OpenCVForUnity 根据特征提取匹配图片
前言
遇到一个需要匹配图片的需求,就是给定一张图片,然后打开摄像头实时获取画面,如果画面中出现给定图片,则出现视觉效果。 本文只记录图片匹配方法。
感谢会思考的猴子的鼎力相助。
效果
这里相似度 < 15 的点有多少: 77 中的15 需要根据实际情况进行修改,值越低说明越相似。 匹配的点 越多,则说明图片相似特征越多。
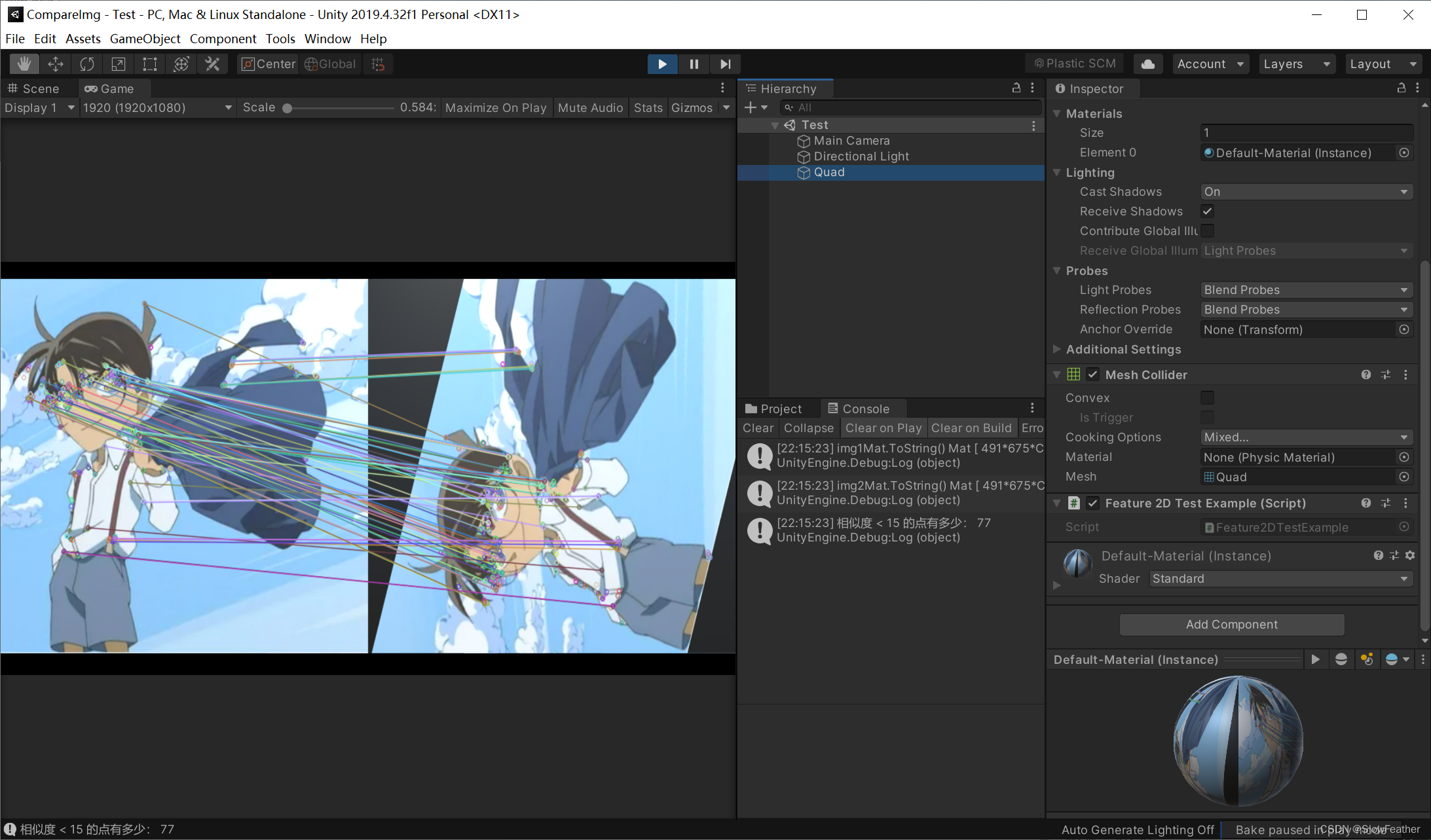
源码
原理在注释中
using UnityEngine;
using UnityEngine.SceneManagement;
using System.Collections;
using OpenCVForUnity.CoreModule;
using OpenCVForUnity.ImgprocModule;
using OpenCVForUnity.Features2dModule;
using OpenCVForUnity.UnityUtils;
using System.Linq;
using System.Collections.Generic;
namespace OpenCVForUnityExample
{
public class Feature2DTestExample : MonoBehaviour
{
void Start()
{
Texture2D imgTexture = Resources.Load("MainT") as Texture2D;
Mat img1Mat = new Mat(imgTexture.height, imgTexture.width, CvType.CV_8UC3);
Utils.texture2DToMat(imgTexture, img1Mat);
Debug.Log("img1Mat.ToString() " + img1Mat.ToString());
Texture2D tempTexture = Resources.Load("MainT2") as Texture2D;
Mat img2Mat = new Mat(tempTexture.height, tempTexture.width, CvType.CV_8UC3);
Utils.texture2DToMat(tempTexture, img2Mat);
Debug.Log("img2Mat.ToString() " + img2Mat.ToString());
float angle = UnityEngine.Random.Range(0, 360), scale = 1.0f;
Point center = new Point(img2Mat.cols() * 0.5f, img2Mat.rows() * 0.5f);
Mat affine_matrix = Imgproc.getRotationMatrix2D(center, angle, scale);
Imgproc.warpAffine(img2Mat, img2Mat, affine_matrix, img2Mat.size());
ORB detector = ORB.create();
ORB extractor = ORB.create();
MatOfKeyPoint keypoints1 = new MatOfKeyPoint();
Mat descriptors1 = new Mat();
detector.detect(img1Mat, keypoints1);
extractor.compute(img1Mat, keypoints1, descriptors1);
MatOfKeyPoint keypoints2 = new MatOfKeyPoint();
Mat descriptors2 = new Mat();
detector.detect(img2Mat, keypoints2);
extractor.compute(img2Mat, keypoints2, descriptors2);
DescriptorMatcher matcher = DescriptorMatcher.create(DescriptorMatcher.BRUTEFORCE_HAMMINGLUT);
MatOfDMatch matches = new MatOfDMatch();
matcher.match(descriptors1, descriptors2, matches);
int count = matches.toList().OrderBy(_ => _.distance).Count(_=>_.distance<15);
Debug.Log("相似度 < 15 的点有多少: " + count);
if(count<5){
return;
}
matches.fromList(matches.toList().OrderBy(_ => _.distance).Where(_=>_.distance<15).ToList());
Mat resultImg = new Mat();
Features2d.drawMatches(img1Mat, keypoints1, img2Mat, keypoints2, matches, resultImg);
Texture2D texture = new Texture2D(resultImg.cols(), resultImg.rows(), TextureFormat.RGBA32, false);
Utils.matToTexture2D(resultImg, texture);
gameObject.GetComponent<Renderer>().material.mainTexture = texture;
}
}
}
|