当使用TArray的AddUnique方法给结构体数组添加元素时,如果结构体没有重写==操作符时就会报错,那么为什么会报这个错呢?

因为AddUnique方法在添加元素前会将传入的元素在数组内搜索一遍,也就是Find()函数,在Find函数内部用到了==操作符,但是结构体是自定义的数据类型,所以必须重写==操作符才不会报错。
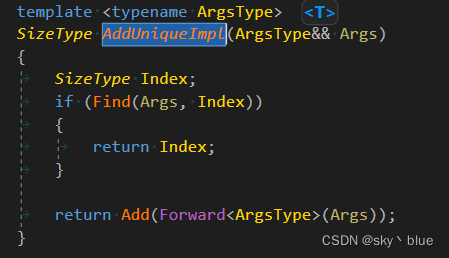
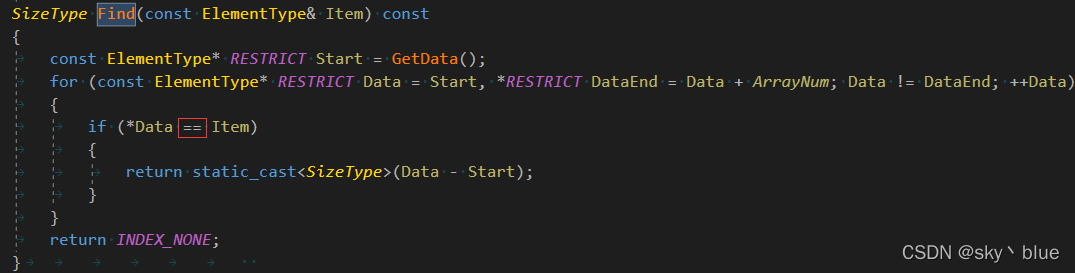
示例:
.h
// Fill out your copyright notice in the Description page of Project Settings.
#pragma once
#include "CoreMinimal.h"
#include "GameFramework/Actor.h"
#include "TestActors.generated.h"
UENUM(BlueprintType)
enum class EOrient : uint8
{
North,
East,
South,
West,
};
USTRUCT(BlueprintType)
struct FATestActor
{
GENERATED_USTRUCT_BODY()
public:
UPROPERTY(EditAnyWhere, BlueprintReadWrite)
FString str;
UPROPERTY(EditAnyWhere, BlueprintReadWrite)
EOrient orient;
UPROPERTY(EditAnyWhere, BlueprintReadWrite)
float a;
bool operator==(const FATestActor& ATestActor) const
{
return str == ATestActor.str && orient == ATestActor.orient && a == ATestActor.a;
}
};
UCLASS()
class TESTACTOR_API ATestActors : public AActor
{
GENERATED_BODY()
public:
// Sets default values for this actor's properties
ATestActors();
protected:
// Called when the game starts or when spawned
virtual void BeginPlay() override;
public:
// Called every frame
virtual void Tick(float DeltaTime) override;
public:
UPROPERTY(EditAnyWhere, BlueprintReadWrite)
TArray<FATestActor> testArrayStruct;
};
.cpp
// Fill out your copyright notice in the Description page of Project Settings.
#include "TestActors.h"
// Sets default values
ATestActors::ATestActors()
{
// Set this actor to call Tick() every frame. You can turn this off to improve performance if you don't need it.
PrimaryActorTick.bCanEverTick = true;
}
// Called when the game starts or when spawned
void ATestActors::BeginPlay()
{
//给自定义结构体的数组添加不重复内容
FATestActor testActor;
testActor.str = UTF8_TO_TCHAR("小明");
testActor.orient = EOrient::East;
testActor.a = 1.0f;
FATestActor testActor1;
testActor1.str = L"小红";
testActor1.orient = EOrient::East;
testActor1.a = 1.0f;
testArrayStruct.AddUnique(testActor);
testArrayStruct.AddUnique(testActor1);
Super::BeginPlay();
}
// Called every frame
void ATestActors::Tick(float DeltaTime)
{
Super::Tick(DeltaTime);
}
|