package sort;
public class MyMerge {
private static int[] other;
public static void sort(int[] arr){
int lo=0;
int hi=arr.length-1;
other=new int[arr.length];
div(arr,lo,hi);
}
private static void div(int[] arr,int lo,int hi){
if(lo>=hi){
return;
}
int mid =(lo+hi)/2;
div(arr,lo,mid);
div(arr,mid+1,hi);
merge(arr,lo,mid,hi);
}
private static void merge(int[] arr,int lo,int mid,int hi){
int i=lo;
int left=lo;
int right=mid+1;
while (left<=mid&&right<=hi){
if(arr[left]<arr[right]){
other[i++]=arr[left++];
}
else {
other[i++]=arr[right++];
}
}
while (left<=mid){
other[i++]=arr[left++];
}
while (right<=hi){
other[i++]=arr[right++];
}
for(int p=lo;p<=hi;p++){
arr[p]=other[p];
}
}
}
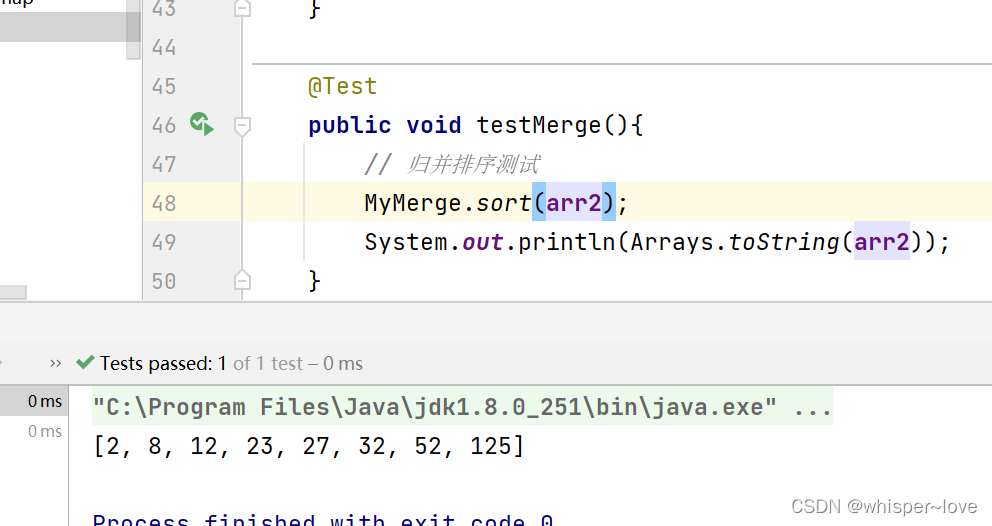
|