前期准备
不管在调用什么SDK,都得先查看相应的SDK文档,本人比较懒,就直接贴出百度人脸检测的文档内容。之后跟着文档一步一步的去实现。 可以看到SDK的功能有人脸检测、人脸对比和查找人脸。本篇主要实现人脸检测的功能。 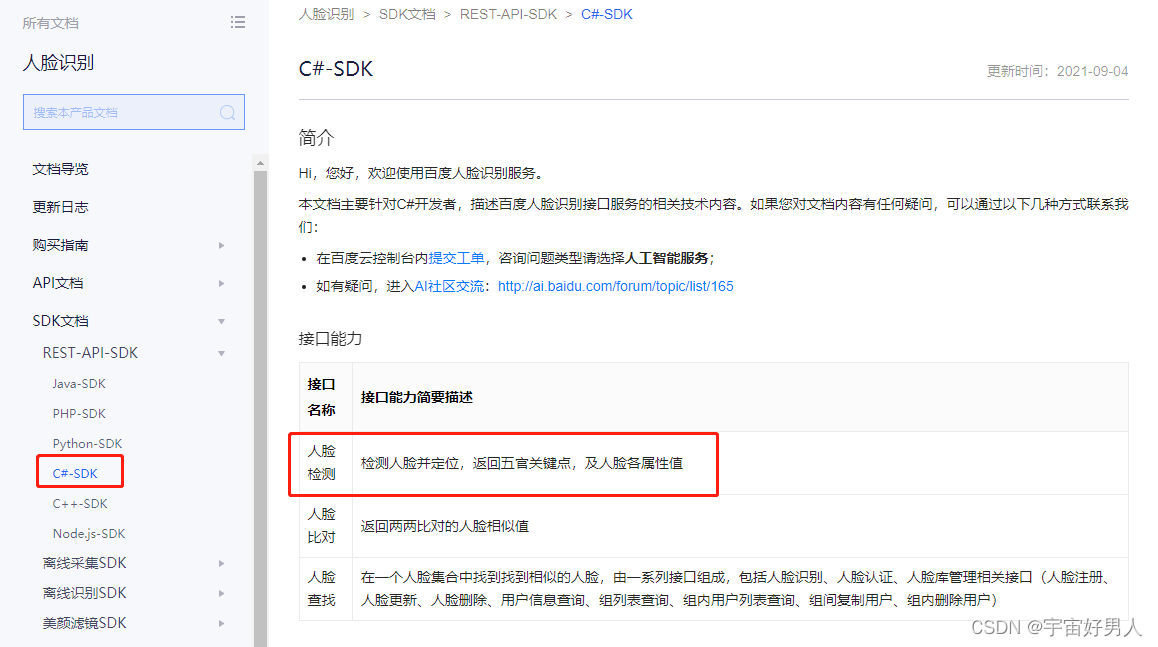 选择方法二进行SDK的下载安装 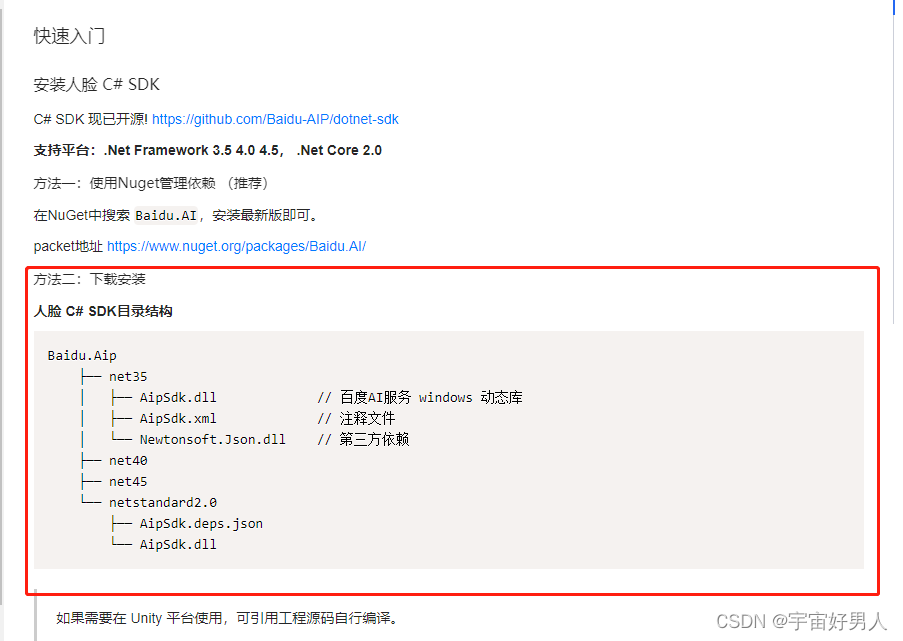 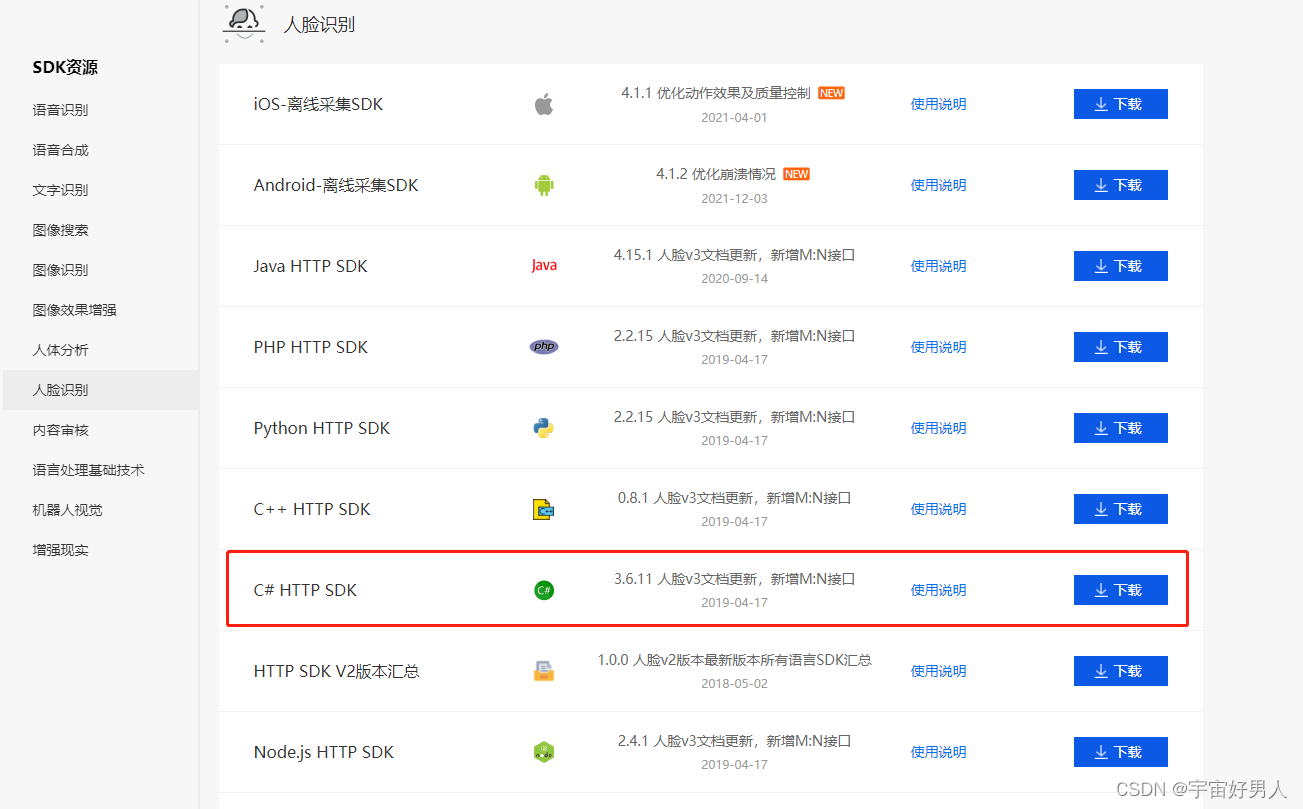 在下载好的SDK里面找到net40文件夹中的AipSdk.dll和Newtonsoft.Json.dll导入Unity工程中 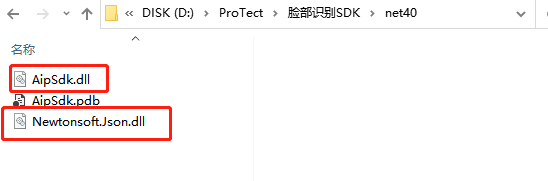 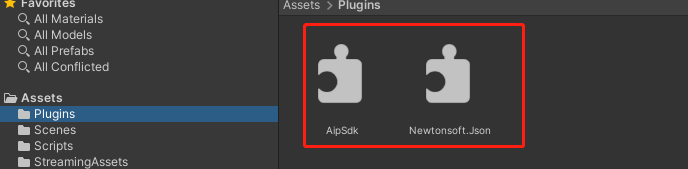 编写代码调用摄像头,根据SDK文档参数选择,实现实时上传图片接收返回相应数据: 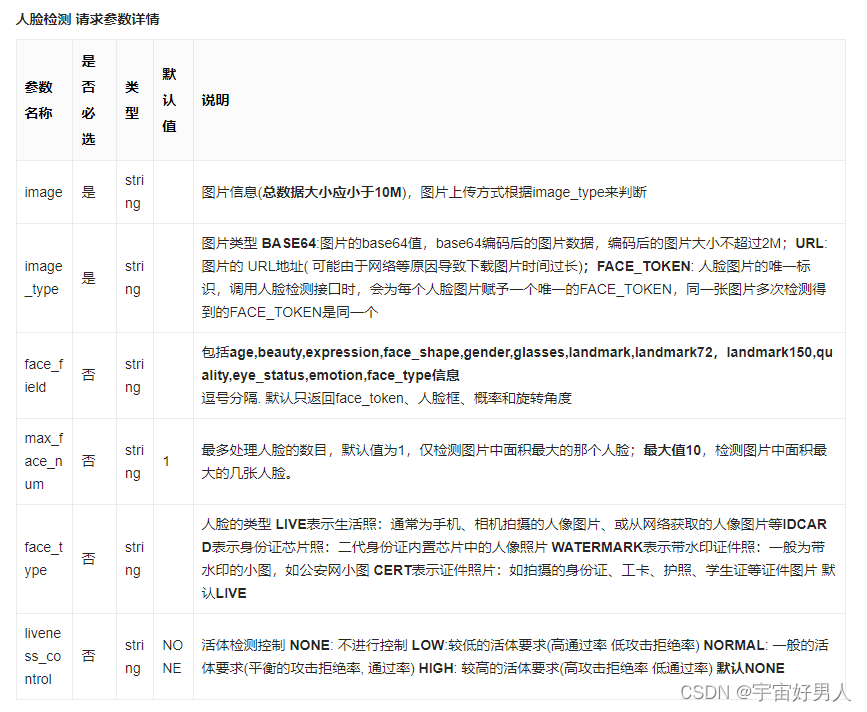 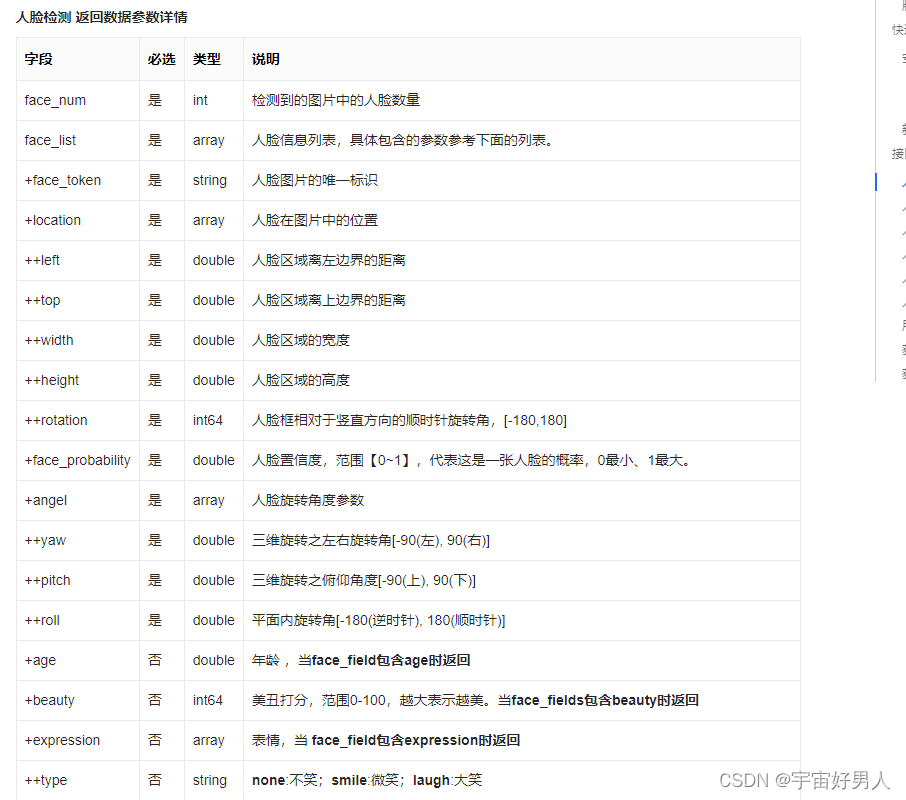 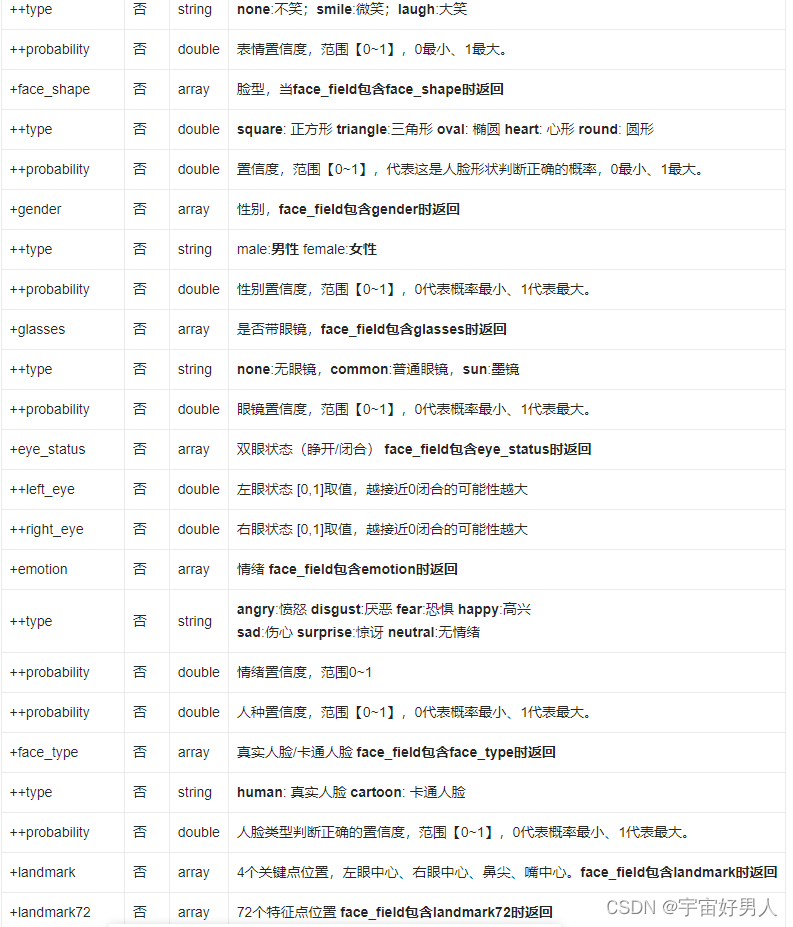 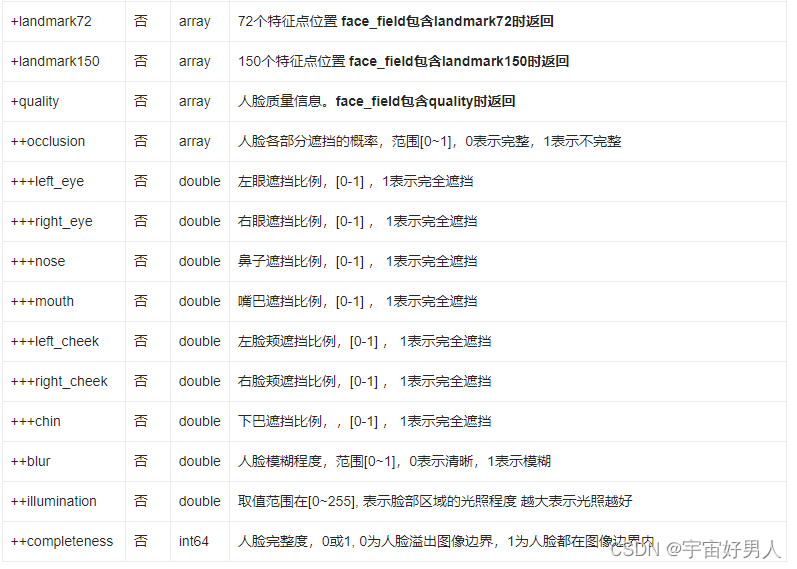 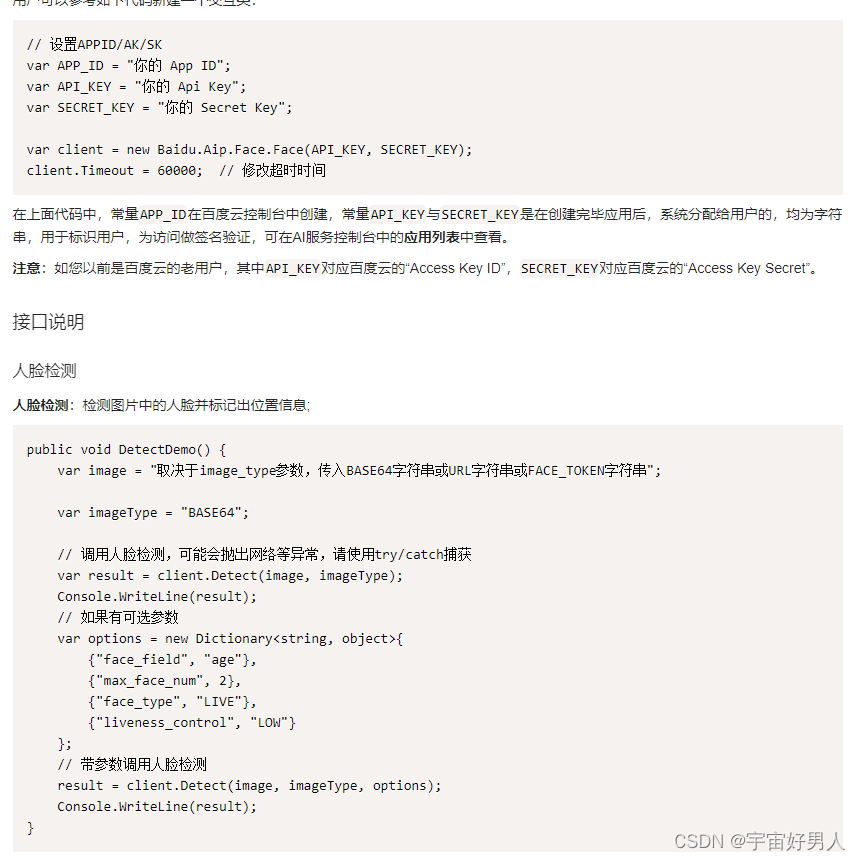
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using Baidu.Aip.Face;
using UnityEngine.UI;
using System.IO;
using System;
using System.Text;
public class FaceDetect : MonoBehaviour
{
public string app_id;
public string api_key;
public string secret_key;
Face client;
private string devicename;
private WebCamTexture webCamTex;
float timer = 1f;
RawImage mainImage;
public Camera cam;
string imagename = Application.streamingAssetsPath + "/" + "face.jpg";
public int width;
public int height;
private int Frame = 30;
public Text resultText;
string result;
bool isStart=false;
void Start()
{
StartCoroutine(OpenCamera());
mainImage = transform.GetComponent<RawImage>();
client = new Face(api_key,secret_key);
client.Timeout = 60000;
}
IEnumerator OpenCamera()
{
yield return Application.RequestUserAuthorization(UserAuthorization.WebCam);
if (Application.HasUserAuthorization(UserAuthorization.WebCam))
{
WebCamDevice[] devices = WebCamTexture.devices;
devicename = devices[0].name;
webCamTex = new WebCamTexture(devicename,width,height,Frame);
webCamTex.Play();
mainImage.texture = webCamTex;
isStart = true;
}
}
private void Update()
{
if (isStart)
{
isStart = false;
StartCoroutine(CupScreenImage());
}
}
IEnumerator CupScreenImage()
{
while (true)
{
yield return new WaitForSeconds(timer);
if (File.Exists(imagename))
{
File.Delete(imagename);
}
Texture2D screenShot;
RenderTexture rt = new RenderTexture(width,height,1);
cam.targetTexture = rt;
cam.Render();
RenderTexture.active = rt;
screenShot = new Texture2D(width, height, TextureFormat.RGB24, false);
screenShot.ReadPixels(new Rect(0,0,width,height),0,0);
screenShot.Apply();
SaveImage(screenShot);
}
}
void SaveImage(Texture2D tex)
{
Color[] pixels = new Color[(int)(width * height)];
Texture2D newTex = new Texture2D(Mathf.CeilToInt(width), Mathf.CeilToInt(height));
pixels = tex.GetPixels(0, 0, (int)width, (int)height);
newTex.SetPixels(pixels);
newTex.anisoLevel = 2;
newTex.Apply();
byte[] bytes = newTex.EncodeToJPG();
File.WriteAllBytes(imagename, bytes);
FaceVecDectect(bytes);
}
public void FaceVecDectect(byte[] vs)
{
string imageType = "BASE64";
if (File.Exists(imagename))
{
var options = new Dictionary<string, object>
{
{"face_field","age,beauty,gender,glasses"},
{"max_face_num",3},
{"face_type","LIVE"},
{"liveness_control","LOW"}
};
var result = client.Detect(Texture2DToBase64(vs), imageType, options);
this.result = result.ToString();
resultText.text = this.result;
}
}
public string Texture2DToBase64(byte[] texture2)
{
string str = Convert.ToBase64String(texture2);
return str;
}
}
实现效果演示: 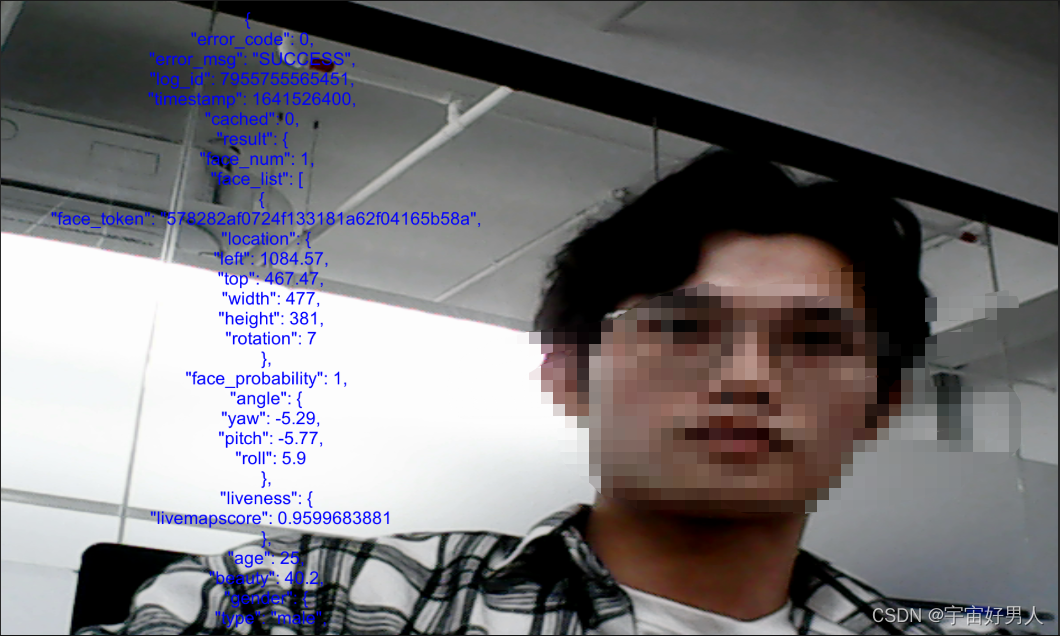
|