?前言:Unity 提供了一个方法AssetDatabase.GetDependencies() 可以查找资源文件引用了那些资源。但是这是正向查找,如果我们需要反向查找(查找那些资源依赖了某个资源),应该如何呢?
?基本原理:Unity 会给工程中的每一个资源分配一个guid (全局唯一标识),而如果某个资源引用了其他资源,会在资源的文件中记录下引用信息,引用信息中也就包括了引用的资源的guid 。 ?我们便可以通过遍历工程中的资源文件,来查找出那些资源引用了我们指定的资源。
?下面以一个简单的场景进行示例: ?在场景中新建一个Cube ,再创建一个Material ,命名为Red ,再将这个Material 赋给Cube ,最后将Cube 做成预制体。 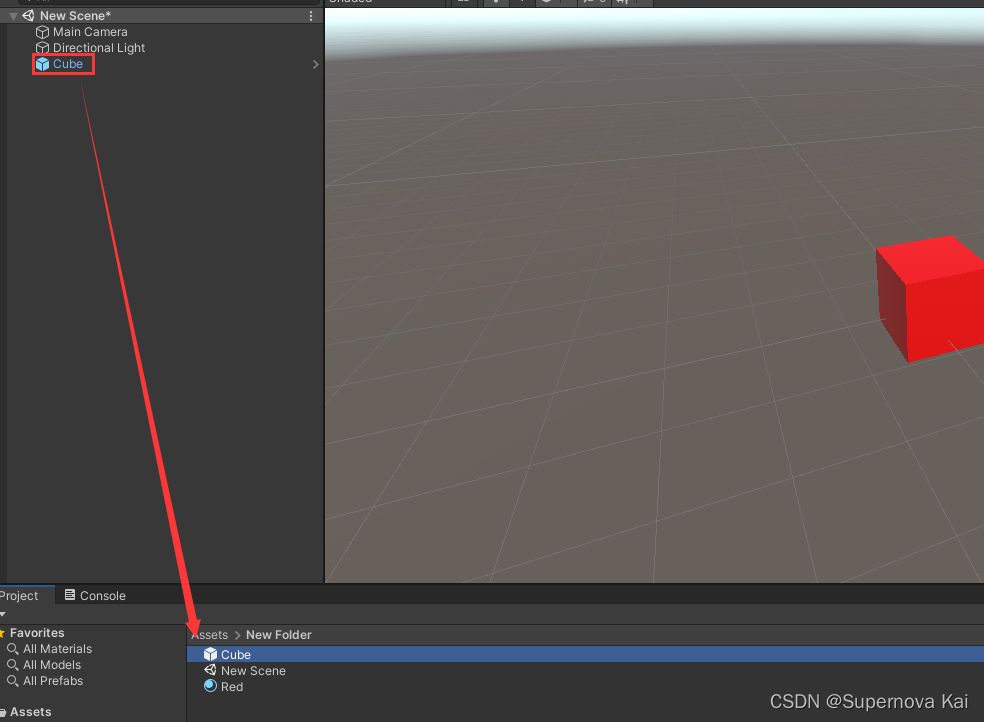 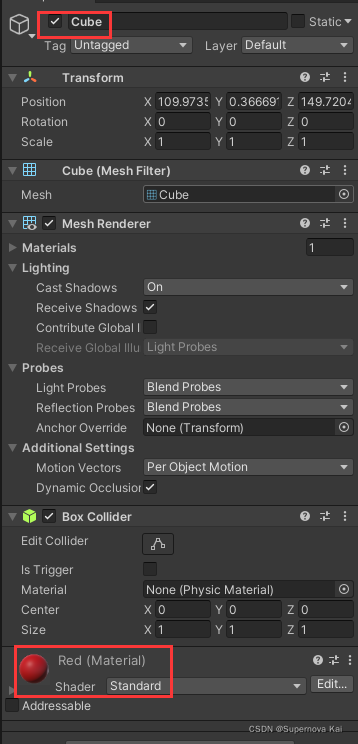
?首先,我们看一下这个名为Red 的Material 的guid 。 ?注意:资源文件自己本身的guid 记录在它的.meta 文件中。 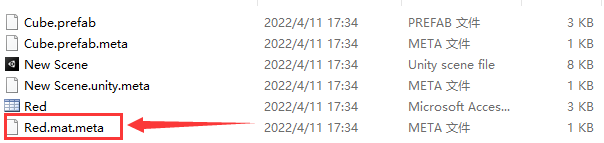 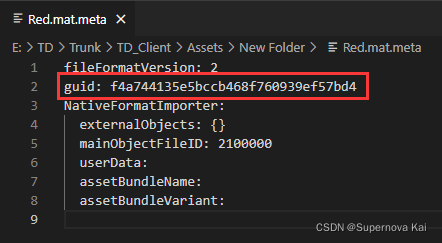 ?我们再打开我们的预制体Cube ,搜索一下这个guid 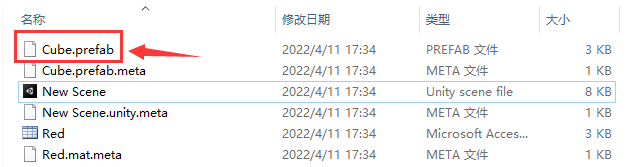 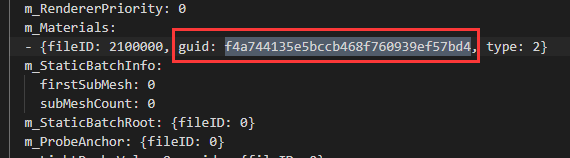 ?验证了我们的原理。
?代码如下:
using UnityEngine;
using UnityEditor;
using System.IO;
using System.Collections.Generic;
using System.Linq;
using System.Text.RegularExpressions;
public class FindReferences
{
[MenuItem("Assets/Find References", priority = 10)]
private static void Find()
{
EditorSettings.serializationMode = SerializationMode.ForceText;
string path = AssetDatabase.GetAssetPath(Selection.activeObject);
if(!string.IsNullOrEmpty(path))
{
string guid = AssetDatabase.AssetPathToGUID(path);
List<string> includeExtensions = new List<string>() { ".prefab", ".unity", ".mat", ".asset" };
string[] files = Directory.GetFiles(Application.dataPath, "*", SearchOption.AllDirectories).Where(s => includeExtensions.Contains(Path.GetExtension(s).ToLower())).ToArray();
int index = 0;
EditorApplication.update = delegate ()
{
string file = files[index];
EditorUtility.DisplayProgressBar("正在搜索中...", file, index / files.Length);
if(Regex.IsMatch(File.ReadAllText(file), guid))
{
Debug.Log(file, AssetDatabase.LoadAssetAtPath<Object>(GetRelativeAssetsPath(file)));
}
index++;
if(index >= files.Length)
{
EditorUtility.ClearProgressBar();
EditorApplication.update = null;
index = 0;
Debug.Log("搜索结束");
}
};
}
}
private static string GetRelativeAssetsPath(string path)
{
return "Assets" + Path.GetFullPath(path).Replace(Path.GetFullPath(Application.dataPath), "").Replace('\\', '/');
}
}
|