本讲进行Unity的第二个案例练习,预计10个小时,会同时更新博客。熟悉Unity的一些简单操作。
1 搭建环境
1.1 设计地面
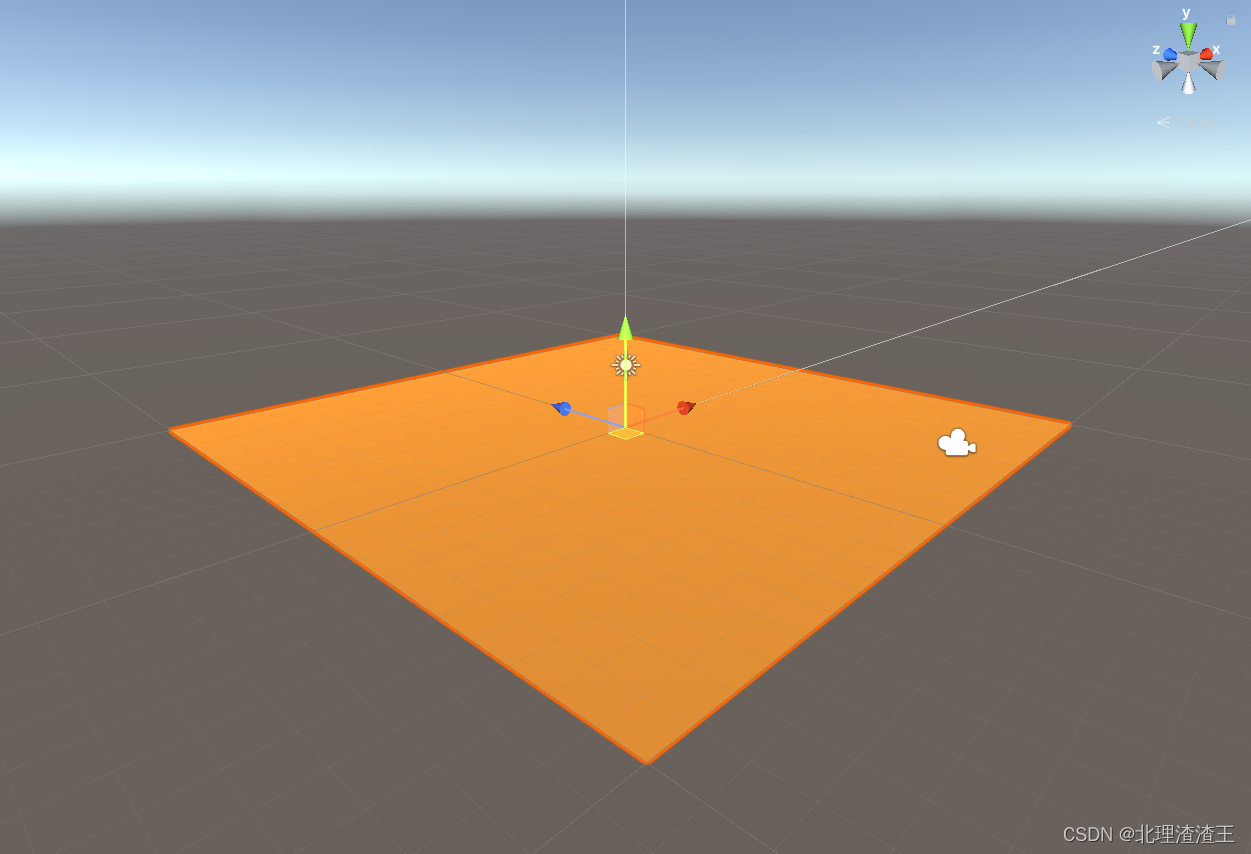
首先,创建20x20一个平面,平面Plane默认是10x10的,所以平面Scale的x和z轴的值设为2 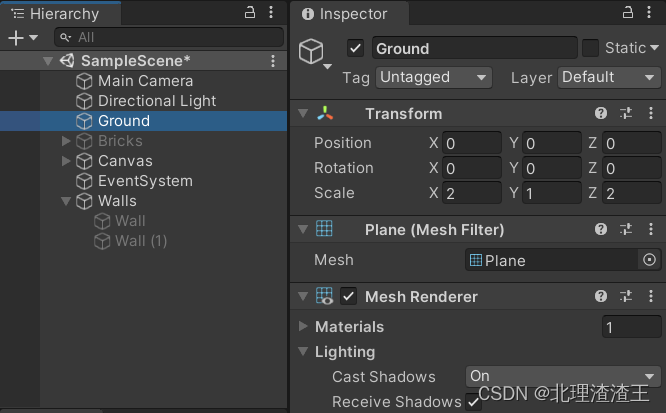 接着,为地面添加材料和颜色 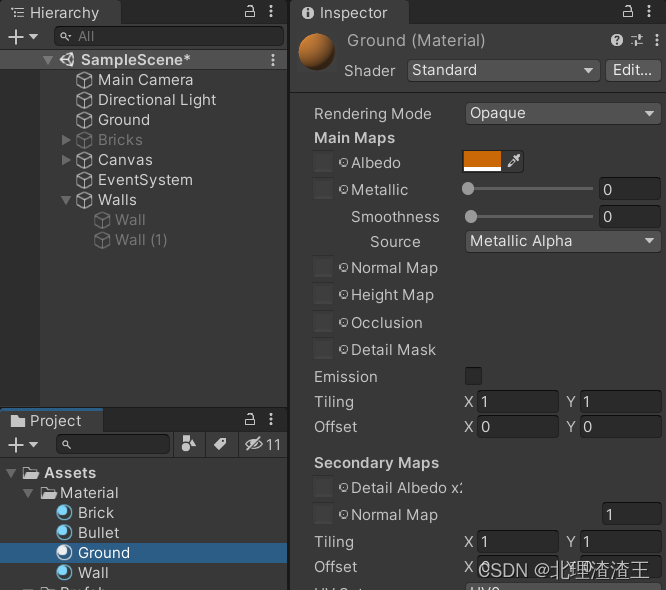
1.2 设计墙体和砖块
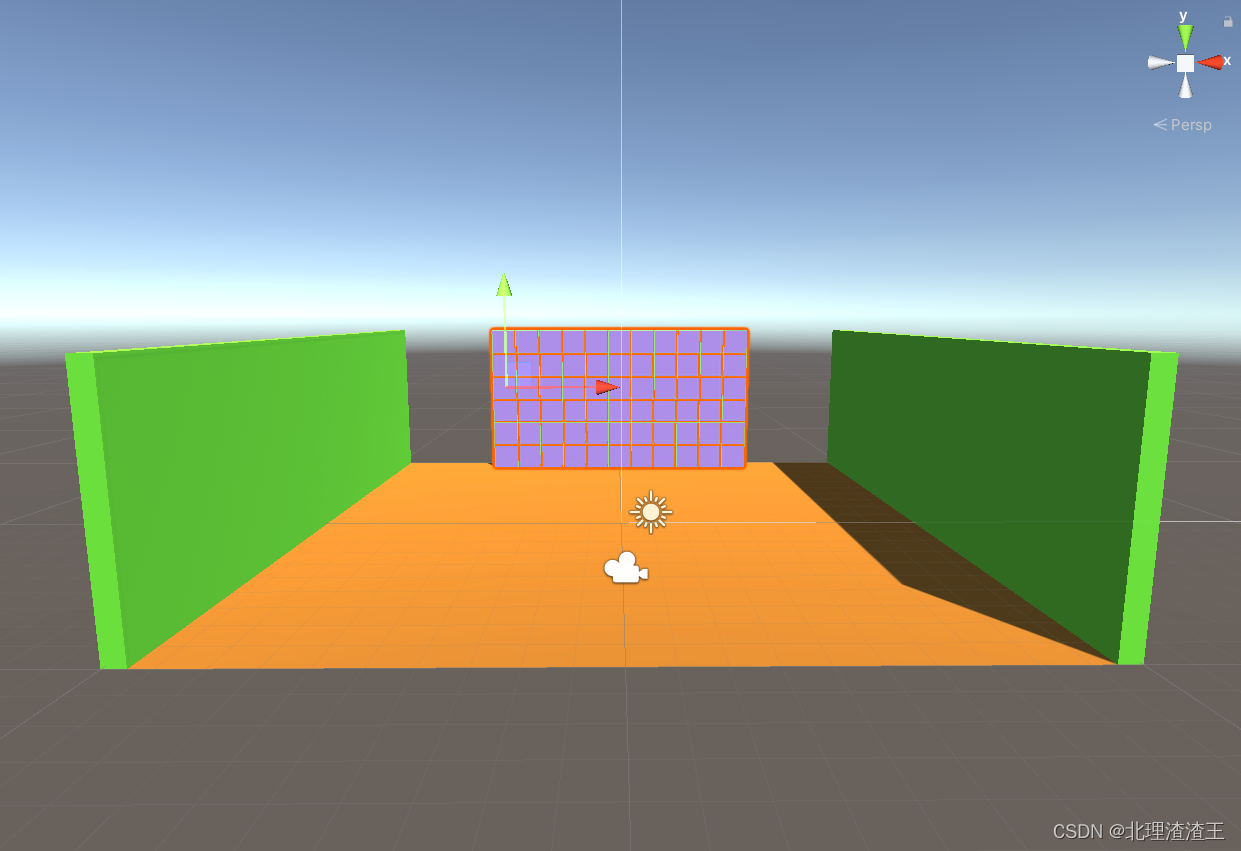 设计的墙体和砖块如上图所示。砖块采用预制体设计(Ctrl+D可以复制物体),Ctrl+鼠标左键可以控制物体进行步移(Edit ->Grid and Snap Settings可以设置步移距离) 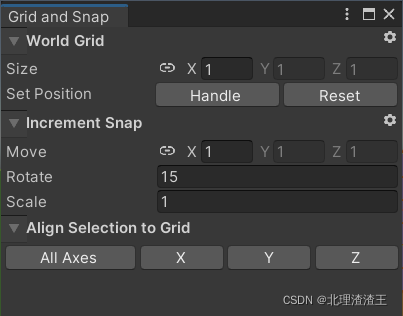 砖块可以建立一个空物体作为父物体,方便管理 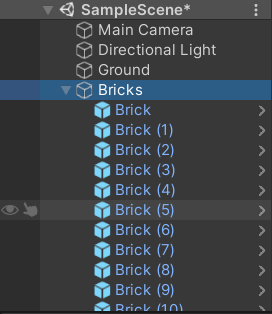
2 相机移动(第一人称视角移动)
相机应该能够根据方向键的按下进行移动。
2.1 初始化位置
相机的初始位置应该正对砖块墙 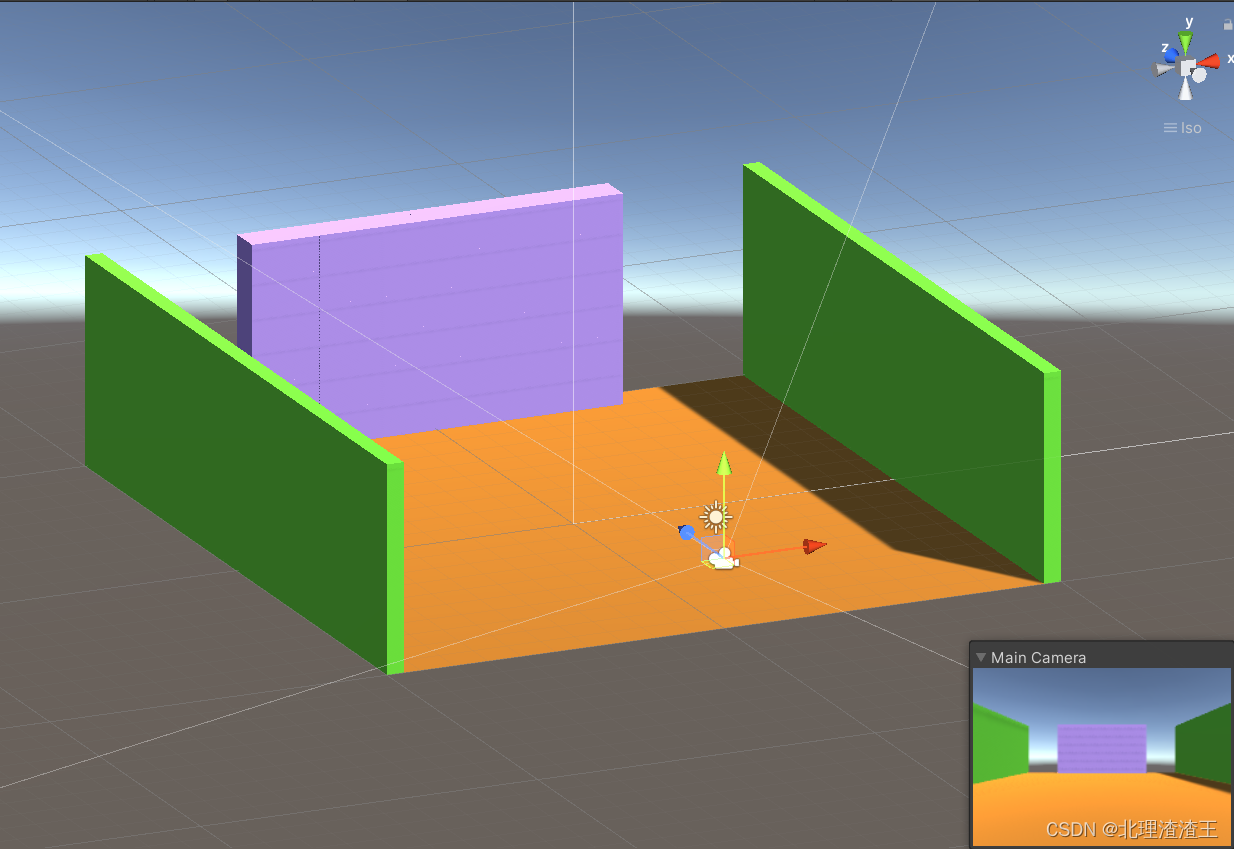
2.2 位置更新策略
相机的水平移动(Horizontal)沿 x 轴方向,垂直移动(Vertical)沿 y 轴方向
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class Movement : MonoBehaviour
{
public int speed = 1;
void Update()
{
float h = Input.GetAxis("Horizontal");
float v = Input.GetAxis("Vertical");
transform.Translate(new Vector3(h, v, 0)*speed*Time.deltaTime);
}
private void FixedUpdate()
{
Debug.Log("FPS:"+1 / Time.deltaTime);
}
}
3 创建子弹(小球)
我们希望创建的小球初始位置与相机位置重合,并且沿 z 轴方向以一定的初始速度射出 子弹的创建采用预制体实例化的方式,子弹预制体采用红色Sphere设计 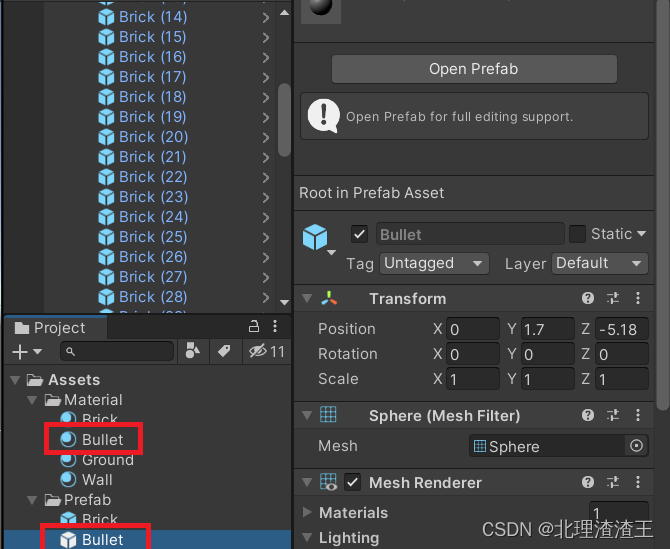
4 游戏程序设计
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.UI;
public class Shoot : MonoBehaviour
{
public GameObject bulletPrefab;
public int speed = 30;
public Text countText;
public Text winText;
private int count=0;
private bool isGameOver = false;
GameObject[] bricks = new GameObject[66];
Transform[] Ts = new Transform[66];
void Start()
{
bricks = GameObject.FindGameObjectsWithTag("Brick");
for (int i = 0; i < 66; i++)
{
Ts[i]=bricks[i].GetComponent<Transform>();
}
}
void Update()
{
if (Input.GetMouseButtonDown(0))
{
GameObject bullet=GameObject.Instantiate(bulletPrefab, transform.position, transform.rotation);
Rigidbody rd = bullet.GetComponent<Rigidbody>();
rd.velocity = Vector3.forward*speed;
count++;
countText.text = "消耗子弹数:" + count;
}
int brickCount = 0;
for (int i = 0; i < 66; i++)
{
if (Ts[i].position.y > -6)
{
brickCount++;
}
}
if (brickCount == 0)
isGameOver = true;
if(isGameOver == true)
{
winText.text = "Win the Game!\nYour score is:" + count;
}
}
}
5 游戏整体展示
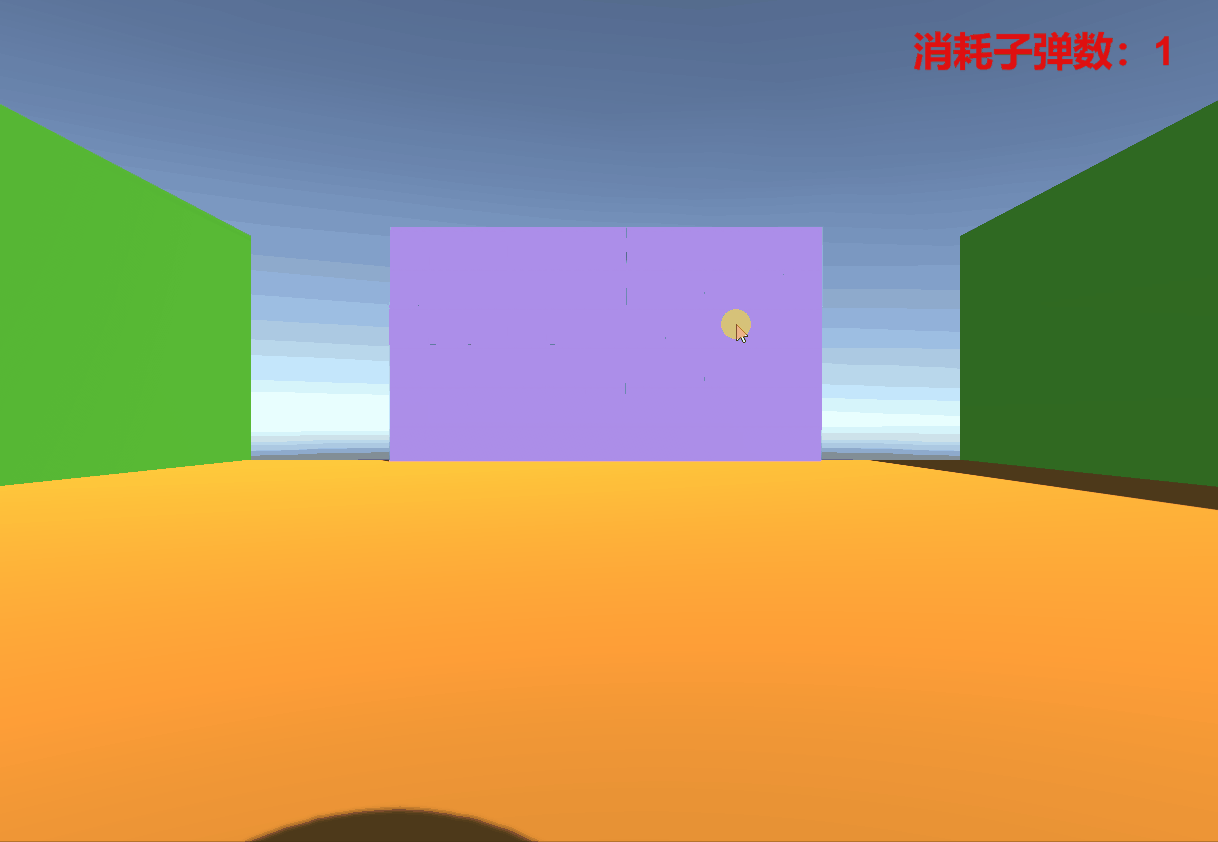 本次的打砖块游戏主要涉及到预制体设计、步移移动、键盘控制、鼠标左键点击控制、位置变化、标签搜索、文字UI设计等相关的Unity知识
|