提示:文章写完后,目录可以自动生成,如何生成可参考右边的帮助文档
数据持久化
前言
提示:在使用unity制作游戏时,经常需要对数据进行存储与读取(例如:角色的属性) 我们刚开始的时候接触的应该是unity提供的PlayerPrefs(通过过key-value的形式写入、读取、更新),在这里记录一下如何使用二进制、XML、JSON来实现:
一、PlayerPrefs
PlayerPrefs 是unity的内置的方法,用来储存、读取一些数据(计分板、排名) 使用Set来保存数据(长用于保存的类型:int float string bool) PlayerPrefs.SetInt(key,value); PlayerPrefs.SetFloat(); Playerprefs.SetString(); 用0、1来实现bool值的存储
例如:
PlayerPrefs.SetString(“Name”,mName);
PlayerPrefs.SetInt(“Age”,mAge);
PlayerPrefs.SetFloat(“Grade”,mGrade);
PlayerPrefs.SetInt(“isTrue”,1); //标记0、1做bool值
数据的读取(常使用Get方法)
例如:
String mName=PlayerPrefs.GetString(“Name”);
Int mAge=PlayerPrefs.GetInt(“Age”);
Float mGrade=PlayerPrefs.GetFloat(“Grade”);
注意:Unity中值是通过键名来读取的,当值不存在时,返回默认值。
二、二进制
二进制
序列化: 通过将Object (对象,脚本,数据)转化为字节流写入二进制文件
反序列化: 将二进制文件解析为object(对象,脚本,数据)
代码如下:
using System.IO;
using System.Runtime.Serialization.Formatters.Binary;
using UnityEngine.UI;
using System;
public void Save()
{
BinaryFormatter bf = new BinaryFormatter();
FileStream file = File.Create(Application.dataPath + "/StudentInfo.dat");
StudentData data = new StudentData();
data.SchoolName = Schoolfield.text;
data.peofessionName = Professionfield.text;
data.ClassName = Classfield.text;
data.Name = Namefield.text;
bf.Serialize(file, data);
file.Close();
}
public void Load()
{
if(File.Exists(Application.dataPath+ "/StudentInfo.dat"))
{
BinaryFormatter bf = new BinaryFormatter();
FileStream file = File.Open(Application.dataPath+"/StudentInfo.dat", FileMode.Open);
StudentData data = new StudentData();
data = bf.Deserialize(file) as StudentData;
file.Close();
Schoolfield.text=data.SchoolName ;
Professionfield.text=data.peofessionName;
Classfield.text=data.ClassName;
Namefield.text=data.Name;
}
else{
DeBug.Log("加载数据错误");
}
}
[Serializable]
class StudentData
{
public string SchoolName;
public string peofessionName;
public string ClassName;
public string Name;
}
二进制方法(简单,但可读性差):序列化:新建或打开一个二进制文件,通过二进制格式器将对象写入该二进制文件 反序列化:打开反序列化的二进制文件,通过二进制格式器将文件解析成对象 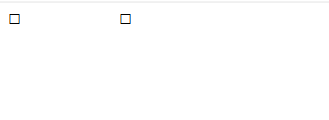 创建出来的二进制文件如上(乱码,看不懂)
三、XML
XML: 扩展标记语言 可以用来标记数据、定义数据类型。
序列化与反序列化的方式与二进制方法十分相似
C# 读取XML文件的方法:
- XmlDocument(把数据加载到内存中,比较耗内存,方便读取)
- XmlTextReader(以流形式加载,内存占用更少,单向只读,使用不方便)
XML文件格式: 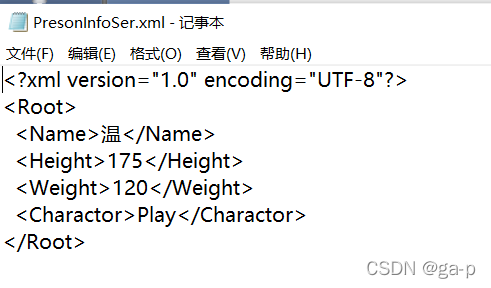 代码如下:
using System;
using System.IO;
using UnityEngine.UI;
using System.Xml;
public class PresonInfoSer : MonoBehaviour {
public InputField NameText;
public InputField HeightText;
public InputField WeightText;
public InputField CharText;
public Button SaveBtn;
public Button LoadBtn;
private string path;
private Dictionary<string, string> DataDic = new Dictionary<string, string>();
void Awake()
{
ReadXml();
}
public void Save()
{
DataDic.Add("Name", NameText.text);
DataDic.Add("Height",HeightText.text.ToString());
DataDic.Add("Weight", WeightText.text.ToString());
DataDic.Add("Charactor", CharText.text);
path = Application.persistentDataPath + "/PresonInfoSer.xml";
Debug.Log("文件被存储在"+path);
XmlDocument xml = new XmlDocument();
XmlDeclaration xmlDec = xml.CreateXmlDeclaration("1.0", "UTF-8", null);
xml.AppendChild(xmlDec);
XmlElement rootNode = xml.CreateElement("Root");
xml.AppendChild(rootNode);
foreach(KeyValuePair<string,string> pair in DataDic)
{
XmlElement element = xml.CreateElement(pair.Key);
element.InnerText = pair.Value;
rootNode.AppendChild(element);
}
xml.Save(path);
}
public void ReadXml()
{
path = Application.persistentDataPath + "/PresonInfoSer.xml";
XmlReader reader = XmlReader.Create(path);
while (reader.Read())
{
if (reader.IsStartElement("Name"))
{
NameText.text = reader.ReadElementContentAsString();
}
if (reader.IsStartElement("Height"))
{
HeightText.text = reader.ReadElementContentAsString();
}
if (reader.IsStartElement("Weight"))
{
WeightText.text = reader.ReadElementContentAsString();
}
if (reader.IsStartElement("Charactor"))
{
CharText.text = reader.ReadElementContentAsString();
}
}
reader.Close();
}
}
[Serializable]
class PeopleInfo{
public string Name;
public float Height;
public float Weight;
public string Charactor;
}
四、JSON
通过JSON来读写(利用插件LitJson)
# JSON官方介绍:
JSON 是轻量级的文本数据交换格式 JSON 独立于语言 * JSON 具有自我描述性,更易理解 * JSON 使用 JavaScript 语法来描述数据对象,但是 JSON 仍然独立于语言和平台。JSON 解析器和 JSON 库支持许多不同的编程语言。
JSON(数据格式简单,易于读写,但是不够直观,可读性比XML差):是一种语言无关的发送和接受数据的常用格式。可以使用它来跨平台的传输数据。
Json是目前的使用较为频繁、方便的,推荐使用
Json文件(字符串): 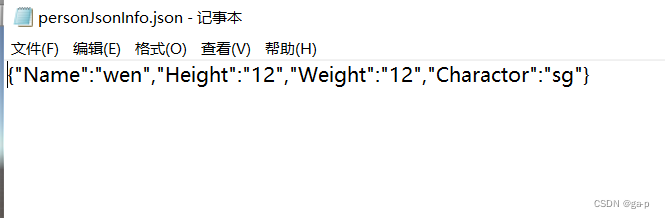 将LitJson.dill引入项目中: 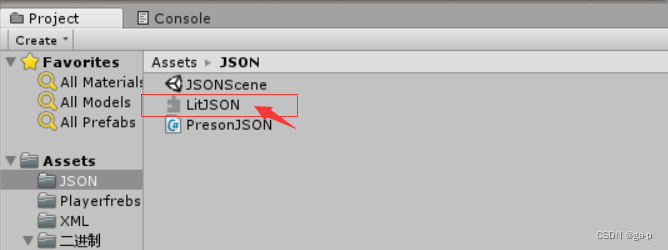 没有的小伙伴点击下面的链接(免费下载):
LitJson下载地址
存储和加载Json文件:
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.UI;
using System.IO;
using LitJson;
public class PresonJSON : MonoBehaviour {
public InputField NameText;
public InputField HeightText;
public InputField WeightText;
public InputField CharText;
public Button SaveBtn;
public Button LoadBtn;
private string path;
personList list = new personList();
void Awake()
{
Load();
}
void Start () {
}
void Update () {
}
void WriteJSON(personInfo_JSON person,string path)
{
if (!File.Exists(path))
{
list.data.Add("Name",person.Name);
list.data.Add("Height",person.Height.ToString());
list.data.Add("Weight",person.Weight.ToString());
list.data.Add("Charactor", person.Charactor);
}
else
{
list.data["Name"] = person.Name;
list.data["Height"] = person.Height.ToString();
list.data["Weight"] = person.Weight.ToString();
list.data["Charactor"] = person.Charactor;
}
string jsonStr = JsonMapper.ToJson(list.data);
File.WriteAllText(path, jsonStr);
}
public void Save()
{
personInfo_JSON js = new personInfo_JSON();
js.Name = NameText.text;
js.Height = float.Parse(HeightText.text);
js.Weight = float.Parse(WeightText.text);
js.Charactor = CharText.text;
path = Application.persistentDataPath + "/" + "personJsonInfo.json";
WriteJSON(js, path);
}
public void Load()
{
path = Application.persistentDataPath + "/" + "personJsonInfo.json";
if (File.Exists(path))
{
string Reader = File.ReadAllText(path);
JsonData jsonData = JsonMapper.ToObject(Reader);
NameText.text = jsonData["Name"].ToString();
HeightText.text = jsonData["Height"].ToString();
WeightText.text = jsonData["Weight"].ToString();
CharText.text = jsonData["Charactor"].ToString();
}
else
{
Debug.Log("读取数据错误");
}
}
}
public class personInfo_JSON{
public string Name;
public float Height;
public float Weight;
public string Charactor;
}
public class personList
{
public Dictionary<string, string> data = new Dictionary<string, string>();
}
总结
以上就是本人理解的存储读取数据内容的基本方法,在此总结一下,有错误请指出,感谢。
|