例如:
- 思路 通过自定义注解,标注实体类上并设置列表头,反射获取里面值通过apache.poi 这个jar生成Excel表
提示:默认Dao取出来数据List<T> 这种类型数据 啥也不说直接开搞
实现步骤:
提示:环境 springboot 和maven
例如:
- 使用Maven导入jar包
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>poi</artifactId>
<version>5.2.2</version>
</dependency>
- 自定义注解
@Documented
@Retention(RetentionPolicy.RUNTIME)
@Target(ElementType.FIELD)
public @interface ExcelHead {
public String value() default "默认设置列头";
}
-
标注实体类后效果 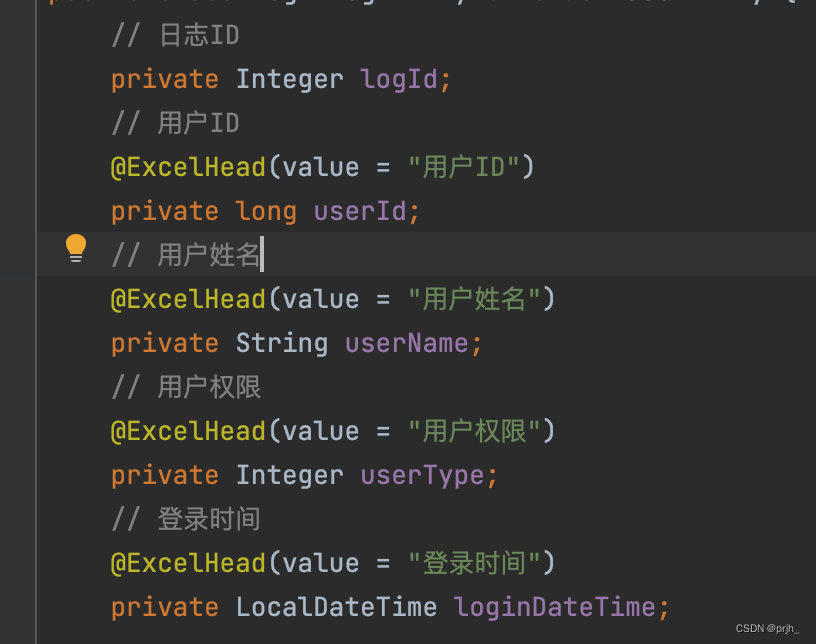 -
封装工具类
@Component
public class ExcelUtil<T> {
public void createExcel(String savePath, String listName, List<T> list, int columnWidth, int rowHeight, Class target) throws Exception {
FileOutputStream outputStream = null;
HSSFWorkbook workbook = new HSSFWorkbook();
HSSFSheet sheet = workbook.createSheet(listName);
setHead(target, sheet, columnWidth, rowHeight);
int i = 1;
for (T t : list) {
HSSFRow row = sheet.createRow(i++);
row.setHeight((short) (rowHeight * 10));
Class<?> aClass = t.getClass();
Field[] declaredFields = aClass.getDeclaredFields();
int j = 0;
for (Field field : declaredFields) {
field.setAccessible(true);
if (field.getAnnotation(ExcelHead.class) == null){
continue;
}
Object o = field.get(t);
HSSFCell cell = row.createCell(j++);
cell.setCellValue(o.toString());
}
}
try {
outputStream = new FileOutputStream(savePath);
workbook.write(outputStream);
} catch (Exception e) {
e.printStackTrace();
} finally {
close(outputStream);
}
}
public void setHead(Class target, HSSFSheet sheet, int ColumnWidth, int rowHeight) {
HSSFRow row = sheet.createRow(0);
Field[] fields = target.getDeclaredFields();
int i = 0;
for (Field field : fields) {
field.setAccessible(true);
if (field.getAnnotation(ExcelHead.class) == null) {
continue;
}
ExcelHead annotation = field.getAnnotation(ExcelHead.class);
String value = annotation.value();
HSSFCell cell = row.createCell(i++);
sheet.setColumnWidth(i, ColumnWidth * 256);
row.setHeight((short) (rowHeight * 10));
cell.setCellValue(value);
}
}
public void close(FileOutputStream fileOutputStream) {
if (fileOutputStream != null) {
try {
fileOutputStream.flush();
fileOutputStream.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
public void createExcel(String savePath, String listName, List<T> list, Class target) throws Exception {
this.createExcel(savePath, listName, list, 20, 30, target);
}
}
- 最后完成业务层
@Service
public class CsvServiceImpl implements CsvService{
@Autowired
ExcelUtil<LoginLogEntity> excelUtil;
@Override
public void getCSVFile(HttpServletResponse response, HttpSession session) throws Exception{
List<LoginLogEntity> list = (List<LoginLogEntity>)session.getAttribute("userLog");
String savePath = "./src/main/resources/static/用户日志表.xls";
try {
excelUtil.createExcel(savePath,"用户日志表",list,LoginLogEntity.class);
}catch (Exception e){
e.printStackTrace();
}
FileInputStream inputStream = null;
ServletOutputStream stream = null;
try {
String filename = "日志表.xls";
String filename1 = new String(filename.getBytes("utf-8"),"ISO-8859-1");
response.addHeader("content-type","application/x-msdownload");
response.setContentType("application/octet-stream");
response.addHeader("Content-Disposition","attachment;filename="+filename1);
File file = new File("./src/main/resources/static/用户日志表.xls");
if (!file.exists()){
throw new RuntimeException("文件不存在");
}
inputStream = new FileInputStream(file);
stream = response.getOutputStream();
byte[] bytes = new byte[200];
int len = 0;
while ((len = inputStream.read(bytes)) != -1){
stream.write(bytes,0,len);
}
}finally {
if (stream != null){
stream.flush();
stream.close();
}
if (inputStream != null){
inputStream.close();
}
}
}
}
|