一、元素/组件过渡
transition标签
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Document</title>
<!--引入vue-->
<script src="https://cdn.jsdelivr.net/npm/vue/dist/vue.js"></script>
</head>
<body>
<div id="demo">
<button v-on:click="show = !show">
点我
</button>
<!--当插入或者删除包含在transition组件中的元素时,Vue会进行一系列处理-->
<!--1、自动嗅探目标元素是否应用了CSS过渡或动画
2、是否提供了钩子函数
3、如果都没有,将立即执行-->
<transition name="fade">
<p v-if="show">动画实例</p>
</transition>
</div>
<script>
new Vue({
el: '#demo',
data: {
show: true
}
})
</script>
</body>
</html>
二、使用过渡类实现动画
1、六个class
- v-enter:定义进入过渡的开始状态
- v-enter-active:定义进入过渡生效时的状态
- v-enter-to:定义进入过渡的结束状态
- v-leave:定义离开过渡的开始状态
- v-leave-active:定义离开过渡生效时的状态
- v-leave-to:定义离开过渡的的结束状态
2、CSS过渡
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Document</title>
<!--引入vue-->
<script src="https://cdn.jsdelivr.net/npm/vue/dist/vue.js"></script>
<style>
.v-enter{
opacity: 0;
}
.v-enter-active{
transition: opacity 3s;
}
.v-leave-to{
opacity: 0;
}
.v-leave-active{
transition: opacity 3s;
}
</style>
</head>
<body>
<div id="app">
<button @click="show=!show">点我</button>
<!--若transition存在name属性,则上面的v-enter等都要修改为相应的name,若wuname,则默认为v-->
<transition >
<!--在transition必须指定enter或者leave,不然不会变化-->
<div v-if="show">Hello world</div>
</transition>
<div v-if="show">Hello world</div>
</div>
<script>
new Vue({
el: '#app',
data: {
show: true
}
})
</script>
</body>
</html>
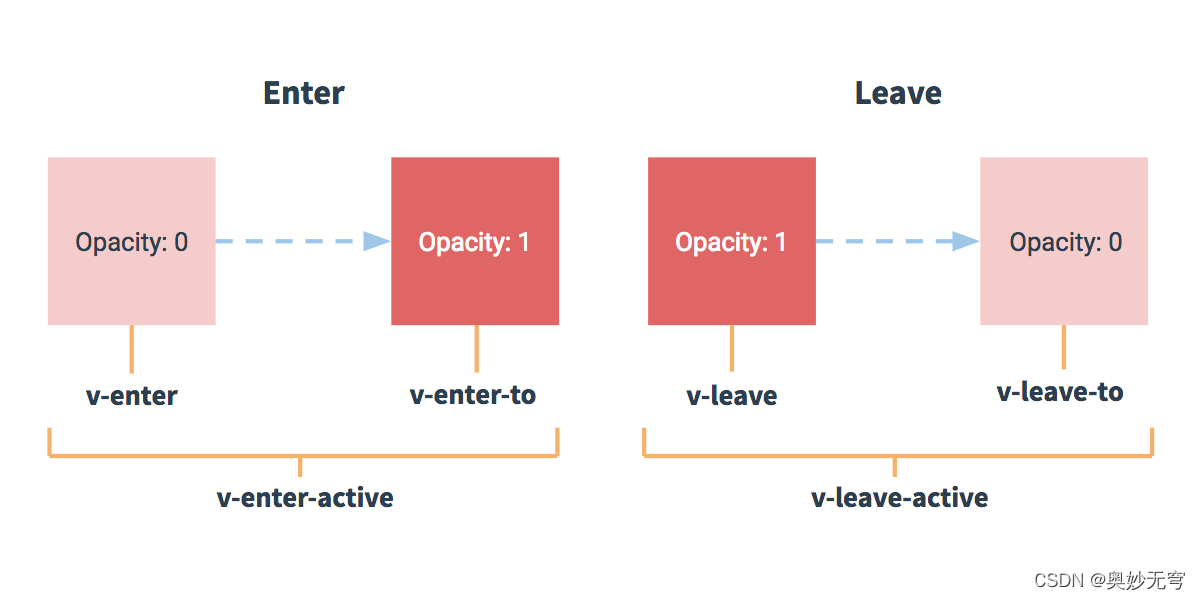
3、CSS动画
<!DOCTYPE html>
<html lang="en" xmlns:v-on="http://www.w3.org/1999/xhtml">
<head>
<meta charset="UTF-8">
<title>Document</title>
<!--引入vue-->
<script src="https://cdn.jsdelivr.net/npm/vue/dist/vue.js"></script>
<style>
.bounce-enter-active{
animation:bounce-in .5s;
}
.bounce-leave-active{
animation:bounce-in .5s reverse;
}
@keyframes bounce-in{
0%{transform:scale(0);}
50%{transform:scale(1.5);}
100%{transform:scale(1);}
}
</style>
</head>
<body>
<div id="app">
<button v-on:click="show = !show">点我</button>
<transition name="bounce">
<p v-if="show">Hello World</p>
</transition>
</div>
<script>
new Vue({
el: '#app',
data: {
show: true
}
})
</script>
</body>
</html>
动画和过渡同时使用
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Document</title>
<!--引入vue-->
<script src="https://cdn.jsdelivr.net/npm/vue/dist/vue.js"></script>
<style>
.fade-enter,.fade-leave-to{
opacity:0;
}
.fade-enter-active,.fade-leave-active{
transition:opacity 1s;
animation:bounce-in 5s;
}
@keyframes bounce-in{
0%{transform:scale(0);}
50%{transform:scale(1.5);}
100%{transform:scale(1);}
}
</style>
</head>
<body>
<div id="app">
<button v-on:click="show = !show">点我</button>
<transition name="fade" type="transition">
<p v-if="show">Hello World</p>
</transition>
</div>
<script>
new Vue({
el: '#app',
data: {
show: true,
},
})
</script>
</body>
</html>
4、自定义过渡的类名
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Document</title>
<!--引入vue-->
<script src="https://cdn.jsdelivr.net/npm/vue/dist/vue.js"></script>
<style>
.fade-in-active, .fade-out-active{
transition: all 1.5s ease
}
.fade-in-enter, .fade-out-active{
opacity: 0
}
</style>
</head>
<body>
<div id="app">
<button @click="show = !show">
点我
</button>
<transition
name="fade"
<!--这里自定义,分别填上对应的类名即可-->
enter-class="fade-in-enter"
enter-active-class="fade-in-active"
leave-class="fade-out-enter"
leave-active-class="fade-out-active"
>
<p v-show="show">hello</p>
</transition>
</div>
<script>
new Vue({
el: '#app',
data: {
show: true
}
})
</script>
</body>
</html>
5、CSS过渡钩子函数
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Document</title>
<!--引入vue-->
<script src="https://cdn.jsdelivr.net/npm/vue/dist/vue.js"></script>
<!--
Velocity 和 jQuery.animate 的工作方式类似,也是用来实现 JavaScript 动画的一个很棒的选择
-->
<script src="https://cdnjs.cloudflare.com/ajax/libs/velocity/1.2.3/velocity.min.js"></script>
</head>
<body>
<div id="app">
<button @click="show = !show">
点我
</button>
<transition
v-on:before-enter="beforeEnter"
v-on:enter="enter"
v-on:leave="leave"
v-bind:css="false"
>
<p v-if="show">
Hello
</p>
</transition>
</div>
<script>
new Vue({
el: '#app',
data: {
show: false
},
methods: {
beforeEnter: function (el) {
el.style.opacity = 0
el.style.transformOrigin = 'left'
},
enter: function (el, done) {
Velocity(el, { opacity: 1, fontSize: '1.4em' }, { duration: 300 })
Velocity(el, { fontSize: '1em' }, { complete: done })
},
leave: function (el, done) {
Velocity(el, { translateX: '15px', rotateZ: '50deg' }, { duration: 600 })
Velocity(el, { rotateZ: '100deg' }, { loop: 2 })
Velocity(el, {
rotateZ: '45deg',
translateY: '30px',
translateX: '30px',
opacity: 0
}, { complete: done })
}
}
})
</script>
</body>
</html>
|