一、用户签到:BitMap功能
代表某个用户某一天的签到记录;如果有1000万用户,平均没人每年签到10次,一年则为1亿条;数据庞大;也耗内存;
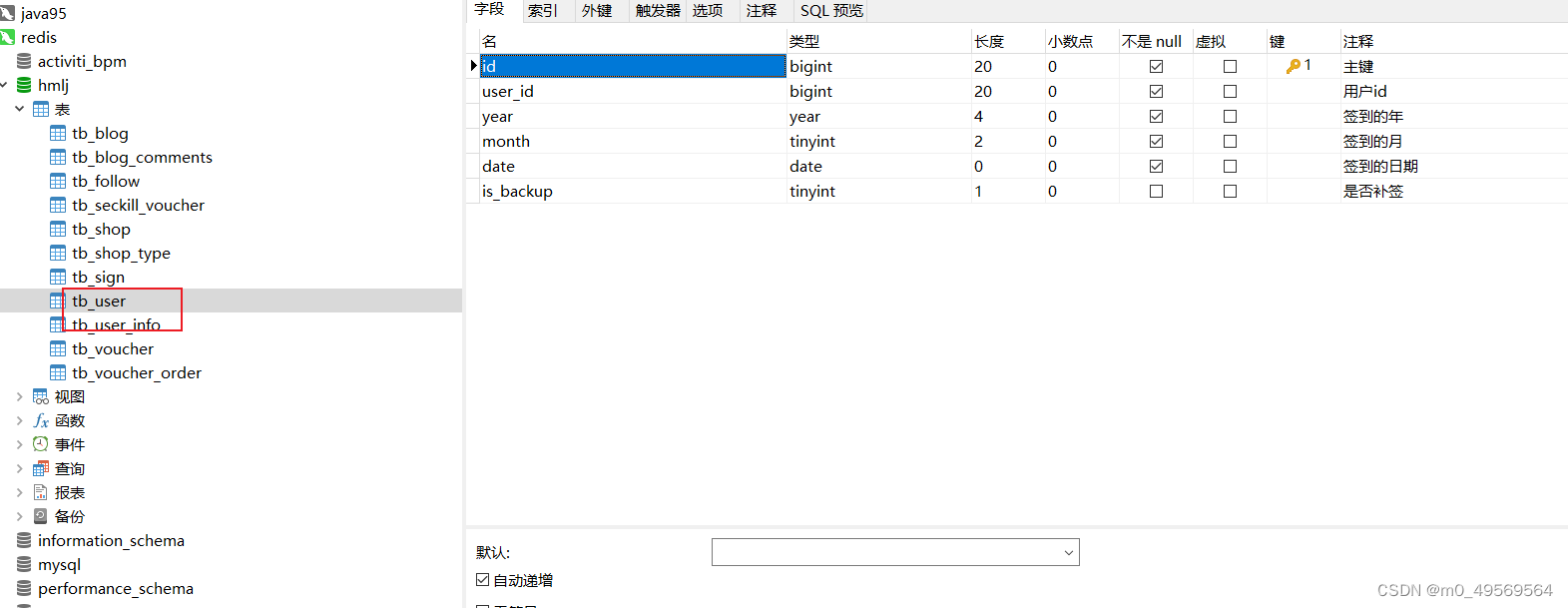 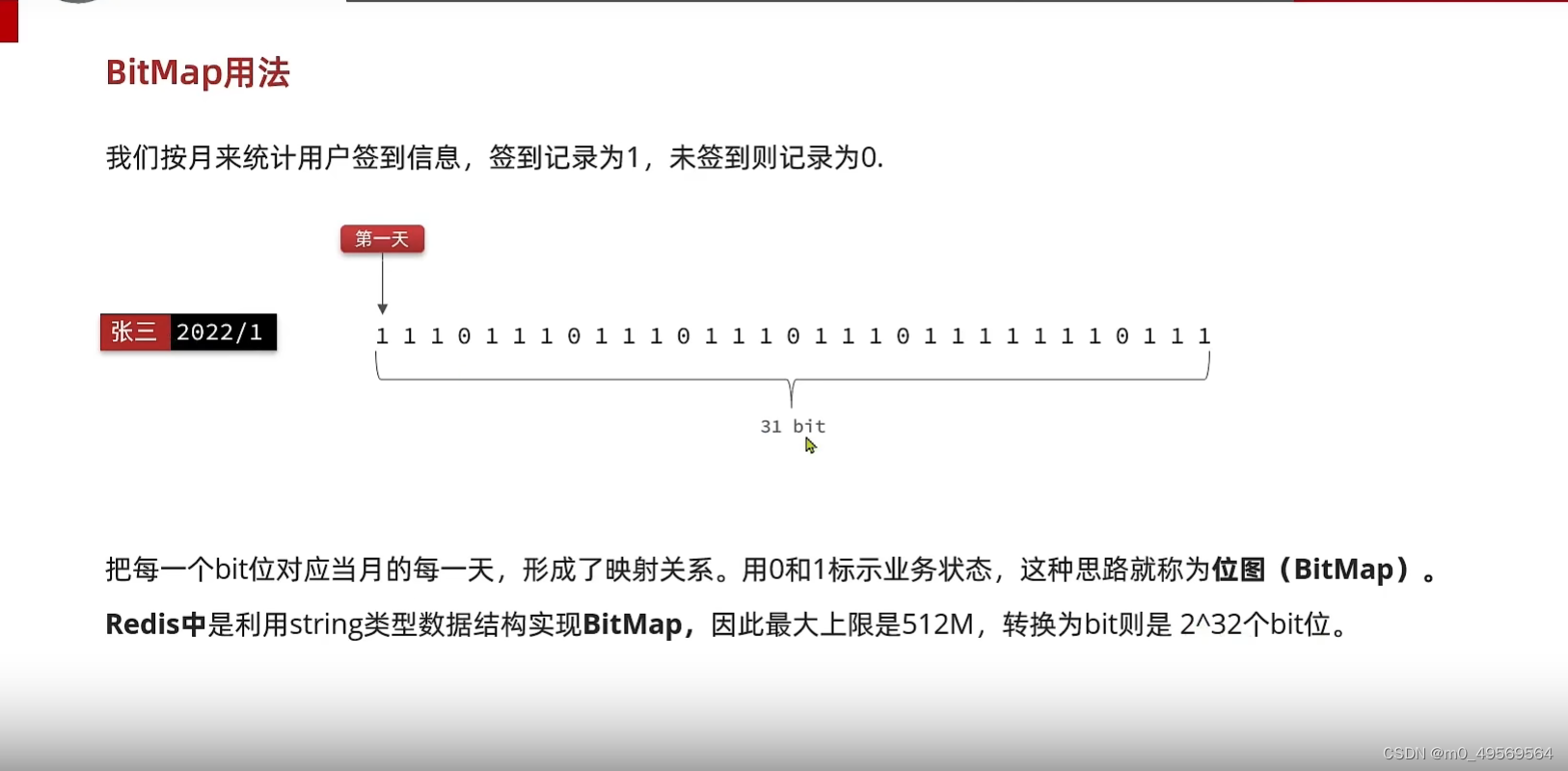 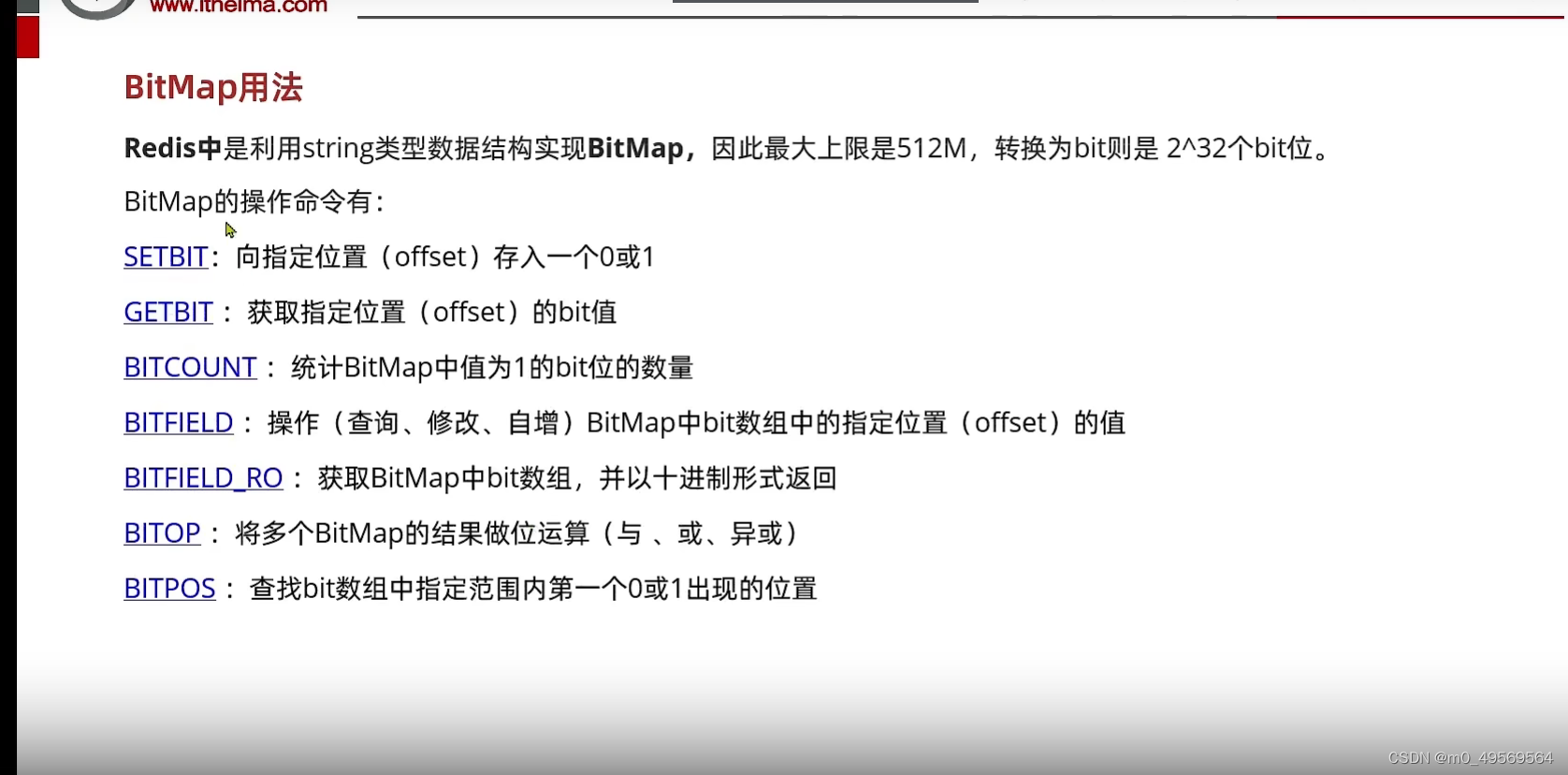
二、用户签到:实现签到功能
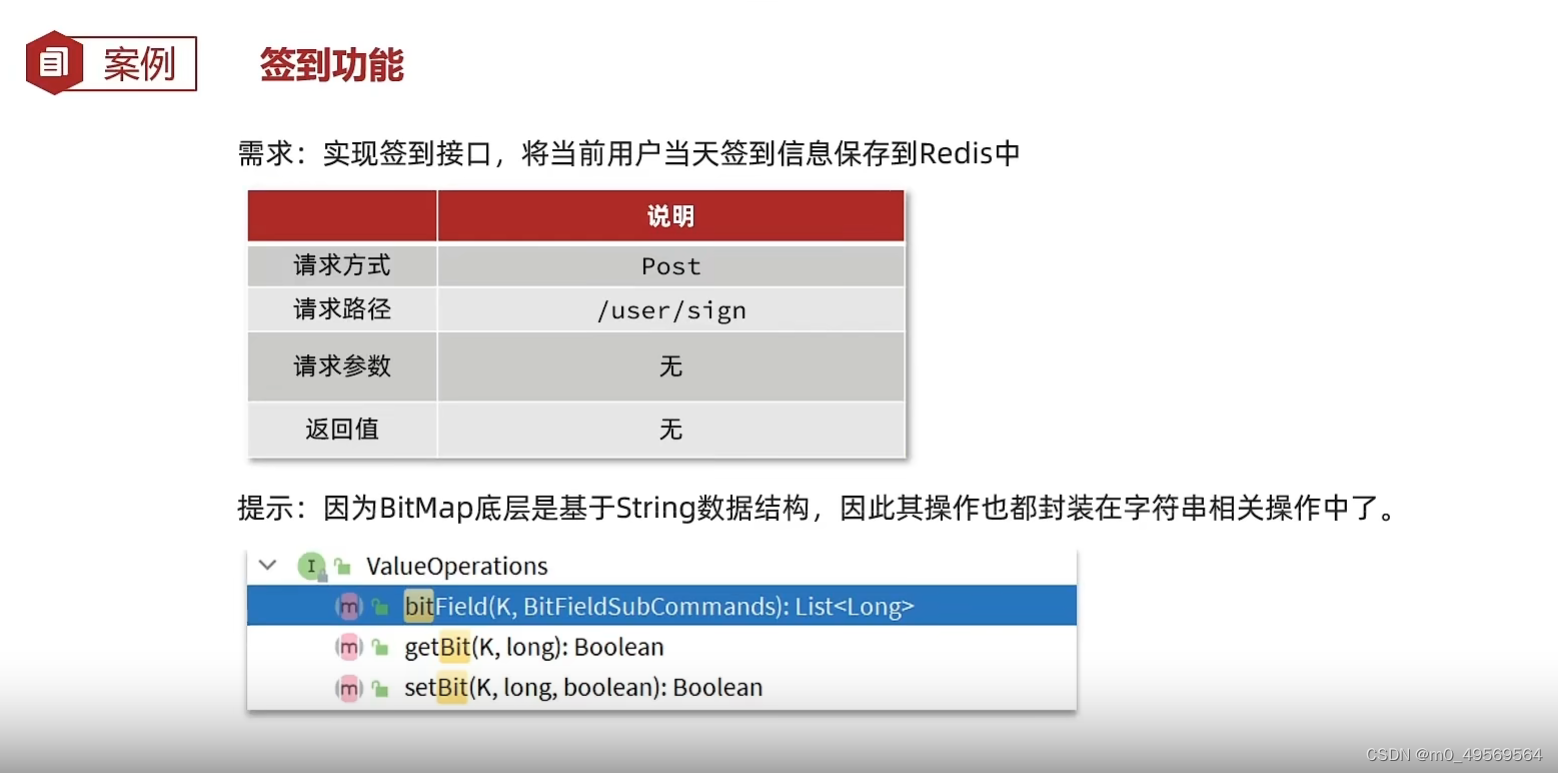
@Override
public Result sign() {
UserDTO user = UserHolder.getUser();
Long userId = user.getId();
LocalDateTime now = LocalDateTime.now();
String keySuffix = now.format(DateTimeFormatter.ofPattern(":yyyyMM"));
String key = com.hmdp.utils.RedisConstants.USER_SIGN_KEY + userId + keySuffix;
int dayOfMonth = now.getDayOfMonth();
redisTemplate.opsForValue().setBit(key,dayOfMonth - 1,true);
return Result.ok();
}
三、连续签到统计
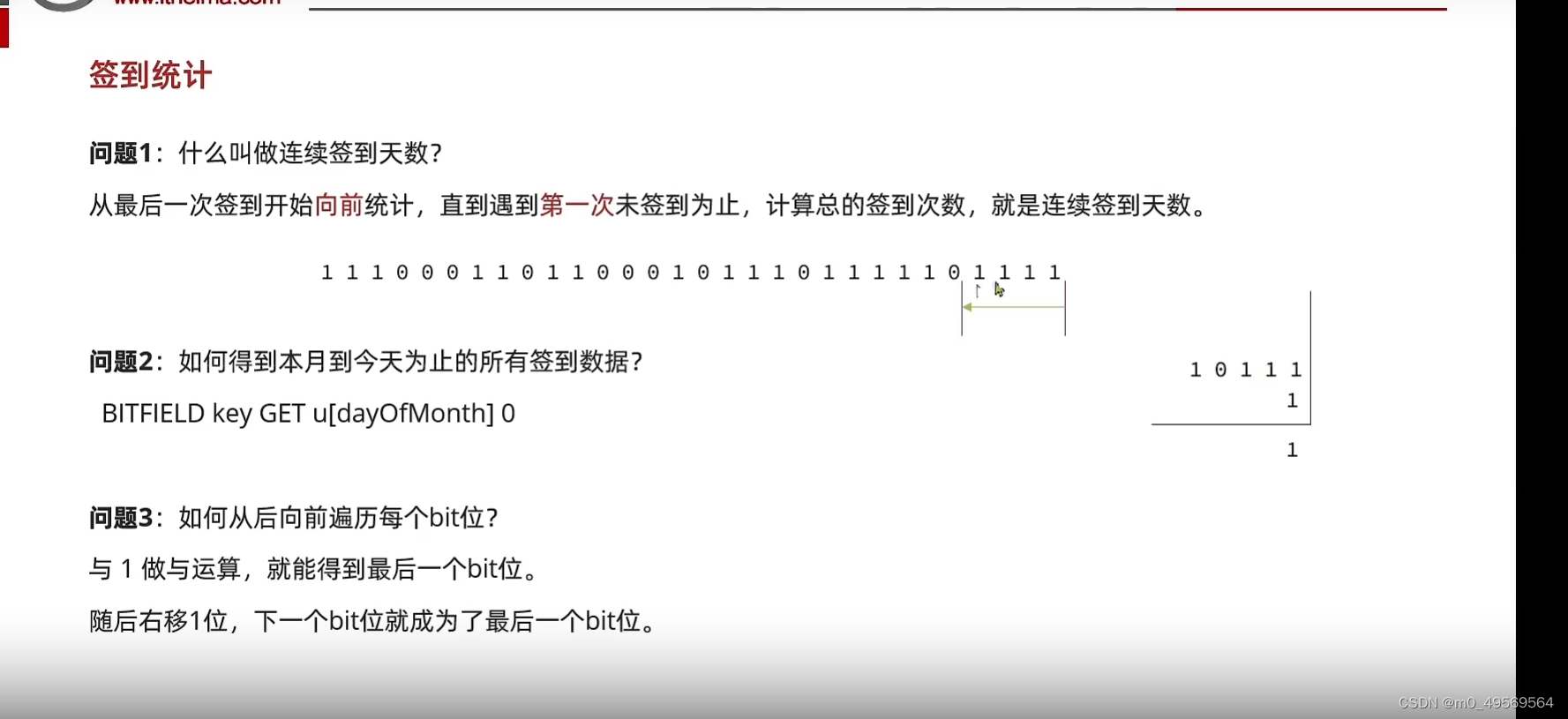
@Override
public Result signCount() {
Long userId = UserHolder.getUser().getId();
LocalDateTime now = LocalDateTime.now();
String keySuffix = now.format(DateTimeFormatter.ofPattern(":yyyyMM"));
String key = USER_SIGN_KEY + userId + keySuffix;
int dayOfMonth = now.getDayOfMonth();
List<Long> result = redisTemplate.opsForValue().bitField(
key,
BitFieldSubCommands.create()
.get(BitFieldSubCommands.BitFieldType.unsigned(dayOfMonth)).valueAt(0)
);
if (result == null || result.isEmpty()) {
return Result.ok(0);
}
Long num = result.get(0);
if (num == null || num == 0) {
return Result.ok(0);
}
int count = 0;
while (true) {
if ((num & 1) == 0) {
break;
}else {
count++;
}
num >>>= 1;
}
return Result.ok(count);
}
四、UV统计
UV:unique vistitor :独立访客量,指通过互联网访问、浏览网页的自然人。1天内同一个用户多次访问该网站,只记录1次。 PV:page view.页面访问量或者点击量,用户每访问一个页面,记录1次叫pv。往往用来衡量网站的流量。 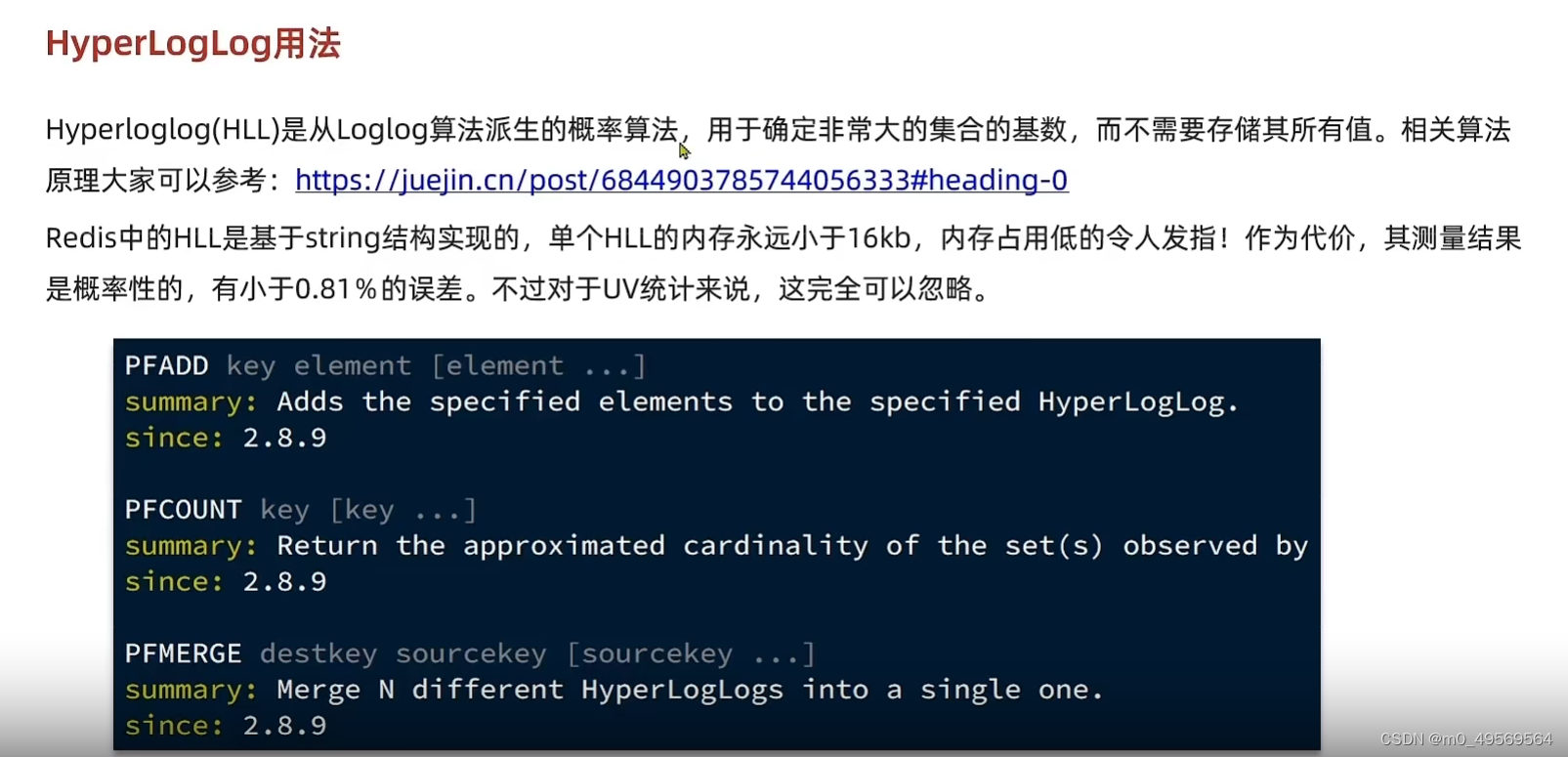
五、UV统计实现:百万数据
@Test
void testHyperLogLog() {
String[] values = new String[1000];
int j = 0;
for (int i = 0; i < 1000000; i++) {
j = i % 1000;
values[j] = "user_" + i;
if(j == 999){
stringRedisTemplate.opsForHyperLogLog().add("hl2", values);
}
}
Long count = stringRedisTemplate.opsForHyperLogLog().size("hl2");
System.out.println("count = " + count);
}
|