2D游戏跳跃下落速度问题
有些2D游戏会感觉到向上跳跃和下落速度不一致,感觉下落时更干脆一些,比如马里奥,不过也跟具体的项目需求手感有关系。 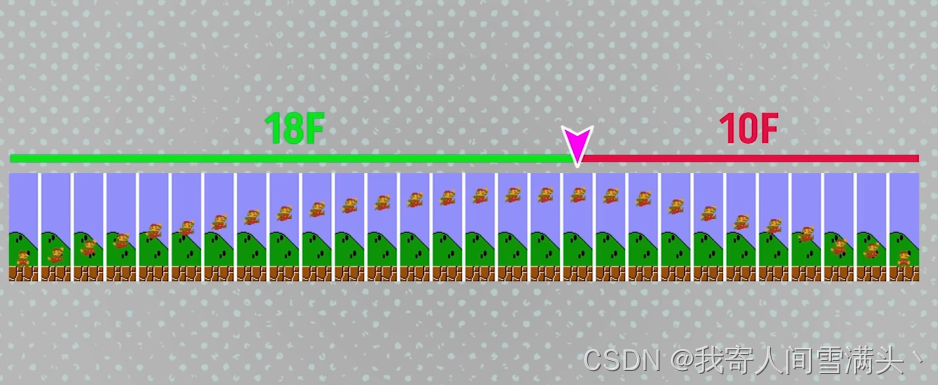 直接上代码。下面是优化下落速度手感的代码。
using UnityEngine;
public class BettlerJump : MonoBehaviour
{
public float fallMultiplier = 2.5f;
public float lowJumpMultiplier = 2f;
bool isPressJump;
Rigidbody2D rb;
private void Awake()
{
rb = GetComponent<Rigidbody2D>();
}
public void Update()
{
if(Input.GetButton("Jump"))
{
isPressJump = true;
}
else
{
isPressJump = false;
}
}
public void FixedUpdate()
{
if(rb.velocity.y < 0)
{
rb.velocity += Vector2.up * Physics2D.gravity.y * (fallMultiplier - 1) * Time.deltaTime;
}
else if (rb.velocity.y > 0 && !isPressJump)
{
rb.velocity += Vector2.up * Physics2D.gravity.y * (lowJumpMultiplier - 1) * Time.deltaTime;
}
}
}
跳跃手感优化
如果操作都放在Update中有时会出现跳跃手感不一致的情况。 优化跳跃手感的核心思路就是按键检测放在Update中,物理相关的放到FixedUpdate中。 代码示例
public Transform groundCheck;
public LayerMask ground;
public float jumpForce;
public bool isGround, isJump, isJumpPressed;
private void Update()
{
if(Input.GetButtonDown("Jump"))
{
isJumpPressed = true;
}
}
private void FixedUpdate()
{
isGround = Physics2D.OverlapCircle(groundCheck.position, 0.1f, ground);
if (isJumpPressed && isGround)
{
rb.velocity = new Vector2(rb.velocity.x, jumpForce);
isJumpPressed = false;
}
}
|