编写一个简单的考试程序,在控制台完成出题、答题的交互。试题(Question)分为单选(SingleChoice)和多选( MultiChoice)两种。其中,单选题和多选题继承试题类,如下图所示。
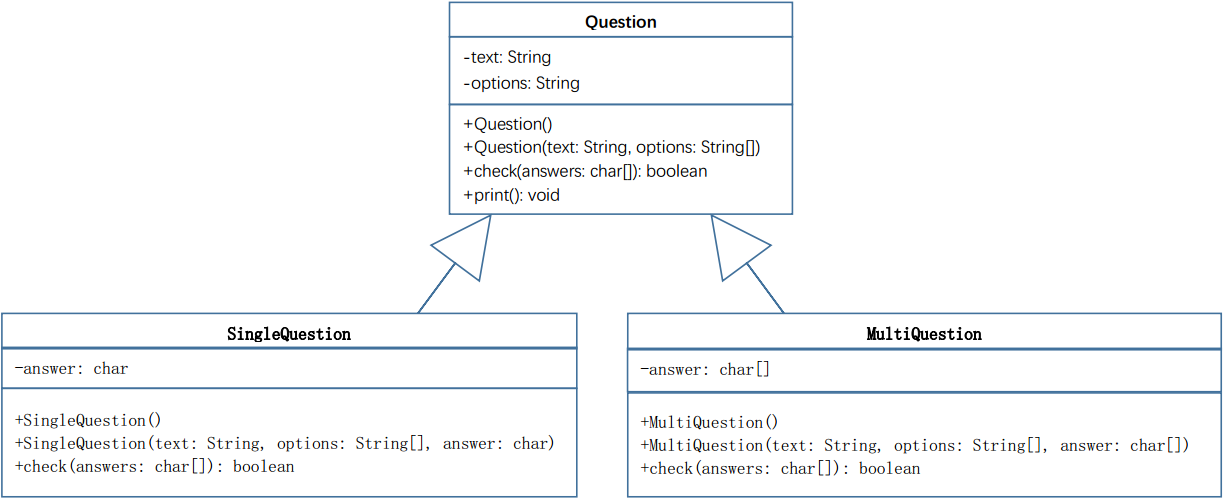
要求:
1)在MultiChoice类中实现参数为( String text, String[] options, char[] answers) 的构造方法。在SingleChoice实现参数为( String text, String[] options ,char answer) 的构造方法。
2)在MutiChie和SingleChoice 类中重写Question类chek0方法,分别实现多选题的验证答案和单选题的验证答案方法。
3)设计测试类进行考试、答题。
例如,初始化如下试题:
new MultiQuestion("三国演义中的三绝是谁?",new String[]{"A.曹操","B.刘备","C.关羽","D.诸葛亮"},new char[]{'A','C',D''});
new SingleQuestion("最早向刘备推荐诸葛亮的是谁?",new String[]{"A.徐庶","B.司马微","C.鲁肃","D.关羽"},'B');
考试的运行效果如下:
三国演义中的三绝是谁?
A.曹操? ? ?B.刘备? ? ?C.关羽? ? ? D.诸葛亮
请选择:cad
恭喜,答对了!
最早向刘备推荐诸葛亮的是谁?
A.徐庶? ? ?B.司马微? ? C.鲁肃? ? D.关羽
请选择:A
还得努力
说明:
1)用户输入答案时,大小写均应支持。提示:可以利用Character. toUpperCase()方法将用户输入的答案字符转换为大写宇母后再进行比较(标准答案为大写字母)。
2)判断单选题正误时,如果用户未答题,或者选项多于1个均视为答错。
3)判断多选题正误时,如果用户未答题,选项多或者少于标准答案,或者选项错误均视为答错(多选题答案需要连续输入,选项之间不能有空格、回车等字符)。
4)多选题允许用户输入的答案次序与标准答案不同,即只要正确选择了所有选项即可。
提示:可以利用Arrays. binarySearch()方法在答案数组中查询是否存在用户的选项。
?
import java.util.Scanner;
import java.util.Arrays;
abstract class Question {
String text;
String[] options = new String[4];
Question() {
}
Question(String text, String[] options) {
this.text = text;
this.options = options;
}
abstract boolean check(char[] answers);
void print() {
System.out.println(text);
System.out.println(options[0] + " " + options[1] + " " + options[2] + " " + options[3]);
System.out.println("请根据问题选择你认为正确的选项:");
}
}
class SingleQuestion extends Question {
char answer;
SingleQuestion(String text, String[] options, char answer) {
this.text = text;
this.options = options;
this.answer = answer;
}
boolean check(char[] answer) {
if (answer.length == 1) {
if (Character.toUpperCase(answer[0]) == this.answer)
return true;
else
return false;
} else
return false;
}
}
class MultiQuestion extends Question {
char[] answer = new char[4];
MultiQuestion(String text, String[] options, char[] answer) {
this.text = text;
this.options = options;
this.answer = answer;
}
boolean check(char[] answer) {
int count = 0;
for (int i = 0; i < answer.length; i++) {
int x = Arrays.binarySearch(this.answer, Character.toUpperCase(answer[i]));
if (x < 0)
return false;
else
count++;
}
if (count == 3)
return true;
else
return false;
}
}
public class Test{
public static void main(String[] args) {
SingleQuestion s = new SingleQuestion("最早向刘备推荐诸葛亮的是谁?", new String[] { "A.徐庶", "B.司马微", "C.鲁肃", "D.关羽" }, 'A');
MultiQuestion m = new MultiQuestion("三国演义中的三绝是谁?", new String[] { "A.曹操", "B.刘备", "C.关羽", "D.诸葛亮" }, new char[] { 'A', 'C', 'D' });
m.print();
Scanner sc1 = new Scanner(System.in);
String t1 = null;
t1 = sc1.nextLine();
char[] a = t1.toCharArray();
if (m.check(a))
System.out.println("恭喜,答对了!");
else
System.out.println("还得努力!");
s.print();
Scanner sc2 = new Scanner(System.in);
String t2 = null;
t2 = sc2.nextLine();
char[] b = t2.toCharArray();
if (s.check(b))
System.out.println("恭喜,答对了!");
else
System.out.println("还得努力!");
}
}