0、演示
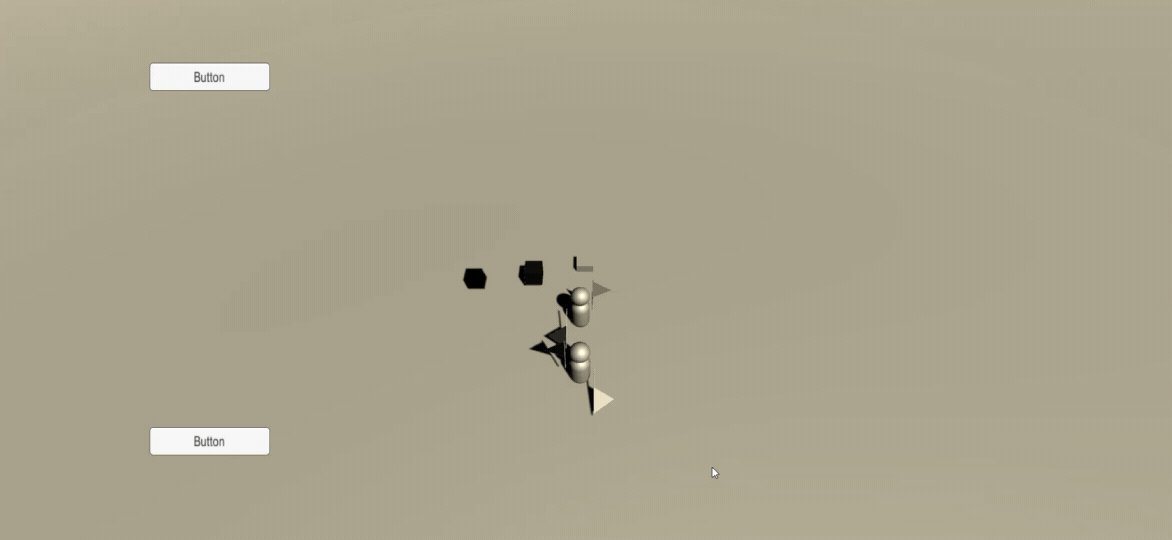
1、定义变量
public Camera theCamera;
private GameObject Element;
private bool clicked = false;
private bool is_element = false;
Vector3 newWorldPos;
2、点击发出射线,判断是否命中tag为elelment的控件
if (!clicked && Input.GetMouseButtonDown(0))
{
Ray ray = theCamera.ScreenPointToRay(Input.mousePosition);
RaycastHit hit;
if (Physics.Raycast(ray, out hit) && hit.collider.tag == "element")
{
Element = hit.collider.gameObject;
Element.GetComponent<Collider>().enabled = false;
is_element = true;
clicked = true;
}
}
3、获取控件后,按住鼠标左键移动控件,移动的新位置为相机射线与tag为Plane或者element的控件碰撞的位置
if (clicked && Input.GetMouseButton(0))
{
if (is_element)
{
Ray ray = theCamera.ScreenPointToRay(Input.mousePosition);
RaycastHit hit;
if (Physics.Raycast(ray, out hit) && (hit.collider.tag == "Plane"|| hit.collider.tag == "element"))
{
newWorldPos = hit.point;
newWorldPos.y = newWorldPos.y+1.5f;
Element.transform.position = newWorldPos;
}
}
}
4、松开左键,放下物体
if (clicked && Input.GetMouseButtonUp(0) )
{
newWorldPos.y = 0.77f;
Element.transform.position = newWorldPos;
Element.GetComponent<Collider>().enabled = true;
is_element = false;
clicked = false;
Element = null;
}
注意:要自己设置tag
5、完整代码
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class move_controller2 : MonoBehaviour
{
public Camera theCamera;
private GameObject Element;
private bool clicked = false;
private bool is_element = false;
Vector3 newWorldPos;
void Start()
{
}
void Update()
{
if (!clicked && Input.GetMouseButtonDown(0))
{
Ray ray = theCamera.ScreenPointToRay(Input.mousePosition);
RaycastHit hit;
if (Physics.Raycast(ray, out hit) && hit.collider.tag == "element")
{
Element = hit.collider.gameObject;
Element.GetComponent<Collider>().enabled = false;
is_element = true;
clicked = true;
}
}
if (clicked && Input.GetMouseButton(0))
{
if (is_element)
{
Ray ray = theCamera.ScreenPointToRay(Input.mousePosition);
RaycastHit hit;
if (Physics.Raycast(ray, out hit) && (hit.collider.tag == "Plane"|| hit.collider.tag == "element"))
{
newWorldPos = hit.point;
newWorldPos.y = newWorldPos.y+1.5f;
Element.transform.position = newWorldPos;
}
}
}
if (clicked && Input.GetMouseButtonUp(0) )
{
newWorldPos.y = 0.77f;
Element.transform.position = newWorldPos;
Element.GetComponent<Collider>().enabled = true;
is_element = false;
clicked = false;
Element = null;
}
}
}
|