1 lambda表达式
java8中引入了 -> 操作符,该操作符将表达式拆成了两部分:
- 左侧:lambda表达式参数列表
- 右侧:lambda需要执行的功能
语法格式一:无参数,无返回值
() -> System.out.println(“abc”);
语法格式二:一个参数,无返回值
(x) -> System.out.println(x); () 可以省略: x -> System.out.println(x);
语法格式三:两个以上参数,有返回值,且lambda中有多条语句
Comparator<Integer> comparator = (x, y) -> {
System.out.println(x + y);
return Integer.compare(x, y);
};
2 四大核心函数式接口
消费型接口
public interface Consumer<T> {
void accept(T t);
供给型接口
@FunctionalInterface
public interface Supplier<T> {
T get();
}
函数型接口
public interface Function<T, R> {
R apply(T t);
断言型接口
@FunctionalInterface
public interface Predicate<T> {
boolean test(T t);
3 Stream API
流(Stream)是数据渠道,用于操作数据源(集合、数组等)所产生的元素序列。 集合讲的是数据,流讲的是计算 注意
- Stream自己不会存储元素
- Stream不会改变源对象,会返回一个持有结果的新stream
- Stream操作是延迟执行的,会等到需要结果的时候才执行
Stream操作的三个步骤 
3.1 创建Stream
- 通过一个集合获取流
new ArrayList().stream(); - 通过Arrays的静态方法获取流
Arrays.stream(new ArrayList); - 通过Stream静态方法获取流
Stream stream = Stream.of(“aa”, “bb”, “cc”); - 创建无限流
Stream stream = Stream.iterate(0, (x) -> x + 2);
3.2 中间操作
一个中间操作链,对数据源的数据进行处理 多个中间操作可以连起来形成一个流水线,除非流水线上触发终止操作,否则中间操作不会执行任何处理!而是在终止操作的时候一次性全部处理,称为惰性求值
3.2.1 筛选与切片
- filter:接收lambda,从流中排除某些元素
- distinct:筛选,通过流所生成元素的hashCode和equals方法去重
- limit:截断流,使其元素不超过给定的数量
- skip:跳过元素,返回一个扔掉了前n个元素的流,若流中元素不足n个则返回一个空流,与limit(n)互补
3.2.2 映射
- map:接收lambda,将元素转换成其他形式或提取信息,接收一个函数作为参数,该函数会应用到每一个元素上,并将其映射称一个新元素
- flatmap:接收一个函数作为参数,将流中每一个值都换成另一个流,然后把所有的流连成一个流
3.2.3 排序
- sort()
- sorted(Comparator com)
3.3 终止操作(终端操作)
3.3.1 查找与匹配
一个终止操作,执行中间操作链,并产生结果
- allMatch:检查是否匹配所有元素
- anyMatch:检查是否匹配其中一个元素
- noneMatch:检查是否没有匹配所有元素
- findFirst:返回第一个元素
- findAny:返回任意一个元素
- count:返回元素总个数
- max:返回流中最大值
- min:返回流中最小值
3.3.2 规约与收集
- reduce:将集合中的元素反复结合起来得到一个值
System.out.println(Stream.of(1, 2, 3, 4, 5).reduce(0, Integer::sum));
- collect:将流转换为其他形式,接收一个Collector接口的实现,用于给stream中元素做汇总的方法
new ArrayList<>().stream().collect(Collectors.counting());
new ArrayList<>().stream().collect(Collectors.averagingInt());
new ArrayList<>().stream().collect(Collectors.summarizingInt());
new ArrayList<>().stream().collect(Collectors.maxBy());
new ArrayList<>().stream().collect(Collectors.minBy());
new ArrayList<>().stream().collect(Collectors.groupingBy());
3.4 并行流与顺序流
3.4.1 fork/join框架
fork/join框架:在必要的情况下,将一个大任务进行拆分成若干个小任务,再将一个个小任务运算结果进行join汇总 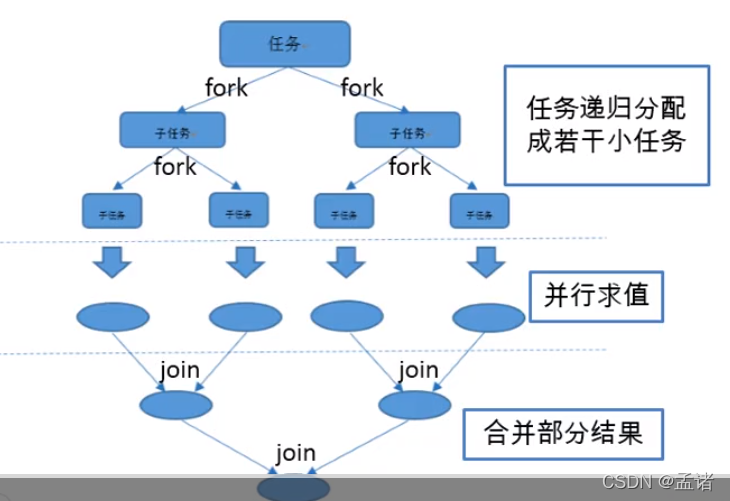 fork/join框架和普通线程池的区别 采用“工作窃取”模式,当执行新的任务时,它可以将其拆分成更小的任务执行,并将小任务加到线程队列中,所有被ForkJoinPool管理的线程尝试窃取提交到池子里的任务来执行,执行中又可产生子任务提交到池子中。 相对于一般的线程池实现,fork/join框架优势体现在对其中包含的任务的处理方式上,在一般的线程池中,如果一个线程执行的任务由于某种原因无法继续执行,那么该线程会处于等待状态,而在fork/join框架实现中,如果某个子问题由于等待另外一个子问题的完成而无法继续运行,那么处理该子问题的线程会主动寻找其他尚未运行的子问题来执行,这样的方式减少了线程等待时间,提高了性能
3.4.2 手写fork/join功能
@Test
public void test() {
ForkJoinPool pool = new ForkJoinPool();
Long result = pool.invoke(new ForkJoinCalculate(1L, 1000000L));
System.out.println(result);
}
public static class ForkJoinCalculate extends RecursiveTask<Long> {
private Long start;
private Long end;
private final int THRESHOLD = 10000;
public ForkJoinCalculate(Long start, Long end) {
this.start = start;
this.end = end;
}
@Override
protected Long compute() {
if (end - start < THRESHOLD) {
long sum = 0;
for (long i = start; i <= end; i ++) {
sum += i;
}
return sum;
} else {
long middle = (end + start) / 2;
ForkJoinCalculate left = new ForkJoinCalculate(start, middle);
left.fork();
ForkJoinCalculate right = new ForkJoinCalculate(middle + 1, end);
right.fork();
return left.join() + right.join();
}
}
}
3.4.3 并行流
LongStream.rangeClosed(0, 10000000L)
.parallel()
.reduce(0, Long::sum);
4 Optional类
Optional是一个容器类,更好地表示一个值存在或不存在,主要用于避免空指针异常
4.1 创建:
- Optional.empty(): 创建一个空的 Optional 实例
- Optional.of(T t):创建一个 Optional 实例,当 t为null时抛出异常
- Optional.ofNullable(T t):创建一个 Optional 实例,但当 t为null时不会抛出异常,而是返回一个空的实例
4.2 获取:
- get():获取optional实例中的对象,当optional 容器为空时报错
4.3 判断:
- isPresent():判断optional是否为空,如果空则返回false,否则返回true
- ifPresent(Consumer c):如果optional不为空,则将optional中的对象传给Comsumer函数
- orElse(T other):如果optional不为空,则返回optional中的对象;如果为null,则返回 other 这个默认值
- orElseGet(Supplier other):如果optional不为空,则返回optional中的对象;如果为null,则使用Supplier函数生成默认值other
- orElseThrow(Supplier exception):如果optional不为空,则返回optional中的对象;如果为null,则抛出Supplier函数生成的异常
4.4 过滤:
- filter(Predicate p):如果optional不为空,则执行断言函数p,如果p的结果为true,则返回原本的optional,否则返回空的optional
4.5 映射:
- map(Function<T, U> mapper):如果optional不为空,则将optional中的对象 t 映射成另外一个对象 u,并将 u 存放到一个新的optional容器中。
- flatMap(Function< T,Optional> mapper):跟上面一样,在optional不为空的情况下,将对象t映射成另外一个optional
区别:map会自动将u放到optional中,而flatMap则需要手动给u创建一个optional
5 时间与日期API
5.1 时间格式化
5.1.1 SimpleDateFormat线程不安全
测试代码:
private SimpleDateFormat simpleDateFormat = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");
ThreadPoolExecutor poolExecutor = new ThreadPoolExecutor(10, 100, 1, TimeUnit.MINUTES, new LinkedBlockingQueue<>(1000));
@Test
public void test() {
while (true) {
poolExecutor.execute(() -> {
String dateString = simpleDateFormat.format(new Date());
try {
Date parseDate = simpleDateFormat.parse(dateString);
String dateString2 = simpleDateFormat.format(parseDate);
System.out.println(dateString.equals(dateString2));
} catch (ParseException e) {
e.printStackTrace();
}
});
}
}
输出的值有false 原因:
- 多个线程之间共享变量calendar,并修改calendar。因此在多线程环境下,当多个线程同时使用相同的SimpleDateFormat对象(如static修饰)的话,如调用format方法时,多个线程会同时调用calender.setTime方法,导致time被别的线程修改,因此线程是不安全的。
- parse方法也是线程不安全的,parse方法实际调用的是CalenderBuilder的establish来进行解析,其方法中主要步骤不是原子操作。
5.1.2 DateTimeFormatter时间格式化
@Test
public void test() throws InterruptedException {
DateTimeFormatter dateTimeFormatter = DateTimeFormatter.ISO_DATE;
LocalDateTime localDateTime = LocalDateTime.now();
System.out.println(dateTimeFormatter.format(localDateTime));
DateTimeFormatter dateTimeFormatter2 = DateTimeFormatter.ofPattern("yyyyMMdd");
System.out.println(dateTimeFormatter2.format(localDateTime));
}
输出 2022-03-14 20220314
5.2 新时间API
- LocalDate : 只含年月日的日期对象
- LocalTime :只含时分秒的时间对象
- LocalDateTime : 同时含有年月日时分秒的日期对象
5.2.1 获取时间实例
@Test
public void test() {
LocalDateTime.now();
LocalDateTime.of(2018, 1, 13, 9, 43, 20)
}
5.2.2 时间运算
每次都会返回一个新的对象,旧对象不变
LocalDateTime ldt = LocalDateTime.of(2018, 1, 13, 9, 43, 20);
LocalDateTime ldt2 = ldt.plusYears(2);
LocalDateTime ldt3 = ldt.minusMonths(2);
System.out.println(ldt);
System.out.println(ldt2);
System.out.println(ldt3);
输出 2018-01-13T09:43:20 2020-01-13T09:43:20 2017-11-13T09:43:20
5.2.3 获取时间信息
System.out.println(ldt.getYear());
System.out.println(ldt.getMonth());
System.out.println(ldt.getDayOfMonth());
System.out.println(ldt.getHour());
System.out.println(ldt.getMinute());
System.out.println(ldt.getSecond());
5.2.4 Instant时间戳
以Unix元年:1970年1月1日到某个时间的毫秒值
@Test
public void test() {
Instant instant = Instant.now();
System.out.println(instant);
OffsetDateTime offsetDateTime = instant.atOffset(ZoneOffset.ofHours(8));
System.out.println(offsetDateTime);
System.out.println(instant.toEpochMilli());
Instant instant2 = Instant.ofEpochSecond(60);
System.out.println(instant2);
}
5.2.5 计算时间间隔
- Duration:计算两个时间之间的间隔
- Period:计算两个日期之间的间隔
public void test() throws InterruptedException {
Instant instant = Instant.now();
Thread.sleep(1000);
Instant instant2 = Instant.now();
Duration duration = Duration.between(instant, instant2);
System.out.println(duration.toMillis());
LocalTime localTime = LocalTime.now();
Thread.sleep(1000);
LocalTime localTime2 = LocalTime.now();
Duration duration2 = Duration.between(localTime, localTime2);
System.out.println(duration2.toMillis());
LocalDate localDate = LocalDate.now();
LocalDate localDate2 = LocalDate.of(2020, 10, 15);
Period period = Period.between(localDate2, localDate);
System.out.println(period.getYears() + " " + period.getMonths() + " " + period.getDays());
}
输出 1006 1000 1 4 27
|