遵循以下流程 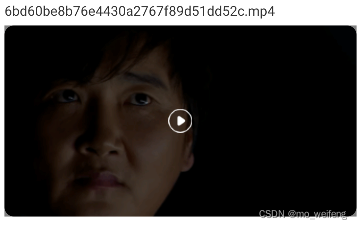
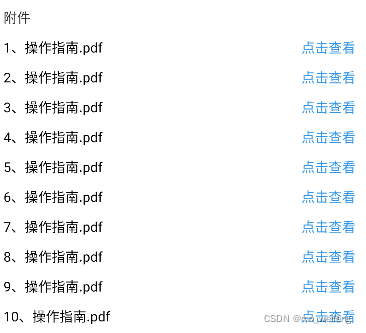
1、加权限
<!-- 在sdcard中创建/删除文件的权限 -->
<uses-permission android:name="android.permission.MOUNT_UNMOUNT_FILESYSTEMS" />
<uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE" />
<uses-permission android:name="android.permission.READ_EXTERNAL_STORAGE" />
2、添加FileProvider(打开文件用)
<provider
android:name="androidx.core.content.FileProvider"
android:authorities="${applicationId}.fileProvider2"
android:exported="false"
android:grantUriPermissions="true">
<meta-data
android:name="android.support.FILE_PROVIDER_PATHS"
android:resource="@xml/filepaths" />
</provider>
filepaths.xml
<?xml version="1.0" encoding="utf-8"?>
<paths xmlns:android="http://schemas.android.com/apk/res/android">
<paths>
<external-path path="." name="jiuxing" />
</paths>
</paths>
3、先创建文件夹,下载用
视频放在Picture,文件放在Download
public static String getSystemVideoFolder(Context context, String folderName) {
String folder = getSystemVideoDir(context)
+ File.separator + folderName;
LogUtils.i("folder=" + folder);
File folderFile = new File(folder);
if (!folderFile.exists()) {
boolean isSuccess = folderFile.mkdirs();
LogUtils.i("创建文件夹路径是否成功=" + isSuccess);
}
return folder;
}
public static String getSystemVideoDir(Context context) {
File folder = Environment.getExternalStoragePublicDirectory(DIRECTORY_PICTURES);
return folder.getAbsolutePath();
}
public static String getSystemFileFolder(Context context, String folderName) {
String folder = getSystemFileDir(context)
+ File.separator + folderName;
LogUtils.i("folder=" + folder);
File folderFile = new File(folder);
if (!folderFile.exists()) {
boolean isSuccess = folderFile.mkdirs();
LogUtils.i("创建文件夹路径是否成功=" + isSuccess);
}
return folder;
}
public static String getSystemFileDir(Context context) {
File folder = Environment.getExternalStoragePublicDirectory(DIRECTORY_DOWNLOADS);
return folder.getAbsolutePath();
}
现在就可以得到下载的目录了
String dir = FolderUtil.getSystemVideoFolder(getContext(), "jiuxing");
String dir = FolderUtil.getSystemFileFolder(getContext(), "jiuxing");
4、下载类
DownloadManager
package com.zues.module_network.util;
import android.text.TextUtils;
import android.util.Log;
import androidx.annotation.NonNull;
import java.io.File;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.InputStream;
import okhttp3.Call;
import okhttp3.Callback;
import okhttp3.OkHttpClient;
import okhttp3.Request;
import okhttp3.Response;
public class DownloadManager {
private final String TAG = getClass().getSimpleName();
private static DownloadManager downloadManager;
private final OkHttpClient okHttpClient;
public static DownloadManager get() {
if (downloadManager == null) {
downloadManager = new DownloadManager();
}
return downloadManager;
}
private DownloadManager() {
okHttpClient = new OkHttpClient();
}
public void download(final String url, final String saveDir, final OnDownloadListener listener) {
download(url, saveDir, listener);
}
public void download(final String url, final String saveDir, String name, final OnDownloadListener listener) {
Request request = new Request.Builder().url(url).build();
okHttpClient.newCall(request).enqueue(new Callback() {
@Override
public void onFailure(Call call, IOException e) {
listener.onFail();
}
@Override
public void onResponse(Call call, Response response) throws IOException {
InputStream is = null;
FileOutputStream fos = null;
try {
byte[] buf = new byte[2048];
int len = 0;
String savePath = isExistDir(saveDir);
String fileName = TextUtils.isEmpty(name) ? getNameFromUrl(url) : name;
is = response.body().byteStream();
long total = response.body().contentLength();
File file = new File(savePath, fileName);
fos = new FileOutputStream(file);
long sum = 0;
while ((len = is.read(buf)) != -1) {
fos.write(buf, 0, len);
sum += len;
int progress = (int) (sum * 1.0f / total * 100);
listener.onProgress(progress);
}
fos.flush();
listener.onSuccess(file);
} catch (Exception e) {
Log.i(TAG, "onResponse: ");
listener.onFail();
} finally {
try {
if (is != null) {
is.close();
}
} catch (IOException e) {
}
try {
if (fos != null) {
fos.close();
}
} catch (IOException e) {
}
}
}
});
}
private String isExistDir(String saveDir) throws IOException {
File downloadFile = new File(saveDir);
if (!downloadFile.mkdirs()) {
downloadFile.createNewFile();
}
return downloadFile.getAbsolutePath();
}
@NonNull
private String getNameFromUrl(String url) {
return url.substring(url.lastIndexOf("/") + 1);
}
public interface OnDownloadListener {
void onSuccess(File file);
void onProgress(int progress);
void onFail();
}
}
5、下载完直接打开文件,通知图库更新
private void downloadVideo(String url, String name) {
String dir = FolderUtil.getSystemVideoFolder(getContext(), "jiuxing");
CustomDialogUtils.showPosDialog2(getContext(), null, "请下载后观看", "取消", "立即下载", new View.OnClickListener() {
@Override
public void onClick(View view) {
showDialog("正在下载");
DownloadManager.get().download(url, dir, name, new DownloadManager.OnDownloadListener() {
@Override
public void onSuccess(File file) {
dismissDialog();
getContext().sendBroadcast(new Intent(Intent.ACTION_MEDIA_SCANNER_SCAN_FILE,
Uri.fromFile(new File(file.getPath()))));
Bundle bundle = new Bundle();
bundle.putString("file", file.getAbsolutePath());
Message message = Message.obtain();
message.setData(bundle);
mVideoHandler.sendMessage(message);
LogUtils.e("文件已下载:" + dir + "/" + name);
}
@Override
public void onProgress(int progress) {
LogUtils.e("progress=" + progress);
}
@Override
public void onFail() {
dismissDialog();
showToast("视频下载失败,请刷新后重试");
}
});
}
}, null);
}
private void downloadFile(String url, String name) {
String dir = FolderUtil.getSystemFileFolder(getContext(), "jiuxing");
CustomDialogUtils.showPosDialog2(getContext(), null, "请下载后查看", "取消", "立即下载", new View.OnClickListener() {
@Override
public void onClick(View view) {
showDialog("正在下载");
DownloadManager.get().download(url, dir, name, new DownloadManager.OnDownloadListener() {
@Override
public void onSuccess(File file) {
dismissDialog();
Bundle bundle = new Bundle();
bundle.putString("file", file.getAbsolutePath());
Message message = Message.obtain();
message.setData(bundle);
mFileHandler.sendMessage(message);
LogUtils.e("文件已下载:" + dir + "/" + name);
}
@Override
public void onProgress(int progress) {
LogUtils.e("progress=" + progress);
}
@Override
public void onFail() {
dismissDialog();
showToast("文件下载失败,请刷新后重试");
}
});
}
}, null);
}
Handler mVideoHandler = new Handler(){
@Override
public void handleMessage(Message msg) {
super.handleMessage(msg);
Bundle bundle = msg.getData();
String url = bundle.getString("file");
FileOpenUtil.openFile(getContext(), new File(url));
}
};
Handler mFileHandler = new Handler(){
@Override
public void handleMessage(Message msg) {
super.handleMessage(msg);
Bundle bundle = msg.getData();
String url = bundle.getString("file");
FileOpenUtil.openFile(getContext(), new File(url));
}
};
6、打开文件工具
FileOpenUtil
package com.zues.module_base.base_util;
import android.content.Context;
import android.content.Intent;
import android.net.Uri;
import android.os.Build;
import android.os.Environment;
import android.webkit.MimeTypeMap;
import android.widget.Toast;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.text.DecimalFormat;
import java.util.ArrayList;
import java.util.Locale;
import androidx.core.content.FileProvider;
public class FileOpenUtil {
public static String SDPATH = Environment.getExternalStorageDirectory().getAbsolutePath();
public static String ZFILEPATH = Environment.getExternalStorageDirectory().getAbsolutePath() + File.separator + "ZFile" + File.separator;
public static final int SIZETYPE_B = 1;
public static final int SIZETYPE_KB = 2;
public static final int SIZETYPE_MB = 3;
public static final int SIZETYPE_GB = 4;
public static File createSDDirs(String path) {
if (Environment.getExternalStorageState().equals(Environment.MEDIA_MOUNTED)) {
File dir = new File(path);
boolean bool = true;
if (!dir.exists()) bool = dir.mkdirs();
if (!bool)
return null;
else
return dir;
}
return null;
}
public static File createSDDir(String path) {
if (Environment.getExternalStorageState().equals(Environment.MEDIA_MOUNTED)) {
File dir = new File(path);
boolean bool = true;
if (!dir.exists()) bool = dir.mkdir();
if (!bool)
return null;
else
return dir;
}
return null;
}
public static File createTimeMillisFile() {
try {
long timeMillis = System.currentTimeMillis();
String filePath = SDPATH + File.separator + timeMillis;
File file = new File(filePath);
boolean bool = file.createNewFile();
if (!bool)
return null;
else
return file;
} catch (IOException e) {
e.printStackTrace();
}
return null;
}
public static File createNameFile(String fileName) {
try {
String filePath = SDPATH + File.separator + fileName;
File file = new File(filePath);
boolean bool = file.createNewFile();
if (!bool)
return null;
else
return file;
} catch (IOException e) {
e.printStackTrace();
}
return null;
}
public static boolean delFile(String path) {
File file = new File(path);
return file.isFile() && file.exists() && file.delete();
}
public static boolean deleteDir(String path) {
try {
File dir = new File(path);
if (!dir.exists() || !dir.isDirectory())
return false;
for (File file : dir.listFiles()) {
if (file != null) {
if (file.isFile()) {
if (!file.delete()) return false;
} else if (file.isDirectory()) {
deleteDir(file.getAbsolutePath());
}
}
}
return dir.delete();
} catch (Exception e) {
return false;
}
}
public static String readFile(Context context, String filename) {
FileInputStream fis = null;
byte[] buffer = null;
try {
fis = context.openFileInput(filename);
buffer = new byte[fis.available()];
fis.read(buffer);
} catch (IOException e) {
e.printStackTrace();
} finally {
try {
if (fis != null)
fis.close();
} catch (IOException e) {
e.printStackTrace();
}
}
return new String(buffer != null ? buffer : new byte[0]);
}
public static void writeFile(Context context, String content, String filename, int mode) {
FileOutputStream fos = null;
try {
fos = context.openFileOutput(filename, mode);
fos.write(content.getBytes());
} catch (IOException e) {
e.printStackTrace();
} finally {
try {
if (fos != null)
fos.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
public static void copyFile(String oldPath, String newPath) {
try {
int byteRead;
File oldFile = new File(oldPath);
if (oldFile.exists()) {
InputStream inStream = new FileInputStream(oldPath);
FileOutputStream fs = new FileOutputStream(newPath);
byte[] buffer = new byte[1024 * 5];
while ((byteRead = inStream.read(buffer)) != -1) {
fs.write(buffer, 0, byteRead);
}
inStream.close();
fs.close();
}
} catch (Exception e) {
e.printStackTrace();
}
}
public static void copyFolder(String oldPath, String newPath) {
try {
File newFile = new File(newPath);
boolean bool = newFile.exists();
if (!bool) {
bool = newFile.mkdirs();
}
if (bool) {
File oldFile = new File(oldPath);
String[] files = oldFile.list();
File temp;
for (String file : files) {
if (oldPath.endsWith(File.separator)) {
temp = new File(oldPath + file);
} else {
temp = new File(oldPath + File.separator + file);
}
if (temp.isFile()) {
FileInputStream input = new FileInputStream(temp);
FileOutputStream output = new FileOutputStream(newPath + File.separator + temp.getName());
byte[] b = new byte[1024 * 5];
int len;
while ((len = input.read(b)) != -1) {
output.write(b, 0, len);
}
output.flush();
output.close();
input.close();
}
if (temp.isDirectory()) {
copyFolder(oldPath + File.separator + file, newPath + File.separator + file);
}
}
}
} catch (Exception e) {
e.printStackTrace();
}
}
public static boolean isFileExist(String path) {
LogUtils.i("path="+path);
File file = new File(path);
return file.exists();
}
public static double getFileOrFilesSize(String filePath, int sizeType) {
long blockSize = 0;
try {
File file = new File(filePath);
if (file.exists()) {
if (file.isDirectory()) {
blockSize = getFileSizes(file);
} else {
blockSize = getFileSize(file);
}
}
} catch (Exception e) {
e.printStackTrace();
}
return formatFileSize(blockSize, sizeType);
}
public static String getAutoFileOrFilesSize(String filePath) {
long blockSize = 0;
try {
File file = new File(filePath);
if (file.exists()) {
if (file.isDirectory()) {
blockSize = getFileSizes(file);
} else {
blockSize = getFileSize(file);
}
}
} catch (Exception e) {
e.printStackTrace();
}
return formatFileSize(blockSize);
}
public static long getFileSize(File file) {
long size = 0;
FileInputStream fis = null;
try {
if (file.exists() && file.isFile()) {
fis = new FileInputStream(file);
size = fis.available();
}
} catch (IOException e) {
e.printStackTrace();
} finally {
try {
if (fis != null)
fis.close();
} catch (IOException e) {
e.printStackTrace();
}
}
return size;
}
public static long getFileSizes(File files) {
long size = 0;
try {
if (files.exists()) {
File[] fList = files.listFiles();
if (fList != null)
for (File file : fList) {
if (file.isDirectory()) {
size = size + getFileSizes(file);
} else {
size = size + getFileSize(file);
}
}
}
} catch (Exception e) {
e.printStackTrace();
}
return size;
}
public static String formatFileSize(long fileSize) {
String fileSizeStr = null;
try {
DecimalFormat df = new DecimalFormat("#.00");
if (fileSize <= 0) {
fileSizeStr = "0B";
} else if (fileSize < 1024) {
fileSizeStr = df.format((double) fileSize) + "B";
} else if (fileSize < 1048576) {
fileSizeStr = df.format((double) fileSize / 1024) + "KB";
} else if (fileSize < 1073741824) {
fileSizeStr = df.format((double) fileSize / 1048576) + "MB";
} else {
fileSizeStr = df.format((double) fileSize / 1073741824) + "GB";
}
} catch (Exception e) {
e.printStackTrace();
}
return fileSizeStr;
}
public static double formatFileSize(long fileSize, int sizeType) {
try {
DecimalFormat df = new DecimalFormat("#.00");
double fileSizeLong = 0;
switch (sizeType) {
case SIZETYPE_B:
fileSizeLong = Double.valueOf(df.format((double) fileSize));
break;
case SIZETYPE_KB:
fileSizeLong = Double.valueOf(df.format((double) fileSize / 1024));
break;
case SIZETYPE_MB:
fileSizeLong = Double.valueOf(df.format((double) fileSize / 1048576));
break;
case SIZETYPE_GB:
fileSizeLong = Double.valueOf(df.format((double) fileSize / 1073741824));
break;
default:
break;
}
return fileSizeLong;
} catch (Exception e) {
return 0;
}
}
public static long getTotalCacheSize(Context context) {
long cacheSize = 0;
try {
cacheSize = getFileSizes(context.getCacheDir());
if (Environment.getExternalStorageState().equals(Environment.MEDIA_MOUNTED)) {
cacheSize += getFileSizes(context.getExternalCacheDir());
}
} catch (Exception e) {
e.printStackTrace();
}
return cacheSize;
}
public static String getFormatTotalCacheSize(Context context) {
try {
long cacheSize = getFileSizes(context.getCacheDir());
if (Environment.getExternalStorageState().equals(Environment.MEDIA_MOUNTED)) {
cacheSize += getFileSizes(context.getExternalCacheDir());
}
return formatFileSize(cacheSize);
} catch (Exception e) {
return null;
}
}
public static void clearAllCache(Context context) {
try {
deleteDir(context.getCacheDir().getAbsolutePath());
if (Environment.getExternalStorageState().equals(Environment.MEDIA_MOUNTED)) {
if (context.getExternalCacheDir() != null)
deleteDir(context.getExternalCacheDir().getAbsolutePath());
}
} catch (Exception e) {
e.printStackTrace();
}
}
public static Boolean cleanSharedPreference(Context context) {
return deleteDir(new File(File.separator + "data" + File.separator + "data" + File.separator + context.getPackageName() + File.separator + "sharedprefs").getAbsolutePath());
}
public static Boolean delDatabaseByName(Context context, String dbName) {
return context.deleteDatabase(dbName);
}
public static void openFile(Context context,File file) {
if (file.exists()) {
String filePath = file.getAbsolutePath();
String suffix = filePath.substring(filePath.lastIndexOf('.')).toLowerCase(Locale.US);
try {
MimeTypeMap mimeTypeMap = MimeTypeMap.getSingleton();
String temp = suffix.substring(1);
String mime = mimeTypeMap.getMimeTypeFromExtension(temp);
Intent intent = new Intent();
intent.setAction(Intent.ACTION_VIEW);
if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.N) {
intent.setFlags(Intent.FLAG_GRANT_READ_URI_PERMISSION);
Uri contentUri = FileProvider.getUriForFile(context, context.getApplicationContext().getPackageName() + ".fileProvider2", file);
intent.setDataAndType(contentUri, mime);
} else {
intent.setDataAndType(Uri.fromFile(file), mime);
intent.setFlags(Intent.FLAG_ACTIVITY_NEW_TASK);
}
context.startActivity(intent);
} catch (Exception e) {
e.printStackTrace();
Toast.makeText(context, "无法打开后缀名为" + suffix + "的文件!", Toast.LENGTH_SHORT).show();
}
}
}
public static File[] getFiles(String path) {
File file = new File(path);
return file.listFiles();
}
public static ArrayList<FileInfoBean> getFileInfos(String path) {
ArrayList<FileInfoBean> fileInfoBeans = new ArrayList<>();
File files = new File(path);
if (files.exists()) {
File[] fileList = files.listFiles();
if (files.isDirectory() && fileList != null) {
for (File file1 : fileList) {
String fileName = file1.getName();
String filePath = file1.getAbsolutePath();
long fileSize = getFileSizes(file1);
fileInfoBeans.add(new FileInfoBean(fileName, filePath, fileSize));
}
} else {
String fileName = files.getName();
String filePath = files.getAbsolutePath();
long fileSize = getFileSizes(files);
fileInfoBeans.add(new FileInfoBean(fileName, filePath, fileSize));
}
}
return fileInfoBeans;
}
}
package com.zues.module_base.base_util;
public class FileInfoBean {
private String fileName;
private String filePath;
private long fileSize;
public FileInfoBean() {
super();
}
public FileInfoBean(String fileName, String filePath, long fileSize) {
this.fileName = fileName;
this.filePath = filePath;
this.fileSize = fileSize;
}
public String getFileName() {
return fileName;
}
public void setFileName(String fileName) {
this.fileName = fileName;
}
public String getFilePath() {
return filePath;
}
public void setFilePath(String filePath) {
this.filePath = filePath;
}
public long getFileSize() {
return fileSize;
}
public void setFileSize(long fileSize) {
this.fileSize = fileSize;
}
@Override
public String toString() {
return "FileInfoBean{" +
"fileName='" + fileName + '\'' +
", filePath='" + filePath + '\'' +
", fileSize=" + fileSize +
'}';
}
}
7、提示框
public static void showPosDialog2(Context context, String title, String content, String cancelText, String okText, View.OnClickListener onConfirmClickListener, View.OnClickListener onCancelClickListener) {
android.app.AlertDialog.Builder builder = new android.app.AlertDialog.Builder(context);
View dv = View.inflate(context, R.layout.pos_dialog2, null);
TextView tv_content = (TextView) dv.findViewById(R.id.tv_content);
TextView btn_ok = (TextView) dv.findViewById(R.id.btn_ok);
TextView btn_cancel = (TextView) dv.findViewById(R.id.btn_cancel);
TextView tv_title = (TextView) dv.findViewById(R.id.tv_title);
tv_content.setText(content);
if (TextUtils.isEmpty(title)) {
tv_title.setVisibility(View.GONE);
} else {
tv_title.setVisibility(View.VISIBLE);
tv_title.setText(title);
}
if (!TextUtils.isEmpty(cancelText)) {
btn_cancel.setText(cancelText);
}
if (!TextUtils.isEmpty(okText)) {
btn_ok.setText(okText);
}
final Dialog dialog = builder.create();
final Window window = dialog.getWindow();
window.setBackgroundDrawable(new ColorDrawable(0));
dialog.show();
dialog.setCanceledOnTouchOutside(false);
dialog.setCancelable(false);
dialog.getWindow().setContentView(dv);
btn_ok.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
dialog.dismiss();
if (onConfirmClickListener != null) {
onConfirmClickListener.onClick(v);
}
}
});
btn_cancel.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
dialog.dismiss();
if (onCancelClickListener != null) {
onCancelClickListener.onClick(v);
}
}
});
}
<?xml version="1.0" encoding="utf-8"?>
<FrameLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginStart="28dp"
android:layout_marginEnd="28dp"
android:background="@drawable/border_solid_radius15_white"
android:orientation="vertical">
<TextView
android:id="@+id/tv_title"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center_horizontal"
android:layout_marginTop="16dp"
android:text="提示"
android:textColor="@color/black"
android:textSize="16sp"
android:textStyle="bold" />
<ScrollView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="16dp">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginLeft="16dp"
android:layout_marginRight="16dp"
android:orientation="vertical">
<TextView
android:id="@+id/tv_content"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="3dp"
android:gravity="center_vertical"
android:lineSpacingExtra="3dp"
android:lineSpacingMultiplier="1.2"
android:minHeight="80dp"
android:textColor="@color/black"
android:textSize="16sp"
tools:text="这是一条自定义消息。用用户对此无感知,为演示做此效果。" />
</LinearLayout>
</ScrollView>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginTop="16dp"
android:layout_marginBottom="16dp"
android:gravity="center">
<TextView
android:id="@+id/btn_cancel"
android:layout_width="0dp"
android:layout_height="42dp"
android:layout_marginLeft="16dp"
android:layout_marginRight="8dp"
android:layout_weight="1"
android:background="@drawable/border_radius999_hint"
android:gravity="center"
android:text="取消"
android:textColor="@color/hint"
android:textSize="16sp"
android:textStyle="bold" />
<TextView
android:id="@+id/btn_ok"
android:layout_width="0dp"
android:layout_height="42dp"
android:layout_marginLeft="8dp"
android:layout_marginRight="16dp"
android:layout_weight="1"
android:background="@drawable/border_custom_dialog"
android:gravity="center"
android:text="确认"
android:textColor="@android:color/white"
android:textSize="16sp"
android:textStyle="bold" />
</LinearLayout>
</LinearLayout>
</FrameLayout>
border_solid_radius15_white
<?xml version="1.0" encoding="utf-8"?>
<shape xmlns:android="http://schemas.android.com/apk/res/android">
<!-- <stroke android:width="1dp" android:color="#BDBDBD"/>-->
<solid android:color="@color/white"/>
<corners android:radius="15dp"></corners>
</shape>
border_radius999_hint
<?xml version="1.0" encoding="utf-8"?>
<shape xmlns:android="http://schemas.android.com/apk/res/android">
<stroke android:width="0.5dp" android:color="@color/hint"/>
<!-- <solid android:color="@color/hint" />-->
<corners android:radius="999dp"></corners>
</shape>
border_custom_dialog
<?xml version="1.0" encoding="utf-8"?>
<shape xmlns:android="http://schemas.android.com/apk/res/android">
<solid android:color="#ff0084f6" />
<corners android:radius="999dp" />
</shape>
|