一、filter()
Array.prototype.filter() - JavaScript | MDNfilter()?方法创建一个新数组, 其包含通过所提供函数实现的测试的所有元素。 https://developer.mozilla.org/zh-CN/docs/Web/JavaScript/Reference/Global_Objects/Array/filter(1)基本用法
语法:
var newArray = arr.filter(callback(element[, index[, array]])[, thisArg])
//【1】filter
const words = ['spray', 'limit', 'elite', 'exuberant', 'destruction', 'present'];
//(1)箭头函数写法
const result = words.filter(word => word.length > 6);
console.log(result.toString()) //exuberant,destruction,present
//(2)普通函数写法
const result_two = words.filter(
function(word ,index ,array){
//do something
console.log(word,index,array);
return word.length > 6//最终return true的word可以返回到result_two中
}
)
console.log(result_two);//['exuberant', 'destruction', 'present']
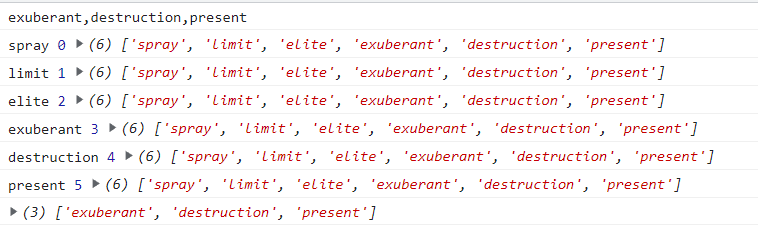
?(2)自己实现fliter方法
核心步骤有:
- 判断参数是否为函数,如果不是则直接返回
- 创建一个空数组用于承载新的内容
- 循环遍历数组中的每个值,分别调用函数参数,将满足判断条件的元素添加进空数组中
- 返回新的数组
//语法:var newArray = arr.filter(callback(element[, index[, array]])[, thisArg])
//要求实现Array.filter函数的功能且该新函数命名为"_filter"
//在Array的原型上增加_filter()方法
Array.prototype._filter = function(fn){
//1、判断fn是否为方法
if (typeof fn !== 'function') {
throw new TypeError('should be function')
}
//2、创建一个新数组接收过滤后的值
const newArray = []
//3、循环遍历数组中的每个值,分别调用函数参数,将满足判断条件的元素添加进空数组中
//(1)拿到数组
let arr = this //this指向调用方法的Array
//(2)将满足判断条件的元素添加进空数组中
for(var i=0; i<arr.length; i++){
let result = fn.call(null, arr[i], i, arr);//result为true or false
result && newArray.push(arr[i])
}
return newArray
}
//第二种方式
Array.prototype._filter_one = function(fn){
let newArr = [];
this.forEach(item => {
if(fn(item)){//item在fn方法中返回true
newArr.push(item);
}
})
return newArr;
}
|