1.一个例子带你快速了解Java中方法的使用
public class TestDemo {
public static void main(String[] args) {
Scanner sc=new Scanner(System.in);
while(sc.hasNextInt()){
int year=sc.nextInt();
isLeapYear(year);
}
}
public static void isLeapYear(int year){
if((year%4==0&&year%100!=0)||year%400==0){
System.out.println("闰年");
}
else{
System.out.println("平年");
}
}
}
2.形参和实参的关系
在Java中,实参永远都是拷贝到形参中,形参和实参本质是两个实体
public static void swap(int x,int y){
int tmp=x;
x=y;
y=tmp;
System.out.println("x="+x+" , y="+y);
}
public static void main(String[] args) {
int a=10;
int b=20;
swap(a,b);
System.out.println("a="+a+" , b="+b);
}
可以看到, 对 x 和 y 的修改, 不影响 a 和 b 注意:对于基础类型来说, 形参相当于实参的拷贝. 即 传值调用
解决方法:传引用类型参数 (例如数组来解决这个问题)
public static void swap1(int []arr){
int tmp=arr[0];
arr[0]=arr[1];
arr[1]=tmp;
}
public static void main(String[] args) {
int [] arr;
arr=new int [2];
arr[0]=10;
arr[1]=20;
System.out.println("交换前: arr[0]="+arr[0]+" arr[1]= "+arr[1]);
swap1(arr);
System.out.println("交换后: arr[0]="+arr[0]+" arr[1]= "+arr[1]);
}
运行结果: 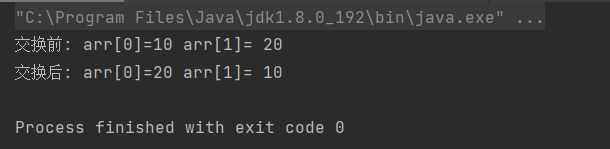
3.方法重载
重载规则:
- 方法名必须相同
- 参数列表必须不同(参数的个数不同、参数的类型不同、类型的次序必须不同)
- 与返回值类型是否相同无关
例:
public static int add(int x,int y){
return x+y;
}
public static double add(double x,double y){
return x+y;
}
public static double add(double x,double y,double z){
return x+y+z;
}
public static void main(String[] args) {
int a=10;
int b=20;
double c=20.0;
double d=30.0;
double e=40.0;
System.out.println(add(a,b));
System.out.println(add(c,d));
System.out.println(add(c,d,e));
}
编译器在编译代码时,会对实参类型进行推演,根据推演的结果来确定调用哪个方法
4.方法的递归:
4.1求斐波那契数列某一项的值

public static int fib(int n){
if(n==1||n==2){
return 1;
} else{
return fib(n-1)+fib(n-2);
}
}
public static void main(String[] args) {
int n=0;
Scanner sc=new Scanner(System.in);
while(sc.hasNextInt()){
n=sc.nextInt();
int ret=fib(n);
System.out.println(ret);
}
}
4.2青蛙跳台阶问题
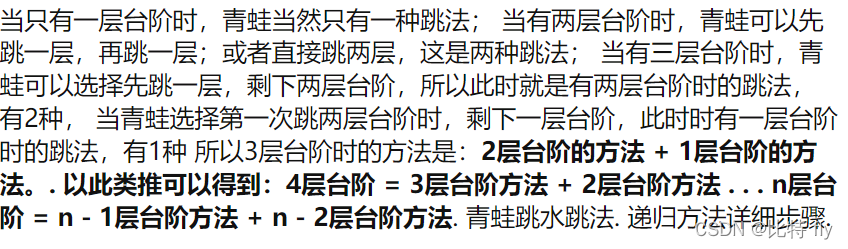
public static int footStep(int n){
if(n==1){
return 1;
}else if(n==2){
return 2;
}else{
return footStep(n-1)+footStep(n-2);
}
}
public static void main(String[] args) {
int n=0;
Scanner sc=new Scanner(System.in);
while(sc.hasNextInt()){
n=sc.nextInt();
int ret=footStep(n);
System.out.println(ret);
}
}
4.3汉诺塔问题
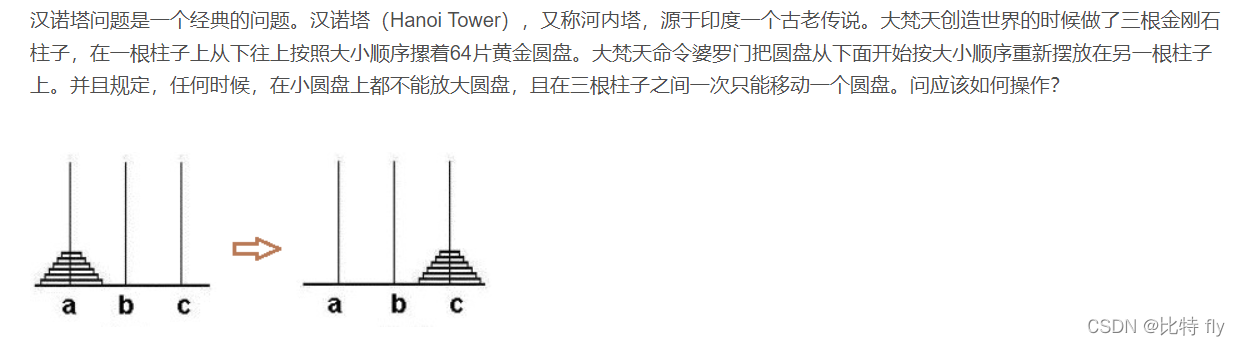
public static int hanoi(int n){
if(n==1){
return 1;
}else{
return 2*hanoi(n-1)+1;
}
}
public static void main(String[] args) {
int n=0;
Scanner sc=new Scanner(System.in);
while(sc.hasNextInt()){
n=sc.nextInt();
int ret=hanoi(n);
System.out.println(ret);
}
}
|