源代码
新建Pawn
创建C++类
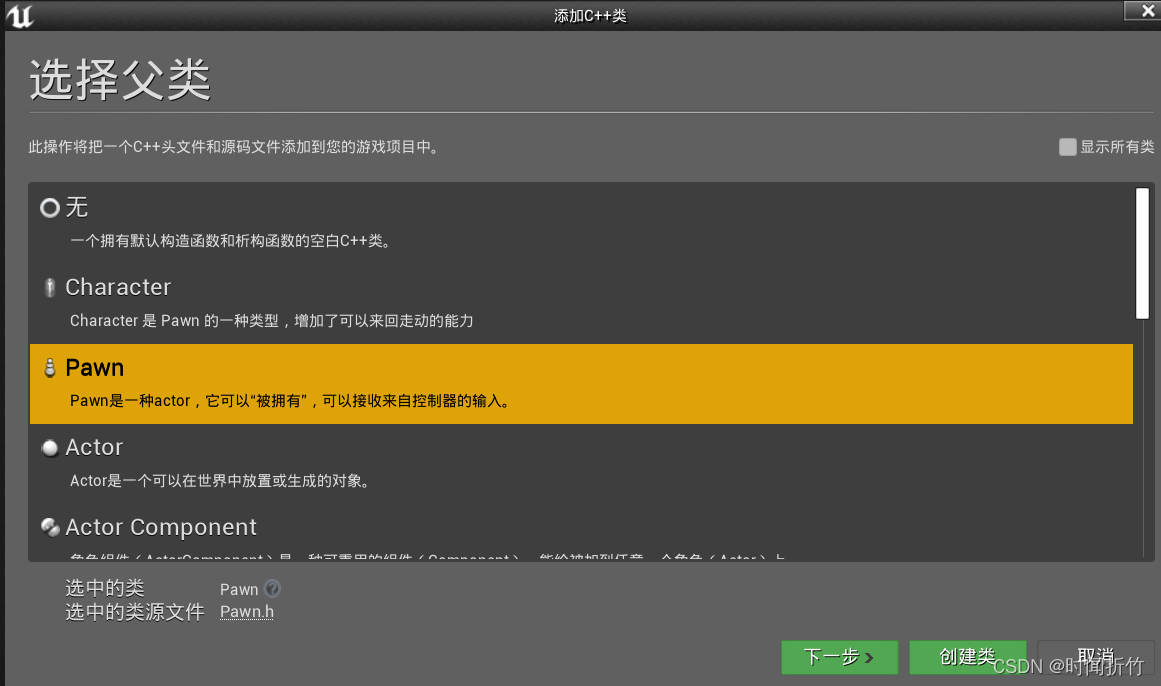
.h文件
配置变量属性
UPROPERTY(EditAnywhere)
UStaticMeshComponent* OurVisibleComponent;
.cpp文件
实例化各类对象
AMyPawn::AMyPawn()
{
PrimaryActorTick.bCanEverTick = true;
AutoPossessPlayer = EAutoReceiveInput::Player0;
RootComponent = CreateDefaultSubobject<USceneComponent>(TEXT("One"));
UCameraComponent* OurCamera = CreateDefaultSubobject<UCameraComponent>(TEXT("Two"));
OurVisibleComponent = CreateDefaultSubobject<UStaticMeshComponent>(TEXT("Three"));
OurCamera->SetupAttachment(RootComponent);
OurCamera->SetRelativeLocation(FVector(-250.0f, 0.0f, 250.0f));
OurCamera->SetRelativeRotation(FRotator(-45.0f, 0.0f, 0.0f));
OurVisibleComponent->SetupAttachment(RootComponent);
}
配置用户输入
设置输入表
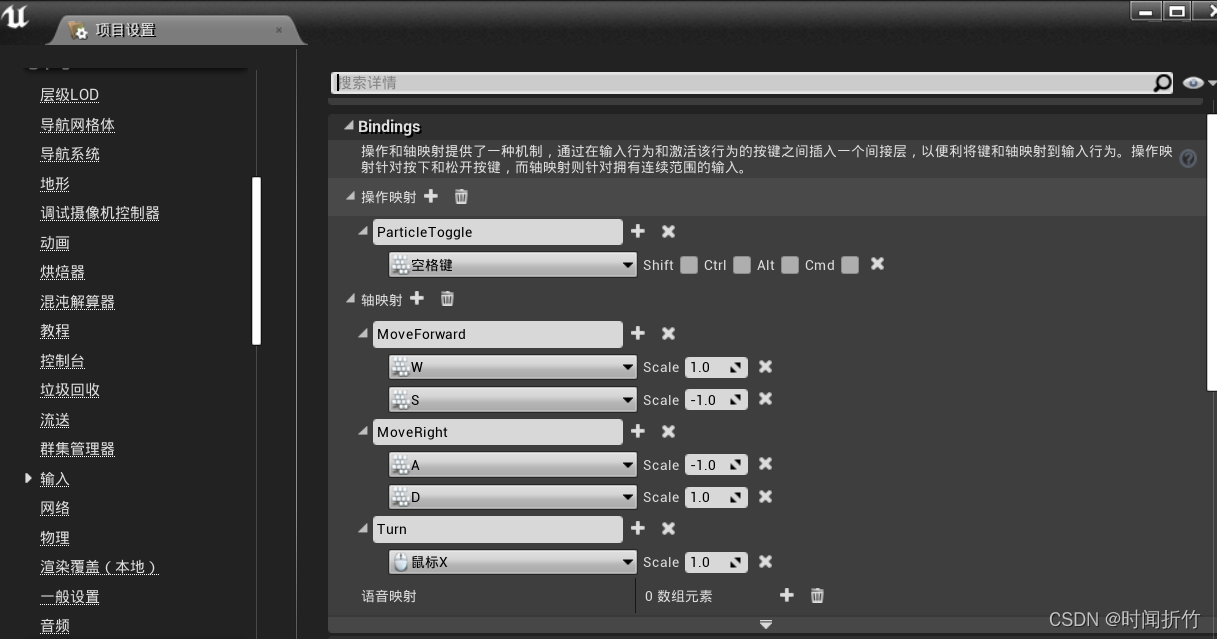
.h文件
定义输入函数和相关变量
void MoveX(float Value);
void MoveY(float Value);
void StartG();
void StopG();
FVector CurrentVelocity;
bool bGrowing;
.cpp文件
void AMyPawn::Tick(float DeltaTime)
{
Super::Tick(DeltaTime);
{
float CurrentScale = OurVisibleComponent->GetComponentScale().X;
if (bGrowing)
{
CurrentScale += DeltaTime;
}
else
{
CurrentScale -= (DeltaTime * 0.5f);
}
CurrentScale = FMath::Clamp(CurrentScale, 1.0f, 2.0f);
OurVisibleComponent->SetWorldScale3D(FVector(CurrentScale));
}
{
if (!CurrentVelocity.IsZero())
{
FVector NewLocation = GetActorLocation() + (CurrentVelocity * DeltaTime);
SetActorLocation(NewLocation);
}
}
}
将输入和Pawn进行绑定
#include "MyPawn.h"
#include "Camera/CameraComponent.h"
#include "Components/StaticMeshComponent.h"
#include "Components/InputComponent.h"
在回调函数中进行响应函数绑定
void AMyPawn::SetupPlayerInputComponent(UInputComponent* PlayerInputComponent)
{
Super::SetupPlayerInputComponent(PlayerInputComponent);
PlayerInputComponent->BindAction("ParticleToggle", IE_Pressed, this, &AMyPawn::StartG);
PlayerInputComponent->BindAction("ParticleToggle", IE_Released, this, &AMyPawn::StopG);
PlayerInputComponent->BindAxis("MoveForward", this, &AMyPawn::MoveX);
PlayerInputComponent->BindAxis("MoveRight", this, &AMyPawn::MoveY);
}
响应函数用来修改变量
void AMyPawn::MoveX(float Value)
{
CurrentVelocity.X = FMath::Clamp(Value, -1.0f, 1.0f) * 100.f;
}
void AMyPawn::MoveY(float Value)
{
CurrentVelocity.Y = FMath::Clamp(Value, -1.0f, 1.0f) * 100.f;
}
void AMyPawn::StartG()
{
bGrowing = true;
}
void AMyPawn::StopG()
{
bGrowing = false;
}
|