1.栈
栈: 又名堆栈,它是一种运算受限的线性表。限定仅在表尾 进行插入和删除操作的线性表。这一端被称为栈顶,相对地,把另一端称为栈底。
2.入栈操作
- 首先判断栈是否满
- 若栈未满,先将数据入栈,然后深度再+1
public boolean push(char paraChar) {
if (depth == MAX_DEPTH) {
System.out.println("Stack full.");
return false;
}
data[depth] = paraChar;
depth++;
return true;
}
3.出栈操作
- 先判断栈是否为空
- 若栈不为空,将栈底元素赋值给resultChar
public char pop() {
if (depth == 0) {
System.out.println("Nothing to pop.");
return '\0';
}
char resultChar = data[depth - 1];
depth--;
return resultChar;
}
4.完整代码实现
package com.datastructure;
public class CharStack {
public static final int MAX_DEPTH = 10;
int depth;
char[] data;
public CharStack() {
depth = 0;
data = new char[MAX_DEPTH];
}
public String toString() {
String resultString = "";
for (int i = 0; i < depth; i++) {
resultString += data[i] + " ";
}
return resultString;
}
public boolean push(char paraChar) {
if (depth == MAX_DEPTH) {
System.out.println("Stack full.");
return false;
}
data[depth] = paraChar;
depth++;
return true;
}
public char pop() {
if (depth == 0) {
System.out.println("Nothing to pop.");
return '\0';
}
char resultChar = data[depth - 1];
depth--;
return resultChar;
}
public static void main(String args[]) {
CharStack tempStack = new CharStack();
for (char ch = 'a'; ch < 'm'; ch++) {
tempStack.push(ch);
System.out.println("The current stack is: " + tempStack);
}
char tempChar;
for (int i = 0; i < 12; i++) {
tempChar = tempStack.pop();
System.out.println("Poped: " + tempChar);
System.out.println("The current stack is: " + tempStack);
}
}
}
代码效果:
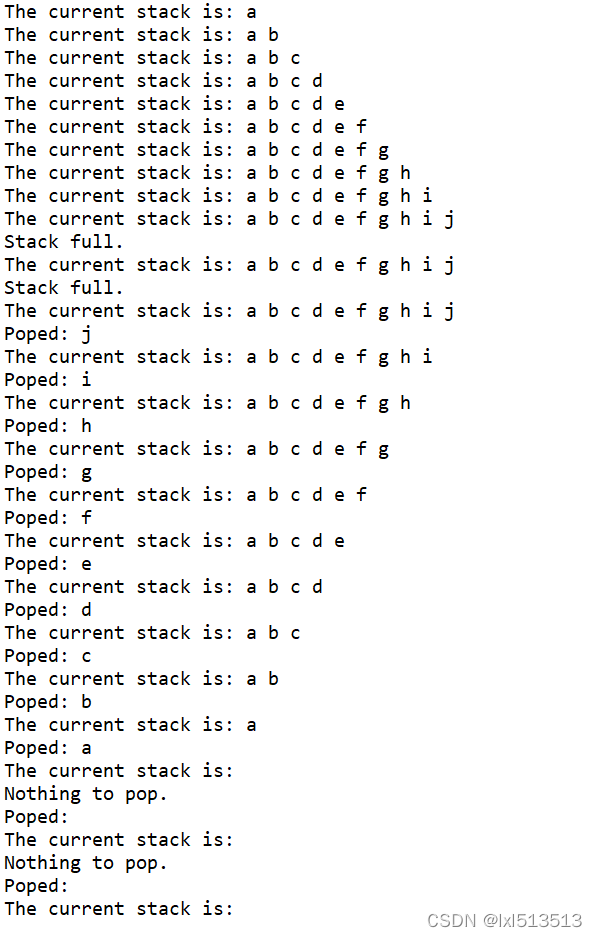
|