缓冲
缓冲,顾名思义,就是暂时不急着用的内容。 OpenGL中,缓冲是指内存的线性分配。每个缓冲都有个缓冲名,同时还有分配给缓冲的内存,叫做缓冲的数据库。 若得到一个缓冲的名称,则可以通过将它绑定到缓存绑定点并附加到OpenGL环境中。绑定点又叫做靶点(target)
创建缓冲和分配内存
在OpenGL中缓冲的创建和vao是一样的流程 glCreateBuffer(GLsizei n, GLuint* buffers) glBindBuffer(GLenum target, GLuint buffer) //绑定缓冲到OpenGL中 分配内存 glBufferStorage(GLenum target, GLsizeiptr size, const void* data, GLbitfield flags) 这是给这类靶点分配内存空间的 glNamedBufferStorage(GLuint buffer, GLsizeiptr size, const void* data, GLbitfield flags) 这是给单独的缓冲分配空间的
将数据传入缓冲中去
glBufferSubData(GLenum targer, GLintptr offset, GLsizeptr size, const GLvoid* data); glNamedBufferSubData(GLuint buffer, GLintptr offset, GLsizeptr size, const GLvoid* data); 还有一种就是映射缓冲的数据库 glMapNamedBuffer(GLuint buffer, GLenum usage) 这是生成一个指针指向缓冲的内存 还有一种就是限定映射内存的位置 glMapNamedBufferRange(GLuint buffer, GLintptr offset, GLsizeptr length, GLbitfield access)
使用缓冲为顶点着色器提供数据
自己封装的Shader类(修改)
上一版的Shader类有点小问题,所以这次做了修改,主要是在链接之前检查了shader对象
Shader.h
#pragma once
#include "sb7.h"
#include <string>
#include <fstream>
#include <sstream>
#include <iostream>
#include <exception>
class Shader
{
public:
Shader();
Shader(const std::string vertexPath, const std::string fragmentPath);
Shader(const std::string vertexPath, const std::string tescPath, const std::string tesePath, const std::string fragmentPath);
void setVertexShder(const std::string vertexPath);
void setTescShader(const std::string tescPath);
void setTeseShader(const std::string tesePath);
void setFragmentShader(const std::string fragmentPath);
void use();
private:
GLuint program;
std::string shaderString;
const char* shaderSource;
std::ifstream shaderFile;
std::stringstream shaderSStream;
private:
void checkCompileErrors(unsigned int ID, std::string type);
void readShaderSource(std::string shaderFilePath);
void linkShader(GLuint shaderObject, std::string type);
};
Shader.cpp
#include "Shader.h"
Shader::Shader() {}
Shader::Shader(const std::string vertexPath, const std::string fragmentPath)
{
program = glCreateProgram();
setVertexShder(vertexPath);
setFragmentShader(fragmentPath);
}
Shader::Shader(const std::string vertexPath, const std::string tescPath, const std::string tesePath, const std::string fragmentPath)
{
program = glCreateProgram();
setVertexShder(vertexPath);
setFragmentShader(fragmentPath);
setTescShader(tescPath);
setTeseShader(tesePath);
}
void Shader::setVertexShder(const std::string vertexPath)
{
readShaderSource(vertexPath);
GLuint vertexShader;
vertexShader = glCreateShader(GL_VERTEX_SHADER);
linkShader(vertexShader, "VERTEX");
}
void Shader::setTescShader(const std::string tescPath)
{
readShaderSource(tescPath);
GLuint tescShader;
tescShader = glCreateShader(GL_TESS_CONTROL_SHADER);
linkShader(tescShader, "TESC");
}
void Shader::setTeseShader(const std::string tesePath)
{
readShaderSource(tesePath);
GLuint teseShader;
teseShader = glCreateShader(GL_TESS_EVALUATION_SHADER);
linkShader(teseShader, "TESE");
}
void Shader::setFragmentShader(const std::string fragmentPath)
{
readShaderSource(fragmentPath);
GLuint fragmentShader;
fragmentShader = glCreateShader(GL_FRAGMENT_SHADER);
linkShader(fragmentShader, "FRAGMENT");
}
void Shader::use()
{
glUseProgram(program);
}
void Shader::readShaderSource(std::string shaderFilePath)
{
shaderSStream.str("");
memset(&shaderSource, '\0', sizeof(shaderSource));
if (!shaderFilePath.empty())
{
shaderFile.open(shaderFilePath);
shaderFile.exceptions(std::ifstream::badbit || std::ifstream::failbit);
try
{
if (!shaderFile.is_open())
{
throw std::exception("open fragment shader file fail");
}
shaderSStream << shaderFile.rdbuf();
shaderFile.close();
shaderString = shaderSStream.str();
shaderSource = shaderString.c_str();
}
catch (const std::exception& e)
{
std::cout << e.what() << std::endl;
}
}
}
void Shader::linkShader(GLuint shaderObject, std::string type)
{
glShaderSource(shaderObject, 1, &shaderSource, NULL);
glCompileShader(shaderObject);
checkCompileErrors(shaderObject, type);
glAttachShader(program, shaderObject);
glLinkProgram(program);
checkCompileErrors(program, "PROGRAM");
glDeleteShader(shaderObject);
}
void Shader::checkCompileErrors(unsigned int ID, std::string type)
{
int success;
char infoLog[512];
if (type != "PROGRAM")
{
glGetShaderiv(ID, GL_COMPILE_STATUS, &success);
if (!success)
{
glGetShaderInfoLog(ID, 512, NULL, infoLog);
std::cout << "shader compile error: " << infoLog << std::endl;
}
}
else
{
glGetProgramiv(ID, GL_LINK_STATUS, &success);
if (!success)
{
glGetProgramInfoLog(ID, 512, NULL, infoLog);
std::cout << "program linking error: " << infoLog << std::endl;
}
}
}
shader
vertex shader
#version 450 core
layout(location = 0) in vec3 aPos;
layout(location = 1) in vec4 aColor;
out vec4 vs_color;
void main()
{
vs_color = aColor;
gl_Position = vec4(aPos, 1.0);
}
fragment shader
#version 450 core
out vec4 color;
in vec4 vs_color;
void main()
{
color = vs_color;
}
代码
#include "sb7.h"
#include "Shader.h"
#include <iostream>
class my_application : public sb7::application
{
public:
void startup() {
shader = new Shader("vs.vert", "fs.frag");
glCreateVertexArrays(1, &vertex_arrays_object);
glBindVertexArray(vertex_arrays_object);
glCreateBuffers(2, buffer);
glNamedBufferStorage(buffer[0], sizeof(vertices), NULL, GL_DYNAMIC_STORAGE_BIT);
glVertexArrayVertexBuffer(vertex_arrays_object, 0, buffer[0], 0, sizeof(float) * 3);
glVertexArrayAttribFormat(vertex_arrays_object, 0, 3, GL_FLOAT, GL_FALSE, 0);
glVertexArrayAttribBinding(vertex_arrays_object, 0, 0);
glEnableVertexArrayAttrib(vertex_arrays_object, 0);
glNamedBufferStorage(buffer[1], sizeof(bindcolor), NULL, GL_DYNAMIC_STORAGE_BIT);
glVertexArrayVertexBuffer(vertex_arrays_object, 1, buffer[1], 0, sizeof(float) * 4);
glVertexArrayAttribFormat(vertex_arrays_object, 1, 4, GL_FLOAT, GL_FALSE, 0);
glVertexArrayAttribBinding(vertex_arrays_object, 1, 1);
glEnableVertexArrayAttrib(vertex_arrays_object, 1);
}
void render(double currentTime) {
GLfloat color[] = { 1.0, 1.0, 1.0, 1.0 };
glClearBufferfv(GL_COLOR, 0, color);
shader->use();
glNamedBufferSubData(buffer[0], 0, sizeof(vertices), vertices);
glNamedBufferSubData(buffer[1], 0, 4 * 4 * 1, bindcolor);
glDrawArrays(GL_TRIANGLES, 0, 3);
}
void shutdown(){
}
private:
Shader* shader;
GLuint vertex_arrays_object;
GLuint buffer[2];
GLfloat vertices[9] = {
0.5, 0.0, 0.5,
0.0, 0.5, 0.5,
-0.5, 0.0, 0.5
};
GLfloat bindcolor[4] = { 1.0, 1.0, 0.0, 1.0 };
};
DECLARE_MAIN(my_application);
效果
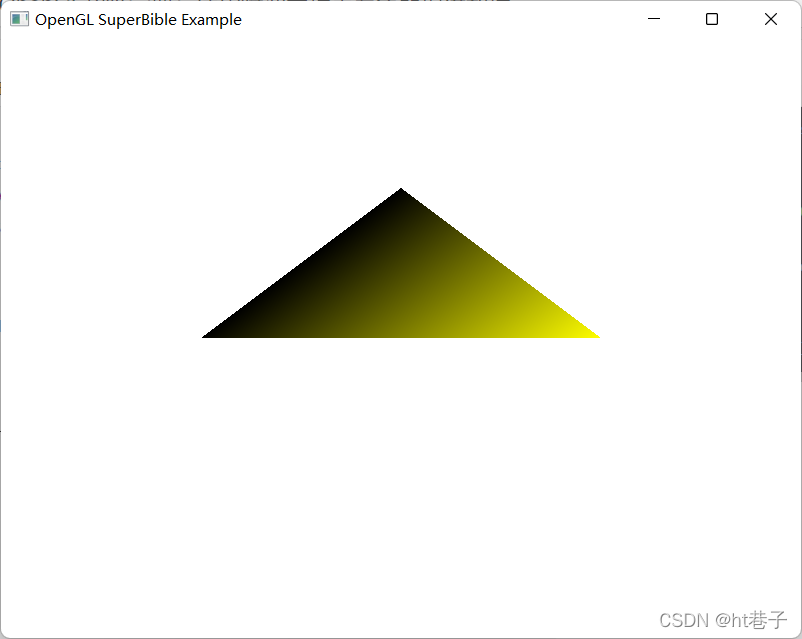
|