1.实现效果
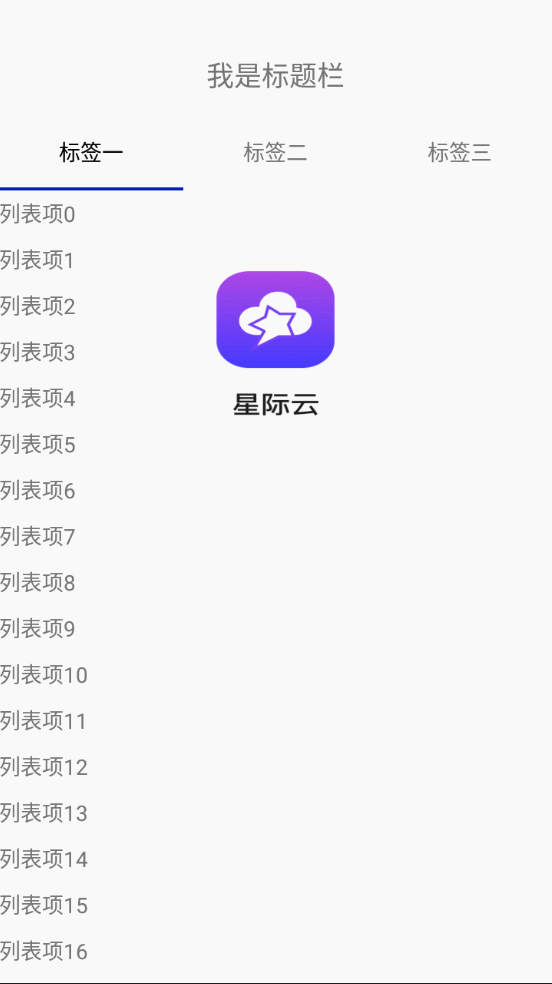
?2.布局文件
<android.support.design.widget.CoordinatorLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:id="@+id/coordinatorLayout"
android:layout_width="match_parent"
android:layout_height="match_parent">
<android.support.design.widget.AppBarLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
app:elevation="0dp"
android:background="@android:color/white">
<RelativeLayout
android:id="@+id/title_layout"
android:layout_width="match_parent"
android:layout_height="50dp"
app:layout_scrollFlags="scroll">
<TextView
android:id="@+id/tv"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_centerInParent="true"
android:text="我是标题栏"
android:textSize="18sp" />
</RelativeLayout>
<android.support.design.widget.TabLayout
android:id="@+id/tabLayout"
android:layout_width="match_parent"
android:layout_height="50dp"
android:background="@android:color/white"
app:tabMode="fixed"
app:tabSelectedTextColor="@android:color/black" />
</android.support.design.widget.AppBarLayout>
<android.support.v4.view.ViewPager
android:id="@+id/viewPager"
android:layout_width="match_parent"
android:layout_height="match_parent"
app:layout_behavior="@string/appbar_scrolling_view_behavior" />
</android.support.design.widget.CoordinatorLayout>
3.主函数
public class MainActivity3 extends AppCompatActivity {
private TabLayout tabLayout;
private ViewPager viewPager;
private FragmentPagerAdapter mPageAdapter;
private ArrayList<String> titleList = new ArrayList<>();
private ArrayList<Fragment> fragmentList=new ArrayList<>();
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main3);
initData();
initView();
}
private void initData(){
titleList.clear();
titleList.add("标签一");
titleList.add("标签二");
titleList.add("标签三");
fragmentList.clear();
fragmentList.add(new OneFragment());
fragmentList.add(new OneFragment());
fragmentList.add(new OneFragment());
mPageAdapter=new FragmentPagerAdapter(getSupportFragmentManager()) {
@Override
public Fragment getItem(int i) {
return fragmentList.get(i);
}
@Nullable
@Override
public CharSequence getPageTitle(int position) {
return titleList.get(position);
}
@Override
public int getCount() {
return titleList.size();
}
};
}
private void initView(){
tabLayout=findViewById(R.id.tabLayout);
viewPager=findViewById(R.id.viewPager);
viewPager.setAdapter(mPageAdapter);
tabLayout.setupWithViewPager(viewPager);
}
}
4.OneFragment
public class OneFragment extends Fragment {
private RecyclerView mRecyclerView;
private RecyclerView.LayoutManager mLayoutManager;
private RecyclerViewAdapter mAdapter;
private SwipeRefreshLayout swipeRefreshLayout;
private View mainView;
@Nullable
@Override
public View onCreateView(@NonNull LayoutInflater inflater, @Nullable ViewGroup container,
@Nullable Bundle savedInstanceState) {
mainView = inflater.inflate(R.layout.fragment_one, container, false);
initView();
return mainView;
}
private void initView() {
mRecyclerView = mainView.findViewById(R.id.recyclerview);
swipeRefreshLayout=mainView.findViewById(R.id.swipeRefreshLayout);
mLayoutManager = new LinearLayoutManager(getActivity(),
LinearLayoutManager.VERTICAL, false);
ArrayList<String> data = new ArrayList<>();
for (int i = 0; i < 40; i++) {
data.add("列表项" + i);
}
mAdapter = new RecyclerViewAdapter(getActivity(), data);
mRecyclerView.setLayoutManager(mLayoutManager);
mRecyclerView.setAdapter(mAdapter);
swipeRefreshLayout.setOnRefreshListener(new SwipeRefreshLayout.OnRefreshListener() {
@Override
public void onRefresh() {
new Handler().postDelayed(new Runnable() {
@Override
public void run() {
swipeRefreshLayout.setRefreshing(false);
Toast.makeText(getActivity(),"刷新完成",Toast.LENGTH_SHORT).show();
}
},2000);
}
});
}
}
5.OneFragment布局文件
<android.support.v4.widget.SwipeRefreshLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/swipeRefreshLayout"
android:layout_width="match_parent"
android:layout_height="match_parent">
<android.support.v7.widget.RecyclerView
android:id="@+id/recyclerview"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_centerInParent="true" />
</android.support.v4.widget.SwipeRefreshLayout>
6.RecyclerViewAdapter
public class RecyclerViewAdapter extends RecyclerView.Adapter<RecyclerViewAdapter.ViewHolder> {
public class ViewHolder extends RecyclerView.ViewHolder {
public TextView title;
public ViewHolder(@NonNull View itemView) {
super(itemView);
title = itemView.findViewById(R.id.tv_title);
}
}
private ArrayList<String> mDatas;
private final Context context;
public RecyclerViewAdapter(Context context, ArrayList<String> mDatas) {
this.context = context;
this.mDatas = mDatas;
}
@NonNull
@Override
public ViewHolder onCreateViewHolder(@NonNull ViewGroup viewGroup, int i) {
View view = LayoutInflater.from(context).inflate(R.layout.recyclerview_item,null);
return new ViewHolder(view);
}
@Override
public void onBindViewHolder(@NonNull ViewHolder viewHolder, final int i) {
viewHolder.title.setText(mDatas.get(i));
}
@Override
public int getItemCount() {
return mDatas.size();
}
}
7.recyclerview_item
<LinearLayout
xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="wrap_content">
<TextView
android:id="@+id/tv_title"
android:gravity="center"
android:text="aaa"
android:layout_width="match_parent"
android:layout_height="@dimen/dp_30"/>
</LinearLayout>
|