效果图
?
?
1. 首先创建两个文件夹
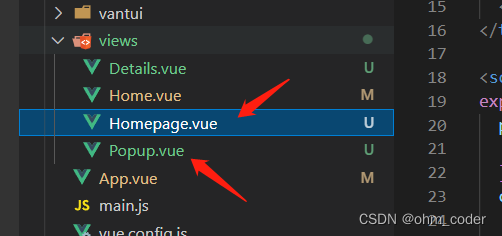
2. 在路由表中配置路由
?
import Vue from 'vue'
import VueRouter from 'vue-router'
import Homepage from '../views/Homepage.vue' //引入
Vue.use(VueRouter)
const routes = [
{
path: '/',
redirect: "/homepage"
},
{
path: '/homepage',
name: 'Homepage',
component: Homepage,
children: [
{
path: 'popup', //这里记得不要有/杆,(就按这样写就行) 要么写全 /homepage/popup
//因为以'/'开头的嵌套路径会被当作根路径,所以子路由上不用加'/';在生成路由时,主路由上的path会被自动添加到子路由之前,所以子路由上的path不用在重新声明主路由上的path了
component: () => import(/* webpackChunkName: "popup" */ '../views/Popup.vue')
}
]
},
]
// 重定向bug修复
const originalPush = VueRouter.prototype.push
VueRouter.prototype.push = function push(location, onResolve, onReject) {
if (onResolve || onReject) return originalPush.call(this, location, onResolve, onReject)
return originalPush.call(this, location).catch(err => err)
}
const router = new VueRouter({
routes
})
export default router
3. 实现淡入淡出的过渡效果
3.1 首先可以去Vue的官网看下<Transition><Transition />这个标签如何使用??Vue.js
3.2 写一个宽100px;高100px的盒子,再对盒子做一个显示控制隐藏的v-show判断
?视图层
<div class="mymodal" v-show="showModal"></div>
数据层
data () {
return {
showModal:false
}
},
methods: {
showPopup () {
this.showModal = !this.showModal
}
},
?css
.mymodal{
width: 100px;
height: 100px;
background: skyblue;
}
/*
fade-enter 淡入的初始位置 与 fade-leave-to 淡出的终止位置一样 opacity 0;
fade-enter-to 淡出的初始位置 与 fade-leave 淡入的终止位置一样 opacity 1;
*/
// 首页内容的淡入淡出
.fade-enter, .fade-leave-to{
opacity: 0;
}
//中间过渡效果
.fade-enter-active,.fade-leave-active{
transition: opacity .5s linear;
}
.fade-enter-to, .fade-leave{
opacity: 1;
}
?3.3初步效果
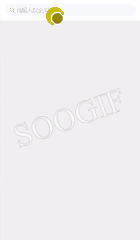
?4. 跳转到popup页面
methods: {
showPopup () {
this.showModal = !this.showModal;
this.$router.push("/homepage/popup") //路由跳转
}
},
4.1 popup页面的css样式 (因为我想让它从右边往左边跳)
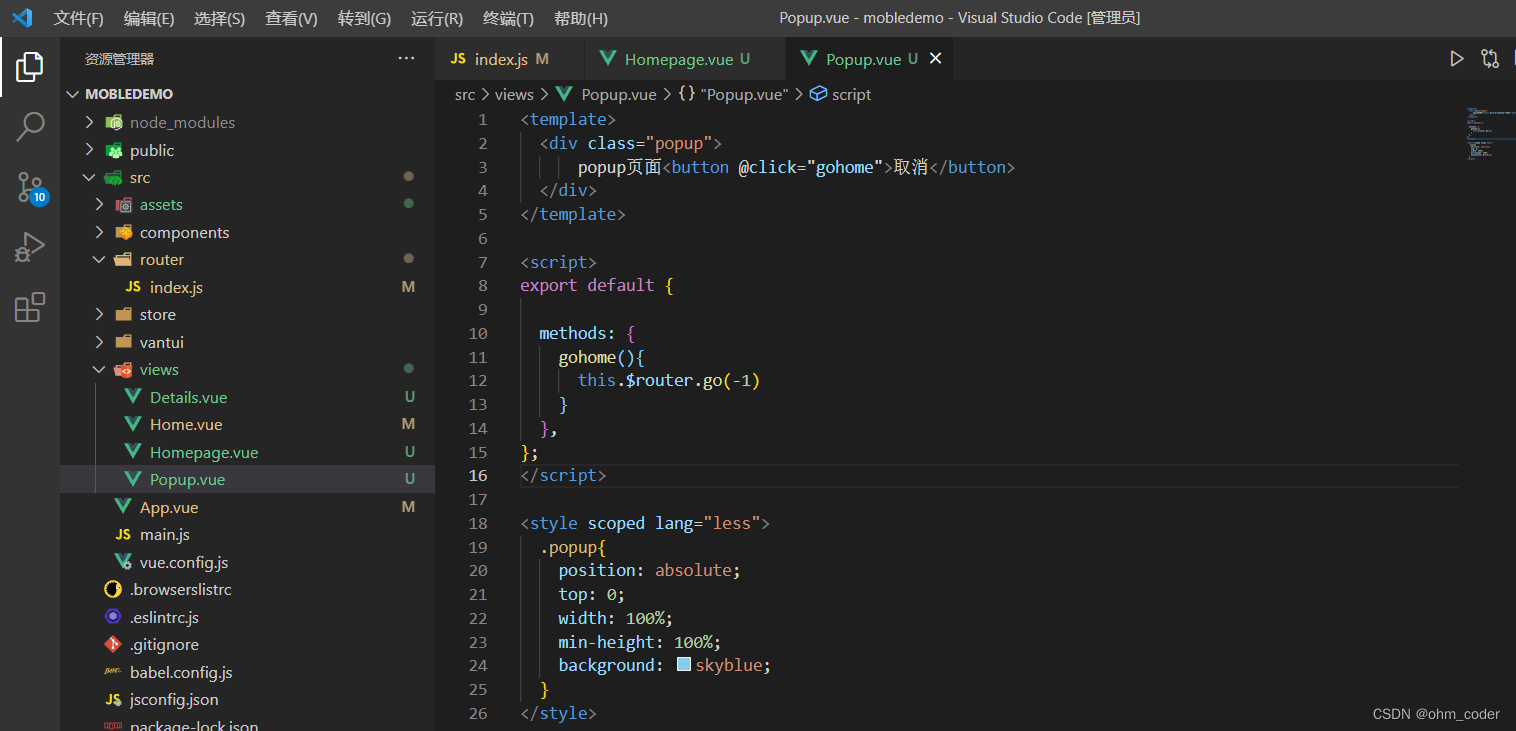
.popup{
position: absolute;
top: 0;
width: 100%;
min-height: 100%;
background: #efefef;
}
5. 给 <router-view>标签添加滑动效果
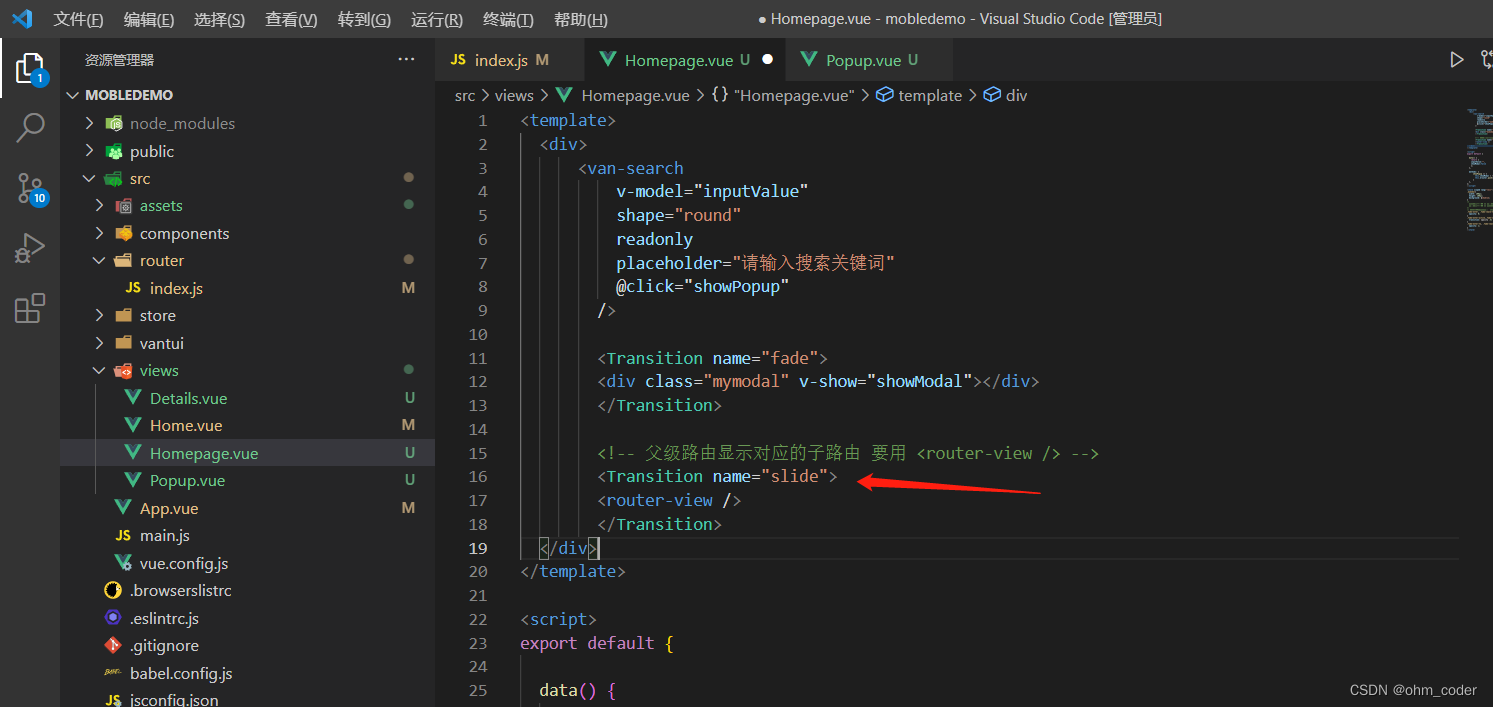
?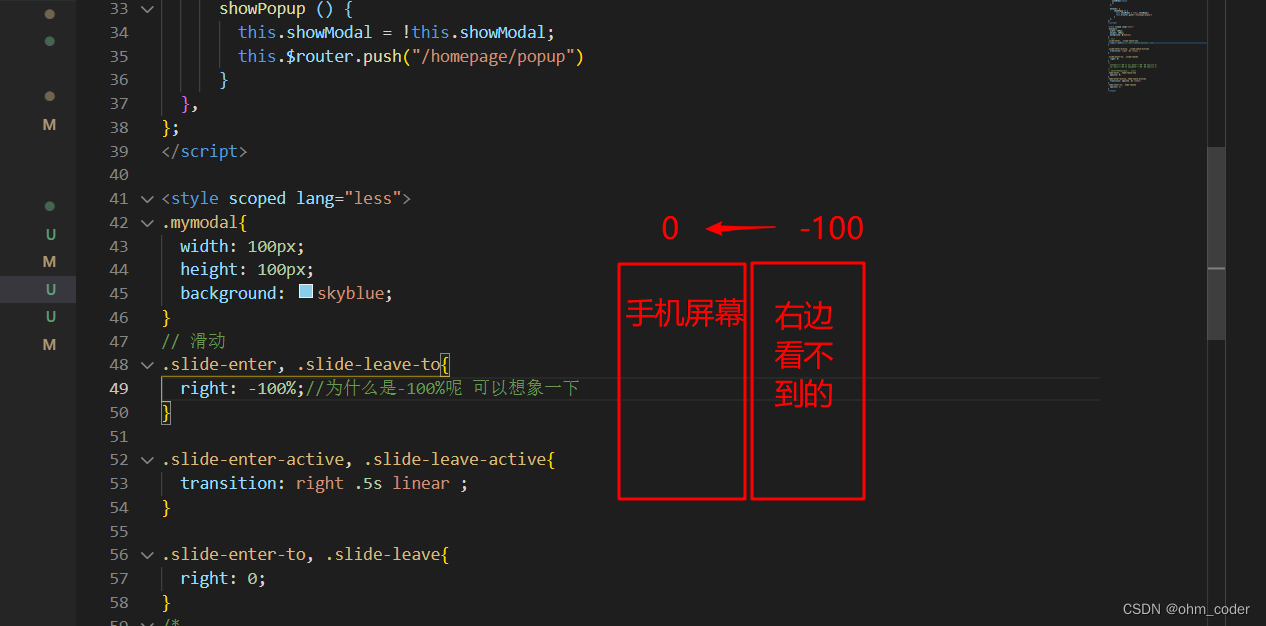
?
?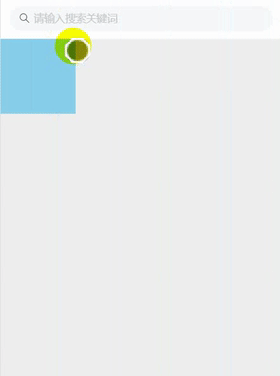
?5.1 效果实现了,但是有个bug,回来的时候小skyblue方块无法隐藏
我们可以利用路由的路径来控制显示隐藏? 不需要 showModal 我们定义的这个来作为控制了
然后通过打印路由
methods: {
showPopup () {
this.$router.push("/homepage/popup")
console.log(this.$route);
}
},
可以得到?
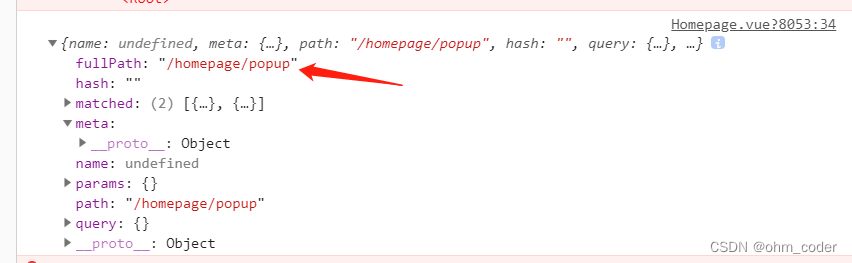
?那么我们可以在这里判断
<Transition name="fade">
<div class="mymodal" v-show="$route.fullPath=='/homepage/popup'"></div>
</Transition>
6.? 最后完成效果图
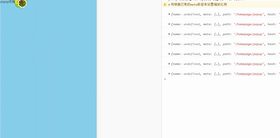
?7. 主要代码
<template>
<div>
<van-search
v-model="inputValue"
shape="round"
readonly
placeholder="请输入搜索关键词"
@click="showPopup"
/>
<Transition name="fade">
<div class="mymodal" v-show="$route.fullPath=='/homepage/popup'"></div>
</Transition>
<!-- 父级路由显示对应的子路由 要用 <router-view /> -->
<Transition name="slide">
<router-view />
</Transition>
</div>
</template>
<script>
export default {
data() {
return {
inputValue:"",
};
},
methods: {
showPopup () {
this.$router.push("/homepage/popup")
console.log(this.$route);
}
},
};
</script>
<style scoped lang="less">
.mymodal{
width: 100%;
height: 100%;
position: fixed;
left: 0;
top: 0;
background: #000;
}
// 滑动
.slide-enter, .slide-leave-to{
right: -100%;//为什么是-100%呢 可以想象一下
}
.slide-enter-active, .slide-leave-active{
transition: right .5s linear ;
}
.slide-enter-to, .slide-leave{
right: 0;
}
/*
淡入的初始位置 与 淡出的终止位置一样 opacity 0;
淡出的初始位置 与 淡入的终止位置一样 opacity 1;
*/
// 首页内容的淡入淡出 start
.fade-enter, .fade-leave-to{
opacity: 0;
}
.fade-enter-active,.fade-leave-active{
transition: opacity .5s linear;
}
.fade-enter-to, .fade-leave{
opacity: 1;
}
</style>
|