本文要解决的问题:
1、如何用代码初始化下拉菜单的item 2、如何乱序加载item 3、如何读取当前选中的item的值
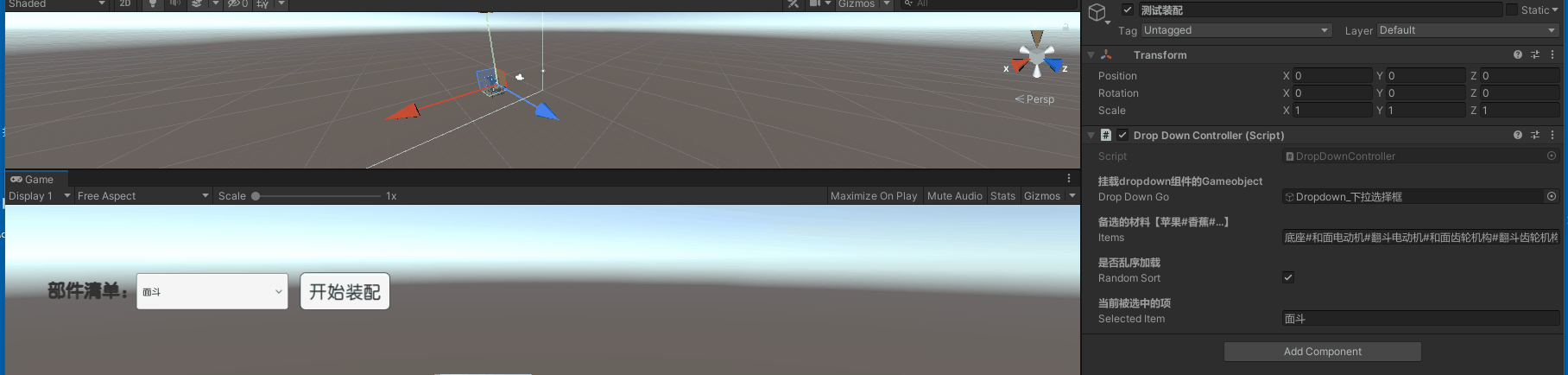
一、下拉框外形——Dropdown
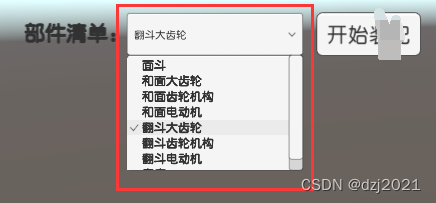
二、脚本的配置
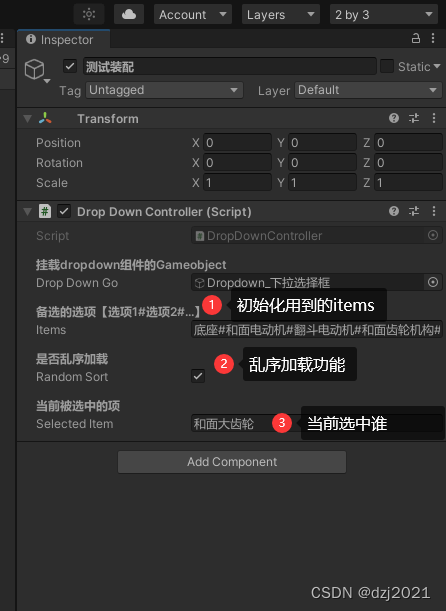
三、功能实现
1、初始化Dropdown的下拉列表
void AddItmes(string strName)
{
m_Index = m_Messages.Count;
Dropdown.OptionData temp = new Dropdown.OptionData();
temp.text = strName;
m_Messages.Add(temp);
m_Dropdown.options.Insert(m_Index, temp);
}
2、乱序排列一个列表
private List<T> RandomSort<T>(List<T> list)
{
var random = new System.Random();
var newList = new List<T>();
foreach (var item in list)
{
newList.Insert(random.Next(newList.Count), item);
}
return newList;
}
3、读取当前的item
关键点: 获取当前项: currentItem = GetComponent().options[idx].text
当前项的idx从哪里来: idx = GetComponent().value
void SetSelectedItem()
{
var dp = dropDownGo.GetComponent<Dropdown>();
var idx = dp.value;
selectedItem = dp.options[idx].text;
}
四、代码清单
using UnityEngine;
using UnityEngine.UI;
using System.Collections.Generic;
using System.Linq;
using System;
public class DropDownController : MonoBehaviour
{
[Header("挂载dropdown组件的Gameobject")]
[SerializeField]
public GameObject dropDownGo;
[Header("备选的选项【选项1#选项2#...】")]
[SerializeField]
public string items;
[Header("是否乱序加载")]
[SerializeField]
public bool randomSort;
[Header("当前被选中的项")]
[SerializeField]
public string selectedItem;
Dropdown.OptionData m_NewData, m_NewData2;
List<Dropdown.OptionData> m_Messages = new List<Dropdown.OptionData>();
Dropdown m_Dropdown;
string m_MyString;
int m_Index;
private void OnEnable()
{
dropDownGo.GetComponent<Dropdown>().onValueChanged.AddListener(delegate { SetSelectedItem(); });
}
void Start()
{
m_Dropdown = dropDownGo.GetComponent<Dropdown>();
m_Dropdown.ClearOptions();
var finItems = randomSort ?
RandomSort(items.Split('#').ToList()).ToArray() : items.Split('#');
LoadItems(finItems);
selectedItem = finItems[0];
}
void AddItmes(string strName)
{
m_Index = m_Messages.Count;
Dropdown.OptionData temp = new Dropdown.OptionData();
temp.text = strName;
m_Messages.Add(temp);
m_Dropdown.options.Insert(m_Index, temp);
}
void LoadItems(string[] ary)
{
ary.ToList().ForEach(x=>AddItmes(x));
}
void SetSelectedItem()
{
var dp = dropDownGo.GetComponent<Dropdown>();
var idx = dp.value;
selectedItem = dp.options[idx].text;
}
private List<T> RandomSort<T>(List<T> list)
{
var random = new System.Random();
var newList = new List<T>();
foreach (var item in list)
{
newList.Insert(random.Next(newList.Count), item);
}
return newList;
}
}
|