题目要求如下:
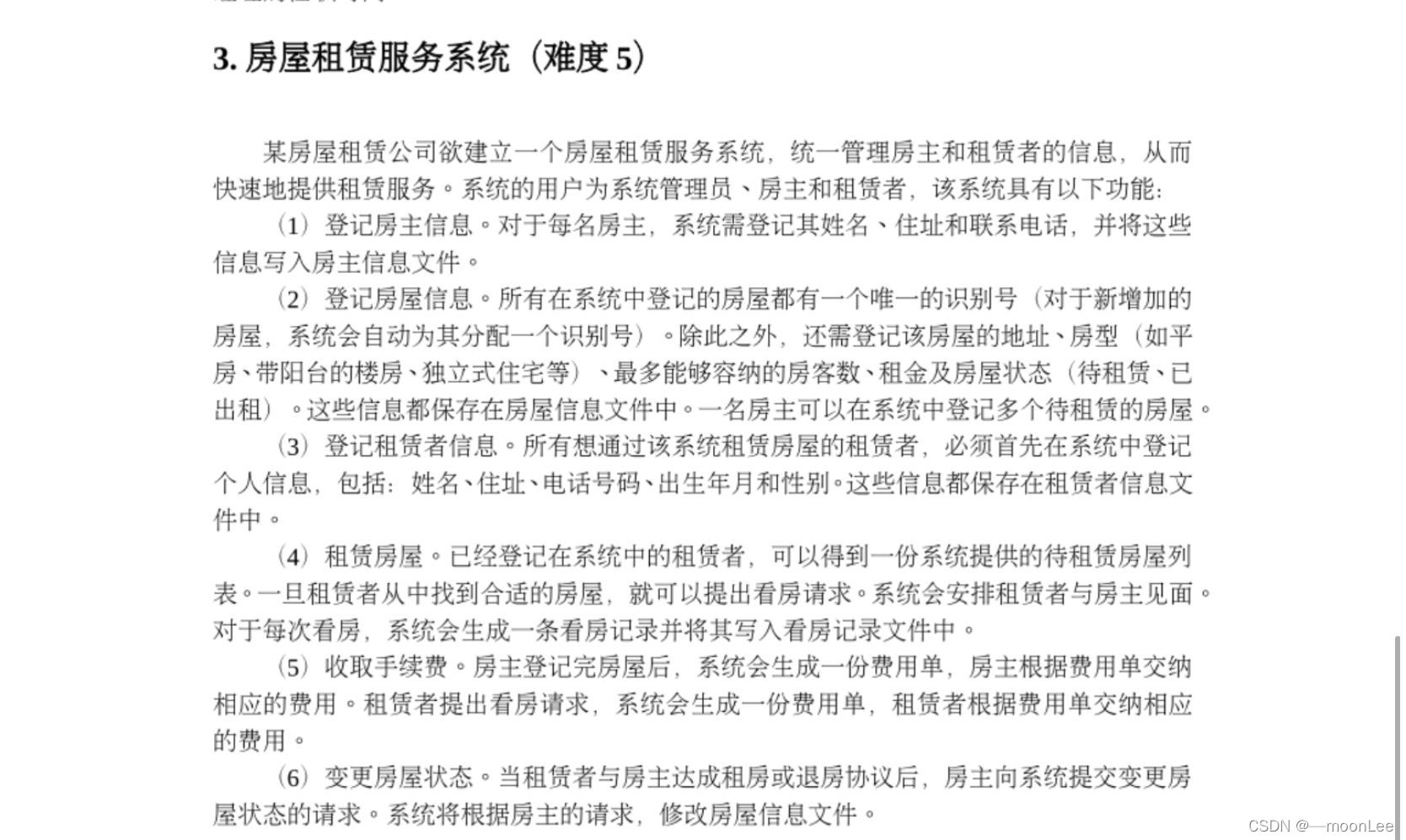
本次主要负责界面的设计
关于java图形界面框架构建:
? 1、Swing是在AWT基础上构建的新的图形界面系统,对AWT进行了扩充。两者的区别:AWT基于c/c++,速度较快;Swing基于AWT的java程序,速度慢一些。基本框架如下图:
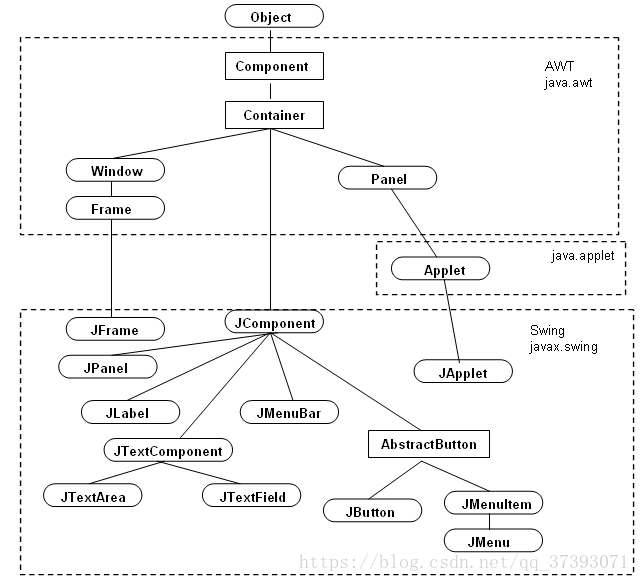
2.图形设计的流程
?java图形界面程序包涵顶层容器、中间容器、组件。在设计这个图形界面的时候就是依次实现这三个的过程。
(1) 对于登录后的管理员界面设计
//登录后的管理员界面
import java.awt.BorderLayout;
import java.awt.EventQueue;
import javax.swing.JFrame;
import javax.swing.JPanel;
import javax.swing.border.EmptyBorder;
import model.Tenant;
import util.LoginConfig;
import view.House;
import javax.swing.JMenuBar;
import javax.swing.JMenu;
import javax.swing.JMenuItem;
import javax.swing.JOptionPane;
import javax.swing.ImageIcon;
import java.awt.event.ActionListener;
import java.sql.SQLException;
import java.awt.event.ActionEvent;
import javax.swing.GroupLayout;
import javax.swing.GroupLayout.Alignment;
import javax.swing.JTable;
public class AdminFrm extends JFrame {
private JPanel contentPane;
private JTable table;
/**
* Launch the application.
*/
public static void main(String[] args) {
EventQueue.invokeLater(new Runnable() {
public void run() {
try {
AdminFrm frame = new AdminFrm();
frame.setVisible(true);
} catch (Exception e) {
e.printStackTrace();
}
}
});
}
/**
* Create the frame.
*/
public AdminFrm() {
setTitle("\u7BA1\u7406\u5458\u754C\u9762");
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setBounds(100, 100, 800, 730);
JMenuBar menuBar = new JMenuBar();
setJMenuBar(menuBar);
JMenu mnNewMenu = new JMenu("\u4FE1\u606F\u7BA1\u7406");
menuBar.add(mnNewMenu);
JMenuItem mntmNewMenuItem = new JMenuItem("\u623F\u5C4B\u4FE1\u606F");
mntmNewMenuItem.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
House userupdatePwd = null;
try {
userupdatePwd = new House();
} catch (ClassNotFoundException | SQLException e1) {
// TODO Auto-generated catch block
e1.printStackTrace();
}
userupdatePwd.setVisible(true);
table.add(userupdatePwd);
}
});
mntmNewMenuItem.setIcon(new ImageIcon(AdminFrm.class.getResource("/image/\u70B9 (1).png")));
mnNewMenu.add(mntmNewMenuItem);
JMenuItem mntmNewMenuItem_1 = new JMenuItem("\u79DF\u5BA2\u4FE1\u606F");
mntmNewMenuItem_1.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
Ten userupdatePwd = null;
try {
userupdatePwd = new Ten();
} catch (ClassNotFoundException | SQLException e1) {
// TODO Auto-generated catch block
e1.printStackTrace();
}
userupdatePwd.setVisible(true);
table.add(userupdatePwd);
}
});
mntmNewMenuItem_1.setIcon(new ImageIcon(AdminFrm.class.getResource("/image/\u70B9 (1).png")));
mnNewMenu.add(mntmNewMenuItem_1);
JMenuItem mntmNewMenuItem_2 = new JMenuItem("\u623F\u4E1C\u4FE1\u606F");
mntmNewMenuItem_2.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
Hos userupdatePwd = null;
try {
userupdatePwd = new Hos();
} catch (ClassNotFoundException | SQLException e1) {
// TODO Auto-generated catch block
e1.printStackTrace();
}
userupdatePwd.setVisible(true);
table.add(userupdatePwd);
}
});
mntmNewMenuItem_2.setIcon(new ImageIcon(AdminFrm.class.getResource("/image/\u70B9 (1).png")));
mnNewMenu.add(mntmNewMenuItem_2);
JMenuItem menuItem = new JMenuItem("\u770B\u623F\u8BB0\u5F55");
menuItem.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
See userupdatePwd = null;
try {
userupdatePwd = new See();
} catch (ClassNotFoundException | SQLException e1) {
// TODO Auto-generated catch block
e1.printStackTrace();
}
userupdatePwd.setVisible(true);
table.add(userupdatePwd);
}
});
menuItem.setIcon(new ImageIcon(AdminFrm.class.getResource("/image/\u70B9 (1).png")));
mnNewMenu.add(menuItem);
JMenuItem menuItem_1 = new JMenuItem("\u79DF\u623F\u8BB0\u5F55");
menuItem_1.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
Rent userupdatePwd = null;
try {
userupdatePwd = new Rent();
} catch (ClassNotFoundException | SQLException e1) {
// TODO Auto-generated catch block
e1.printStackTrace();
}
userupdatePwd.setVisible(true);
table.add(userupdatePwd);
}
});
menuItem_1.setIcon(new ImageIcon(AdminFrm.class.getResource("/image/\u70B9 (1).png")));
mnNewMenu.add(menuItem_1);
JMenuItem menuItem_3 = new JMenuItem("\u9000\u51FA");
menuItem_3.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent arg0) {
//点是返回0.否返回1
int x=JOptionPane.showConfirmDialog(null, "是否退出?");
if(x==0) {
dispose();
LoginConfig.clean();
}
}
});
menuItem_3.setIcon(new ImageIcon(AdminFrm.class.getResource("/image/\u70B9 (1).png")));
mnNewMenu.add(menuItem_3);
contentPane = new JPanel();
contentPane.setBorder(new EmptyBorder(5, 5, 5, 5));
setContentPane(contentPane);
contentPane.setLayout(new BorderLayout(0, 0));
table = new JTable();
contentPane.add(table, BorderLayout.CENTER);
this.setLocationRelativeTo(null);
}
}
(2)退租界面
//退租界面
import java.awt.BorderLayout;
import java.awt.EventQueue;
import javax.swing.JFrame;
import javax.swing.JPanel;
import javax.swing.border.EmptyBorder;
import dao.UserDao;
import dao.hostDao;
import javax.swing.GroupLayout;
import javax.swing.GroupLayout.Alignment;
import javax.swing.JLabel;
import java.awt.Font;
import javax.swing.JTextField;
import javax.swing.JButton;
import javax.swing.LayoutStyle.ComponentPlacement;
import java.awt.event.ActionListener;
import java.sql.SQLException;
import java.awt.event.ActionEvent;
public class Del extends JFrame {
private JPanel contentPane;
private JTextField addtxt;
private UserDao userdao=new UserDao();
private hostDao hostdao=new hostDao();
/**
* Launch the application.
*/
public static void main(String[] args) {
EventQueue.invokeLater(new Runnable() {
public void run() {
try {
Del frame = new Del();
frame.setVisible(true);
} catch (Exception e) {
e.printStackTrace();
}
}
});
}
/**
* Create the frame.
*/
public Del() {
setTitle("\u623F\u5C4B\u9000\u79DF");
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setBounds(100, 100, 800, 730);
contentPane = new JPanel();
contentPane.setBorder(new EmptyBorder(5, 5, 5, 5));
setContentPane(contentPane);
JLabel label = new JLabel("\u623F\u5C4B\u9000\u79DF");
label.setFont(new Font("宋体", Font.BOLD, 35));
JLabel label_1 = new JLabel("\u5730\u5740");
label_1.setFont(new Font("宋体", Font.BOLD, 25));
addtxt = new JTextField();
addtxt.setColumns(10);
JButton button = new JButton("\u9000\u79DF");
button.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
try {
delperformed(e);
} catch (ClassNotFoundException | SQLException e1) {
// TODO Auto-generated catch block
e1.printStackTrace();
}
}
});
button.setFont(new Font("宋体", Font.BOLD, 25));
GroupLayout gl_contentPane = new GroupLayout(contentPane);
gl_contentPane.setHorizontalGroup(
gl_contentPane.createParallelGroup(Alignment.LEADING)
.addGroup(gl_contentPane.createSequentialGroup()
.addGroup(gl_contentPane.createParallelGroup(Alignment.LEADING)
.addGroup(gl_contentPane.createSequentialGroup()
.addGap(185)
.addComponent(label_1)
.addGap(51)
.addGroup(gl_contentPane.createParallelGroup(Alignment.LEADING)
.addComponent(label)
.addComponent(addtxt, GroupLayout.PREFERRED_SIZE, 254, GroupLayout.PREFERRED_SIZE)))
.addGroup(gl_contentPane.createSequentialGroup()
.addGap(322)
.addComponent(button)))
.addContainerGap(230, Short.MAX_VALUE))
);
gl_contentPane.setVerticalGroup(
gl_contentPane.createParallelGroup(Alignment.LEADING)
.addGroup(gl_contentPane.createSequentialGroup()
.addGap(85)
.addComponent(label)
.addGap(121)
.addGroup(gl_contentPane.createParallelGroup(Alignment.BASELINE)
.addComponent(addtxt, GroupLayout.PREFERRED_SIZE, 31, GroupLayout.PREFERRED_SIZE)
.addComponent(label_1, GroupLayout.DEFAULT_SIZE, GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE))
.addGap(188)
.addComponent(button)
.addGap(164))
);
contentPane.setLayout(gl_contentPane);
this.setLocationRelativeTo(null);
}
//退租函数 调用userdao和hostdao中的函数
protected void delperformed(ActionEvent e) throws ClassNotFoundException, SQLException {
// TODO Auto-generated method stub
String address=this.addtxt.getText();
int i=hostdao.delhouse(address);
int j=userdao.delrent(address);
if(i>0&&j>0) {
dispose();
}
}
}
(3)房东信息表
//房东信息表
import java.awt.EventQueue;
import java.sql.SQLException;
import java.util.List;
import java.util.Vector;
import javax.swing.JInternalFrame;
import javax.swing.JScrollPane;
import java.awt.BorderLayout;
import javax.swing.JTable;
import javax.swing.table.DefaultTableModel;
import dao.UserDao;
import model.Host;
import model.Tenant;
public class Hos extends JInternalFrame {
private JTable table;
private UserDao userDao = new UserDao();
/**
* Launch the application.
*/
public static void main(String[] args) {
EventQueue.invokeLater(new Runnable() {
public void run() {
try {
Hos frame = new Hos();
frame.setVisible(true);
} catch (Exception e) {
e.printStackTrace();
}
}
});
}
/**
* Create the frame.
* @throws SQLException
* @throws ClassNotFoundException
*/
public Hos() throws ClassNotFoundException, SQLException {
setTitle("\u623F\u4E1C\u4FE1\u606F");
setMaximizable(true);
setIconifiable(true);
setClosable(true);
setBounds(100, 100, 800, 730);
JScrollPane scrollPane = new JScrollPane();
getContentPane().add(scrollPane, BorderLayout.CENTER);
table = new JTable();
table.setModel(new DefaultTableModel(
new Object[][] {
},
new String[] {
"\u59D3\u540D", "\u5730\u5740", "\u7535\u8BDD"
}
));
scrollPane.setViewportView(table);
fillTable();
}
//对表初始化,这样打开表就能看到内容
public void fillTable() throws ClassNotFoundException, SQLException {
DefaultTableModel dtm =(DefaultTableModel)table.getModel();
dtm.setRowCount(0);
//查询数据
List<Host> h=userDao.listenhost();
for(Host house:h) {
Vector v=new Vector<>();
v.add(house.getName());
v.add(house.getAddress());
v.add(house.getPhone());
dtm.addRow(v);
}
}
}
(4)登陆后的房东界面
//登陆后的房东界面
import java.awt.BorderLayout;
import java.awt.EventQueue;
import javax.swing.JFrame;
import javax.swing.JPanel;
import javax.swing.border.EmptyBorder;
import model.Tenant;
import util.LoginConfig;
import javax.swing.JMenuBar;
import javax.swing.JMenu;
import javax.swing.JMenuItem;
import javax.swing.JOptionPane;
import javax.swing.ImageIcon;
import java.awt.event.ActionListener;
import java.sql.SQLException;
import java.awt.event.ActionEvent;
import javax.swing.JTable;
public class HostFrm extends JFrame {
private JPanel contentPane;
private JTable table;
/**
* Launch the application.
*/
public static void main(String[] args) {
EventQueue.invokeLater(new Runnable() {
public void run() {
try {
HostFrm frame = new HostFrm();
frame.setVisible(true);
} catch (Exception e) {
e.printStackTrace();
}
}
});
}
/**
* Create the frame.
*/
public HostFrm() {
setTitle("\u623F\u4E1C\u754C\u9762");
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setBounds(100, 100, 800, 730);
JMenuBar menuBar = new JMenuBar();
setJMenuBar(menuBar);
JMenu mnNewMenu = new JMenu("\u4FE1\u606F\u67E5\u8BE2");
menuBar.add(mnNewMenu);
JMenuItem mntmNewMenuItem = new JMenuItem("\u623F\u5C4B\u4FE1\u606F");
mntmNewMenuItem.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
House userupdatePwd = null;
try {
userupdatePwd = new House();
} catch (ClassNotFoundException | SQLException e1) {
// TODO Auto-generated catch block
e1.printStackTrace();
}
userupdatePwd.setVisible(true);
table.add(userupdatePwd);
}
});
mntmNewMenuItem.setIcon(new ImageIcon(HostFrm.class.getResource("/image/\u70B9 (1).png")));
mnNewMenu.add(mntmNewMenuItem);
JMenuItem mntmNewMenuItem_1 = new JMenuItem("\u623F\u5BA2\u4FE1\u606F");
mntmNewMenuItem_1.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
Ten userupdatePwd = null;
try {
userupdatePwd = new Ten();
} catch (ClassNotFoundException | SQLException e1) {
// TODO Auto-generated catch block
e1.printStackTrace();
}
userupdatePwd.setVisible(true);
table.add(userupdatePwd);
}
});
mntmNewMenuItem_1.setIcon(new ImageIcon(HostFrm.class.getResource("/image/\u70B9 (1).png")));
mnNewMenu.add(mntmNewMenuItem_1);
JMenuItem mntmNewMenuItem_2 = new JMenuItem("\u9000\u51FA");
mntmNewMenuItem_2.setIcon(new ImageIcon(HostFrm.class.getResource("/image/\u70B9 (1).png")));
mntmNewMenuItem_2.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
//点是返回0.否返回1
int x=JOptionPane.showConfirmDialog(null, "是否退出?");
if(x==0) {
dispose();
LoginConfig.clean();
}
}
});
mnNewMenu.add(mntmNewMenuItem_2);
JMenu mnNewMenu_1 = new JMenu("\u4FE1\u606F\u7BA1\u7406");
menuBar.add(mnNewMenu_1);
JMenuItem menuItem = new JMenuItem("\u623F\u5C4B\u767B\u8BB0");
menuItem.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
new Reghouse().setVisible(true);
}
});
JMenuItem menuItem_2 = new JMenuItem("\u4E2A\u4EBA\u4FE1\u606F\u767B\u8BB0");
menuItem_2.setIcon(new ImageIcon(HostFrm.class.getResource("/image/\u70B9 (1).png")));
menuItem_2.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
new Reghost().setVisible(true);
}
});
mnNewMenu_1.add(menuItem_2);
menuItem.setIcon(new ImageIcon(HostFrm.class.getResource("/image/\u70B9 (1).png")));
mnNewMenu_1.add(menuItem);
JMenuItem menuItem_1 = new JMenuItem("\u623F\u5C4B\u51FA\u79DF");
menuItem_1.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent arg0) {
new Houserent().setVisible(true);
}
});
menuItem_1.setIcon(new ImageIcon(HostFrm.class.getResource("/image/\u70B9 (1).png")));
mnNewMenu_1.add(menuItem_1);
JMenuItem menuItem_3 = new JMenuItem("\u623F\u5C4B\u6536\u56DE");
menuItem_3.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
new Del().setVisible(true);
}
});
menuItem_3.setIcon(new ImageIcon(HostFrm.class.getResource("/image/\u70B9 (1).png")));
mnNewMenu_1.add(menuItem_3);
contentPane = new JPanel();
contentPane.setBorder(new EmptyBorder(5, 5, 5, 5));
contentPane.setLayout(new BorderLayout(0, 0));
setContentPane(contentPane);
table = new JTable();
contentPane.add(table, BorderLayout.CENTER);
this.setLocationRelativeTo(null);
}
}
(5)房屋信息
//房屋信息
import java.awt.EventQueue;
import java.sql.SQLException;
import java.util.List;
import java.util.Vector;
import javax.swing.JInternalFrame;
import javax.swing.JScrollPane;
import java.awt.BorderLayout;
import javax.swing.JTable;
import javax.swing.table.DefaultTableModel;
import dao.UserDao;
import model.Houses;
public class House extends JInternalFrame {
private JTable table;
private UserDao userDao = new UserDao();
/**
* Launch the application.
*/
public static void main(String[] args) {
EventQueue.invokeLater(new Runnable() {
public void run() {
try {
House frame = new House();
frame.setVisible(true);
} catch (Exception e) {
e.printStackTrace();
}
}
});
}
/**
* Create the frame.
* @throws SQLException
* @throws ClassNotFoundException
*/
public House() throws ClassNotFoundException, SQLException {
setIconifiable(true);
setMaximizable(true);
setClosable(true);
setTitle("\u623F\u5C4B\u4FE1\u606F");
setBounds(100, 100, 800, 730);
JScrollPane scrollPane = new JScrollPane();
getContentPane().add(scrollPane, BorderLayout.CENTER);
table = new JTable();
table.setModel(new DefaultTableModel(
new Object[][] {
},
new String[] {
"\u7F16\u53F7", "\u5730\u5740", "\u623F\u578B", "\u623F\u5BA2\u6570", "\u79DF\u91D1", "\u624B\u7EED\u8D39", "\u72B6\u6001"
}
));
scrollPane.setViewportView(table);
fillTable();
}
/*
* 初始化表格
*/
public void fillTable() throws ClassNotFoundException, SQLException {
DefaultTableModel dtm =(DefaultTableModel)table.getModel();
dtm.setRowCount(0);
//查询数据
List<Houses> h=userDao.listhouse();
for(Houses house:h) {
Vector v=new Vector<>();
v.add(dtm.getRowCount()+1);
v.add(house.getAddress());
v.add(house.getType());
v.add(house.getMax());
v.add(house.getRent());
v.add(house.getFee());
v.add(house.getStatus());
dtm.addRow(v);
}
}
}
(6)房屋出租登记
//房屋出租登记
import java.awt.BorderLayout;
import java.awt.EventQueue;
import javax.swing.JFrame;
import javax.swing.JPanel;
import javax.swing.border.EmptyBorder;
import dao.UserDao;
import dao.hostDao;
import javax.swing.GroupLayout;
import javax.swing.GroupLayout.Alignment;
import javax.swing.JLabel;
import java.awt.Font;
import javax.swing.JTextField;
import javax.swing.WindowConstants;
import javax.swing.JButton;
import javax.swing.LayoutStyle.ComponentPlacement;
import java.awt.event.ActionListener;
import java.sql.SQLException;
import java.awt.event.ActionEvent;
public class Houserent extends JFrame {
private JPanel contentPane;
private JTextField nametxt;
private JTextField addtxt;
private UserDao userdao=new UserDao();
private hostDao hostdao=new hostDao();
private JTextField retxt;
/**
* Launch the application.
*/
public static void main(String[] args) {
EventQueue.invokeLater(new Runnable() {
public void run() {
try {
Houserent frame = new Houserent();
frame.setVisible(true);
} catch (Exception e) {
e.printStackTrace();
}
}
});
}
/**
* Create the frame.
*/
public Houserent() {
setTitle("\u623F\u5C4B\u51FA\u79DF");
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setBounds(100, 100, 800, 730);
contentPane = new JPanel();
contentPane.setBorder(new EmptyBorder(5, 5, 5, 5));
setContentPane(contentPane);
JLabel label = new JLabel("\u623F\u5C4B\u51FA\u79DF");
label.setFont(new Font("宋体", Font.BOLD, 35));
JLabel label_1 = new JLabel("\u623F\u4E1C\u59D3\u540D");
label_1.setFont(new Font("宋体", Font.BOLD, 25));
JLabel label_2 = new JLabel("\u5730\u5740");
label_2.setFont(new Font("宋体", Font.BOLD, 25));
nametxt = new JTextField();
nametxt.setColumns(10);
addtxt = new JTextField();
addtxt.setColumns(10);
JButton button = new JButton("\u51FA\u79DF");
button.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent arg0) {
try {
houserentperformed(arg0);
} catch (ClassNotFoundException | SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
});
button.setFont(new Font("宋体", Font.BOLD, 20));
JLabel lblNewLabel = new JLabel("\u79DF\u6237\u59D3\u540D");
lblNewLabel.setFont(new Font("宋体", Font.BOLD, 25));
retxt = new JTextField();
retxt.setColumns(10);
GroupLayout gl_contentPane = new GroupLayout(contentPane);
gl_contentPane.setHorizontalGroup(
gl_contentPane.createParallelGroup(Alignment.LEADING)
.addGroup(gl_contentPane.createSequentialGroup()
.addGroup(gl_contentPane.createParallelGroup(Alignment.LEADING)
.addGroup(gl_contentPane.createSequentialGroup()
.addGap(291)
.addComponent(label))
.addGroup(gl_contentPane.createSequentialGroup()
.addGap(113)
.addGroup(gl_contentPane.createParallelGroup(Alignment.LEADING)
.addComponent(label_1)
.addComponent(label_2)
.addComponent(lblNewLabel))
.addGap(54)
.addGroup(gl_contentPane.createParallelGroup(Alignment.LEADING)
.addComponent(retxt, GroupLayout.PREFERRED_SIZE, 277, GroupLayout.PREFERRED_SIZE)
.addGroup(gl_contentPane.createParallelGroup(Alignment.LEADING, false)
.addComponent(addtxt)
.addComponent(nametxt, GroupLayout.DEFAULT_SIZE, 277, Short.MAX_VALUE))))
.addGroup(gl_contentPane.createSequentialGroup()
.addGap(301)
.addComponent(button)))
.addContainerGap(224, Short.MAX_VALUE))
);
gl_contentPane.setVerticalGroup(
gl_contentPane.createParallelGroup(Alignment.LEADING)
.addGroup(gl_contentPane.createSequentialGroup()
.addGap(85)
.addComponent(label)
.addGap(69)
.addGroup(gl_contentPane.createParallelGroup(Alignment.LEADING, false)
.addComponent(nametxt, GroupLayout.PREFERRED_SIZE, GroupLayout.DEFAULT_SIZE, GroupLayout.PREFERRED_SIZE)
.addComponent(label_1, GroupLayout.DEFAULT_SIZE, GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE))
.addGap(80)
.addGroup(gl_contentPane.createParallelGroup(Alignment.LEADING, false)
.addComponent(addtxt, GroupLayout.PREFERRED_SIZE, GroupLayout.DEFAULT_SIZE, GroupLayout.PREFERRED_SIZE)
.addComponent(label_2, GroupLayout.DEFAULT_SIZE, GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE))
.addGap(67)
.addGroup(gl_contentPane.createParallelGroup(Alignment.BASELINE)
.addComponent(lblNewLabel)
.addComponent(retxt, GroupLayout.PREFERRED_SIZE, GroupLayout.DEFAULT_SIZE, GroupLayout.PREFERRED_SIZE))
.addPreferredGap(ComponentPlacement.RELATED, 64, Short.MAX_VALUE)
.addComponent(button)
.addGap(144))
);setDefaultCloseOperation(WindowConstants.HIDE_ON_CLOSE);
contentPane.setLayout(gl_contentPane);
this.setLocationRelativeTo(null);
}
//同样的使用一个函数
protected void houserentperformed(ActionEvent arg0) throws ClassNotFoundException, SQLException {
String address=this.addtxt.getText();
String rname=this.retxt.getText();
int i=hostdao.houserent(address);
int j=userdao.houserent_1(rname,address);
if(i>0&&j>0) {
dispose();
}
}
}
(7)登录界面
//登录界面
import java.awt.BorderLayout;
import java.awt.EventQueue;
import javax.swing.JFrame;
import javax.swing.JPanel;
import javax.swing.border.EmptyBorder;
import dao.UserDao;
import model.User;
import util.LoginConfig;
import util.StringUtil;
import view.RegFrm;
import javax.swing.GroupLayout;
import javax.swing.GroupLayout.Alignment;
import javax.swing.JLabel;
import javax.swing.JOptionPane;
import java.awt.Font;
import javax.swing.ImageIcon;
import javax.swing.LayoutStyle.ComponentPlacement;
import javax.swing.JTextField;
import javax.swing.JPasswordField;
import javax.swing.JComboBox;
import javax.swing.DefaultComboBoxModel;
import javax.swing.JButton;
import java.awt.event.ActionListener;
import java.sql.SQLException;
import java.util.List;
import java.awt.event.ActionEvent;
public class LoginFrm extends JFrame {
private JPanel contentPane;
private JTextField usernametxt;
private JPasswordField pwdtxt;
private JComboBox combox;
/**
* Launch the application.
*/
public static void main(String[] args) {
EventQueue.invokeLater(new Runnable() {
public void run() {
try {
LoginFrm frame = new LoginFrm();
frame.setVisible(true);
} catch (Exception e) {
e.printStackTrace();
}
}
});
}
/**
* Create the frame.
*/
public LoginFrm() {
setTitle("\u767B\u5F55");
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setBounds(100, 100, 551, 535);
contentPane = new JPanel();
contentPane.setBorder(new EmptyBorder(5, 5, 5, 5));
setContentPane(contentPane);
JLabel label = new JLabel("\u623F\u5C4B\u79DF\u8D41\u670D\u52A1\u7CFB\u7EDF");
label.setIcon(new ImageIcon(LoginFrm.class.getResource("/image/\u9996\u9875 (1).png")));
label.setFont(new Font("宋体", Font.BOLD, 40));
JLabel label_1 = new JLabel("\u7528\u6237\u540D");
label_1.setIcon(new ImageIcon(LoginFrm.class.getResource("/image/\u4E2A\u4EBA (3).png")));
label_1.setFont(new Font("宋体", Font.BOLD, 25));
JLabel label_2 = new JLabel("\u5BC6\u7801");
label_2.setIcon(new ImageIcon(LoginFrm.class.getResource("/image/\u5BC6\u7801 (1).png")));
label_2.setFont(new Font("宋体", Font.BOLD, 25));
JLabel label_3 = new JLabel("\u8EAB\u4EFD");
label_3.setIcon(new ImageIcon(LoginFrm.class.getResource("/image/\u6743\u9650.png")));
label_3.setFont(new Font("宋体", Font.BOLD, 25));
usernametxt = new JTextField();
usernametxt.setColumns(10);
pwdtxt = new JPasswordField();
JButton btnNewButton = new JButton("\u767B\u5F55");
btnNewButton.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
try {
LoginActionPerformed(e);
} catch (ClassNotFoundException | SQLException e1) {
// TODO Auto-generated catch block
e1.printStackTrace();
}
}
});
btnNewButton.setFont(new Font("宋体", Font.BOLD, 20));
JButton btnNewButton_1 = new JButton("\u6CE8\u518C");
btnNewButton_1.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
regactionPerformed(e);
}
});
btnNewButton_1.setFont(new Font("宋体", Font.BOLD, 20));
JButton btnNewButton_2 = new JButton("\u91CD\u7F6E");
btnNewButton_2.setFont(new Font("宋体", Font.BOLD, 20));
btnNewButton_2.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
ResetactionPerformed(e);
}
});
combox = new JComboBox();
combox.setModel(new DefaultComboBoxModel(new String[] {"\u79DF\u6237", "\u623F\u4E1C", "\u7BA1\u7406\u5458"}));
combox.setFont(new Font("宋体", Font.BOLD, 20));
JLabel label_4 = new JLabel("\u5F00\u53D1\u8005\uFF1A\u59DC\u7545 \u674E\u4F73\u9759 \u6A0A\u7949\u9633");
GroupLayout gl_contentPane = new GroupLayout(contentPane);
gl_contentPane.setHorizontalGroup(
gl_contentPane.createParallelGroup(Alignment.LEADING)
.addGroup(gl_contentPane.createSequentialGroup()
.addGap(63)
.addGroup(gl_contentPane.createParallelGroup(Alignment.LEADING)
.addComponent(label)
.addGroup(gl_contentPane.createSequentialGroup()
.addGroup(gl_contentPane.createParallelGroup(Alignment.LEADING)
.addComponent(label_1)
.addComponent(label_2)
.addComponent(label_3))
.addGap(18)
.addGroup(gl_contentPane.createParallelGroup(Alignment.LEADING)
.addComponent(combox, GroupLayout.PREFERRED_SIZE, 98, GroupLayout.PREFERRED_SIZE)
.addGroup(gl_contentPane.createParallelGroup(Alignment.LEADING, false)
.addComponent(usernametxt, GroupLayout.DEFAULT_SIZE, 248, Short.MAX_VALUE)
.addComponent(pwdtxt)))))
.addContainerGap(72, Short.MAX_VALUE))
.addGroup(gl_contentPane.createSequentialGroup()
.addGap(41)
.addComponent(btnNewButton)
.addGap(106)
.addComponent(btnNewButton_1)
.addPreferredGap(ComponentPlacement.RELATED, 108, Short.MAX_VALUE)
.addComponent(btnNewButton_2)
.addGap(43))
.addGroup(gl_contentPane.createSequentialGroup()
.addGap(152)
.addComponent(label_4)
.addContainerGap(175, Short.MAX_VALUE))
);
gl_contentPane.setVerticalGroup(
gl_contentPane.createParallelGroup(Alignment.LEADING)
.addGroup(gl_contentPane.createSequentialGroup()
.addGap(54)
.addComponent(label)
.addGap(18)
.addComponent(label_4)
.addGap(42)
.addGroup(gl_contentPane.createParallelGroup(Alignment.BASELINE)
.addComponent(label_1)
.addComponent(usernametxt, GroupLayout.PREFERRED_SIZE, 32, GroupLayout.PREFERRED_SIZE))
.addGap(26)
.addGroup(gl_contentPane.createParallelGroup(Alignment.BASELINE)
.addComponent(pwdtxt, GroupLayout.PREFERRED_SIZE, 32, GroupLayout.PREFERRED_SIZE)
.addComponent(label_2, GroupLayout.DEFAULT_SIZE, GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE))
.addGap(22)
.addGroup(gl_contentPane.createParallelGroup(Alignment.BASELINE)
.addComponent(label_3)
.addComponent(combox, GroupLayout.PREFERRED_SIZE, 32, GroupLayout.PREFERRED_SIZE))
.addGap(49)
.addGroup(gl_contentPane.createParallelGroup(Alignment.BASELINE)
.addComponent(btnNewButton)
.addComponent(btnNewButton_1)
.addComponent(btnNewButton_2))
.addGap(62))
);
contentPane.setLayout(gl_contentPane);
this.setLocationRelativeTo(null);
}
protected void regactionPerformed(ActionEvent e) {
// TODO Auto-generated method stub
this.dispose();
new RegFrm().setVisible(true);
}
/*
* 重置功能
*/
protected void ResetactionPerformed(ActionEvent e) {
// TODO Auto-generated method stub
reset();
}
public void reset() {
this.usernametxt.setText("");
this.pwdtxt.setText("");
}
/*
* 对登录的处理 id不同身份不同
*/
private void LoginActionPerformed(ActionEvent e) throws ClassNotFoundException, SQLException {
// TODO Auto-generated method stub
String name=this.usernametxt.getText();
String pwd=new String(this.pwdtxt.getPassword());
String qx=(String)(String) this.combox.getSelectedItem();
String userid="";
if(qx.equals("租户")){
userid="2";
List<User> list=UserDao.login(name,pwd,userid);
if(list.size()!=0) {
dispose();
LoginConfig.save(new User(name,pwd,userid));
new MainFrm().setVisible(true);
}else {
JOptionPane.showMessageDialog(null, "登陆失败");
}
}
else if(qx.equals("房东")) {
userid="3";
List<User> list=UserDao.login(name,pwd,userid);
if(list.size()!=0) {
dispose();
LoginConfig.save(new User(name,pwd,userid));
new HostFrm().setVisible(true);
}else {
JOptionPane.showMessageDialog(null, "登陆失败");
}
}
else {
userid="1";
List<User> list=UserDao.login(name,pwd,userid);
if(list.size()!=0) {
dispose();
LoginConfig.save(new User(name,pwd,userid));
new AdminFrm().setVisible(true);
}else {
JOptionPane.showMessageDialog(null, "登陆失败");
}
}
if(StringUtil.isEmpty(name)) {
JOptionPane.showMessageDialog(null, "用户名不能为空");
}
if(StringUtil.isEmpty(pwd)) {
JOptionPane.showMessageDialog(null, "密码不能为空");
}
}
}
(8)登录后的租户界面
//登陆后的租户界面
import java.awt.BorderLayout;
import java.awt.EventQueue;
import javax.swing.JFrame;
import javax.swing.JPanel;
import javax.swing.border.EmptyBorder;
import util.LoginConfig;
import javax.swing.JMenuBar;
import javax.swing.JMenu;
import javax.swing.JMenuItem;
import javax.swing.JOptionPane;
import javax.swing.ImageIcon;
import java.awt.event.ActionListener;
import java.sql.SQLException;
import java.awt.event.ActionEvent;
import javax.swing.JTable;
public class MainFrm extends JFrame {
private JPanel contentPane;
private JTable table;
/**
* Launch the application.
*/
public static void main(String[] args) {
EventQueue.invokeLater(new Runnable() {
public void run() {
try {
MainFrm frame = new MainFrm();
frame.setVisible(true);
} catch (Exception e) {
e.printStackTrace();
}
}
});
}
/**
* Create the frame.
*/
public MainFrm() {
setTitle("\u79DF\u6237\u754C\u9762");
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setBounds(100, 100, 800, 730);
JMenuBar menuBar = new JMenuBar();
setJMenuBar(menuBar);
JMenu mnNewMenu = new JMenu("\u7528\u6237\u4FE1\u606F");
menuBar.add(mnNewMenu);
JMenuItem menuItem_4 = new JMenuItem("\u4E2A\u4EBA\u4FE1\u606F");
menuItem_4.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent arg0) {
new Regten().setVisible(true);
}
});
menuItem_4.setIcon(new ImageIcon(MainFrm.class.getResource("/image/\u70B9 (1).png")));
mnNewMenu.add(menuItem_4);
JMenuItem menuItem_1 = new JMenuItem("\u8BF7\u6C42\u770B\u623F");
menuItem_1.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
new Seehouse().setVisible(true);
}
});
menuItem_1.setIcon(new ImageIcon(MainFrm.class.getResource("/image/\u70B9 (1).png")));
mnNewMenu.add(menuItem_1);
JMenuItem menuItem_3 = new JMenuItem("\u9000\u51FA");
menuItem_3.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
//点是返回0.否返回1
int x=JOptionPane.showConfirmDialog(null, "是否退出?");
if(x==0) {
dispose();
LoginConfig.clean();
}
}
});
menuItem_3.setIcon(new ImageIcon(MainFrm.class.getResource("/image/\u70B9 (1).png")));
mnNewMenu.add(menuItem_3);
JMenu mnNewMenu_1 = new JMenu("\u623F\u6E90\u60C5\u51B5");
menuBar.add(mnNewMenu_1);
JMenuItem menuItem_5 = new JMenuItem("\u623F\u5C4B\u4FE1\u606F");
menuItem_5.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
House userupdatePwd = null;
try {
userupdatePwd = new House();
} catch (ClassNotFoundException | SQLException e1) {
// TODO Auto-generated catch block
e1.printStackTrace();
}
userupdatePwd.setVisible(true);
table.add(userupdatePwd);
}
});
menuItem_5.setIcon(new ImageIcon(MainFrm.class.getResource("/image/\u70B9 (1).png")));
mnNewMenu_1.add(menuItem_5);
contentPane = new JPanel();
contentPane.setBorder(new EmptyBorder(5, 5, 5, 5));
contentPane.setLayout(new BorderLayout(0, 0));
setContentPane(contentPane);
table = new JTable();
contentPane.add(table, BorderLayout.CENTER);
this.setLocationRelativeTo(null);
}
}
(9)注册界面
import java.awt.BorderLayout;
import java.awt.EventQueue;
import javax.swing.JFrame;
import javax.swing.JPanel;
import javax.swing.border.EmptyBorder;
import dao.UserDao;
import util.StringUtil;
import javax.swing.GroupLayout;
import javax.swing.GroupLayout.Alignment;
import javax.swing.JLabel;
import javax.swing.JOptionPane;
import java.awt.Font;
import javax.swing.ImageIcon;
import javax.swing.LayoutStyle.ComponentPlacement;
import javax.swing.JTextField;
import javax.swing.JButton;
import javax.swing.JPasswordField;
import java.awt.event.ActionListener;
import java.sql.SQLException;
import java.awt.event.ActionEvent;
//注册界面
public class RegFrm extends JFrame {
private JPanel contentPane;
private JTextField usernametxt;
private JPasswordField pwdtxt;
private UserDao userdao=new UserDao();
private JTextField qxtxt;
/**
* Launch the application.
*/
public static void main(String[] args) {
EventQueue.invokeLater(new Runnable() {
public void run() {
try {
RegFrm frame = new RegFrm();
frame.setVisible(true);
} catch (Exception e) {
e.printStackTrace();
}
}
});
}
/**
* Create the frame.
*/
public RegFrm() {
setTitle("\u6CE8\u518C");
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setBounds(100, 100, 551, 535);
contentPane = new JPanel();
contentPane.setBorder(new EmptyBorder(5, 5, 5, 5));
setContentPane(contentPane);
JLabel label = new JLabel("\u7528\u6237\u6CE8\u518C");
label.setFont(new Font("宋体", Font.BOLD, 35));
JLabel label_1 = new JLabel("\u7528\u6237\u540D");
label_1.setIcon(new ImageIcon(RegFrm.class.getResource("/image/\u4E2A\u4EBA (3).png")));
label_1.setFont(new Font("宋体", Font.BOLD, 25));
JLabel label_2 = new JLabel("\u5BC6\u7801");
label_2.setFont(new Font("宋体", Font.BOLD, 25));
label_2.setIcon(new ImageIcon(RegFrm.class.getResource("/image/\u5BC6\u7801 (1).png")));
usernametxt = new JTextField();
usernametxt.setColumns(10);
JButton btnNewButton = new JButton("\u6CE8\u518C");
btnNewButton.setFont(new Font("宋体", Font.BOLD, 25));
btnNewButton.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
try {
regactionPerformed(e);
} catch (ClassNotFoundException | SQLException e1) {
// TODO Auto-generated catch block
e1.printStackTrace();
}
}
});
pwdtxt = new JPasswordField();
JLabel label_3 = new JLabel("\u8EAB\u4EFD");
label_3.setIcon(new ImageIcon(RegFrm.class.getResource("/image/\u6743\u9650.png")));
label_3.setFont(new Font("宋体", Font.BOLD, 25));
qxtxt = new JTextField();
qxtxt.setColumns(10);
JLabel label_4 = new JLabel("\u8EAB\u4EFD\u5904\u7BA1\u7406\u5458\u8BF7\u586B\u201C1\u201D\uFF0C\u79DF\u6237\u8BF7\u586B\u201C2\u201D\uFF0C\u623F\u4E1C\u8BF7\u586B\u201C3\u201D");
GroupLayout gl_contentPane = new GroupLayout(contentPane);
gl_contentPane.setHorizontalGroup(
gl_contentPane.createParallelGroup(Alignment.LEADING)
.addGroup(gl_contentPane.createSequentialGroup()
.addGroup(gl_contentPane.createParallelGroup(Alignment.LEADING)
.addGroup(gl_contentPane.createParallelGroup(Alignment.LEADING, false)
.addGroup(gl_contentPane.createSequentialGroup()
.addGap(176)
.addComponent(label))
.addGroup(gl_contentPane.createSequentialGroup()
.addGap(88)
.addGroup(gl_contentPane.createParallelGroup(Alignment.TRAILING)
.addGroup(Alignment.LEADING, gl_contentPane.createSequentialGroup()
.addComponent(label_2)
.addPreferredGap(ComponentPlacement.RELATED, 26, Short.MAX_VALUE))
.addGroup(gl_contentPane.createSequentialGroup()
.addGroup(gl_contentPane.createParallelGroup(Alignment.TRAILING)
.addComponent(label_3, GroupLayout.DEFAULT_SIZE, GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE)
.addComponent(label_1))
.addPreferredGap(ComponentPlacement.UNRELATED)))
.addGap(14)
.addGroup(gl_contentPane.createParallelGroup(Alignment.LEADING)
.addComponent(qxtxt)
.addComponent(btnNewButton)
.addComponent(usernametxt, GroupLayout.PREFERRED_SIZE, 184, GroupLayout.PREFERRED_SIZE)
.addComponent(pwdtxt))))
.addGroup(gl_contentPane.createSequentialGroup()
.addGap(63)
.addComponent(label_4)))
.addContainerGap(76, Short.MAX_VALUE))
);
gl_contentPane.setVerticalGroup(
gl_contentPane.createParallelGroup(Alignment.LEADING)
.addGroup(gl_contentPane.createSequentialGroup()
.addGap(47)
.addComponent(label)
.addPreferredGap(ComponentPlacement.RELATED)
.addGroup(gl_contentPane.createParallelGroup(Alignment.BASELINE)
.addComponent(label_1, GroupLayout.DEFAULT_SIZE, 95, Short.MAX_VALUE)
.addComponent(usernametxt, GroupLayout.PREFERRED_SIZE, 34, GroupLayout.PREFERRED_SIZE))
.addPreferredGap(ComponentPlacement.UNRELATED)
.addGroup(gl_contentPane.createParallelGroup(Alignment.BASELINE)
.addComponent(label_2, GroupLayout.DEFAULT_SIZE, GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE)
.addComponent(pwdtxt, GroupLayout.DEFAULT_SIZE, 32, Short.MAX_VALUE))
.addGap(32)
.addGroup(gl_contentPane.createParallelGroup(Alignment.BASELINE)
.addComponent(qxtxt, GroupLayout.PREFERRED_SIZE, 33, GroupLayout.PREFERRED_SIZE)
.addComponent(label_3, GroupLayout.PREFERRED_SIZE, 51, GroupLayout.PREFERRED_SIZE))
.addGap(26)
.addComponent(label_4)
.addGap(48)
.addComponent(btnNewButton)
.addGap(25))
);
contentPane.setLayout(gl_contentPane);
this.setLocationRelativeTo(null);
}
/*
* 注册
*/
protected void regactionPerformed(ActionEvent e) throws ClassNotFoundException, SQLException {
// TODO Auto-generated method stub
String name=this.usernametxt.getText();
String pwd=new String(this.pwdtxt.getPassword());
String id= this.qxtxt.getText();
int i=userdao.reg(name,pwd,id);
if(StringUtil.isEmpty(name)) {
JOptionPane.showMessageDialog(null, "用户名不能为空");
}
if(StringUtil.isEmpty(pwd)) {
JOptionPane.showMessageDialog(null, "密码不能为空");
}
if(StringUtil.isEmpty(id)) {
JOptionPane.showMessageDialog(null, "身份不能为空");
}
if(i>0) {
dispose();
new LoginFrm().setVisible(true);
}
else {
JOptionPane.showMessageDialog(null, "注册失败");
}
}
}
(10)房东信息登记界面
//房东信息登记界面
import java.awt.BorderLayout;
import java.awt.EventQueue;
import javax.swing.JFrame;
import javax.swing.JPanel;
import javax.swing.border.EmptyBorder;
import dao.UserDao;
import util.StringUtil;
import javax.swing.GroupLayout;
import javax.swing.GroupLayout.Alignment;
import javax.swing.JLabel;
import javax.swing.JOptionPane;
import java.awt.Font;
import javax.swing.JTextField;
import javax.swing.LayoutStyle.ComponentPlacement;
import javax.swing.WindowConstants;
import javax.swing.JButton;
import java.awt.event.ActionListener;
import java.sql.SQLException;
import java.awt.event.ActionEvent;
public class Reghost extends JFrame {
private JPanel contentPane;
private JTextField nametxt;
private JTextField addtxt;
private JTextField phtxt;
private UserDao userdao=new UserDao();
/**
* Launch the application.
*/
public static void main(String[] args) {
EventQueue.invokeLater(new Runnable() {
public void run() {
try {
Reghost frame = new Reghost();
frame.setVisible(true);
} catch (Exception e) {
e.printStackTrace();
}
}
});
}
/**
* Create the frame.
*/
public Reghost() {
setTitle("\u623F\u4E1C\u4FE1\u606F\u767B\u8BB0");
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setBounds(100, 100, 800, 730);
contentPane = new JPanel();
contentPane.setBorder(new EmptyBorder(5, 5, 5, 5));
setContentPane(contentPane);
JLabel label = new JLabel("\u623F\u4E1C\u4FE1\u606F\u767B\u8BB0");
label.setFont(new Font("宋体", Font.BOLD, 35));
JLabel label_1 = new JLabel("\u59D3\u540D");
label_1.setFont(new Font("宋体", Font.BOLD, 25));
JLabel label_2 = new JLabel("\u4F4F\u5740");
label_2.setFont(new Font("宋体", Font.BOLD, 25));
JLabel label_3 = new JLabel("\u8054\u7CFB\u7535\u8BDD");
label_3.setFont(new Font("宋体", Font.BOLD, 25));
nametxt = new JTextField();
nametxt.setColumns(10);
addtxt = new JTextField();
addtxt.setColumns(10);
phtxt = new JTextField();
phtxt.setColumns(10);
JButton button = new JButton("\u767B\u8BB0");
button.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
try {
reghostperformed(e);
} catch (ClassNotFoundException | SQLException e1) {
// TODO Auto-generated catch block
e1.printStackTrace();
}
}
});
button.setFont(new Font("宋体", Font.BOLD, 25));
GroupLayout gl_contentPane = new GroupLayout(contentPane);
gl_contentPane.setHorizontalGroup(
gl_contentPane.createParallelGroup(Alignment.LEADING)
.addGroup(gl_contentPane.createSequentialGroup()
.addGroup(gl_contentPane.createParallelGroup(Alignment.LEADING)
.addGroup(gl_contentPane.createSequentialGroup()
.addGap(241)
.addComponent(label))
.addGroup(gl_contentPane.createSequentialGroup()
.addContainerGap(163, Short.MAX_VALUE)
.addGroup(gl_contentPane.createParallelGroup(Alignment.TRAILING, false)
.addGroup(gl_contentPane.createSequentialGroup()
.addComponent(label_3)
.addGap(22))
.addGroup(gl_contentPane.createSequentialGroup()
.addGroup(gl_contentPane.createParallelGroup(Alignment.LEADING)
.addComponent(label_2)
.addComponent(label_1))
.addGap(52)))
.addGroup(gl_contentPane.createParallelGroup(Alignment.LEADING, false)
.addComponent(nametxt, GroupLayout.DEFAULT_SIZE, 209, Short.MAX_VALUE)
.addComponent(phtxt, GroupLayout.DEFAULT_SIZE, 209, Short.MAX_VALUE)
.addComponent(addtxt)))
.addGroup(gl_contentPane.createSequentialGroup()
.addGap(306)
.addComponent(button)))
.addContainerGap(274, Short.MAX_VALUE))
);
gl_contentPane.setVerticalGroup(
gl_contentPane.createParallelGroup(Alignment.LEADING)
.addGroup(gl_contentPane.createSequentialGroup()
.addGap(81)
.addGroup(gl_contentPane.createParallelGroup(Alignment.TRAILING)
.addGroup(gl_contentPane.createSequentialGroup()
.addComponent(label)
.addGap(73)
.addComponent(nametxt, GroupLayout.PREFERRED_SIZE, 31, GroupLayout.PREFERRED_SIZE))
.addComponent(label_1))
.addGap(56)
.addGroup(gl_contentPane.createParallelGroup(Alignment.LEADING, false)
.addComponent(addtxt)
.addComponent(label_2, GroupLayout.DEFAULT_SIZE, GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE))
.addGap(63)
.addGroup(gl_contentPane.createParallelGroup(Alignment.BASELINE)
.addComponent(phtxt, GroupLayout.PREFERRED_SIZE, 31, GroupLayout.PREFERRED_SIZE)
.addComponent(label_3))
.addGap(116)
.addComponent(button)
.addGap(102))
);
setDefaultCloseOperation(WindowConstants.HIDE_ON_CLOSE);
contentPane.setLayout(gl_contentPane);
this.setLocationRelativeTo(null);
}
protected void reghostperformed(ActionEvent e) throws ClassNotFoundException, SQLException {
String name=this.nametxt.getText();
String address=this.addtxt.getText();
String phone=this.phtxt.getText();
int i=userdao.reghost(name,address,phone);
if(StringUtil.isEmpty(name)) {
JOptionPane.showMessageDialog(null, "姓名不能为空");
}
if(StringUtil.isEmpty(address)) {
JOptionPane.showMessageDialog(null, "地址不能为空");
}
if(StringUtil.isEmpty(phone)) {
JOptionPane.showMessageDialog(null, "电话不能为空");
}
if(i>0) {
dispose();
}
else {
JOptionPane.showMessageDialog(null, "登记失败");
}
}
}
(11)房屋信息登记界面
//房屋信息登记界面
import java.awt.BorderLayout;
import java.awt.EventQueue;
import javax.swing.JFrame;
import javax.swing.JPanel;
import javax.swing.border.EmptyBorder;
import dao.UserDao;
import javax.swing.GroupLayout;
import javax.swing.GroupLayout.Alignment;
import javax.swing.JLabel;
import javax.swing.JOptionPane;
import java.awt.Font;
import javax.swing.JTextField;
import javax.swing.JButton;
import javax.swing.LayoutStyle.ComponentPlacement;
import javax.swing.WindowConstants;
import java.awt.event.ActionListener;
import java.sql.SQLException;
import java.awt.event.ActionEvent;
public class Reghouse extends JFrame {
private JPanel contentPane;
private JTextField addtxt;
private JTextField typetxt;
private JTextField maxtxt;
private JTextField renttxt;
private JTextField statustxt;
private UserDao userdao=new UserDao();
/**
* Launch the application.
*/
public static void main(String[] args) {
EventQueue.invokeLater(new Runnable() {
public void run() {
try {
Reghouse frame = new Reghouse();
frame.setVisible(true);
} catch (Exception e) {
e.printStackTrace();
}
}
});
}
/**
* Create the frame.
*/
public Reghouse() {
setTitle("\u623F\u5C4B\u767B\u8BB0");
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setBounds(100, 100, 800, 730);
contentPane = new JPanel();
contentPane.setBorder(new EmptyBorder(5, 5, 5, 5));
setContentPane(contentPane);
JLabel label = new JLabel("\u623F\u5C4B\u4FE1\u606F\u767B\u8BB0");
label.setFont(new Font("宋体", Font.BOLD, 35));
JLabel label_1 = new JLabel("\u5730\u5740");
label_1.setFont(new Font("宋体", Font.BOLD, 25));
JLabel label_2 = new JLabel("\u623F\u578B");
label_2.setFont(new Font("宋体", Font.BOLD, 25));
JLabel label_3 = new JLabel("\u6700\u5927\u623F\u5BA2\u6570");
label_3.setFont(new Font("宋体", Font.BOLD, 25));
JLabel label_4 = new JLabel("\u79DF\u91D1");
label_4.setFont(new Font("宋体", Font.BOLD, 25));
JLabel label_5 = new JLabel("\u623F\u5C4B\u72B6\u6001");
label_5.setFont(new Font("宋体", Font.BOLD, 25));
addtxt = new JTextField();
addtxt.setColumns(10);
typetxt = new JTextField();
typetxt.setColumns(10);
maxtxt = new JTextField();
maxtxt.setColumns(10);
renttxt = new JTextField();
renttxt.setColumns(10);
statustxt = new JTextField();
statustxt.setColumns(10);
JButton button = new JButton("\u767B\u8BB0");
button.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
try {
reghouseperformed(e);
JOptionPane.showMessageDialog(null, "请及时缴纳手续费50元");
} catch (ClassNotFoundException | SQLException e1) {
// TODO Auto-generated catch block
e1.printStackTrace();
}
}
});
button.setFont(new Font("宋体", Font.BOLD, 25));
GroupLayout gl_contentPane = new GroupLayout(contentPane);
gl_contentPane.setHorizontalGroup(
gl_contentPane.createParallelGroup(Alignment.LEADING)
.addGroup(gl_contentPane.createSequentialGroup()
.addGroup(gl_contentPane.createParallelGroup(Alignment.LEADING)
.addGroup(gl_contentPane.createSequentialGroup()
.addGap(243)
.addComponent(label))
.addGroup(gl_contentPane.createSequentialGroup()
.addGroup(gl_contentPane.createParallelGroup(Alignment.LEADING)
.addGroup(gl_contentPane.createSequentialGroup()
.addGap(66)
.addGroup(gl_contentPane.createParallelGroup(Alignment.TRAILING)
.addComponent(label_2)
.addComponent(label_1)))
.addGroup(gl_contentPane.createSequentialGroup()
.addGap(28)
.addComponent(label_3))
.addGroup(gl_contentPane.createSequentialGroup()
.addGap(72)
.addComponent(label_4))
.addGroup(gl_contentPane.createSequentialGroup()
.addGap(46)
.addComponent(label_5)))
.addGap(67)
.addGroup(gl_contentPane.createParallelGroup(Alignment.LEADING, false)
.addComponent(addtxt)
.addComponent(statustxt, GroupLayout.DEFAULT_SIZE, 305, Short.MAX_VALUE)
.addComponent(typetxt, GroupLayout.DEFAULT_SIZE, 305, Short.MAX_VALUE)
.addComponent(maxtxt, GroupLayout.DEFAULT_SIZE, 305, Short.MAX_VALUE)
.addComponent(renttxt, GroupLayout.DEFAULT_SIZE, 305, Short.MAX_VALUE)))
.addGroup(gl_contentPane.createSequentialGroup()
.addGap(297)
.addComponent(button)))
.addContainerGap(242, Short.MAX_VALUE))
);
gl_contentPane.setVerticalGroup(
gl_contentPane.createParallelGroup(Alignment.LEADING)
.addGroup(gl_contentPane.createSequentialGroup()
.addGap(63)
.addComponent(label)
.addGap(70)
.addGroup(gl_contentPane.createParallelGroup(Alignment.BASELINE)
.addComponent(label_1)
.addComponent(addtxt, GroupLayout.DEFAULT_SIZE, 31, Short.MAX_VALUE))
.addGap(47)
.addGroup(gl_contentPane.createParallelGroup(Alignment.BASELINE)
.addComponent(label_2)
.addComponent(typetxt, GroupLayout.PREFERRED_SIZE, 30, GroupLayout.PREFERRED_SIZE))
.addGap(53)
.addGroup(gl_contentPane.createParallelGroup(Alignment.LEADING)
.addComponent(label_3)
.addComponent(maxtxt, GroupLayout.PREFERRED_SIZE, 30, GroupLayout.PREFERRED_SIZE))
.addGap(46)
.addGroup(gl_contentPane.createParallelGroup(Alignment.BASELINE)
.addComponent(label_4)
.addComponent(renttxt, GroupLayout.PREFERRED_SIZE, 30, GroupLayout.PREFERRED_SIZE))
.addGap(44)
.addGroup(gl_contentPane.createParallelGroup(Alignment.BASELINE)
.addComponent(label_5)
.addComponent(statustxt, GroupLayout.PREFERRED_SIZE, 30, GroupLayout.PREFERRED_SIZE))
.addPreferredGap(ComponentPlacement.RELATED, 65, Short.MAX_VALUE)
.addComponent(button)
.addGap(50))
);this.setLocationRelativeTo(null);
contentPane.setLayout(gl_contentPane);
setDefaultCloseOperation(WindowConstants.HIDE_ON_CLOSE);
}
protected void reghouseperformed(ActionEvent e) throws ClassNotFoundException, SQLException {
// TODO Auto-generated method stub
String address=this.addtxt.getText();
String type=this.typetxt.getText();
String max=this.maxtxt.getText();
String rent=this.renttxt.getText();
String status=this.statustxt.getText();
int i=userdao.reghouse(address,type,max,rent,status);
if(i>0) {
dispose();
}
}
}
(12)租户信息登记界面
//租户信息登记界面
import java.awt.BorderLayout;
import java.awt.EventQueue;
import javax.swing.JFrame;
import javax.swing.JPanel;
import javax.swing.border.EmptyBorder;
import dao.UserDao;
import javax.swing.GroupLayout;
import javax.swing.GroupLayout.Alignment;
import javax.swing.JLabel;
import java.awt.Font;
import javax.swing.LayoutStyle.ComponentPlacement;
import javax.swing.JTextField;
import javax.swing.WindowConstants;
import javax.swing.JButton;
import java.awt.event.ActionListener;
import java.sql.SQLException;
import java.awt.event.ActionEvent;
public class Regten extends JFrame {
private JPanel contentPane;
private JTextField nametxt;
private JTextField addtxt;
private JTextField gentxt;
private JTextField phtxt;
private JTextField datetxt;
private UserDao userdao=new UserDao();
/**
* Launch the application.
*/
public static void main(String[] args) {
EventQueue.invokeLater(new Runnable() {
public void run() {
try {
Regten frame = new Regten();
frame.setVisible(true);
} catch (Exception e) {
e.printStackTrace();
}
}
});
}
/**
* Create the frame.
*/
public Regten() {
setTitle("\u4FE1\u606F\u767B\u8BB0");
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setBounds(100, 100, 800, 730);
contentPane = new JPanel();
contentPane.setBorder(new EmptyBorder(5, 5, 5, 5));
setContentPane(contentPane);
JLabel label = new JLabel("\u79DF\u6237\u4FE1\u606F\u767B\u8BB0");
label.setFont(new Font("宋体", Font.BOLD, 35));
JLabel label_1 = new JLabel("\u59D3\u540D");
label_1.setFont(new Font("宋体", Font.BOLD, 25));
JLabel label_2 = new JLabel("\u4F4F\u5740");
label_2.setFont(new Font("宋体", Font.BOLD, 25));
JLabel label_3 = new JLabel("\u51FA\u751F\u5E74\u6708");
label_3.setFont(new Font("宋体", Font.BOLD, 25));
JLabel label_4 = new JLabel("\u6027\u522B");
label_4.setFont(new Font("宋体", Font.BOLD, 25));
JLabel label_5 = new JLabel("\u7535\u8BDD");
label_5.setFont(new Font("宋体", Font.BOLD, 25));
nametxt = new JTextField();
nametxt.setColumns(10);
addtxt = new JTextField();
addtxt.setColumns(10);
gentxt = new JTextField();
gentxt.setColumns(10);
phtxt = new JTextField();
phtxt.setColumns(10);
datetxt = new JTextField();
datetxt.setColumns(10);
JButton button = new JButton("\u767B\u8BB0");
button.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
try {
regtenatperformed(e);
} catch (ClassNotFoundException | SQLException e1) {
// TODO Auto-generated catch block
e1.printStackTrace();
}
}
});
button.setFont(new Font("宋体", Font.BOLD, 25));
GroupLayout gl_contentPane = new GroupLayout(contentPane);
gl_contentPane.setHorizontalGroup(
gl_contentPane.createParallelGroup(Alignment.LEADING)
.addGroup(gl_contentPane.createSequentialGroup()
.addGroup(gl_contentPane.createParallelGroup(Alignment.LEADING)
.addGroup(gl_contentPane.createSequentialGroup()
.addGap(250)
.addComponent(label))
.addGroup(gl_contentPane.createSequentialGroup()
.addGroup(gl_contentPane.createParallelGroup(Alignment.LEADING)
.addGroup(gl_contentPane.createSequentialGroup()
.addGap(88)
.addGroup(gl_contentPane.createParallelGroup(Alignment.LEADING)
.addComponent(label_1)
.addComponent(label_2)
.addComponent(label_4)
.addComponent(label_5)))
.addGroup(gl_contentPane.createSequentialGroup()
.addGap(66)
.addComponent(label_3)))
.addGap(48)
.addGroup(gl_contentPane.createParallelGroup(Alignment.LEADING)
.addComponent(datetxt, GroupLayout.PREFERRED_SIZE, 315, GroupLayout.PREFERRED_SIZE)
.addComponent(phtxt, GroupLayout.PREFERRED_SIZE, 315, GroupLayout.PREFERRED_SIZE)
.addComponent(gentxt, GroupLayout.PREFERRED_SIZE, 315, GroupLayout.PREFERRED_SIZE)
.addComponent(addtxt, GroupLayout.PREFERRED_SIZE, 315, GroupLayout.PREFERRED_SIZE)
.addComponent(nametxt, GroupLayout.PREFERRED_SIZE, 315, GroupLayout.PREFERRED_SIZE)))
.addGroup(gl_contentPane.createSequentialGroup()
.addGap(310)
.addComponent(button)))
.addContainerGap(239, Short.MAX_VALUE))
);
gl_contentPane.setVerticalGroup(
gl_contentPane.createParallelGroup(Alignment.LEADING)
.addGroup(gl_contentPane.createSequentialGroup()
.addGap(91)
.addComponent(label)
.addGap(75)
.addGroup(gl_contentPane.createParallelGroup(Alignment.LEADING, false)
.addComponent(nametxt)
.addComponent(label_1, GroupLayout.DEFAULT_SIZE, GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE))
.addGap(27)
.addGroup(gl_contentPane.createParallelGroup(Alignment.LEADING)
.addComponent(label_2)
.addComponent(addtxt, GroupLayout.PREFERRED_SIZE, 30, GroupLayout.PREFERRED_SIZE))
.addGap(36)
.addGroup(gl_contentPane.createParallelGroup(Alignment.LEADING)
.addComponent(label_4)
.addComponent(gentxt, GroupLayout.PREFERRED_SIZE, 30, GroupLayout.PREFERRED_SIZE))
.addGap(32)
.addGroup(gl_contentPane.createParallelGroup(Alignment.LEADING)
.addGroup(gl_contentPane.createSequentialGroup()
.addComponent(label_5)
.addGap(27)
.addGroup(gl_contentPane.createParallelGroup(Alignment.BASELINE)
.addComponent(label_3)
.addComponent(datetxt, GroupLayout.PREFERRED_SIZE, 30, GroupLayout.PREFERRED_SIZE)))
.addComponent(phtxt, GroupLayout.PREFERRED_SIZE, 30, GroupLayout.PREFERRED_SIZE))
.addPreferredGap(ComponentPlacement.RELATED, 63, Short.MAX_VALUE)
.addComponent(button)
.addGap(88))
);setDefaultCloseOperation(WindowConstants.HIDE_ON_CLOSE);
contentPane.setLayout(gl_contentPane);this.setLocationRelativeTo(null);
}
protected void regtenatperformed(ActionEvent e) throws ClassNotFoundException, SQLException {
// TODO Auto-generated method stub
String name=this.nametxt.getText();
String address=this.addtxt.getText();
String phone=this.phtxt.getText();
String gen=this.gentxt.getText();
String date=this.datetxt.getText();
int i=userdao.regten(name,address,gen,phone,date);
if(i>0) {
dispose();
}
}
}
(13)租房表
//租房表
import java.awt.EventQueue;
import java.sql.SQLException;
import java.util.List;
import java.util.Vector;
import javax.swing.JInternalFrame;
import javax.swing.JScrollPane;
import java.awt.BorderLayout;
import javax.swing.JTable;
import javax.swing.table.DefaultTableModel;
import dao.UserDao;
import model.Rentmodel;
import model.Tenant;
public class Rent extends JInternalFrame {
private JTable table;
private UserDao userDao = new UserDao();
/**
* Launch the application.
*/
public static void main(String[] args) {
EventQueue.invokeLater(new Runnable() {
public void run() {
try {
Rent frame = new Rent();
frame.setVisible(true);
} catch (Exception e) {
e.printStackTrace();
}
}
});
}
/**
* Create the frame.
* @throws SQLException
* @throws ClassNotFoundException
*/
public Rent() throws ClassNotFoundException, SQLException {
setTitle("\u79DF\u623F\u8BB0\u5F55");
setMaximizable(true);
setIconifiable(true);
setClosable(true);
setBounds(100, 100, 801, 729);
JScrollPane scrollPane = new JScrollPane();
getContentPane().add(scrollPane, BorderLayout.CENTER);
table = new JTable();
table.setModel(new DefaultTableModel(
new Object[][] {
},
new String[] {
"\u79DF\u6237", "\u5730\u5740"
}
));
scrollPane.setViewportView(table);
fillTable();
}
public void fillTable() throws ClassNotFoundException, SQLException {
DefaultTableModel dtm =(DefaultTableModel)table.getModel();
dtm.setRowCount(0);
//查询数据
List<Rentmodel> t=userDao.listrent();
for(Rentmodel rent:t) {
Vector v=new Vector<>();
v.add(rent.getName());
v.add(rent.getAddress());
dtm.addRow(v);
}
}
}
(14)租房表
//看房表
import java.awt.EventQueue;
import java.sql.SQLException;
import java.util.List;
import java.util.Vector;
import javax.swing.JInternalFrame;
import javax.swing.JScrollPane;
import java.awt.BorderLayout;
import javax.swing.JTable;
import javax.swing.table.DefaultTableModel;
import dao.UserDao;
import model.Seemodel;
import model.Tenant;
public class See extends JInternalFrame {
private JTable table;
private UserDao userDao = new UserDao();
/**
* Launch the application.
*/
public static void main(String[] args) {
EventQueue.invokeLater(new Runnable() {
public void run() {
try {
See frame = new See();
frame.setVisible(true);
} catch (Exception e) {
e.printStackTrace();
}
}
});
}
/**
* Create the frame.
* @throws SQLException
* @throws ClassNotFoundException
*/
public See() throws ClassNotFoundException, SQLException {
setMaximizable(true);
setIconifiable(true);
setClosable(true);
setTitle("\u770B\u623F\u8BB0\u5F55");
setBounds(100, 100, 800, 730);
JScrollPane scrollPane = new JScrollPane();
getContentPane().add(scrollPane, BorderLayout.CENTER);
table = new JTable();
table.setModel(new DefaultTableModel(
new Object[][] {
},
new String[] {
"\u79DF\u6237", "\u5730\u5740"
}
));
scrollPane.setViewportView(table);
fillTable();
}
public void fillTable() throws ClassNotFoundException, SQLException {
DefaultTableModel dtm =(DefaultTableModel)table.getModel();
dtm.setRowCount(0);
//查询数据
List<Seemodel> s=userDao.listsee();
for(Seemodel see:s) {
Vector v=new Vector<>();
v.add(see.getName());
v.add(see.getAddress());
dtm.addRow(v);
}
}
}
(15)提出看房请求界面
//提出看房请求
import java.awt.BorderLayout;
import java.awt.EventQueue;
import javax.swing.JFrame;
import javax.swing.JPanel;
import javax.swing.border.EmptyBorder;
import dao.UserDao;
import javax.swing.GroupLayout;
import javax.swing.GroupLayout.Alignment;
import javax.swing.JLabel;
import javax.swing.JOptionPane;
import java.awt.Font;
import javax.swing.JTextField;
import javax.swing.WindowConstants;
import javax.swing.JButton;
import javax.swing.LayoutStyle.ComponentPlacement;
import java.awt.event.ActionListener;
import java.sql.SQLException;
import java.awt.event.ActionEvent;
public class Seehouse extends JFrame {
private JPanel contentPane;
private JTextField nametxt;
private JTextField addtxt;
private UserDao userdao=new UserDao();
/**
* Launch the application.
*/
public static void main(String[] args) {
EventQueue.invokeLater(new Runnable() {
public void run() {
try {
Seehouse frame = new Seehouse();
frame.setVisible(true);
} catch (Exception e) {
e.printStackTrace();
}
}
});
}
/**
* Create the frame.
*/
public Seehouse() {
setTitle("\u770B\u623F\u8BF7\u6C42");
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setBounds(100, 100, 800, 730);
contentPane = new JPanel();
contentPane.setBorder(new EmptyBorder(5, 5, 5, 5));
setContentPane(contentPane);
JLabel label = new JLabel("\u770B\u623F\u8BF7\u6C42");
label.setFont(new Font("宋体", Font.BOLD, 35));
JLabel label_1 = new JLabel("\u59D3\u540D");
label_1.setFont(new Font("宋体", Font.BOLD, 25));
JLabel label_2 = new JLabel("\u6240\u770B\u623F\u5730\u5740");
label_2.setFont(new Font("宋体", Font.BOLD, 25));
nametxt = new JTextField();
nametxt.setColumns(10);
addtxt = new JTextField();
addtxt.setColumns(10);
JButton button = new JButton("\u8BF7\u6C42");
button.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
try {
seeperformed(e);
JOptionPane.showMessageDialog(null,"请及时缴纳手续费,具体费用在房屋信息中查看");
} catch (ClassNotFoundException | SQLException e1) {
// TODO Auto-generated catch block
e1.printStackTrace();
}
}
});
button.setFont(new Font("宋体", Font.BOLD, 25));
GroupLayout gl_contentPane = new GroupLayout(contentPane);
gl_contentPane.setHorizontalGroup(
gl_contentPane.createParallelGroup(Alignment.LEADING)
.addGroup(gl_contentPane.createSequentialGroup()
.addGroup(gl_contentPane.createParallelGroup(Alignment.LEADING)
.addGroup(gl_contentPane.createSequentialGroup()
.addGap(279)
.addComponent(label))
.addGroup(gl_contentPane.createSequentialGroup()
.addGroup(gl_contentPane.createParallelGroup(Alignment.LEADING)
.addGroup(gl_contentPane.createSequentialGroup()
.addGap(115)
.addComponent(label_1))
.addGroup(gl_contentPane.createSequentialGroup()
.addGap(72)
.addComponent(label_2)))
.addGap(59)
.addGroup(gl_contentPane.createParallelGroup(Alignment.LEADING)
.addComponent(addtxt, GroupLayout.PREFERRED_SIZE, 227, GroupLayout.PREFERRED_SIZE)
.addComponent(nametxt, GroupLayout.PREFERRED_SIZE, 227, GroupLayout.PREFERRED_SIZE)))
.addGroup(gl_contentPane.createSequentialGroup()
.addGap(327)
.addComponent(button)))
.addContainerGap(284, Short.MAX_VALUE))
);
gl_contentPane.setVerticalGroup(
gl_contentPane.createParallelGroup(Alignment.LEADING)
.addGroup(gl_contentPane.createSequentialGroup()
.addGap(74)
.addComponent(label)
.addGap(110)
.addGroup(gl_contentPane.createParallelGroup(Alignment.BASELINE)
.addComponent(label_1)
.addComponent(nametxt, GroupLayout.PREFERRED_SIZE, 31, GroupLayout.PREFERRED_SIZE))
.addGap(92)
.addGroup(gl_contentPane.createParallelGroup(Alignment.TRAILING)
.addComponent(label_2)
.addComponent(addtxt, GroupLayout.PREFERRED_SIZE, 31, GroupLayout.PREFERRED_SIZE))
.addPreferredGap(ComponentPlacement.RELATED, 140, Short.MAX_VALUE)
.addComponent(button)
.addGap(111))
);setDefaultCloseOperation(WindowConstants.HIDE_ON_CLOSE);
contentPane.setLayout(gl_contentPane);this.setLocationRelativeTo(null);
}
protected void seeperformed(ActionEvent e) throws ClassNotFoundException, SQLException {
String name=this.nametxt.getText();
String address=this.addtxt.getText();
int i=userdao.regtant(name,address);
if(i>0) {
dispose();
}
}
}
(16)租户表
//租户表
import java.awt.EventQueue;
import java.sql.SQLException;
import java.util.List;
import java.util.Vector;
import javax.swing.JInternalFrame;
import javax.swing.JScrollPane;
import java.awt.BorderLayout;
import javax.swing.JTable;
import javax.swing.table.DefaultTableModel;
import dao.UserDao;
import model.Houses;
import model.Tenant;
public class Ten extends JInternalFrame {
private JTable table;
private UserDao userDao = new UserDao();
/**
* Launch the application.
*/
public static void main(String[] args) {
EventQueue.invokeLater(new Runnable() {
public void run() {
try {
Ten frame = new Ten();
frame.setVisible(true);
} catch (Exception e) {
e.printStackTrace();
}
}
});
}
/**
* Create the frame.
* @throws SQLException
* @throws ClassNotFoundException
*/
public Ten() throws ClassNotFoundException, SQLException {
setMaximizable(true);
setIconifiable(true);
setClosable(true);
setTitle("\u79DF\u6237\u4FE1\u606F");
setBounds(100, 100, 800, 730);
JScrollPane scrollPane = new JScrollPane();
getContentPane().add(scrollPane, BorderLayout.CENTER);
table = new JTable();
table.setModel(new DefaultTableModel(
new Object[][] {
},
new String[] {
"\u59D3\u540D", "\u5730\u5740", "\u6027\u522B", "\u7535\u8BDD", "\u51FA\u751F\u65E5\u671F"
}
));
scrollPane.setViewportView(table);
fillTable();
}
public void fillTable() throws ClassNotFoundException, SQLException {
DefaultTableModel dtm =(DefaultTableModel)table.getModel();
dtm.setRowCount(0);
//查询数据
List<Tenant> t=userDao.listenant();
for(Tenant tenant:t) {
Vector v=new Vector<>();
v.add(tenant.getName());
v.add(tenant.getAddress());
v.add(tenant.getGender());
v.add(tenant.getPhone());
v.add(tenant.getDate_of_birth());
dtm.addRow(v);
}
}
}
|