设计模式
🌈简单工厂模式
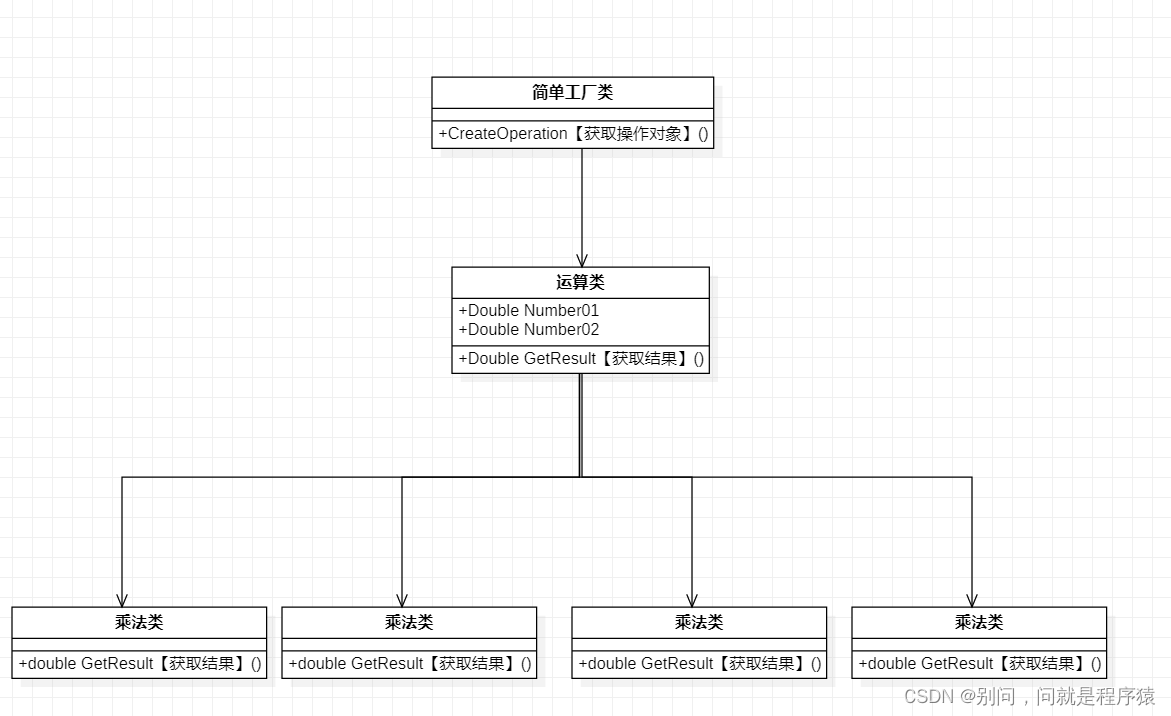
using System.Collections;
using UnityEngine;
using System;
public class UnityAssetsFactory
{
public static UnityAsset CreateUnityAssets(string assetType)
{
switch (assetType)
{
case "GameObject":
return new PrefabAssets("");
case "Sprite":
return new SpriteAsset("");
default:
return null;
}
}
}
public abstract class UnityAsset
{
public string path;
protected object assets;
public UnityAsset(string path)
{
this.path = path;
}
public void Load()
{
assets = Resources.Load(path);
}
public abstract void Instantiate();
public abstract void Show();
}
public class PrefabAssets : UnityAsset
{
public override void Instantiate()
{
GameObject go = GameObject.Instantiate(assets as GameObject);
}
public PrefabAssets(string path) : base(path) { }
public override void Show()
{
}
}
🌈策略模式
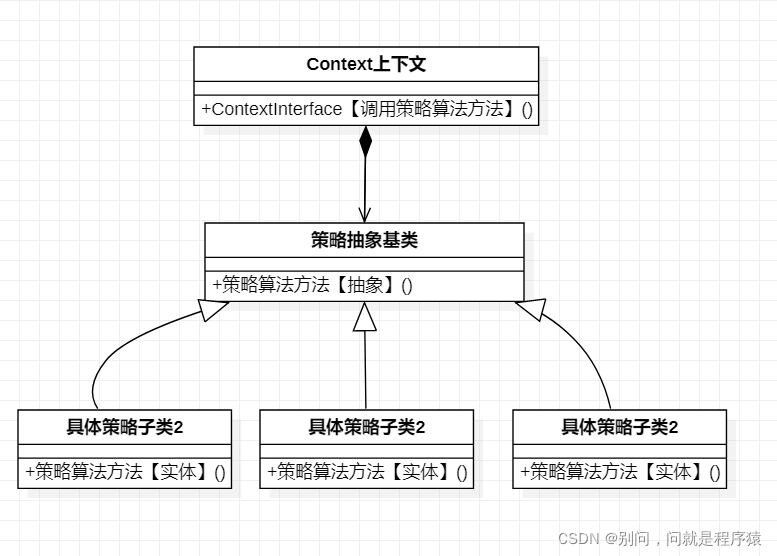
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public abstract class Skill
{
public GameHero[] AttackTargets { set; get; }
public GameHero SkillFireHero { set; get; }
public float[] skillParameters { get; set; }
public float Damage{ get; set; }
public abstract void TakeDamage();
}
/// <summary>
/// 圆形攻击技能
/// </summary>
public class CircleSkill : Skill
{
public override void TakeDamage()
{
// 拿到玩家坐标
Vector3 playerPos = SkillFireHero.heroTra.position;
// 计算技能中心 --> playerPos + forward * length
Vector3 skillCenter = playerPos + SkillFireHero.heroTra.forward * skillParameters[0];
for (int i = 0; i < AttackTargets.Length; i++)
{
// 计算该目标与技能中心的距离
float dis = Vector3.Distance(skillCenter, AttackTargets[i].heroTra.position);
// 如果距离小于技能半径
if (dis <= skillParameters[1])
{
AttackTargets[i].TakeDamage(Damage);
}
}
}
}
public class GameHero
{
private string name;
public float HeroHP{ set; get; }
public Transform heroTra { set; get; }
public Skill Skill{ set; get; }
public GameHero(string name)
{
this.name = name;
}
public void FireSkill()
{
Skill.SkillFireHero = this;
Skill.TakeDamage();
}
public void TakeDamage(float damage)
{
HeroHP -= damage;
Debug.Log(name + "受到伤害" + damage);
}
}
|