📢欢迎点赞👍收藏?留言📝如有错误敬请指正!
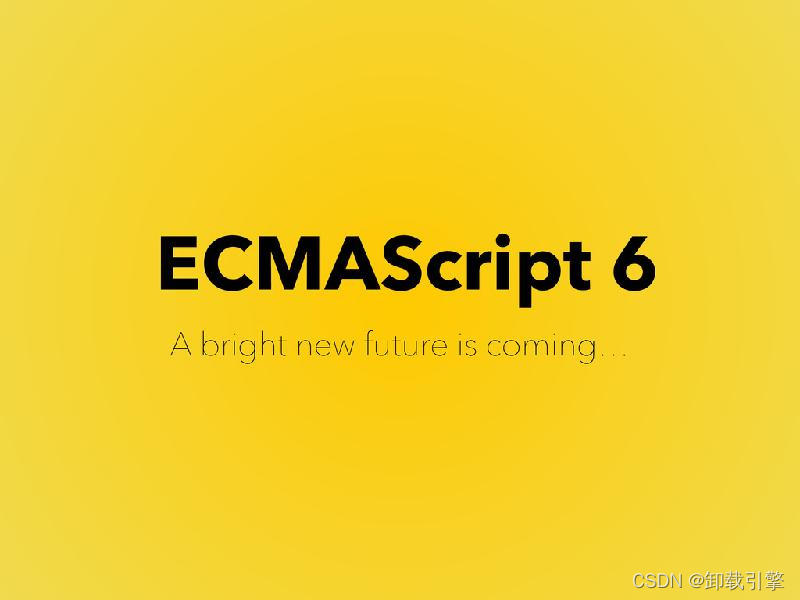
箭头函数
ES6 允许使用“箭头”=> 定义函数。
var f = () => 5;
var f = function () { return 5 };
var sum = (num1, num2) => num1 + num2;
var sum = function(num1, num2) {
return num1 + num2;
};
箭头函数的一个用处是简化回调函数:
[1,2,3].map(function (x) {
return x * x;
});
[1,2,3].map(x => x * x);
var result = values.sort(function (a, b) {
return a - b;
});
var result = values.sort((a, b) => a - b);
注意
-
箭头函数没有自己的this 对象(重要)。 -
不可以当作构造函数,也就是说,不可以对箭头函数使用new 命令,否则会抛出一个错误。 -
不可以使用arguments 对象,该对象在函数体内不存在。如果要用,可以用 rest 参数代替。 -
不可以使用yield 命令,因此箭头函数不能用作 Generator 函数。
上面四点中,最重要的是第一点。 对于普通函数来说,内部的this 指向函数运行时所在的对象,但是这一点对箭头函数不成立。 箭头函数没有自己的this 对象,内部的this 就是定义时上层作用域中的this 。 也就是说,箭头函数内部的this 指向是固定的,相比之下,普通函数的this指向是可变的。
总之,箭头函数根本没有自己的this ,导致内部的this 就是它所在的外层父级函数代码块的this 。正是因为它没有this ,所以也就不能用作构造函数。
下面是 Babel 转箭头函数产生的 ES5 代码,就能清楚地说明this的指向。
function foo() {
setTimeout(() => {
console.log('id:', this.id);
}, 100);
}
function foo() {
var _this = this;
setTimeout(function () {
console.log('id:', _this.id);
}, 100);
}
上面代码中,转换后的 ES5 版本清楚地说明了,箭头函数里面根本没有自己的this ,而是引用外层的this 。 javascript 函数通过function 关键词来定义,因为箭头函数没有function ,所以箭头函数没有形成自己的作用域,箭头函数可以看作为一种语法糖。
我的实际使用
在使用ant design 的穿梭框组件的时候,我需要做穿梭值的二次校验,[nzCanMove]="canMove" 使用普通函数的定义方法定义canMove() 函数会导致无法取到外层的this ,以至于无法使用弹窗提醒。换为箭头函数就可以完美解决问题。
<nz-transfer
[nzDataSource]="list"
[nzCanMove]="canMove"
(nzSelectChange)="select($event)"
(nzChange)="change($event)"
>
</nz-transfer>
canMove = (arg: TransferCanMove): Observable<TransferItem[]> => {
let items = arg.list;
if (arg.direction === 'right' && arg.list.length > 0) {
if (items[0].unitId) {
this.mdlSnackbarService.showToast('此人员被其他部门关联,如有疑问请咨询管理员!');
} else {
this.addStaff(items[0]);
items[0].staffTYpe = 0;
return of(arg.list);
}
} else if (arg.direction === 'left' && arg.list.length > 0){
return of(arg.list);
}
}
|