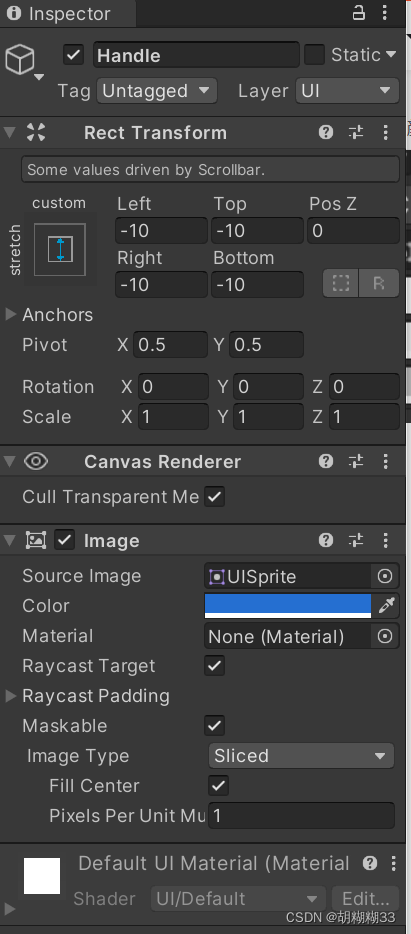 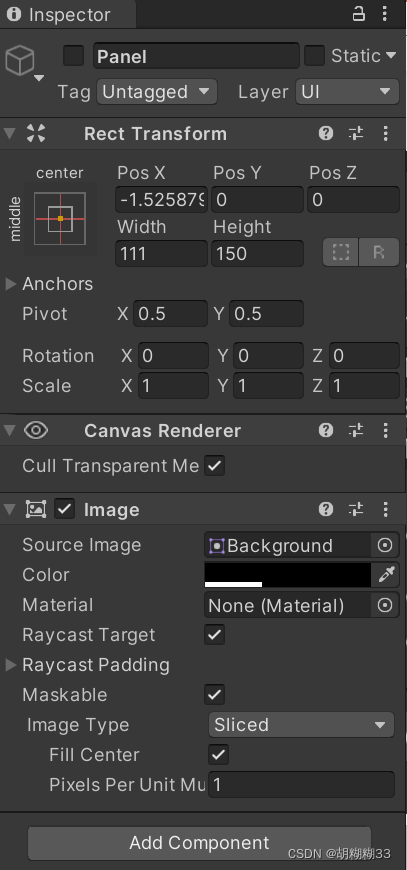 ? ? ? ?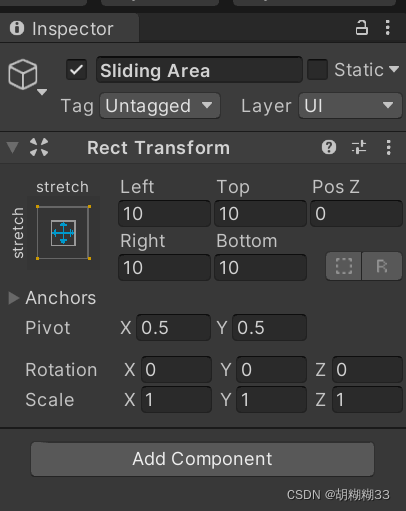 ? ? ?
? ? ? ? 2.Toggle
? ? ? ? ???在Canvas下创建Toggle,用来设置选中中间物体的,用Lable设置文字,用Backgroud设置点击的选取打勾。设置情况如下——
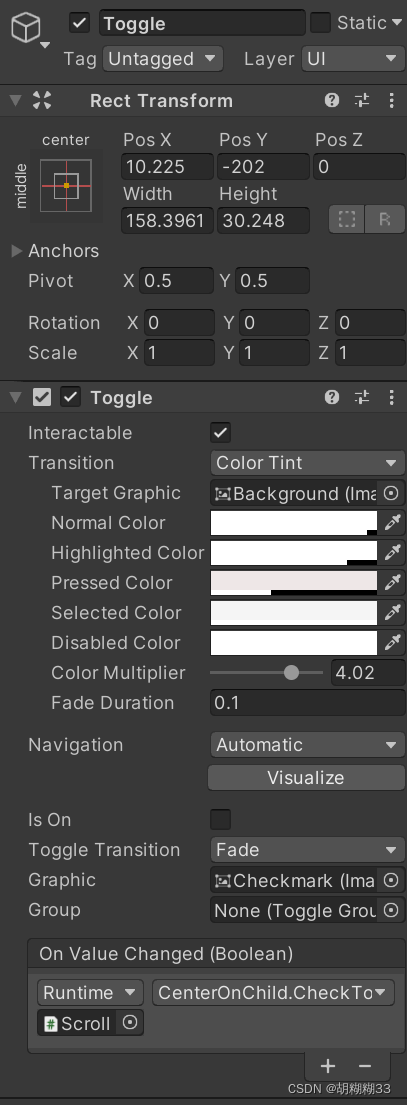 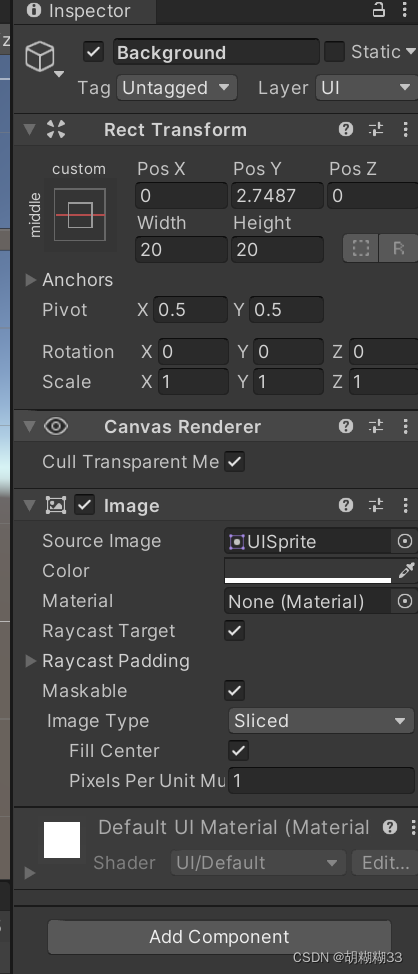 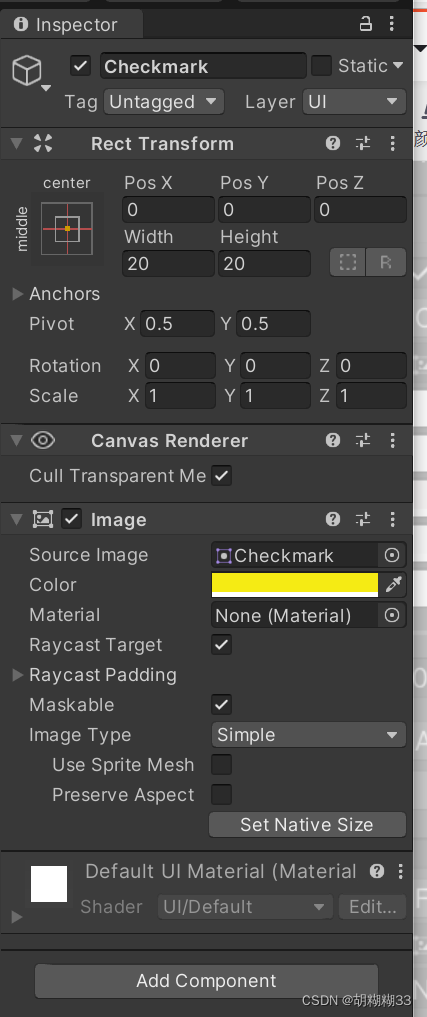 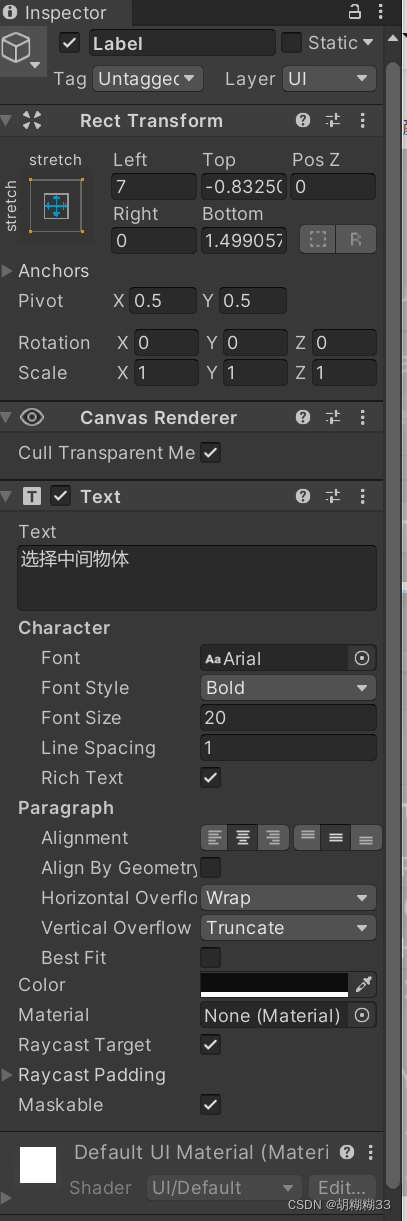
?最终结果如图:
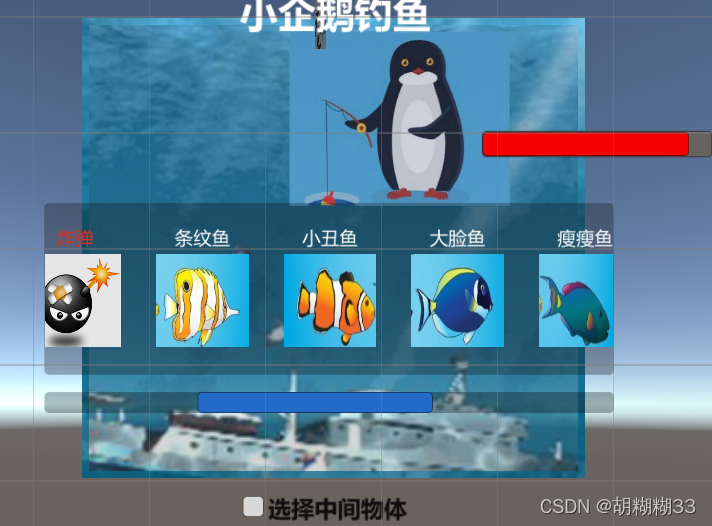
代码部分
有两个部分的代码:
- CenterOnChild:将离中间框最近的物体移动到框中
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.EventSystems;
using UnityEngine.UI;
public class CenterOnChild : MonoBehaviour, IEndDragHandler, IDragHandler
{
private ScrollRect scrollView;
private Transform container;
private List<float> childrenPos = new List<float>();
private float targetPos;
private bool centering = false;
private bool drag = false;
private bool check = false;
public float speed = 10f;
public GameObject panel;
void Awake()
{
scrollView = GetComponent<ScrollRect>();
container = scrollView.content;
GridLayoutGroup grid = container.GetComponent<GridLayoutGroup>();
float childPos = scrollView.GetComponent<RectTransform>().rect.width * 0.5f - grid.cellSize.x * 0.5f;
childrenPos.Add(childPos);
for (int i = 0; i < container.childCount - 1; i++)
{
childPos -= grid.cellSize.x + grid.spacing.x;
childrenPos.Add(childPos);
}
}
void Update()
{
if (centering && Input.GetAxis("Mouse ScrollWheel")==0 && check)
{
Vector3 vec = container.localPosition;
vec.x = Mathf.Lerp(container.localPosition.x, targetPos, speed * Time.deltaTime);
container.localPosition = vec;
if (Mathf.Abs(container.localPosition.x - targetPos) < 0.01f)
{
centering = false;
}
}
}
public void OnEndDrag(PointerEventData eventData)
{
centering = true;
drag = false;
targetPos = FindClosestPos(container.localPosition.x);
}
public void OnDrag(PointerEventData eventData)
{
drag = true;
centering = false;
}
public void ScrollbarDrag()
{
if(!drag)
{
centering = true;
targetPos = FindClosestPos(container.localPosition.x);
}
}
private float FindClosestPos(float currentPos)
{
float closest = 0;
float distance = Mathf.Infinity;
for (int i = 0; i < childrenPos.Count; i++)
{
float pos = childrenPos[i];
float dis = Mathf.Abs(pos - currentPos);
if (dis < distance)
{
distance = dis;
closest = pos;
}
}
return closest;
}
public void CheckToggle()
{
check = !check;
if(check)
{
centering = true;
targetPos = FindClosestPos(container.localPosition.x);
panel.SetActive(true);
}
else
{
panel.SetActive(false);
}
}
}
using UnityEngine;
using UnityEngine.EventSystems;
using UnityEngine.UI;
using System.Collections;
public class DragMove : MonoBehaviour, IBeginDragHandler, IDragHandler, IEndDragHandler
{
public void OnBeginDrag(PointerEventData eventData)
{
SetPosition(eventData);
}
public void OnDrag(PointerEventData eventData)
{
SetPosition(eventData);
}
public void OnEndDrag(PointerEventData eventData)
{
SetPosition(eventData);
}
private void SetPosition(PointerEventData eventData)
{
var rectTransform = gameObject.GetComponent<RectTransform>();
Vector3 globalMousePos;
if (RectTransformUtility.ScreenPointToWorldPointInRectangle(rectTransform, eventData.position, eventData.pressEventCamera, out globalMousePos))
{
rectTransform.position = globalMousePos;
}
}
}
血条部分
UI部分
在Canvas中添加一个外部血条组件,在外部血条下再建一个内部血条。外部血条是血条框的外部,内部血条是真正移动的血液部分,在内部血条中设置血液颜色为红色。
??????????????????????????????????????? ? ??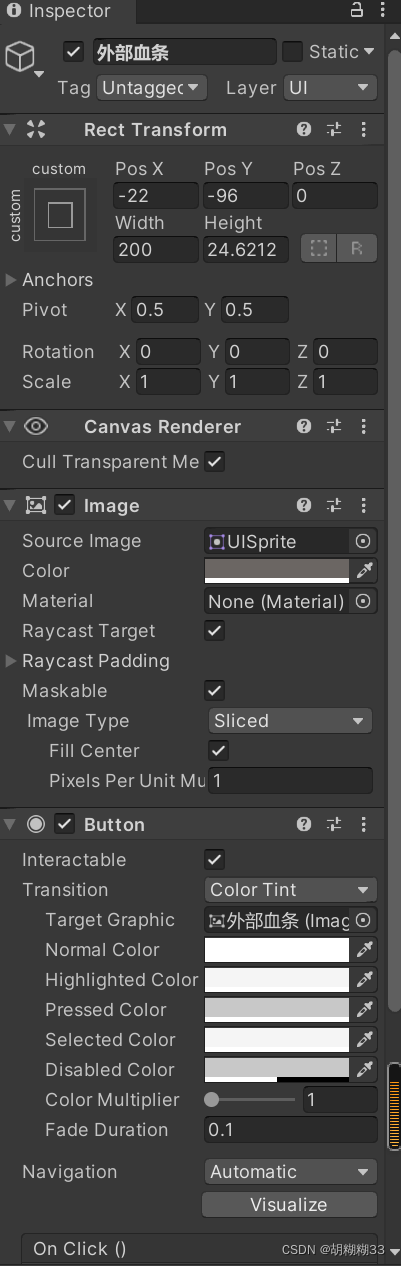 ???????
?最终结果如图:
???????????????????????????????????????????????????????????????????????????? ? ?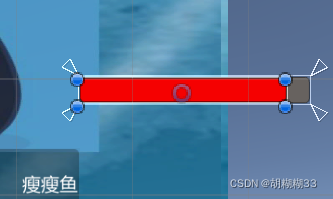
代码部分
有两个部分代码:
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class UGUI : MonoBehaviour {
public RectTransform rectBloodPos;
public RectTransform blood;
public int reduceBlood = 0;
void Update()
{
this.gameObject.transform.Translate(Input.GetAxis("Horizontal") * 10 * Time.deltaTime, 0, 0);
this.gameObject.transform.Translate(0, 0, Input.GetAxis("Vertical") * 10 * Time.deltaTime);
Vector2 vec2 = Camera.main.WorldToScreenPoint(this.gameObject.transform.position);
blood.GetComponent<RectTransform>().Right(reduceBlood);
rectBloodPos.anchoredPosition = new Vector2(vec2.x - Screen.width / 2 + 0, vec2.y - Screen.height / 2 + 60);
}
private void OnGUI()
{
if (GUI.Button(new Rect(530, 420, 55, 20), "选小鱼"))
{
reduceBlood -= 10;
if(reduceBlood < 0)
{
reduceBlood = 0;
}
}
if (GUI.Button(new Rect(470, 420, 55, 20), "选炸弹"))
{
reduceBlood += 10;
if(reduceBlood > 200)
{
reduceBlood = 200;
}
}
}
}
using UnityEngine;
public static class ExtensionMethod
{
public static void Left(this RectTransform rTrans, int value)
{
rTrans.offsetMin = new Vector2(value, rTrans.offsetMin.y);
}
public static void Right(this RectTransform rTrans, int value)
{
rTrans.offsetMax = new Vector2(-value, rTrans.offsetMax.y);
}
public static void Bottom(this RectTransform rTrans, int value)
{
rTrans.offsetMin = new Vector2(rTrans.offsetMin.x, value);
}
public static void Top(this RectTransform rTrans, int value)
{
rTrans.offsetMax = new Vector2(rTrans.offsetMax.x, -value);
}
}
|