SoftWare Engineering – WEEK.2
2022.3.10 @Raoquan
WEEK.2 List
0. Lab 1
1. Requirements
- definition
- Theory and Techniques
2. Requirements in Agile Development
0. Lab 1
虽然我没有上课,但看老师的意思大概是根据银行的背景来开发一个程序,选择waterflow或者agile的方式。(但这个程序真的很小,所以我并没那么正经 但是我们需要有必要的javaDoc和测试
a. exe 1
直接把BankAccount类的代码copy下来就好。然后我们要根据需求,写几个方法,比如存钱,取钱,查看余额。 然后比较有意思的一点是,第二问说最大透支限额是500,那意思是第一题的透支额度可以无限大???反正我先当他是无限大的写了 然后我加了一个flag来判断有没有欠钱,从而让服务更加完整 记得加javaDoc,我这个doc也不是经常用,将就着看
public class BankAccount {
private int accNo;
private String accName;
private double balance;
boolean flagOverdraft = false;
public BankAccount(int accNo,String accName){
this.accNo=accNo;
this.accName=accName;
this.balance=0.0;
}
public BankAccount(String accName,int accNo){
this.accNo=accNo;
this.accName=accName;
this.balance=0.0;
}
public int getAccNo() {
return accNo;
}
public void setAccNo(int accNo) {
this.accNo = accNo;
}
public String getAccName() {
return accName;
}
public void setAccName(String accName) {
this.accName = accName;
}
public double getBalance() {
return balance;
}
public void setBalance(double balance) {
this.balance = balance;
}
public String toString() {
return "BankAccount{" +
"accNo=" + accNo +
", accName='" + accName + '\'' +
", balance=" + balance +
'}';
}
public void deposit(double addBalance){
if(flagOverdraft){
System.out.println("The account "+this.getAccName()+" will add balance: "+addBalance);
if(addBalance>=Math.abs(this.getBalance())){
this.setBalance(this.getBalance()+addBalance);
this.flagOverdraft=false;
System.out.println("Your bill has been paid off, Remaining balance: "+this.getBalance());
return;
}
else{
this.setBalance(this.getBalance()+addBalance);
System.out.println("You still owe money: "+Math.abs(this.getBalance()));
return;
}
}
else{
System.out.println("The account "+this.getAccName()+" will add balance: "+addBalance);
this.setBalance(this.getBalance()+addBalance);
return;
}
}
public void withdraw(double withdrawBalance){
if(flagOverdraft){
System.out.println("The account "+this.getAccName()+" will reduce balance: "+withdrawBalance);
this.setBalance(this.getBalance()-withdrawBalance);
System.out.println("You have owen money: "+Math.abs(this.getBalance()));
return;
}
else{
if(withdrawBalance>=this.getBalance()){
this.setBalance(this.getBalance()-withdrawBalance);
this.flagOverdraft=true;
System.out.println("You have owen money: "+Math.abs(this.getBalance()));
return;
}
else{
this.setBalance(this.getBalance()-withdrawBalance);
System.out.println("Remaining balance: "+this.getBalance());
return;
}
}
}
public double checkBalance(int accNo){
if(flagOverdraft){
System.out.println("The account "+this.getAccName()+" has owen money: "+Math.abs(this.getBalance()));
return this.getBalance();
}
else{
System.out.println("The account "+this.getAccName()+" has balance: "+this.getBalance());
return this.getBalance();
}
}
}
b. exe 2
根据题意,我们将创建一个子类,并且要设置默认每日限额500,就是每天最多能用500.所以我们继承之后要重写取钱的方法。 我们只需要加一句判断就可以
public class CurrentAccount extends BankAccount{
public CurrentAccount(int accNo, String accName) {
super(accNo, accName);
}
public CurrentAccount(String accName,int accNo){
super(accName,accNo);
}
private double overdraft=500.0;
public void withdraw(double withdrawBalance){
if(flagOverdraft){
System.out.println("The account "+this.getAccName()+" will reduce balance: "+withdrawBalance);
this.setBalance(this.getBalance()-withdrawBalance);
System.out.println("You have owen money: "+Math.abs(this.getBalance()));
return;
}
else{
if(withdrawBalance>=this.getBalance()){
if(withdrawBalance-this.getBalance()>this.overdraft){
System.out.println("You cannot exceed your quota");
return;
}
this.setBalance(this.getBalance()-withdrawBalance);
this.flagOverdraft=true;
System.out.println("You have owen money: "+Math.abs(this.getBalance()));
return;
}
else{
this.setBalance(this.getBalance()-withdrawBalance);
System.out.println("Remaining balance: "+this.getBalance());
return;
}
}
}
}
c. exe 3
我们先分析一下题意,bank里面肯定会有所有的账户,而账户的类型有两种,还可以对这些账户进行操作。 那么首先我们需要有一个东西来存所有的账户,然后要有很多方法来对这些账户进行操作 由于继承关系,我们需要利用多态来调用不同账户对应自己的方法(题中只有取钱有所区别,参照上一题)。所以我们的Arraylist要采用泛型
public class Bank {
ArrayList<BankAccount> accountList = new ArrayList<>();
public void openNew(int accNo,int type){
for(int i=0;i<accountList.size();i++){
if(accountList.get(i).getAccNo()==accNo){
System.out.println("Repeat account");
return;
}
else continue;
}
if(type==1){
accountList.add(new BankAccount(accNo,"account"+accNo));
}
else if(type==2){
accountList.add(new CurrentAccount(accNo,"account"+accNo));
}
}
public void closeAccount(int accNo){
for(int i=0;i<accountList.size();i++){
if(accountList.get(i).getAccNo()==accNo){
accountList.remove(i);
return;
}
else continue;
}
System.out.println("Invalid account");
}
public void deposit(int accNo,double addBalance){
for(int i=0;i<accountList.size();i++){
if(accountList.get(i).getAccNo()==accNo){
accountList.get(i).deposit(addBalance);
return;
}
else continue;
}
System.out.println("Invalid account");
}
public void withdraw(int accNo,double withdrawBalance){
for(int i=0;i<accountList.size();i++){
if(accountList.get(i).getAccNo()==accNo){
accountList.get(i).withdraw(withdrawBalance);
return;
}
else continue;
}
System.out.println("Invalid account");
}
public double checkBalance(int accNo){
for(int i=0;i<accountList.size();i++){
if(accountList.get(i).getAccNo()==accNo){
return accountList.get(i).getBalance();
}
else continue;
}
System.out.println("Invalid account");
return 0;
}
public String toString() {
String infor="";
for(int i=0;i<accountList.size();i++){
infor=infor+accountList.get(i).toString()+"\n";
}
return infor;
}
}
最后我们要写测试类来对上面三个题进行测试,我实在不想写doc了,整个lab的代码就这样。我考虑的条件多了些所以比较长
public class Test {
@org.junit.Test
public void BankTest(){
BankAccount t=new BankAccount(1,"account1");
t.deposit(100);
t.checkBalance();
System.out.println("\n");
t.withdraw(200);
t.checkBalance();
System.out.println("\n");
t.deposit(200);
t.checkBalance();
System.out.println("\n");
System.out.println(t.toString());
}
@org.junit.Test
public void currentTest(){
CurrentAccount t=new CurrentAccount(2,"account2");
t.deposit(100);
t.checkBalance();
System.out.println("\n");
t.withdraw(800);
t.checkBalance();
System.out.println("\n");
t.deposit(200);
t.checkBalance();
System.out.println("\n");
System.out.println(t.toString());
}
@org.junit.Test
public void bank(){
Bank b=new Bank();
b.openNew(1,1);
b.openNew(2,1);
b.openNew(3,2);
b.openNew(4,2);
System.out.println(b.toString());
System.out.println("\n");
b.closeAccount(1);
b.closeAccount(5);
System.out.println(b.toString());
System.out.println("\n");
b.deposit(1,200);
b.deposit(2,400);
b.deposit(3,500);
b.deposit(4,100);
System.out.println(b.toString());
System.out.println("\n");
b.withdraw(3,1100);
b.withdraw(3,200);
System.out.println(b.toString());
System.out.println("\n");
System.out.println(b.checkBalance(1));
System.out.println(b.checkBalance(2));
System.out.println(b.checkBalance(3));
System.out.println(b.checkBalance(3));
System.out.println("\n");
}
}
顺便提一下测试类中的 @org.junit.Test 这个东西的作用,当你在测试类的方法上面写上这句代码,我的idea就变成了图中这样。在方法的旁边出现一个绿色的东西,点击就可以run方法体中的代码。 可以理解为加上注解@Test后,不需要写main方法直接运行该方法,这样会省去很多繁琐 但是@Test是在junit这个jar包中,需要先导入才可以使用,如果本地环境没有的话还需要下载。 但idea很方便,你直接写上@Test,然后会报红,根据提示下载导入就可以用了 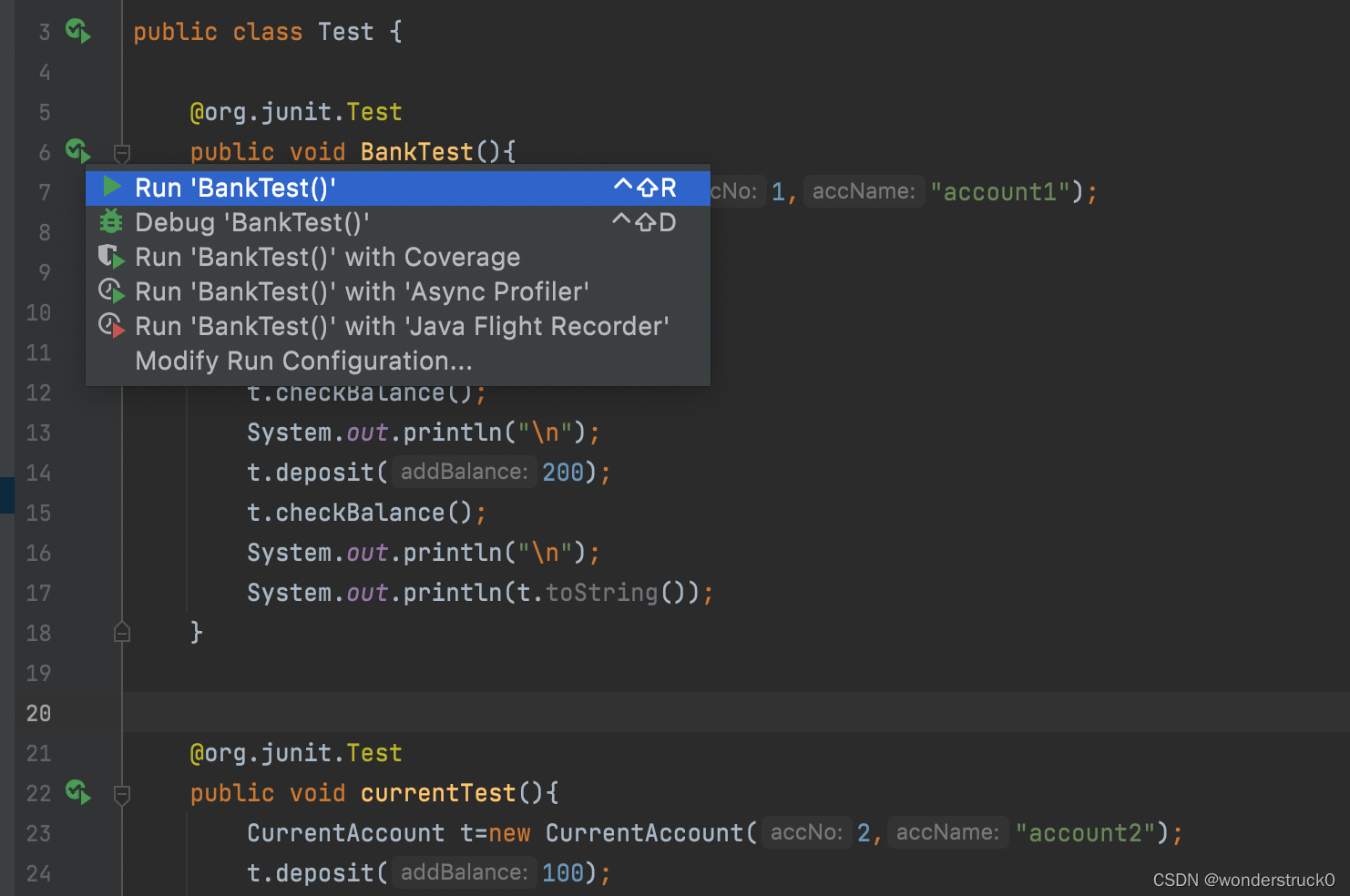
1. Requirements
对于这个ppt,咋说呢,感觉怪乱的,而且都是大白话,现在好像也没啥太值得记忆。准备放到期末再背吧。我就大致列个图先: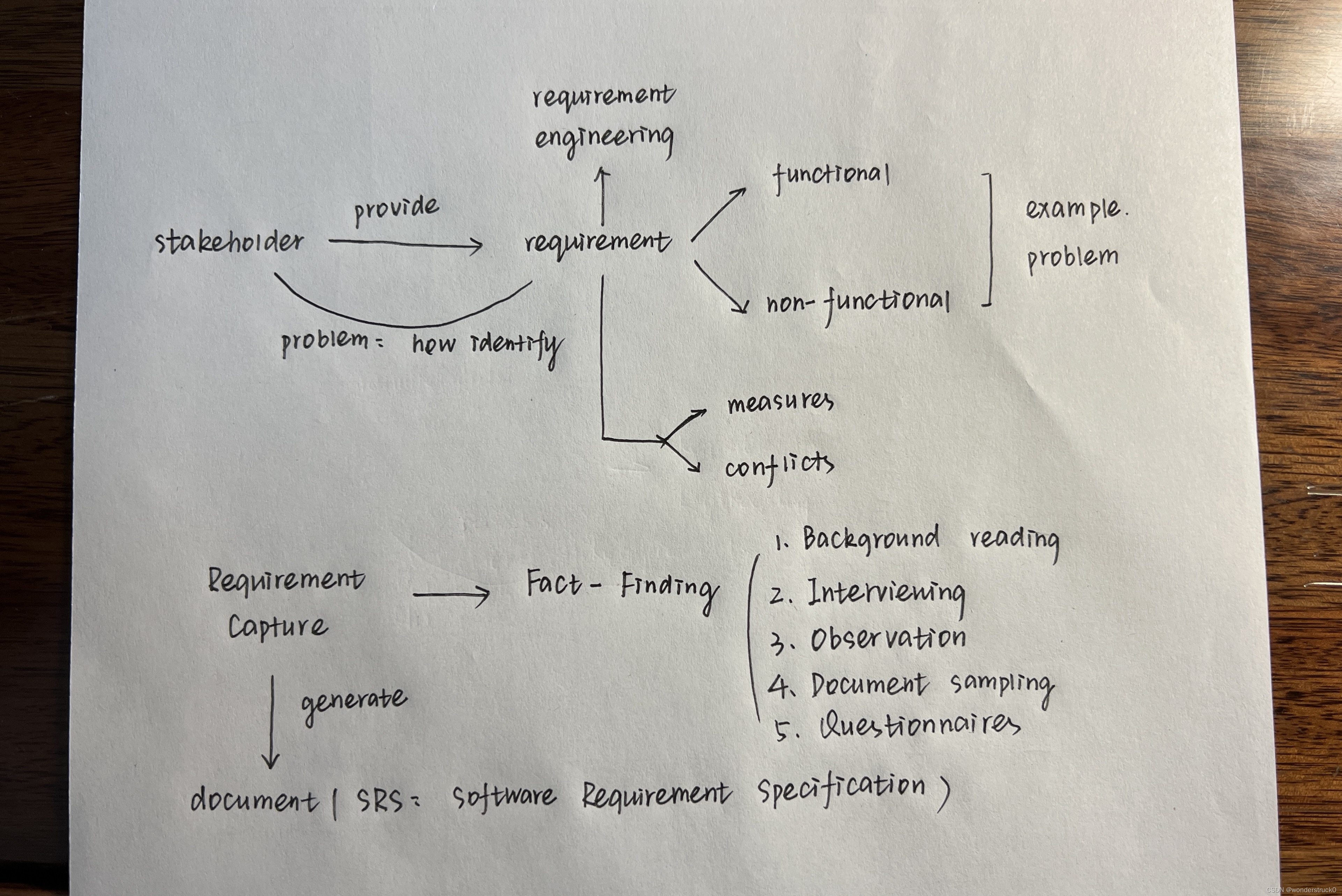
2. Requirements in Agile Development
这里就开始详细讲解敏捷开发中的步骤,这章是关于刚开始的需求分析。也就是之前提过的user stories及它衍生而来的各种东西。然后对于这些story来说,我们要学会写story card
其实我觉得ppt也比较清楚,例子也很多。所以我又画了图,放到期末再背,感觉这些大白话理解一下还是能扯出来。 主要是需要看看哪些例子,明白story怎么写;story是怎么拆分的;story的acceptance criteria是怎么写的;整个项目的词汇表应该收录哪些词汇,以及词汇表的格式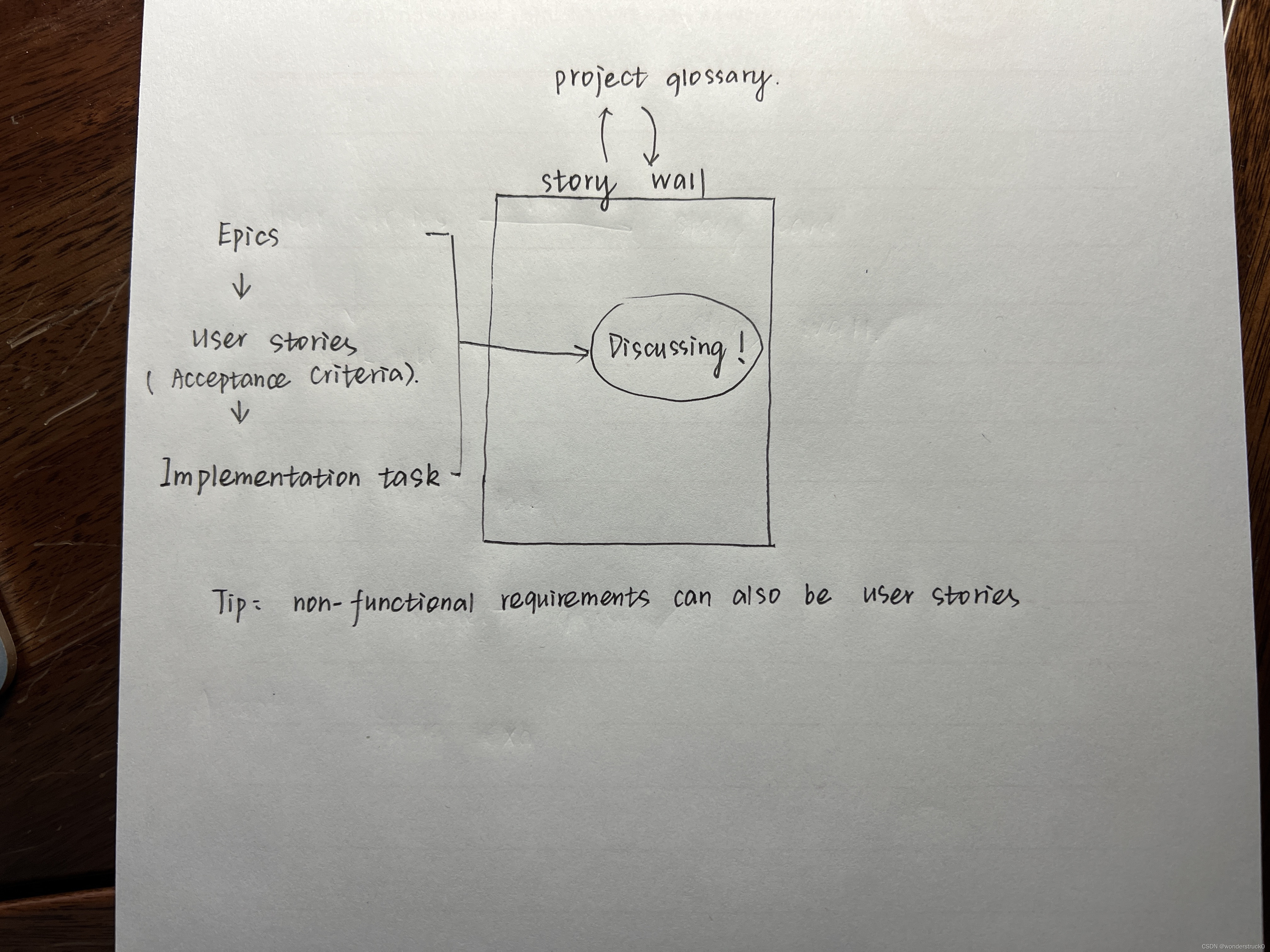
|