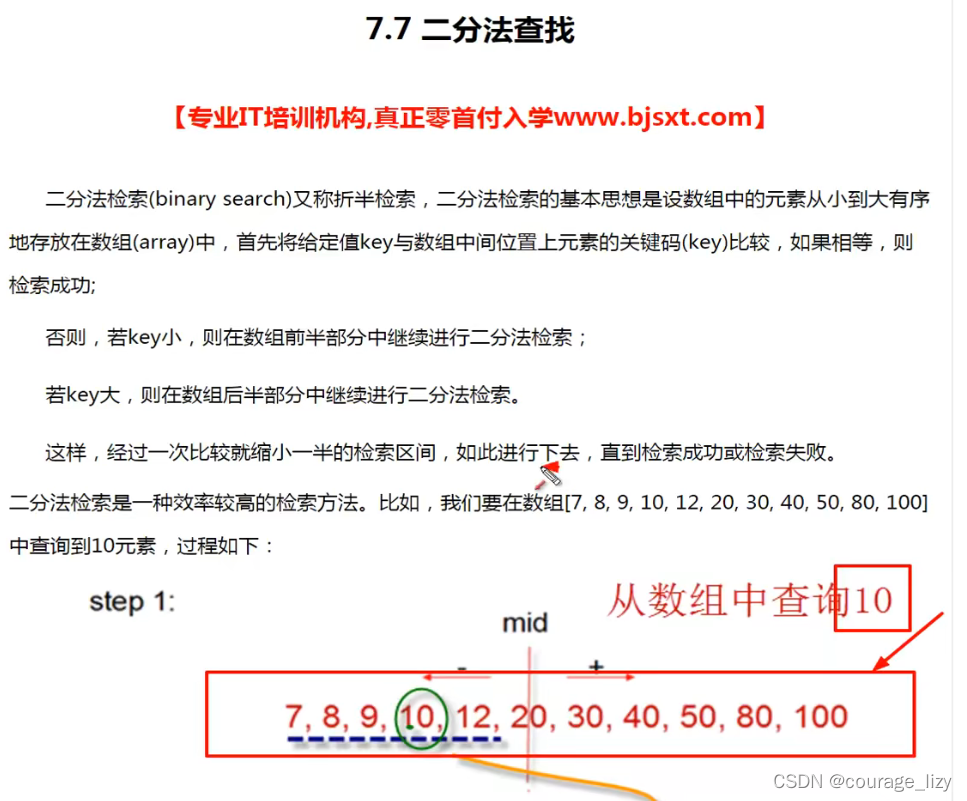
package JA;
import java.util.Arrays;
//测试二分法查找
public class TestBinarySearch {
public static void main(String[] args) {
int[] arr = {30, 20, 50, 10, 80, 9, 7, 12, 100, 40, 8};
Arrays.sort(arr);
System.out.println(Arrays.toString(arr));
System.out.println(myBinarySearch(10,arr));
}
public static int myBinarySearch(int value, int[] arr) {
int low = 0;
int hight = arr.length - 1;
int mid;
while (low < hight) {
mid = (low + hight) / 2;
if (arr[mid] == value) {
return mid;
}
if (arr[mid] > value) {
hight = mid - 1;
}
if (arr[mid] < value) {
low = mid + 1;
}
}
return -1;
}
}
?包装类
package cn.sxt.test;
//提供了基本数据类型,包装类和字符串之间的转化
//Integer包装类的使用
public class TestWrappedClass {
public static void main(String[] args) {
//Integer a=new Integer(3);
Integer b=Integer.valueOf(30);
//把包装类对象转成基本数据类型
int c=b.intValue();
double d=b.doubleValue();
//把字符串转成包装类对象
//Integer e=new Integer(("9999"));
Integer f=Integer.parseInt("999888");
//把包装类对象转成字符串
String str=f.toString();
//常见的常量
System.out.println("int类最大的整数: "+Integer.MAX_VALUE);
}
}
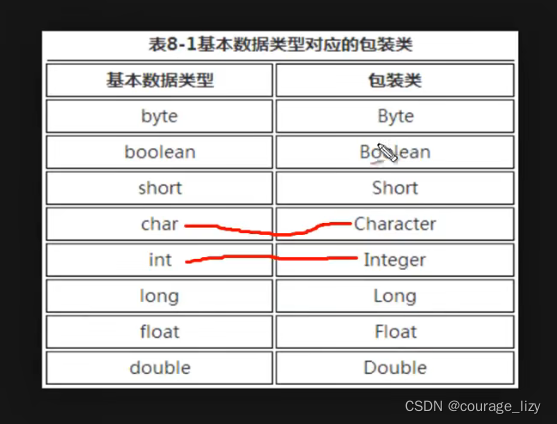
?包装类自动装箱和拆箱
package cn.sxt.test;
//测试自动装箱 自动拆箱
public class TestAutoBox {
public static void main(String[] args) {
Integer a=100;//自动装箱 相当于 Integer a=Integer.valueOf(234);
int b=a;//自动拆箱 编译器会修改为int b=a.intValue();
Integer c=null;
if(c!=null) {
int d = c;//自动拆箱 调用了c.intValue()
}
//缓存[-128,127]之间的数字
Integer in1=Integer.valueOf(-128);
Integer in2=-128;
System.out.println(in1==in2);
System.out.println(in1.equals(in2));
System.out.println("############");
Integer in3=1234;
Integer in4=1234;
System.out.println(in3==in4);
System.out.println(in3.equals(in4));
}
}
String源码分析不可变与可变序列
package cn.sxt.test;
public class TestString {
public static void main(String[] args) {
/*String str="aaabbb";//赋值后不可变
String str2=str.substring(2,5);
System.out.println(str);
System.out.println(str2);*/
String str1="hello"+" java";//编译器做了优化 直接在编译的时候将字符串进行拼接
String str2="hello java";
System.out.println(str1==str2);//true
//==比较对象 equals比较内容
String str3="hello";
String str4=" java";
String str5=str3+str4;//编译的时候不知道变量中存储的是什么 所以没办法在编译的时候优化
System.out.println(str2==str5);//false
System.out.println(str2.equals(str5));
}
}
package cn.sxt.test;
public class TestStringBuilder {
public static void main(String[] args) {
String str;
//StringBuilder线程不安全 效率高(一般使用它);StringBuilder线程安全 效率低
StringBuilder sb=new StringBuilder("abcdefg");
System.out.println(Integer.toHexString(sb.hashCode()));//输出字符串的地址
System.out.println(sb);//输出字符串内容
sb.setCharAt(2,'M');
System.out.println(Integer.toHexString(sb.hashCode()));
System.out.println(sb);
}
}
package cn.sxt.test;
//测试可变序列的常见用法
public class TestStringBuilder2 {
public static void main(String[] args) {
StringBuilder sb=new StringBuilder();
for(int i=0;i<26;i++){
char temp=(char)('a'+i);
sb.append(temp);
}
System.out.println(sb);
sb.reverse();
System.out.println(sb);
sb.setCharAt(3,'高');
System.out.println(sb);
sb.insert(0,'我').insert(6,'爱');//链式调用 核心就是该方法调用了return this把自己返回了
System.out.println(sb);
sb.delete(20,23);
System.out.println(sb);
String str8="";
// for(int i=0;i<5000;i++){
// str8=str8+i;//相当于产生10000个对象
// }
//正确写法
StringBuilder sb1=new StringBuilder();
for (int i=1;i<5000;i++){
sb1.append(i);
}
}
}
|