业务需求:编辑时,对主表中数据的修改,以及子数据可以进行删除,修改,或者新增的操作 前端(主要展示子表):可以点击新增,也可以在原数据上进行编辑,也可以删除该条数据再新增 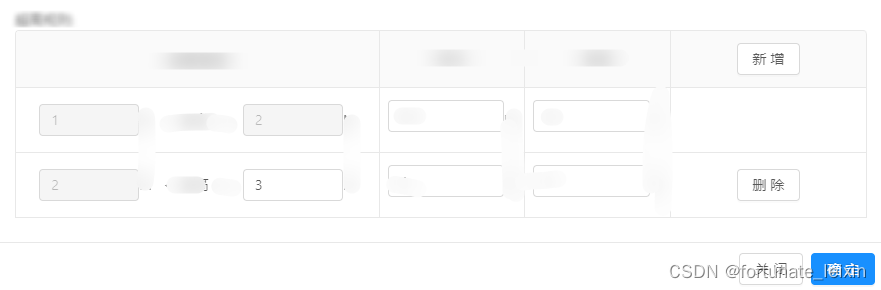
前端传值(主要展示子表): 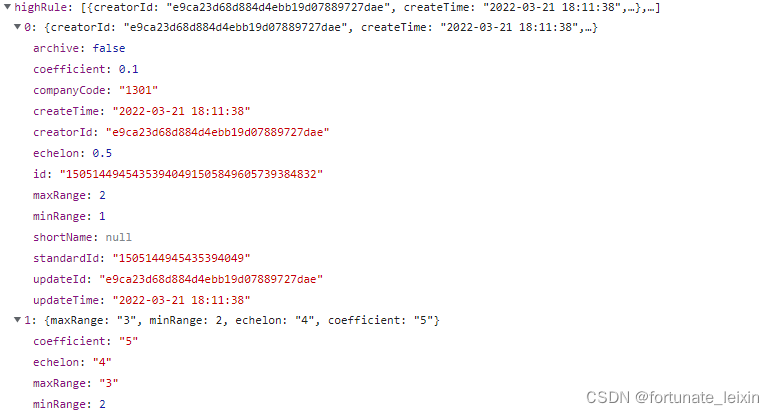 higtRule里面就是子数据,没有id的则是点击新增后新增的数据,所以既有新增操作,又有编辑操作 后台处理(主要对子数据): 思路: 1:首先查询全部子数据 2:创建一个空集合newIds,用于存放未删除的子数据的id,遍历前端传递过来的子数据,将子数据的id存放在newIds集合中 3:过滤数据也就是用全部的数据去过滤newIds的数据,如果全部的数据中的数据和newIds中的数据不一致,则就是要删除的子数据,使用Java8的stream流处理,该方法返回的就是要删除的数据的集合 4:创建一个空集合deleteIds,用于存放要删除的子数据的id,遍历deleteList,将id存放在deleteIds中 最后执行删除操作
controller:
/**
* 面积户表-收费标准-编辑
*
* @param artbFeeStandardVo
* @return
*/
@AutoLog(value = "artb_fee_standard-编辑")
@ApiOperation(value="artb_fee_standard-编辑", notes="artb_fee_standard-编辑")
@PutMapping(value = "/edit")
public Result<?> edit(@RequestBody ArtbStandardVo artbFeeStandardVo) {
return artbFeeStandardService.editArtbFeeStandard(artbFeeStandardVo);
}
service层
Result<?> editArtbFeeStandard(ArtbStandardVo artbFeeStandardVo);
实现类(主要是对子数据的处理):
@Override
@Transactional
public Result<?> editArtbFeeStandard(ArtbStandardVo artbFeeStandardVo) {
// 更新子数据
// 查询全部超高规则
QueryWrapper<ArtbFeeHighRule> oldChildWrapper = new QueryWrapper<>();
oldChildWrapper.eq("standard_id", feeStandard.getId());
List<ArtbFeeHighRule> highRules = highRuleMapper.selectList(oldChildWrapper);
// 存储前端传递过来未删除的id
List<String> newIds = new ArrayList<>();
for (ArtbFeeHighRule rule : artbFeeStandardVo.getHighRuleList()) {
newIds.add(rule.getId());
}
// 筛选出要删除的超高数据,进行批量删除
List<ArtbFeeHighRule> deleteList = highRules.stream().filter(item -> !newIds.contains(item.getId())).collect(Collectors.toList());
// 存放要删除的id
List<String> deleteIds = new ArrayList<>();
for (ArtbFeeHighRule rule : deleteList) {
deleteIds.add(rule.getId());
}
if (deleteIds.size() > 0) {
highRuleMapper.deleteBatchById(deleteIds);
}
if (!CollectionUtils.isEmpty(artbFeeStandardVo.getHighRuleList())) {
List<ArtbFeeHighRule> highList = new ArrayList<>();
List<ArtbFeeHighRuleHistory> highHistoryList = new ArrayList<>();
artbFeeStandardVo.getHighRuleList().forEach(item -> {
item.setInsertBaseColumnNoCompanyCode();
if (StringUtils.isEmpty(item.getId())) {
// 说明是新增
item.setId(IdUtil.getCombineId(feeStandard.getId()));
item.setStandardId(feeStandard.getId());
item.setCompanyCode(feeStandard.getCompanyCode());
}
highList.add(item);
// 更新超高历史表
//操作轨迹
ArtbFeeHighRuleHistory highRuleHistory = new ArtbFeeHighRuleHistory();
BeanUtils.copyProperties(item, highRuleHistory);
highRuleHistory.setStandardId(feeStandardHistory.getId());
highRuleHistory.setCompanyCode(feeStandardHistory.getCompanyCode());
highRuleHistory.setInsertBaseColumnNoCompanyCode();
highRuleHistory.setId(IdUtil.getCombineId(highRuleHistory.getCompanyCode()));
highHistoryList.add(highRuleHistory);
});
// 超过规则做批量新增或者编辑
highService.saveOrUpdateBatch(highList);
// 超过规则历史表做新增操作,记录具体操作数据
highHistoryService.saveBatch(highHistoryList);
}
}
mapper中的删除
/**
* 批量删除超高数据
* @param deleteIds
* @return
*/
int deleteBatchById(List<String> deleteIds);
xml中
<!--批量删除超高数据-->
<delete id="deleteBatchById" parameterType="java.util.List">
delete from artb_fee_high_rule
where id IN
<foreach collection="list" item="item" open="(" separator="," close=")">
#{item}
</foreach>
</delete>
最后返回成功,或者失败:
if (update > 0) {
return Result.OK("修改成功");
} else {
return Result.error("修改失败");
}
|