一、游戏目的及实现
1.完成自身坦克的移动,炮弹预设体的发射。
2.敌方坦克在场景内随机生成。
3.敌方坦克的移动,自动攻击玩家,在前方20m处无玩家时自动巡逻。
4.当炮弹触碰到敌人,敌人被摧毁。
5.主摄像机的自动跟随--3d俯视or第三人称。
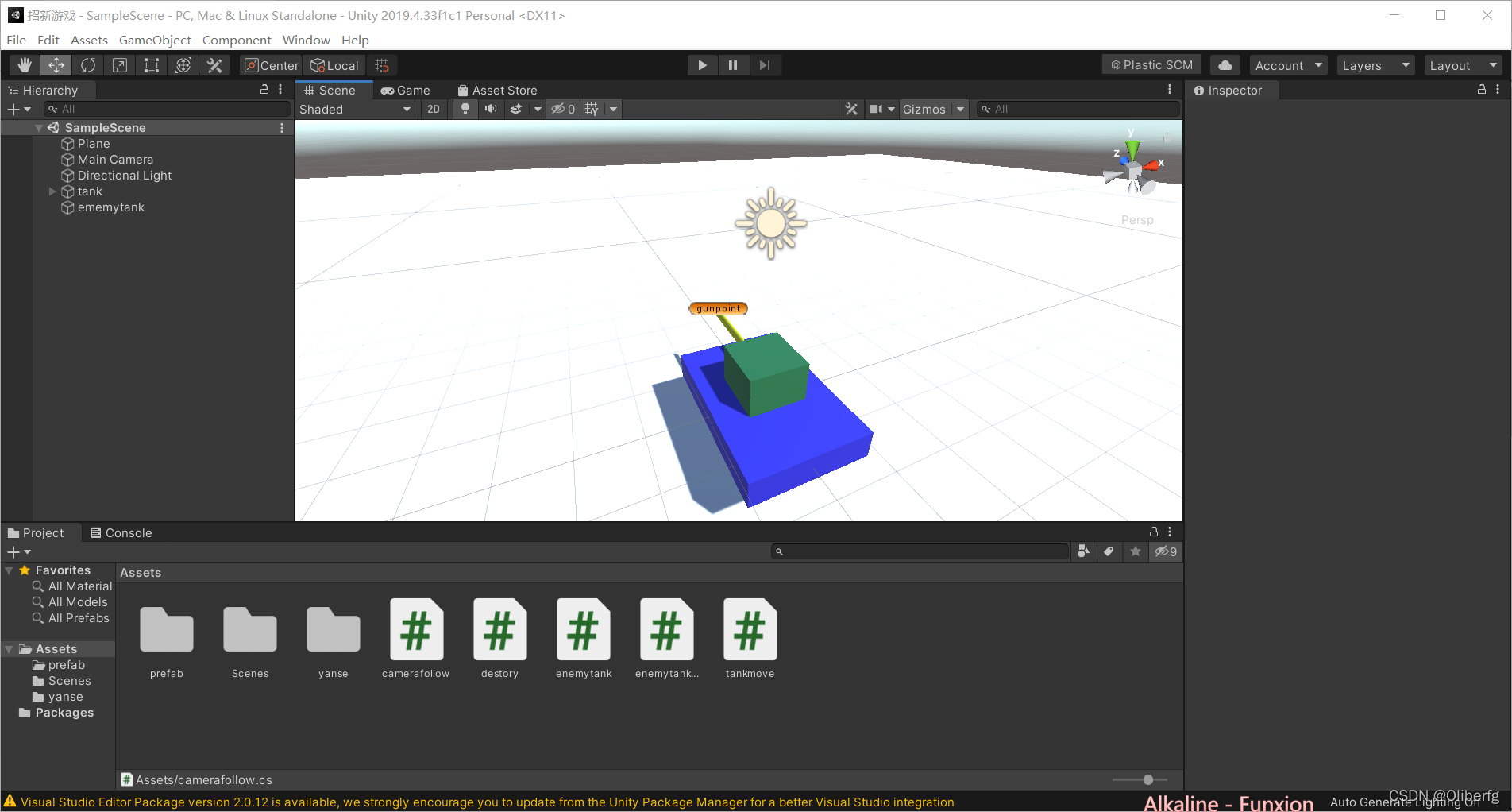
?二、源码上传
1.自身坦克移动.
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class tankmove : MonoBehaviour
{
public GameObject myprefab;
public float moveSpeed = 1;
public float returnSpeed = 1;
public float paodanfly = 10f;
float ho;
float vr;
private Transform gunpoint;
// Start is called before the first frame update
void Start()
{
gunpoint = transform.Find("Cylinder/gunpoint");
}
// Update is called once per frame
void Update()
{
//移动-转向
ho = Input.GetAxis("Horizonal");
vr = Input.GetAxis("Vertical");
transform.position += transform.forward * vr * Time.deltaTime * moveSpeed;
transform.eulerAngles += new Vector3(0, 1 * ho * returnSpeed, 0);
if (Input.GetKeyDown(KeyCode.Space))
{
Fire();
}
}
private void Fire()
{
//发射预设体
GameObject paodan = Instantiate(myprefab,gunpoint.position, Quaternion.identity);
// paodan.GetComponent<paodandong>().vdr = transform.forward;
paodan.GetComponent<Rigidbody>().velocity = transform.forward * paodanfly;
Destroy(paodan, 5f);
// paodan.transform.position = transform.position + new Vector3(0, transform.forward.y, 0);
}
}
2.随机生成,此脚本挂载在空对象中
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class enemytank : MonoBehaviour
{
[Header("敌方坦克预设体")]
public GameObject enemytankprefab;
[Header("时间限制")]
public float interval = 3f;
[Range(20,100)]
public int maxenemy = 50;
//计时器
private float timer;
//计数器
private int counter=0;
// Start is called before the first frame update
void Start()
{
}
// Update is called once per frame
void Update()
{
timer += Time.deltaTime;
if (timer > interval)
{
if (counter <= maxenemy)
{
createtank();
}
counter++;
//计时器归零
timer = 0;
}
}
private void createtank()
{
//范围
float x = Random.Range(-40f, 40f);
float z = Random.Range(-40f, 40f);
int y = Random.Range(0, 360);
Quaternion qua= Quaternion.Euler(new Vector3(0, y, 0));
GameObject direntank= Instantiate(enemytankprefab, new Vector3(x, 0, z), qua);
}
}
3.此脚本挂载至敌方坦克预设体上.
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class enemytankmove : MonoBehaviour
{
private Transform player;
private float distance;
private RaycastHit hit;
private Transform gunpoint;
private float timer;
private float y = 0;
public float turnspeed = 2f;
public float movespeed = 5f;
public float fireinterval = 3f;
public float paodanfly = 30f;
public GameObject myprefab;
// Start is called before the first frame update
void Start()
{
player = GameObject.FindWithTag("Player").transform;
gunpoint = transform.Find("Cylinder/gunpoint");
}
// Update is called once per frame
void Update()
{
timer += Time.deltaTime;
//计算距离
distance = Vector3.Distance(transform.position, player.position);
if(distance<10)
{
//开炮
fire();
}
else if(distance<20)
{
//向前逼近
MoveToPlayer();
}
else
{
MoveFree();
//瞎走,逛街
}
}
private void fire()
{
RotateToPlayer();
if(timer>fireinterval)
{
//计时器归零
timer = 0;
GameObject paodan = Instantiate(myprefab, gunpoint.position, Quaternion.identity);
//炮弹移动
paodan.GetComponent<Rigidbody>().velocity = transform.forward * paodanfly;
Destroy(paodan, 5f);
}
}
private void MoveToPlayer()
{
RotateToPlayer();
transform.position += transform.forward * Time.deltaTime * movespeed;
}
private void MoveFree()
{
transform.position += transform.forward * Time.deltaTime * movespeed;
rotate(Quaternion.Euler(Vector3.up * y));
if (timer>fireinterval)
{
y = Random.Range(0, 360);
//转向
timer = 0;
}
}
private void Rotateto(Vector3 dir)
{
Quaternion qur = Quaternion.LookRotation(dir);
rotate(qur);
}
private void RotateToPlayer()
{
Vector3 dir = player.position - transform.position;
Rotateto(dir);
}
private void rotate(Quaternion n)
{
transform.rotation = Quaternion.Lerp(transform.rotation, n, Time.deltaTime * turnspeed);
}
}
4.摧毁敌人
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class destory : MonoBehaviour
{
// Start is called before the first frame update
private void OnTriggerEnter(Collider other)
{
if(other.gameObject.tag=="diren1")
{
other.gameObject.SetActive(false);
}
}
void Start()
{
}
// Update is called once per frame
void Update()
{
}
}
5.摄像机跟随
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class camerafollow : MonoBehaviour
{
//跟随的目标
public Transform followTarget;
private Vector3 dir;
// Start is called before the first frame update
void Start()
{
//计算方向向量
dir = followTarget.position - transform.position;
}
// Update is called once per frame
void Update()
{
//时刻保持方向向量
transform.position = followTarget.position - dir;
}
}
三、总结
在编译过程中出现了种种错误和难以解决的报错,同时也体现了我对编译的不熟练及开荒试错的艰难。也感谢实验室的学长学姐。
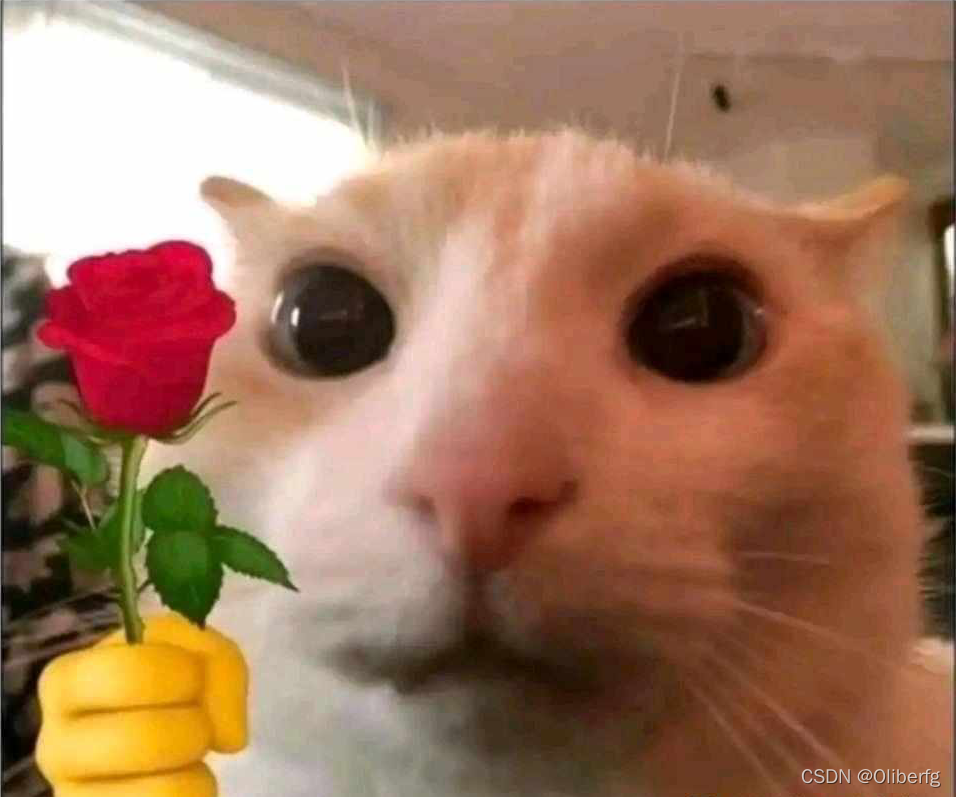
|