字节输入流 InputStream,该抽象类是所有类字节输入流的超类 字节输出流 OutputStream,该抽象类是所有类字节输出流的超类
1.文件输入输出流
1.1 文件输入流 FileInputStream
import org.junit.jupiter.api.Test;
import java.io.FileInputStream;
import java.io.IOException;
public class FileInputStream_ {
public static void main(String[] args) {
}
@Test
public void readFile01() {
String filePath = "D:\\test1.txt";
int readData = 0;
FileInputStream fileInputStream = null;
try {
fileInputStream = new FileInputStream(filePath);
while ((readData = fileInputStream.read()) != -1) {
System.out.print((char) readData);
}
} catch (IOException e) {
e.printStackTrace();
} finally {
try {
fileInputStream.close();
} catch (Exception e) {
e.printStackTrace();
}
}
}
@Test
public void readFile02() {
String filePath = "D:\\test1.txt";
int readLen = 0;
byte[] buf = new byte[8];
FileInputStream fileInputStream = null;
try {
fileInputStream = new FileInputStream(filePath);
while ((readLen = fileInputStream.read(buf)) != -1) {
System.out.print(new String(buf, 0, readLen));
}
} catch (IOException e) {
e.printStackTrace();
} finally {
try {
fileInputStream.close();
} catch (Exception e) {
e.printStackTrace();
}
}
}
}
1.2 文件输出流 FileOutputStream
import org.junit.jupiter.api.Test;
import java.io.FileOutputStream;
import java.io.IOException;
public class FileOutputStream_ {
public static void main(String[] args) {
}
@Test
public void writeFile(){
String filePath = "D:\\yy.txt";
FileOutputStream fileOutputStream = null;
try {
fileOutputStream = new FileOutputStream(filePath,true);
fileOutputStream.write("hi,java".getBytes(),0,2);
} catch (IOException e) {
e.printStackTrace();
}finally {
try {
fileOutputStream.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
1.3 文件输入输出流的应用
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
public class FileCopy {
public static void main(String[] args) {
String srcFilePath = "D:\\1.png";
String destFilePath = "D:\\test\\1.png";
FileInputStream fileInputStream = null;
FileOutputStream fileOutputStream = null;
try {
fileInputStream = new FileInputStream(srcFilePath);
fileOutputStream = new FileOutputStream(destFilePath);
byte[] buf = new byte[1024];
int readLen = 0;
while((readLen = fileInputStream.read(buf)) != -1){
fileOutputStream.write(buf, 0, readLen);
}
System.out.println("拷贝成功");
}catch (IOException e) {
e.printStackTrace();
} finally {
try {
if(fileInputStream != null){
fileInputStream.close();
}
if(fileOutputStream != null){
fileOutputStream.close();
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
2.缓冲字节输入输出流
缓冲字节输入流 BufferedInputStream,在创建BufferedInputStream时,会创建一个内部缓冲区数组
缓冲字节输出流 BufferedOutputStream,实现缓冲的输出流,可以将多个字节写入底层输出流中,不必对每次字节写入调用底层系统
字节流可以操作二进制文件,字符流不能操作二进制文件
应用
import java.io.*;
public class BufferedCopy__ {
public static void main(String[] args) {
String srcFilePath = "D:\\IMG_7489.JPG";
String destFilePath = "D:\\1\\h.JPG";
BufferedInputStream bis = null;
BufferedOutputStream bos = null;
try {
bis = new BufferedInputStream(new FileInputStream(srcFilePath));
bos = new BufferedOutputStream(new FileOutputStream(destFilePath));
byte[] buff = new byte[1024];
int readLen = 0;
while((readLen = bis.read(buff)) != -1){
bos.write(buff, 0, readLen);
}
} catch (IOException e) {
e.printStackTrace();
} finally {
try {
if(bis != null){
bis.close();
}
if(bos != null){
bos.close();
}
} catch (IOException e) {
e.printStackTrace();
}
}
System.out.println("拷贝成功");
}
}
3.序列化(ObjectOutputStream)与反序列化(ObjectInputStream)
1.仅将程序(内存)中的数据写入至文件(磁盘),保存的是值 2.将程序(内存)中的保存值和数据类型写入至文件(磁盘),称作序列化 3.将保存在文件的数据(值和数据类型)恢复至程序,称作反序列化 4.若某个对象支持序列化机制,其类必须是可序列化的,需要是实现两个接口之一(Serializable(标记接口,没有方法)、Externalizable(需要是实现方法))
3.1 对象字节输出流 ObjectOutputStream(序列化)
创建一个Person类
import java.io.Serializable;
public class Person implements Serializable {
private String name;
private int age;
private transient String nation;
private static String city;
private Hobby hobby = new Hobby();
private static final long serialVersionUID = 1L;
public Person(String name, int age, String nation, String city) {
this.name = name;
this.age = age;
this.nation = nation;
this.city = city;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
public String getNation() {
return nation;
}
public void setNation(String nation) {
this.nation = nation;
}
public static String getCity() {
return city;
}
public static void setCity(String city) {
Person.city = city;
}
@Override
public String toString() {
return "Person{" +
"name='" + name + '\'' +
", age=" + age +
", nation='" + nation + '\'' +
'}' + city + hobby;
}
}
实现序列化
import com.yl.IO.Person;
import java.io.FileOutputStream;
import java.io.ObjectOutputStream;
public class ObjectOutputStream_ {
public static void main(String[] args) throws Exception {
String filePath = "D:\\data.dat";
ObjectOutputStream oos = new ObjectOutputStream(new FileOutputStream(filePath));
oos.writeInt(10);
oos.writeBoolean(true);
oos.writeChar('q');
oos.writeDouble(3.14159);
oos.writeUTF("杨杨杨");
oos.writeObject(new Person("架构师", 28, "china", "beijing"));
oos.close();
System.out.println("序列化成功");
}
}

3.2 对象字节输入流 ObjectInputStream(反序列化)
import com.yl.IO.Person;
import java.io.FileInputStream;
import java.io.IOException;
import java.io.ObjectInputStream;
public class ObjectInputStream_ {
public static void main(String[] args) throws IOException, ClassNotFoundException {
String filePath = "D:\\data.dat";
ObjectInputStream ois = new ObjectInputStream(new FileInputStream(filePath));
System.out.println(ois.readInt());
System.out.println(ois.readBoolean());
System.out.println(ois.readChar());
System.out.println(ois.readDouble());
System.out.println(ois.readUTF());
Object person = ois.readObject();
System.out.println("运行类型:" + person.getClass());
System.out.println("对象信息:" + person);
Person person2 = (Person)person;
System.out.println(person2.getName());
ois.close();
}
}
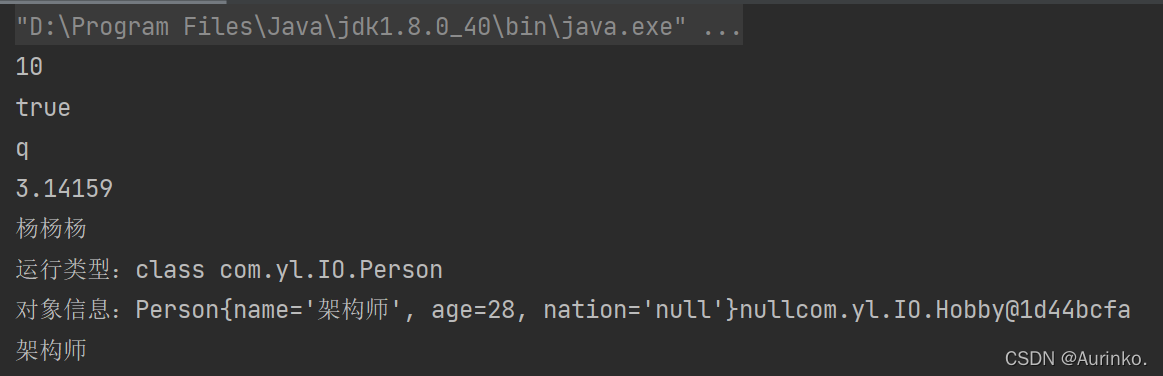
小结 1.读写顺序一致 2.要求实现序列化\反序列化对象,需要实现Serializablea 3.序列化的类中添加序列化版本号 private static final long serialVersionUID = 1L 可以提高版本兼容性 4.序列化对象时,默认将其所有属性都序列化,除static和transient修饰的成员 5.序列化对象时,要求其属性的类型也要是实现序列化接口 6.序列化具备可继承性,若某类已实现序列化,则其所有子类也默认实现了序列化
|