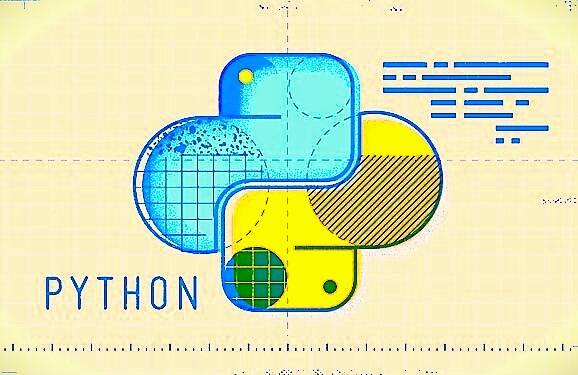
python常用的字符串操作
- 记录python中字符串的操作,以后有了其他的操作会在这个文件中追加
目录
- 主函数
- 字符串相加
- N个字符串相加
- 字符串替换
- 循环读取字符串
- 字符串截取
- 字符串查找
-
以下所以函数调用的main函数,结果都是基于主函数中的字符串得出的 if __name__ == "__main__":
str1 = "Yuang"
str2 = "PangZi"
str3 = "YuangPangZi"
str_list = [str1, str2, str3]
StrAdd(str1, str2)
StrSub(str3, str1)
StrAddList(str_list)
LoopRead(str1)
StrSplit(str3)
FindStr(str3, str1)
FindStr2(str3, str1)
-
字符串相加 def StrAdd(str1:str, str2:str):
res = str1 + str2
print("StrAdd: ",res)
return res
StrAdd: YuangPangZi
-
N个字符串相加 def StrAddList(*args):
res = ""
for s in args[0]:
if isinstance(s, str):
res += s
print("StrAddList: ", res)
return res
StrAddList: YuangPangZiYuangPangZi
-
字符串替换 def StrSub(source:str, str1:str):
res = source.replace(str1, "")
print("StrSub: ", res)
return res
StrSub: PangZi
-
循环读取字符串 def LoopRead(source:str):
for i in source:
print("Loop Str: ", i)
Loop Str: Y
Loop Str: u
Loop Str: a
Loop Str: n
Loop Str: g
-
字符串截取 def StrSplit(source:str):
print("get one char:", source[0], source[1])
print("get sting in range:", source[0:2])
print("get char in range for behand:", source[-3: -1])
print("get one char:", source[-1], source[-2])
get one char: Y u
get sting in range: Yu
get char in range for behand: gZ
get one char: i Z
-
字符串查找
def FindStr(source:str, tag:str):
if tag in source:
print("True")
return True
else:
print("False")
return False
True
import re
def FindStr2(source:str, tag:str):
res = re.findall(tag, source)
print("res:", res)
print("res len", len(res))
res: ['Yuang']
res len 1
s = re.findall(tag, source) print(“res:”, res) print(“res len”, len(res))
```shell
res: ['Yuang']
res len 1
|