游戏内基本都采用MVC模式实现,主要通过SendEvent来接收时间信息,决定下一步要怎么做。
Game类继承于ApplicationBase,搭在游戏第一个场景,启动后自动跳到下一场景,并且在之后场景一直存在。
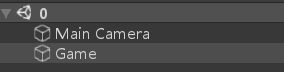 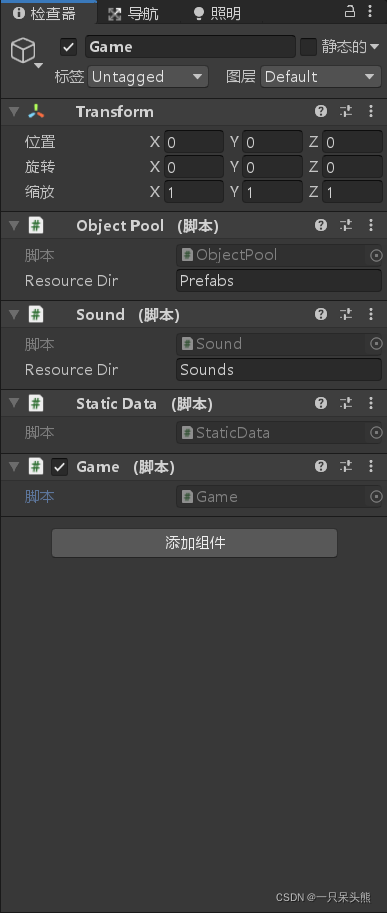
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.SceneManagement;
[RequireComponent(typeof(ObjectPool))]
[RequireComponent(typeof(Sound))]
[RequireComponent(typeof(StaticData))]
public class Game : ApplicationBase<Game>
{
//全局访问功能
[HideInInspector]
public ObjectPool ObjectPool = null;
[HideInInspector]
public Sound Sound = null;
[HideInInspector]
public StaticData StaticData = null; //静态数据
//全局方法
public void LoadScene(int level)
{
//-----退出前一场景
//事件参数
SceneArgs e = new SceneArgs();
e.Level = SceneManager.GetActiveScene().buildIndex;
//发送事件
SendEvent(Const.E_ExitScene, e);
//-----新场景
SceneManager.LoadScene(level, LoadSceneMode.Single);
}
//当前场景加载完后触发
void OnLevelWasLoaded(int level)
{
Debug.Log("OnLevelWasLoaded" + level);
SceneArgs e = new SceneArgs();
e.Level = level;
SendEvent(Const.E_EnterScene, e);
}
//游戏入口
void Start()
{
Object.DontDestroyOnLoad(this.gameObject);
//全局单例赋值
ObjectPool = ObjectPool.Instance;
Sound = Sound.Instance;
StaticData = StaticData.Instance;
//注册启动命令
RegisterController(Const.E_StartUp, typeof(StartUpCommand));
//启动游戏
SendEvent(Const.E_StartUp);
}
}
其中,启动命令StartUpCommand类继承于Controller类:
public class StartUpCommand : Controller
{
public override void Execute(object data)
{
//注册模型
//注册命令
RegisterController(Const.E_EnterScene, typeof(EnterSceneCommand));
RegisterController(Const.E_ExitScene, typeof(ExitSceneCommand));
//进入开始界面
//UnityEngine.SceneManagement.SceneManager.LoadScene(1);
Game.Instance.LoadScene(1);
}
}
|