Player游戏物体
从官方案例中获取模型并导入到Unity引擎中,给Player加上碰撞体和刚体组件,使其碰撞到敌人,敌人子弹或者陨石时爆炸,同时给Player增加碰撞时的爆炸粒子特效。 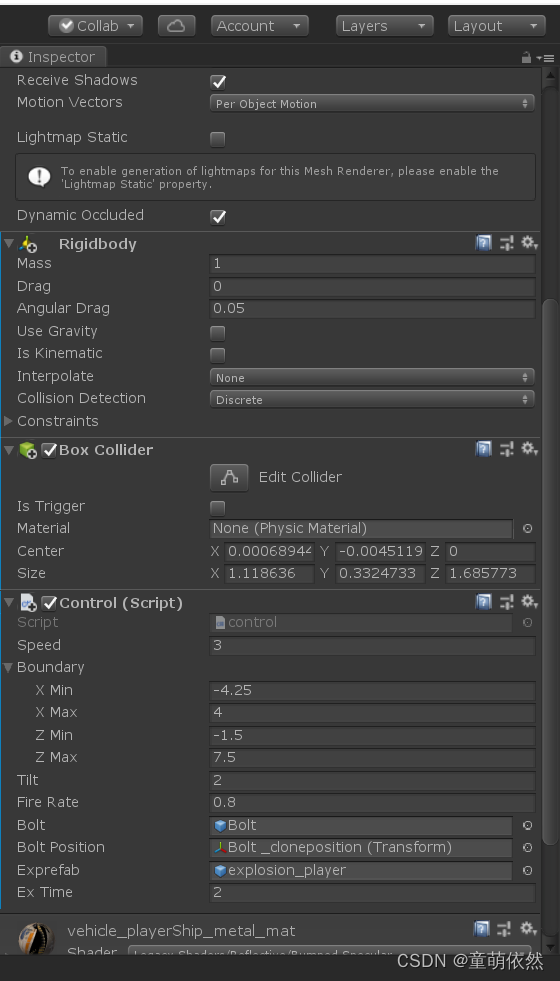 再给Player增加脚本实现上述功能
public class control : MonoBehaviour
{
public float Speed = 3F;
public Boundary boundary;
public float tilt = 2F;
private Rigidbody rb;
public float FireRate = 0.8F;
public GameObject Bolt;
public Transform BoltPosition;
private float NextFire = 0F;
public GameObject Exprefab;
public float ExTime = 2;
void Start()
{
rb = this.GetComponent<Rigidbody>();
}
void Update()
{
if (Input.GetButton("Fire1")&&Time.time>NextFire)
{
NextFire = Time.time + FireRate;
Instantiate(Bolt, BoltPosition.position, BoltPosition.rotation);
}
}
void FixedUpdate()
{
float floMoveHorizontal = Input.GetAxis("Horizontal");
float floMoveVertiacl = Input.GetAxis("Vertical");
Vector3 move = new Vector3(floMoveHorizontal, 0, floMoveVertiacl);
if (rb!=null)
{
rb.velocity= move * Speed;
rb.position = new Vector3(Mathf.Clamp(rb.position.x,boundary.xMin,boundary.xMax),
0.0F,
Mathf.Clamp(rb.position.z,boundary.zMin,boundary.zMax));
rb.rotation = Quaternion.Euler(0.0F,0.0F,rb.velocity.x*-tilt);
}
}
private void OnTriggerEnter(Collider other)
{
if (other.CompareTag("Enemy")|| other.CompareTag("Asteroid")||other.CompareTag("Enemybolt"))
{
GameObject ex = Instantiate(Exprefab, transform.position, Quaternion.identity);
Destroy(other.gameObject);
Destroy(gameObject);
Destroy(ex, ExTime);
GameControl.Instance.isGameOver = true;
}
}
}
陨石游戏物体
陨石是玩家要进行击碎的,并且撞击到玩家的子弹时会爆炸,并且陨石爆炸后会有爆炸效果,给陨石增加了刚体和碰撞体但是,需要让碰撞体的Is Trigger勾选,否则不会触发检测器,陨石碰撞后不会被销毁。 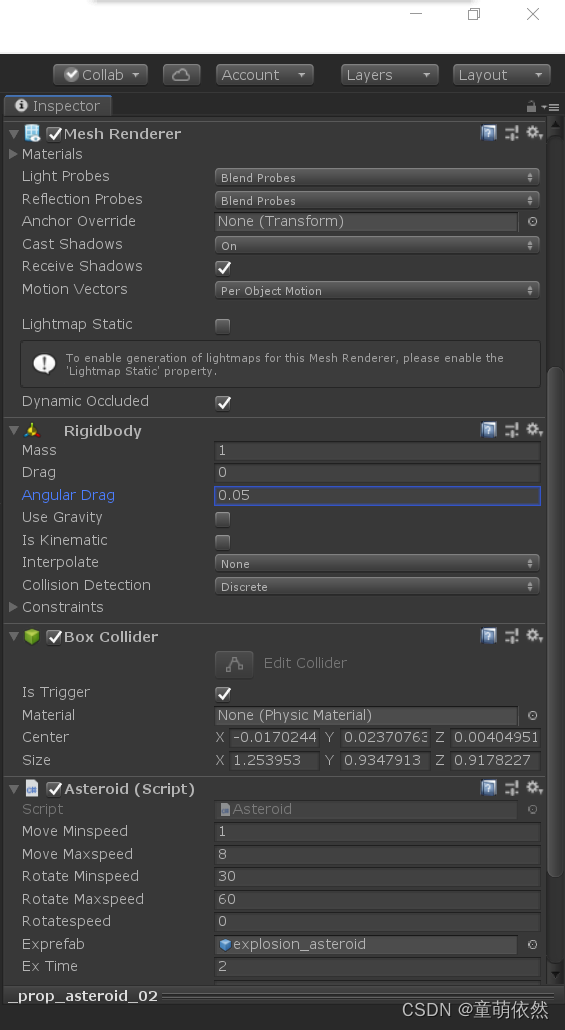
并给陨石增加脚本实现上述功能
public class Asteroid : MonoBehaviour
{
public float moveMinspeed=1F;
public float moveMaxspeed = 8F;
public float RotateMinspeed = 30F;
public float RotateMaxspeed = 60F;
public float Rotatespeed;
public GameObject Exprefab;
public float ExTime=2;
private float movespeed;
public float DestroyTime = 30F;
private float CurrentTime = 0F;
Rigidbody rb;
void Start()
{
rb = GetComponent<Rigidbody>();
movespeed = Random.Range(moveMinspeed, moveMaxspeed);
rb.velocity = Vector3.back * movespeed;
rb.angularVelocity = Random.insideUnitSphere.normalized * Random.Range(RotateMinspeed, RotateMaxspeed) * Time.deltaTime;
}
void Update()
{
CurrentTime += Time.deltaTime;
if (CurrentTime >= DestroyTime)
{
Destroy(this.gameObject);
}
}
private void OnTriggerEnter(Collider other)
{
if (other.CompareTag("bolt")||other.CompareTag("Player"))
{
GameObject ex= Instantiate(Exprefab, transform.position, Quaternion.identity);
Destroy(other.gameObject);
Destroy(gameObject);
Destroy(ex, ExTime);
GameControl.Instance.score += 10;
}
}
}
敌机游戏物体
设置敌机可以发射子弹,并且碰撞到玩家子弹和玩家时会爆炸,且碰撞后会出现粒子效果,给敌机加上刚体和碰撞体,且增加检测器,使物体接触时摧毁。 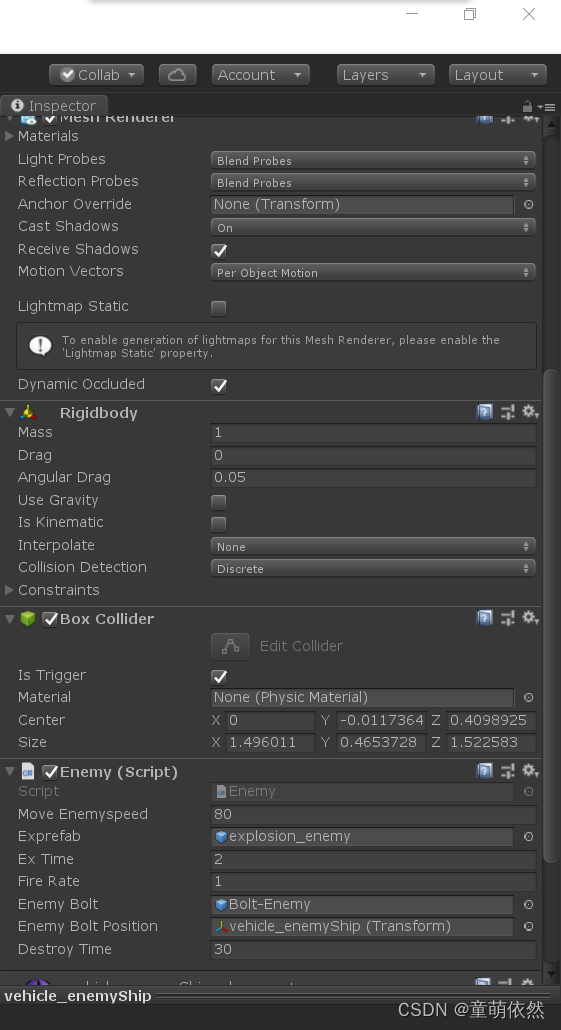 给敌机添加脚本实现上述功能
public class Enemy : MonoBehaviour
{
public float moveEnemyspeed=20F;
public GameObject Exprefab;
public float ExTime = 2;
Rigidbody rb;
public float FireRate = 1F;
public GameObject EnemyBolt;
public Transform EnemyBoltPosition;
private float NextFire = 0F;
public float DestroyTime = 30F;
private float CurrentTime = 0F;
void Start()
{
}
void Update()
{
if (Time.time > NextFire)
{
NextFire = Time.time + FireRate;
NextFire = Time.time + FireRate;
Instantiate(EnemyBolt, EnemyBoltPosition.position, EnemyBoltPosition.rotation);
}
this.GetComponent<Rigidbody>().velocity = Vector3.back * moveEnemyspeed * Time.deltaTime;
CurrentTime += Time.deltaTime;
if (CurrentTime >= DestroyTime)
{
Destroy(this.gameObject);
}
}
private void OnTriggerEnter(Collider other)
{
if (other.CompareTag("bolt") || other.CompareTag("Player"))
{
GameObject ex = Instantiate(Exprefab, transform.position, Quaternion.identity);
Destroy(other.gameObject);
Destroy(gameObject);
Destroy(ex, ExTime);
GameControl.Instance.score += 20;
}
}
}
敌机以及玩家子弹
给子弹增加速度,以及射出位置,以及碰撞体和刚体,使其碰撞到敌机和陨石时销毁,并且子弹在飞出屏幕后在一定时间会自动销毁。 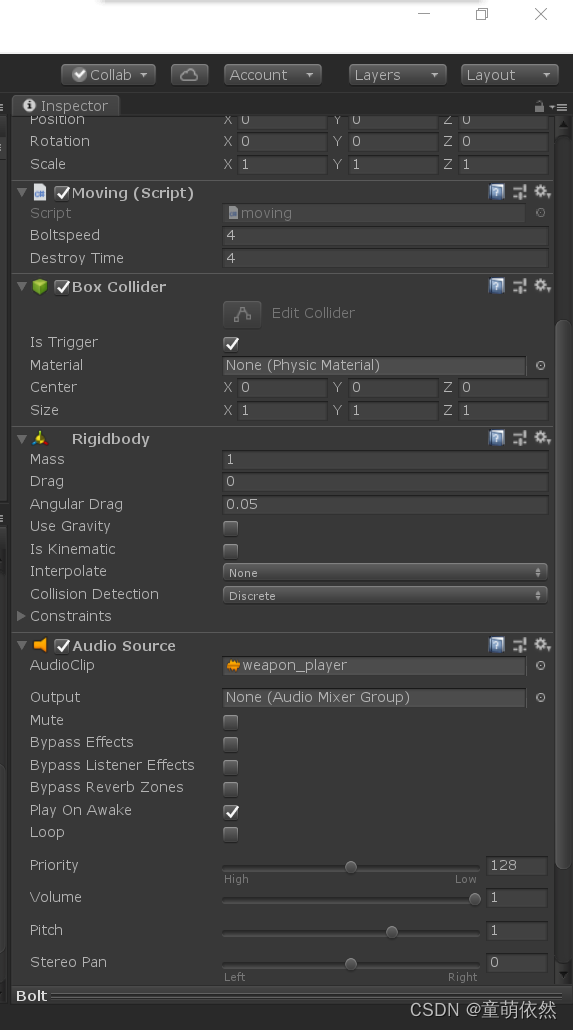 给子弹添加脚本,实现上述功能
public class moving : MonoBehaviour
{
public float boltspeed = 4F;
public float DestroyTime = 4F;
private float CurrentTime = 0F;
void Start()
{
this.GetComponent<Rigidbody>().velocity = transform.forward * boltspeed;
}
void Update()
{
CurrentTime += Time.deltaTime;
if (CurrentTime >= DestroyTime)
{
Destroy(this.gameObject);
}
}
}
游戏控制器
实现循环,使游戏持续运行。直到人物死亡后按R重来。下面是游戏控制器的脚本组件
public class GameControl : MonoBehaviour
{
public int score;
public bool isGameOver = false;
public GameObject gameover;
public GameObject[] enemyprefab;
public float StartTime = 3F;
public float enemyFate = 0.3F;
public float enemyNum = 10;
public float waveFate = 2F;
private static GameControl instance;
public static GameControl Instance
{
get { return instance; }
}
private void Awake()
{
if (instance == null)
instance = this;
}
void Start()
{
StartCoroutine("SpwanEnemy");
}
void Update()
{
if (isGameOver)
{
gameover.SetActive(true);
if (Input.GetKeyDown(KeyCode.R))
{
SceneManager.LoadScene(0);
}
}
}
IEnumerator SpwanEnemy()
{
yield return new WaitForSeconds(StartTime);
while (true)
{
for (int i = 0; i < enemyNum; i++)
{
GameObject enemy = enemyprefab[Random.Range(0, enemyprefab.Length)];
Vector3 pos = new Vector3(Random.Range(-4F, 4F), 0, 12);
Instantiate(enemy, pos, enemy.transform.rotation);
yield return new WaitForSeconds(enemyFate);
}
enemyNum++;
yield return new WaitForSeconds(waveFate);
if (isGameOver)
break;
}
}
private void OnGUI()
{
GUI.Label(new Rect(350, 0, 100, 50), "分数:" + score);
if (isGameOver)
{
GUI.Label(new Rect(0, 0, 100, 20), "GameOver");
if (isGameOver)
{
GUI.Label(new Rect(Screen.width - 200, 0, 200, 20), "请按'R'键重新开始游戏");
}
}
}
}
|