一、定义结构
使用 struct 语句来定义结构,结构是一个包含多个成员的数据类型。 struct 语句的格式如下:
struct tag {
member-list
member-list
member-list
...
} variable-list ;
在一般情况下,tag、member-list、variable-list 这 3 部分至少要出现 2 个。
符号 | 含义 |
---|
tag | 结构体标签 | member-list | 标准的变量定义,比如 int i; 或者 float f 等 | variable-list | 结构变量,定义在结构的末尾,最后一个分号之前,可以指定一个或多个结构变量 |
二、声明结构的形式
第一种
struct point {
int x;
float y;
double z;
};
struct point p1, p2[10], *p3;
第二种
struct {
int x;
float y;
double z;
}p1, p2[10], *p3;
第三种
struct point {
int x;
float y;
double z;
}p1, p2, p3;
第一种和第三种形式,都声明了结构 point,但第二种形式没有声明 point,只是定义了三个变量。
第四种
struct COMPLEX
{
char string[100];
struct SIMPLE a;
};
struct NODE
{
char string[100];
struct NODE *next_node;
};
第五种
struct B;
struct A
{
struct B *partner;
};
struct B
{
struct A *partner;
};
三、结构的初始化
第一种
#include <stdio.h>
struct date {
int month;
int day;
int year;
}today = { 3,28,2022 }, thismonth = { .month = 3, .year = 2022 };
int main()
{
printf("Today's date is %d-%d-%d.\n",
today.year, today.month, today.day);
printf("This month is %d-%d-%d.\n",
thismonth.year, thismonth.month, thismonth.day);
return 0;
}
运行结果
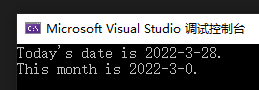
第二种
#include <stdio.h>
struct date {
int month;
int day;
int year;
};
int main()
{
struct date today = { 3,28,2022 };
struct date thismonth = { .month = 3, .year = 2022 };
printf("Today's date is %d-%d-%d.\n",
today.year, today.month, today.day);
printf("This month is %d-%d-%d.\n",
thismonth.year, thismonth.month, thismonth.day);
return 0;
}
运行结果
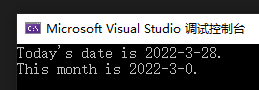
四、访问结构成员
- 结构用成员访问运算符(.)和变量名访问其成员。
- 要访问整个结构,直接用结构变量的名字
五、结构运算
对于整个结构,可以做以下运算:赋值、取地址、传递给函数作为参数(传参方式与其他类型的变量或指针类似)。下面的实例1、2、3 表达的结果是一致的,只是表达方式有所不同。
实例1
#include <stdio.h>
struct date {
int month;
int day;
int year;
};
int main()
{
struct date today = { 3,28,2022 };
struct date day;
day = today;
printf("Today's date is %d-%d-%d.\n",
today.year, today.month, today.day);
printf("The day is %d-%d-%d.\n",
day.year, day.month, day.day);
return 0;
}
运行结果
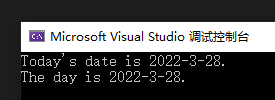
实例2
#include <stdio.h>
struct date {
int month;
int day;
int year;
};
int main()
{
struct date today;
today = (struct date){ 3, 28, 2022 };
struct date day;
day = today;
printf("Today's date is %d-%d-%d.\n",
today.year, today.month, today.day);
printf("The day is %d-%d-%d.\n",
day.year, day.month, day.day);
return 0;
}
运行结果
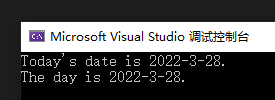
实例3
#include <stdio.h>
struct date {
int month;
int day;
int year;
};
int main()
{
struct date today;
today.month = 3;
today.day = 28;
today.year = 2022;
struct date day;
day.month = today.month;
day.day = today.day;
day.year = today.year;
printf("Today's date is %d-%d-%d.\n",
today.year, today.month, today.day);
printf("The day is %d-%d-%d.\n",
day.year, day.month, day.day);
return 0;
}
运行结果
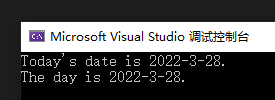
六、结构指针
和数组不同,结构变量的名字并不是结构变量的地址,必须使用 & 运算符
struct date* pDate = &today;
实例
#include <stdio.h>
struct date {
int month;
int day;
int year;
};
int main()
{
struct date today;
today = (struct date){ 3, 28, 2022 };
struct date day;
day = today;
struct date* pDate = &today;
printf("Today's date is %d-%d-%d.\n",
today.year, today.month, today.day);
printf("The day is %d-%d-%d.\n",
day.year, day.month, day.day);
printf("address of today is %p\n", pDate);
return 0;
}
运行结果
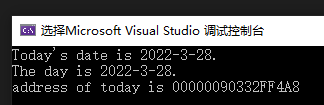
|