一 根据实体类迁移创建表
-
在项目NuGet中安装SqlSugar和System.Data.SqlClient 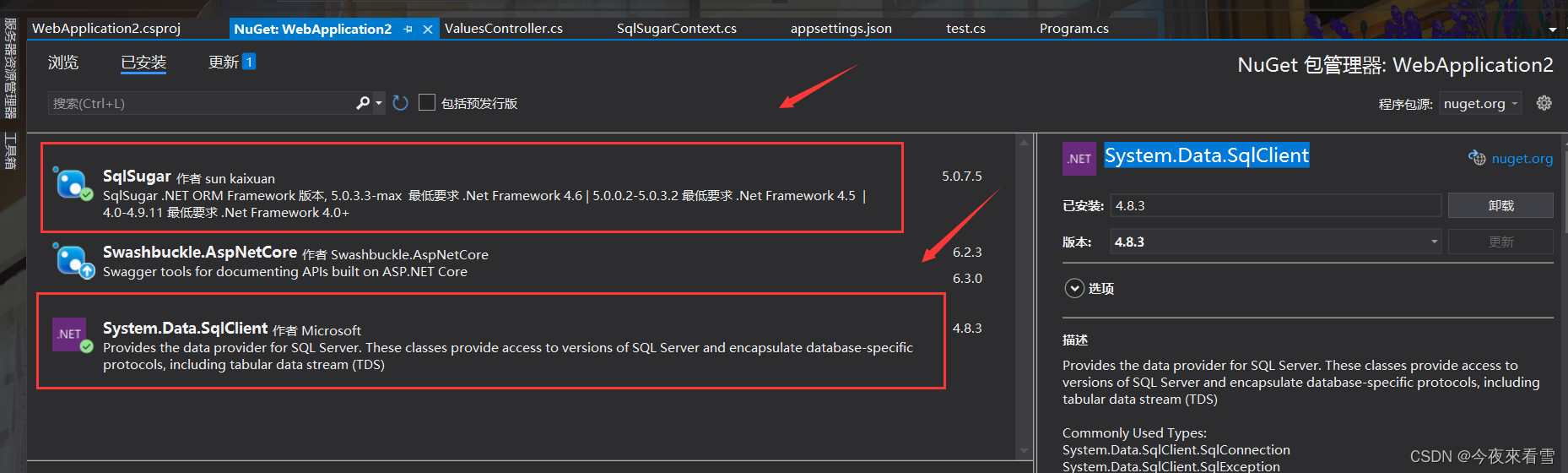 -
创建实体类,存放在Models文件夹下 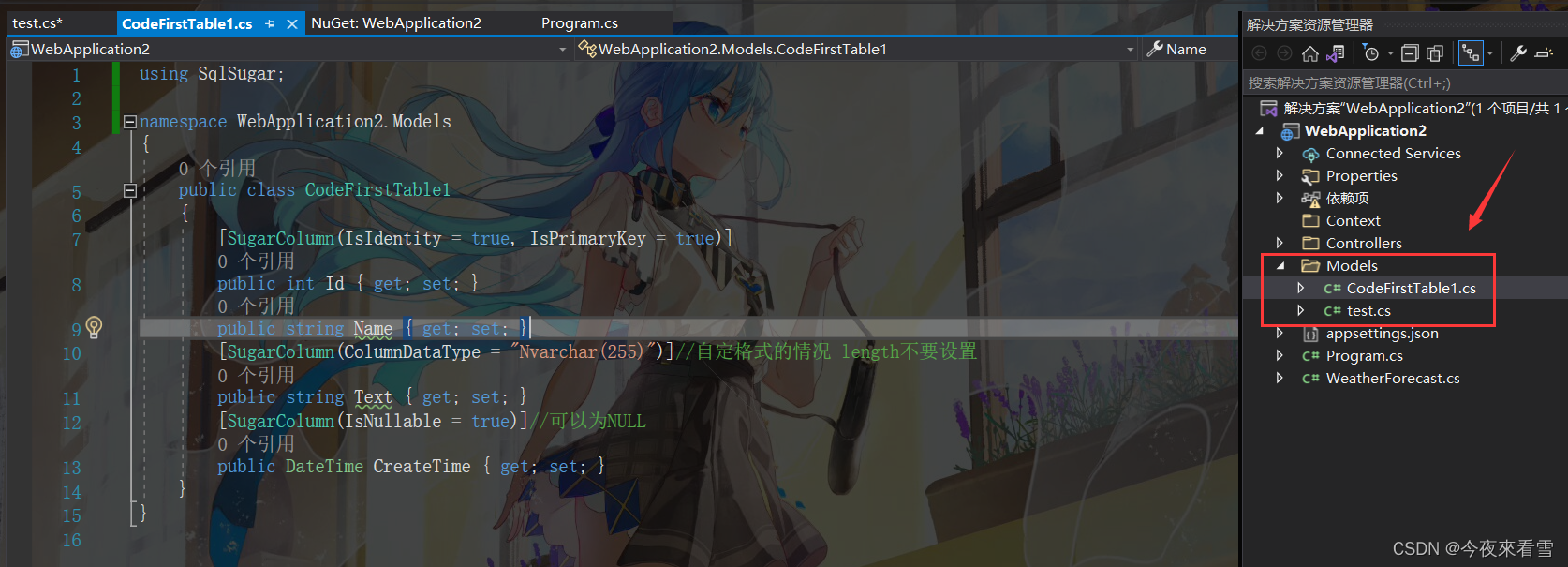 CodeFirstTable1表实体类
using SqlSugar;
namespace WebApplication2.Models
{
public class CodeFirstTable1
{
[SugarColumn(IsIdentity = true, IsPrimaryKey = true)]
public int Id { get; set; }
public string Name { get; set; }
[SugarColumn(ColumnDataType = "Nvarchar(255)")]
public string Text { get; set; }
[SugarColumn(IsNullable = true)]
public DateTime CreateTime { get; set; }
}
}
test表实体类
using SqlSugar;
namespace WebApplication2.Models
{
public class test
{
[SugarColumn(IsIdentity = true, IsPrimaryKey = true)]
public int Id { get; set; }
public string Name { get; set; }
}
}
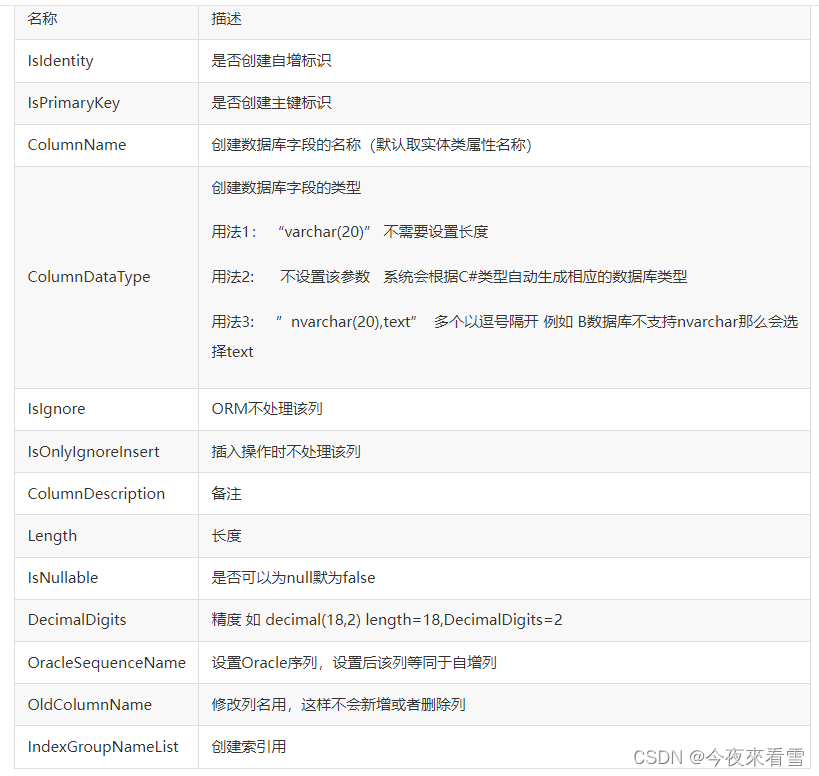 3. 封装迁移创建表和库的方法,新建一个Context文件夹,里面新建一个SqlSugarContext类,用于封装迁移表方法
using SqlSugar;
using WebApplication2.Models;
namespace WebApplication2.Context
{
public class SqlSugarContext
{
public readonly ISqlSugarClient db;
public SqlSugarContext(ISqlSugarClient DBContext)
{
this.db = DBContext;
}
public void CreateTable()
{
db.DbMaintenance.CreateDatabase();
db.CodeFirst.SetStringDefaultLength(50).BackupTable().InitTables(new Type[]
{
typeof(CodeFirstTable1),
typeof(test)
});
}
}
}
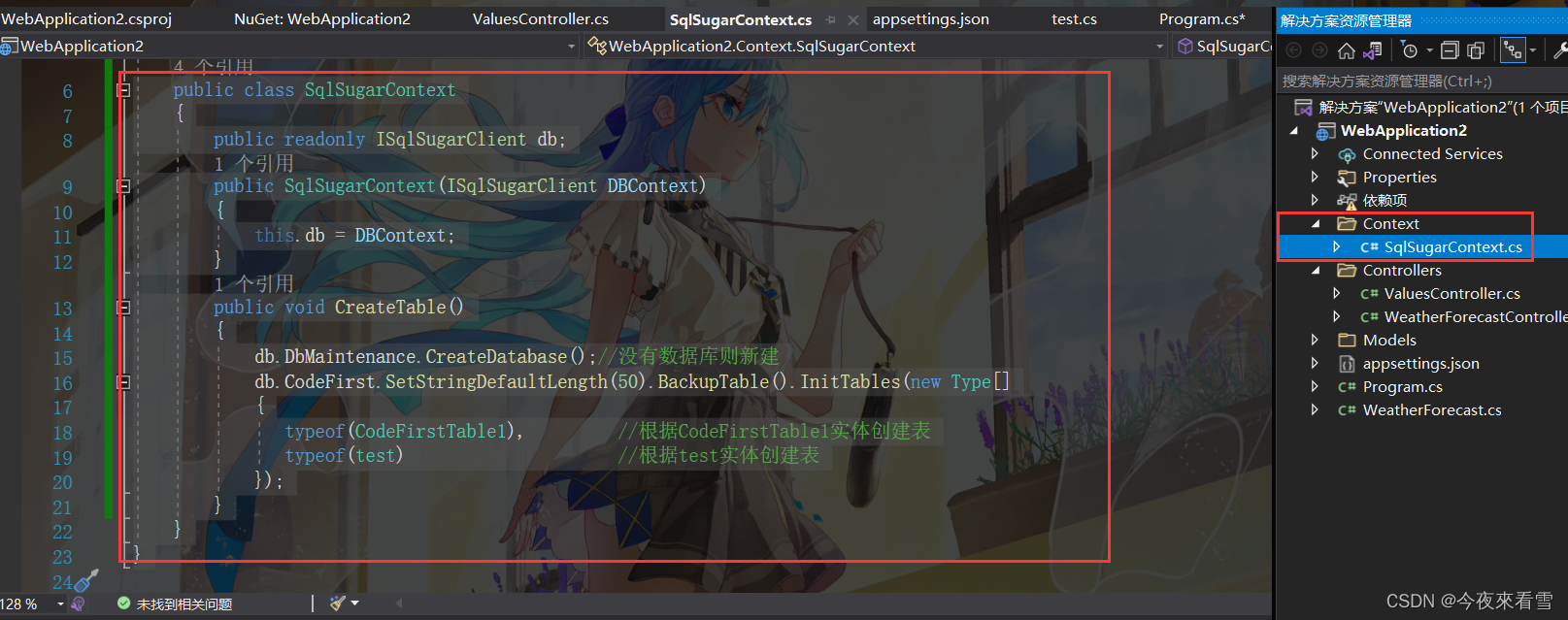
- 添加数据库连接字符串,注册服务
在appsettings.json添加数据库连接字符串
"ConnectionStrings": {
"DbConnectionString": "Data Source = LAPTOP-RMDBADPQ\\NING;Initial Catalog = myDataBasetest;User Id = sa;Password = 123456;"
}
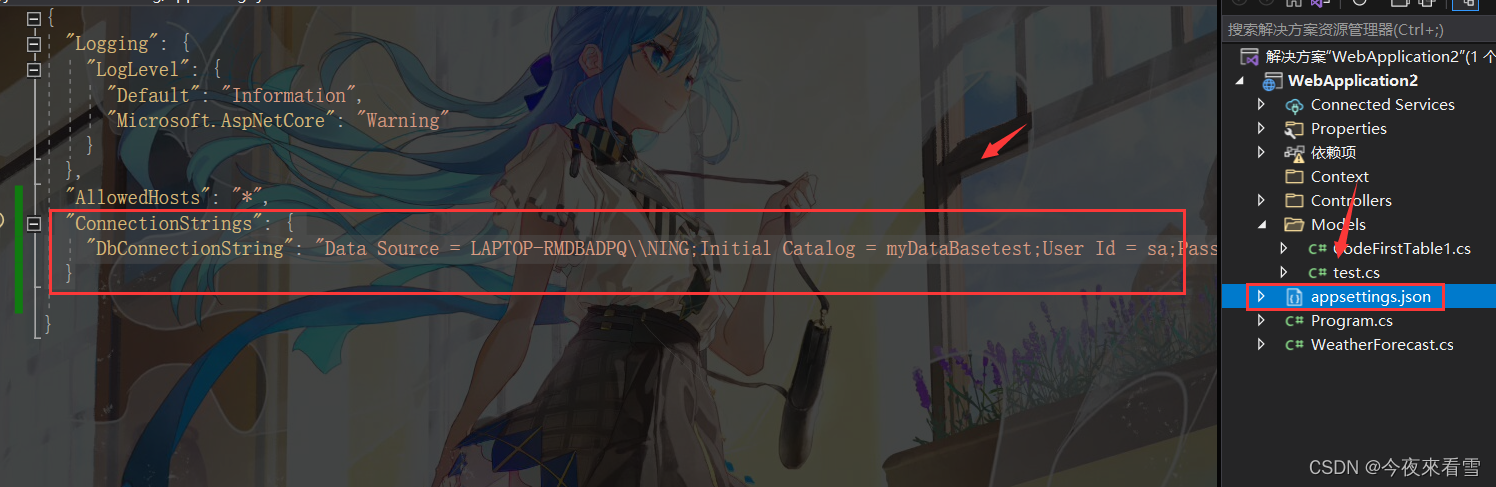 在Program类给刚才封装好的类注册sqlsugar服务
builder.Services.AddSingleton(sp => new SqlSugarContext(
new SqlSugarClient(new ConnectionConfig()
{
ConnectionString = builder.Configuration.GetConnectionString("DbConnectionString"),
DbType = DbType.SqlServer,
IsAutoCloseConnection = true
})
));
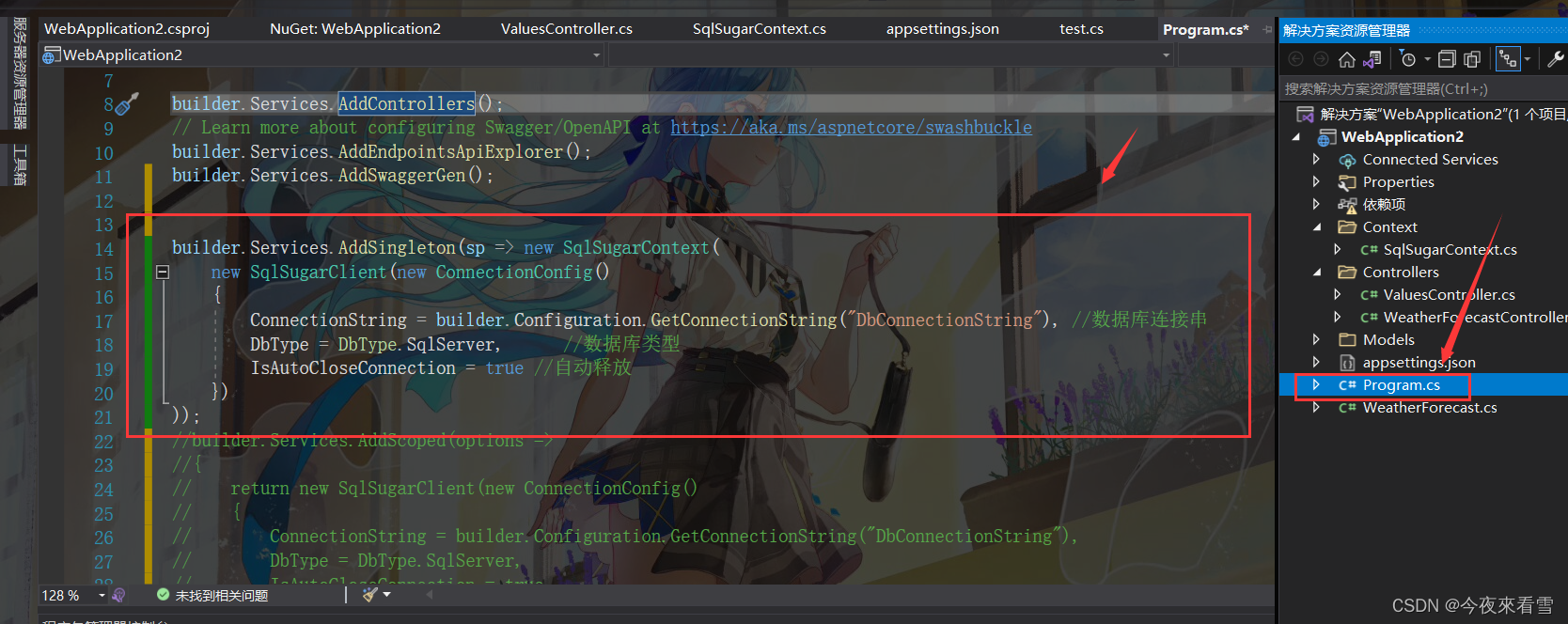 5. 新建api控制器,新建接口调用迁移创建表方法
using Microsoft.AspNetCore.Mvc;
using WebApplication2.Context;
namespace WebApplication2.Controllers
{
[Route("api/[controller]")]
[ApiController]
public class ValuesController : ControllerBase
{
public readonly SqlSugarContext db;
public ValuesController(SqlSugarContext DBContext)
{
this.db = DBContext;
}
[HttpGet("Create")]
public string CreateTable()
{
db.CreateTable();
return "hehe";
}
}
}
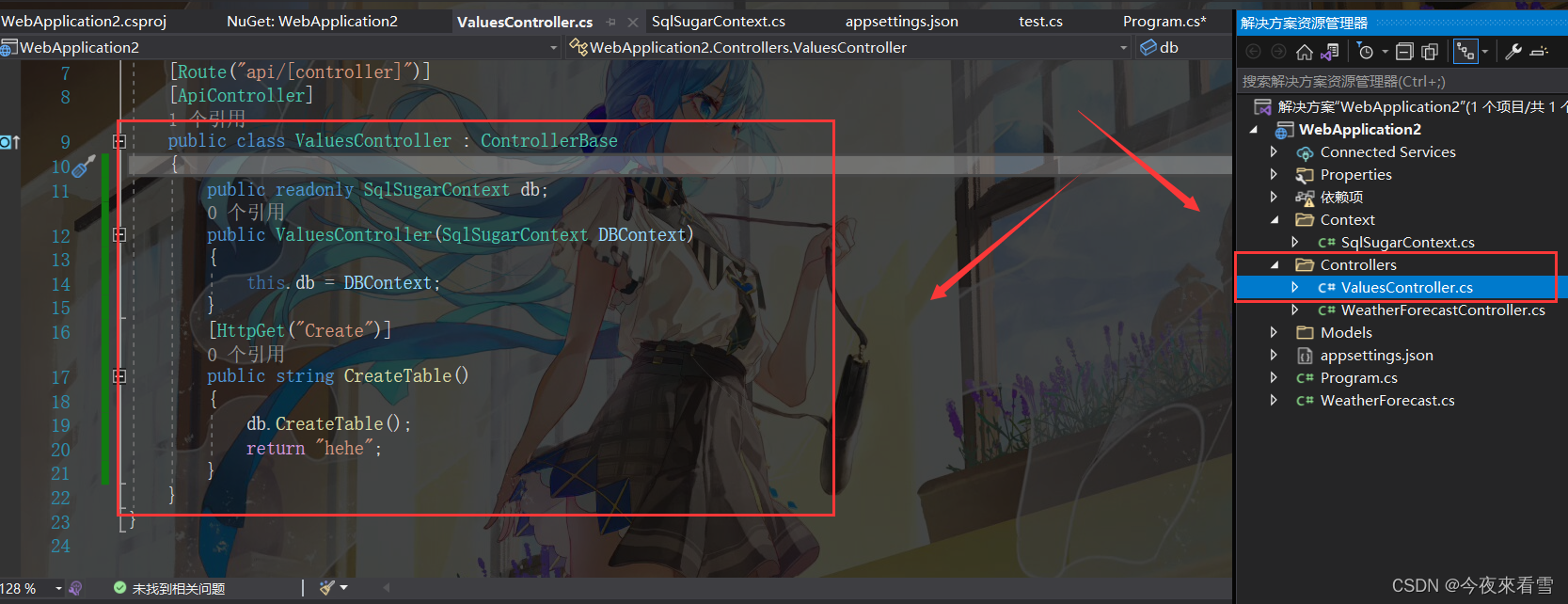 6. 运行接口,创建成功 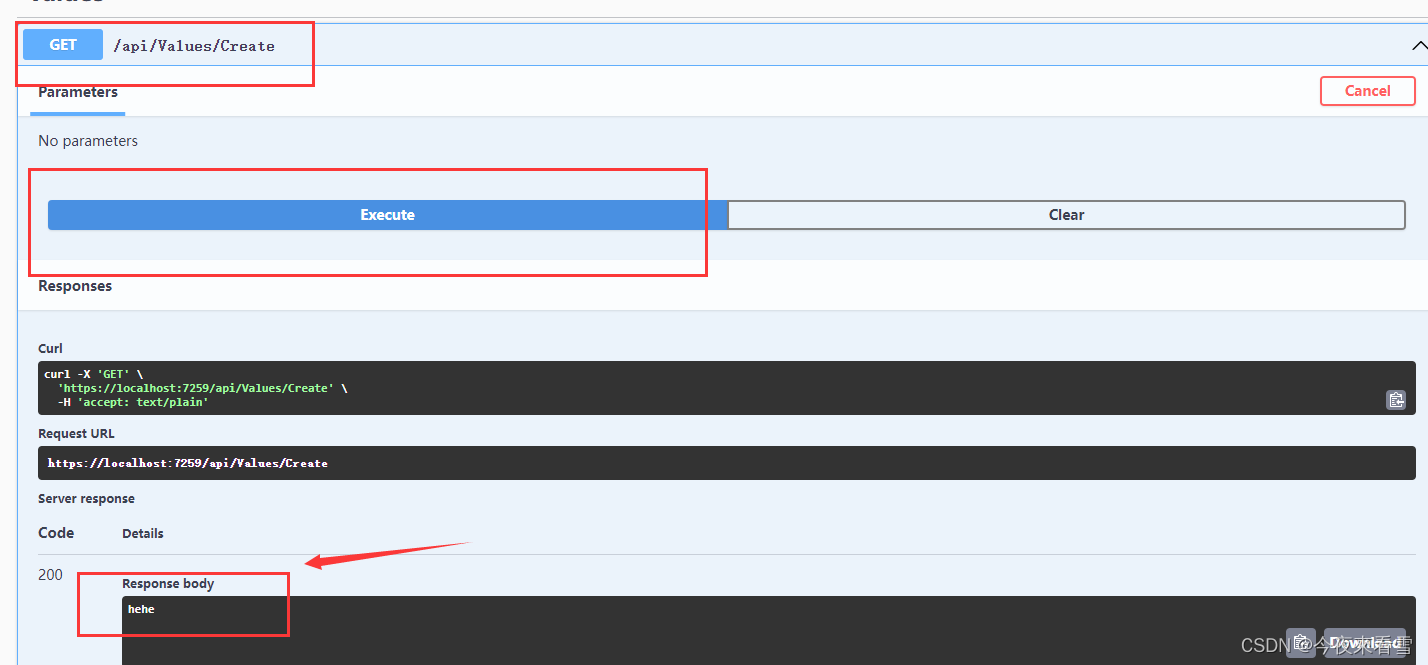 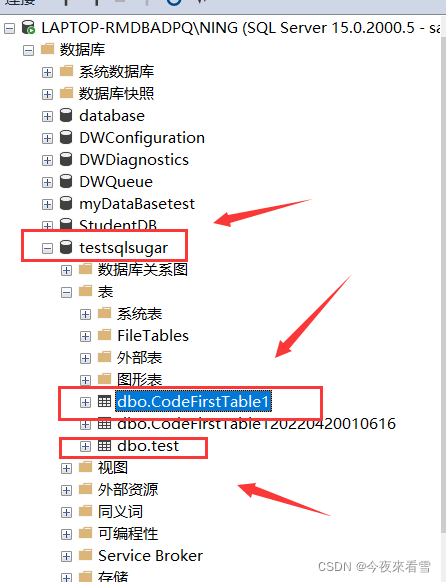
二 根据数据库表生成实体类
- 安装NuGet包 Newtonsoft.Json
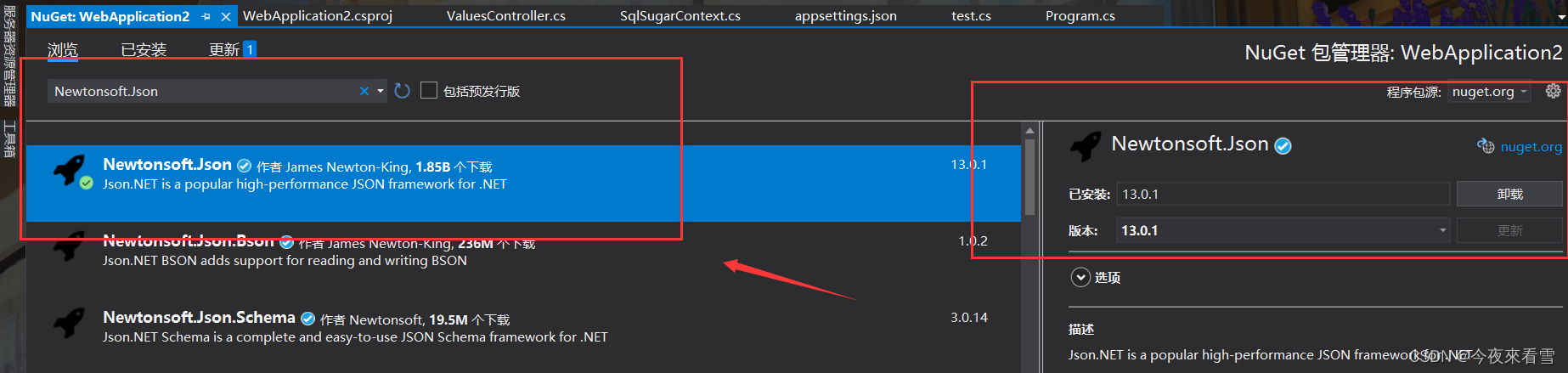 - 在 一 中封装的SqlSugarContext类中添加生成实体方法
public void CreateClassFile()
{
db.DbFirst.IsCreateAttribute().CreateClassFile("D:\\Demo\\1", "Models");
}
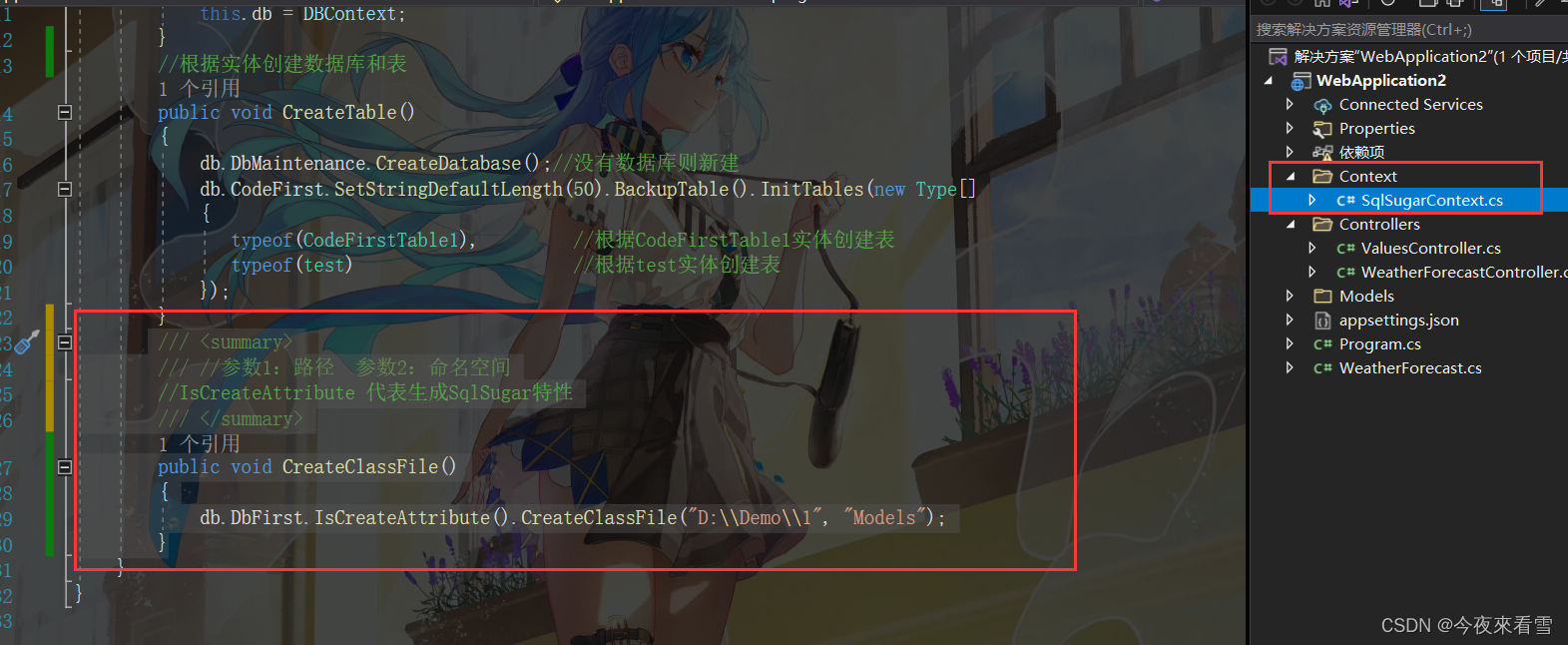 3. 添加api接口调用运行此方法
[HttpGet("CreateClassFile")]
public bool CreateClassFile()
{
db.CreateClassFile();
return true;
}
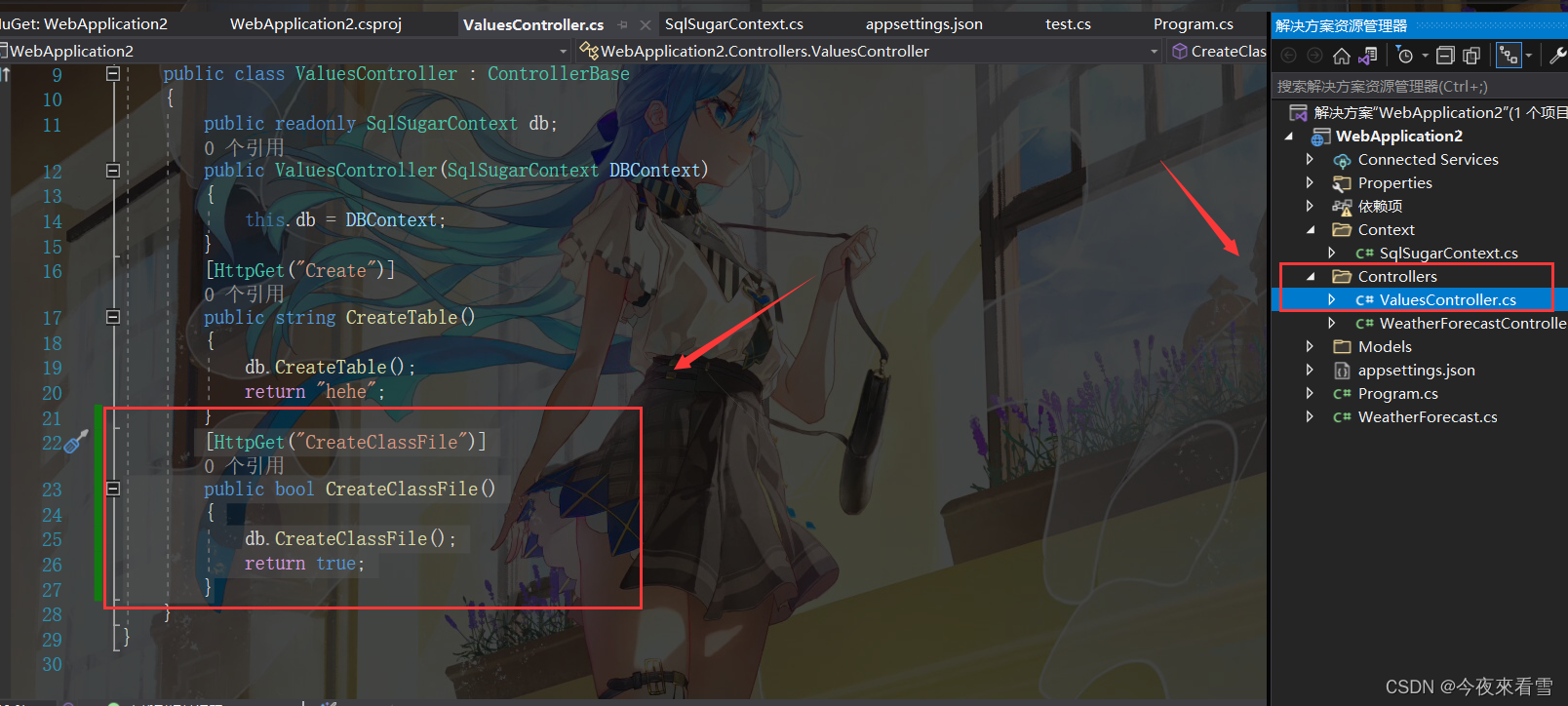 4. 运行项目,调用此api接口,下面运行结果成功 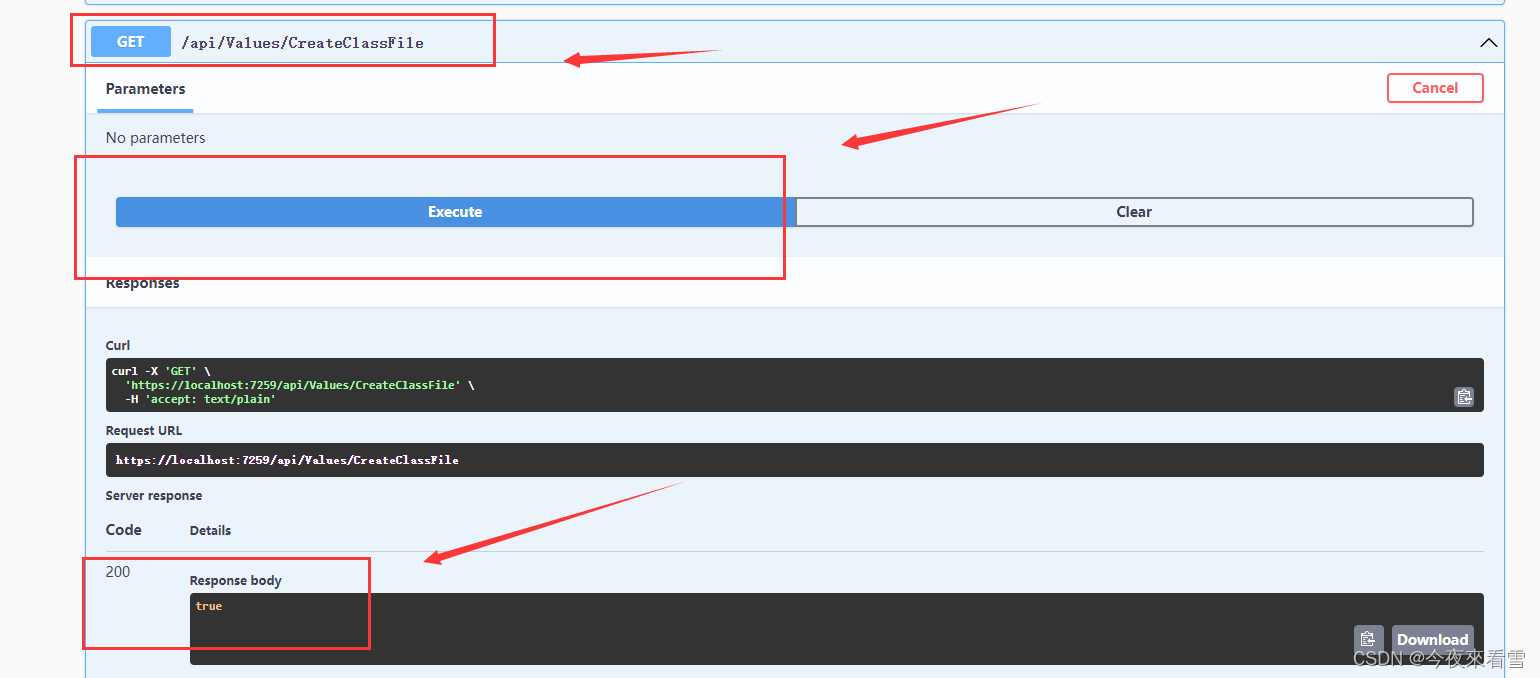 查看生成的实体类,成功 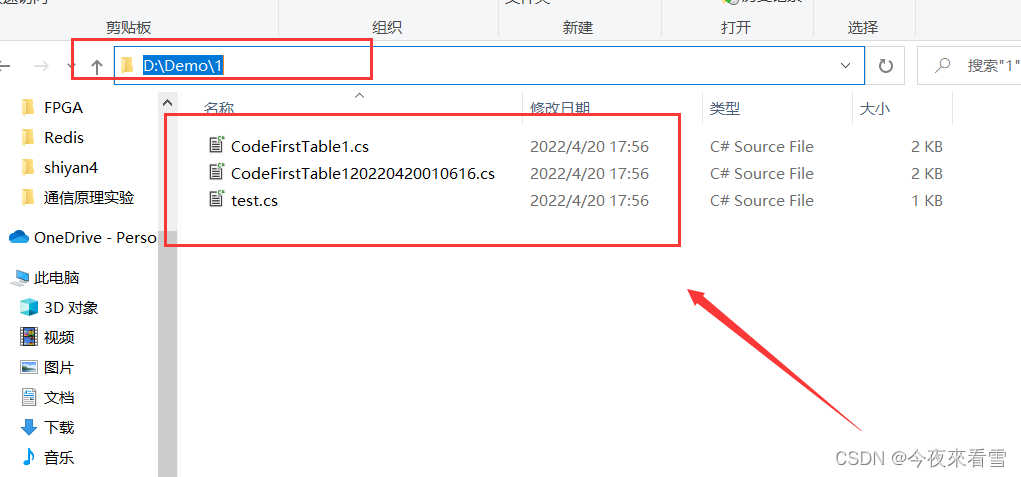
|