一、需求
拖动分割条可以改变div宽度 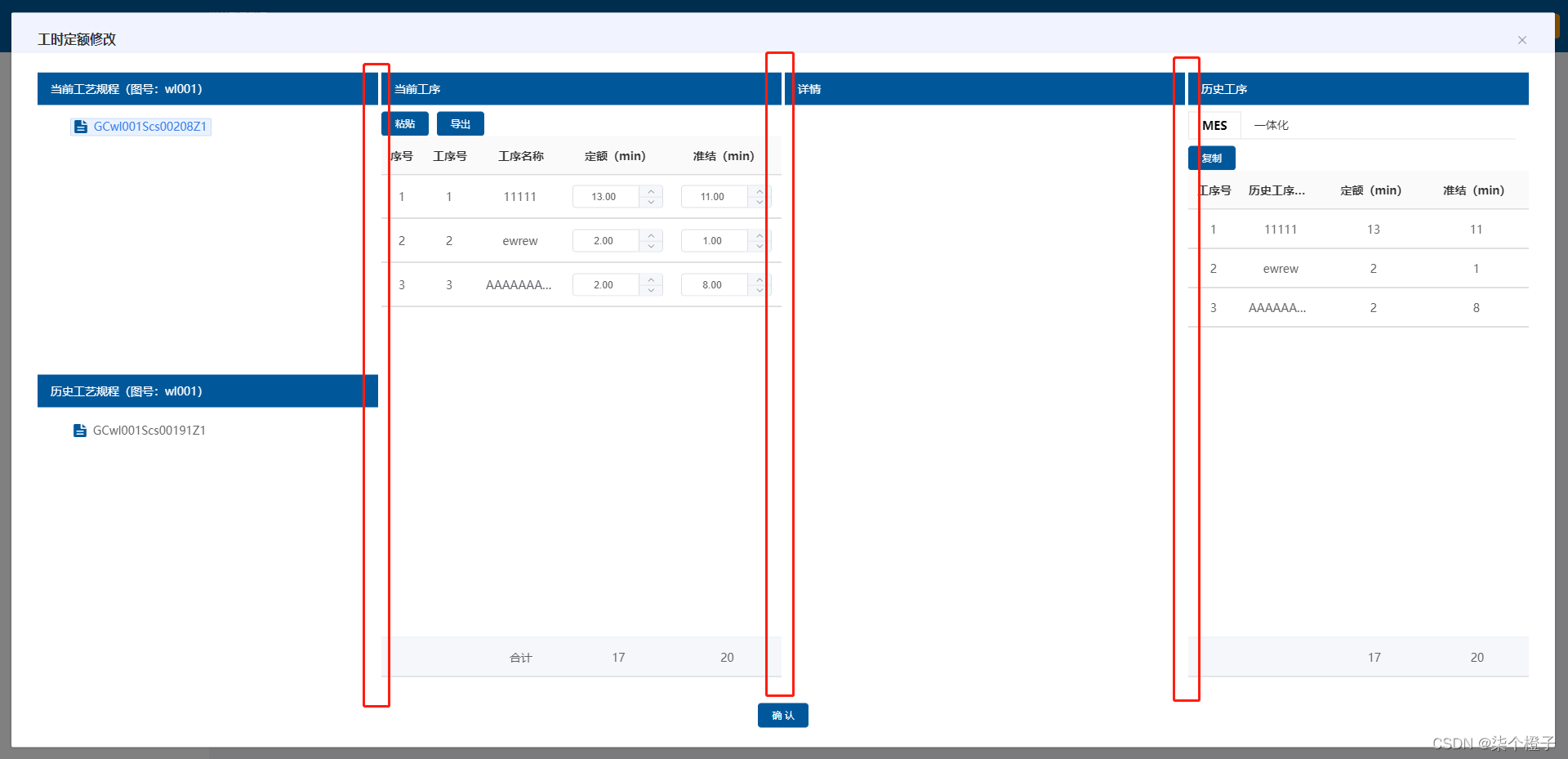
二、实现
- html
<div ref="boxContent" class="h100p flex-a-center-j-space-between">
<div ref="firstContent" class="h100p content">第一列</div>
<div ref="dividerLeft" class="divider"></div>
<div ref="secondContent" class="h100p content">第二列</div>
<div ref="dividerMid" class="divider"></div>
<div ref="thirdContent" class="h100p content">第三列</div>
<div ref="dividerRight" class="divider"></div>
<div ref="forthContent" class="h100p content">第四列</div>
</div>
- js
mounted() {
this.drapContent();
},
methods: {
drapContent() {
let dividerLeft = this.$refs.dividerLeft;
let dividerMid = this.$refs.dividerMid;
let dividerRight = this.$refs.dividerRight;
let firstContent = this.$refs.firstContent;
let secondContent = this.$refs.secondContent;
let thirdContent = this.$refs.thirdContent;
let forthContent = this.$refs.forthContent;
let boxContent = this.$refs.boxContent;
dividerLeft.onmousedown = function (e) {
var startX = e.clientX;
dividerLeft.left = dividerLeft.offsetLeft;
document.onmousemove = function (e) {
var endX = e.clientX;
var thirdContentWidth = thirdContent.offsetWidth;
var forthContentWidth = forthContent.offsetWidth;
var moveLen = dividerLeft.left + (endX - startX);
var maxT = boxContent.clientWidth - dividerLeft.offsetWidth;
if (moveLen < 250) { moveLen = 250; }
if (moveLen > maxT - thirdContentWidth - forthContentWidth - 250) { moveLen = maxT - thirdContentWidth - forthContentWidth - 250; }
dividerLeft.style.left = moveLen;
firstContent.style.width = moveLen + 'px';
secondContent.style.width = (boxContent.clientWidth - moveLen - thirdContentWidth - forthContentWidth - 4) + 'px';
};
document.onmouseup = function (evt) {
document.onmousemove = null;
document.onmouseup = null;
dividerLeft.releaseCapture && dividerLeft.releaseCapture();
};
dividerLeft.setCapture && dividerLeft.setCapture();
return false;
};
dividerMid.onmousedown = function (e) {
var startX = e.clientX;
dividerMid.left = dividerMid.offsetLeft;
document.onmousemove = function (e) {
var endX = e.clientX;
var firstContentWidth = firstContent.offsetWidth;
var forthContentWidth = forthContent.offsetWidth;
var moveLen = dividerMid.left + (endX - startX) - firstContentWidth;
var maxT = boxContent.clientWidth - dividerMid.offsetWidth - 2;
if (moveLen < 250) { moveLen = 250; }
if (moveLen > maxT - firstContentWidth - forthContentWidth - 250) { moveLen = maxT - firstContentWidth - forthContentWidth - 250; }
dividerMid.style.left = moveLen;
secondContent.style.width = moveLen + 'px';
thirdContent.style.width = (boxContent.clientWidth - moveLen - firstContentWidth - forthContentWidth - 4) + 'px';
};
document.onmouseup = function (evt) {
document.onmousemove = null;
document.onmouseup = null;
dividerMid.releaseCapture && dividerMid.releaseCapture();
};
dividerMid.setCapture && dividerMid.setCapture();
return false;
};
dividerRight.onmousedown = function (e) {
var startX = e.clientX;
dividerRight.left = dividerRight.offsetLeft;
document.onmousemove = function (e) {
var endX = e.clientX;
var firstContentWidth = firstContent.offsetWidth;
var secondContentWidth = secondContent.offsetWidth;
var moveLen = dividerRight.left + (endX - startX) - firstContentWidth - secondContentWidth;
var maxT = boxContent.clientWidth - dividerRight.offsetWidth - 2;
if (moveLen < 250) { moveLen = 250; }
if (moveLen > maxT - firstContentWidth - secondContentWidth - 250) { moveLen = maxT - firstContentWidth - secondContentWidth - 250; }
dividerRight.style.left = moveLen;
thirdContent.style.width = moveLen + 'px';
forthContent.style.width = (boxContent.clientWidth - moveLen - firstContentWidth - secondContentWidth - 4) + 'px';
};
document.onmouseup = function (evt) {
document.onmousemove = null;
document.onmouseup = null;
dividerRight.releaseCapture && dividerRight.releaseCapture();
};
dividerRight.setCapture && dividerRight.setCapture();
return false;
};
}
}
- css
.content {
width: calc(50% - 2px);
}
.divider {
height: 100%;
width: 4px;
background-color: #fff;
cursor: ew-resize;
}
再次进入该页面,各列宽度为拖动之后的宽度,需要进行初始化
mounted() {
this.$nextTick(() => {
if (this.$refs.firstContent) {
this.$refs.firstContent.style.width = this.$refs.boxContent.clientWidth * 0.25 - 2 + 'px';
}
if (this.$refs.secondContent) {
this.$refs.secondContent.style.width = this.$refs.boxContent.clientWidth * 0.25 - 2 + 'px';
}
if (this.$refs.thirdContent) {
this.$refs.thirdContent.style.width = this.$refs.boxContent.clientWidth * 0.25 - 2 + 'px';
}
if (this.$refs.forthContent) {
this.$refs.forthContent.style.width = this.$refs.boxContent.clientWidth * 0.25 - 2 + 'px';
}
this.drapContent();
});
}
|