1.Opengl的Hello world
最基础的程序,画了个三角形,请确保理解这个程序,核心是opengl是一个状态机。想象opengl是一个几何画板,glBegin(GL_TRIANGLES); 就是点击绘制三角。glColor3f(1.0, 0.0, 0.0); 就是将颜色设置为红色。然后你在三个不同位置点了三个点。glFlush(); 就是保存提交给画面。然后你就得到了结果。
#include <gl/glut.h>
void mydisplay()
{
glClear(GL_COLOR_BUFFER_BIT);
glBegin(GL_TRIANGLES);
glColor3f(1.0, 0.0, 0.0);
glVertex3f(1.0, 0.0, 0.0);
glColor3f(0.0, 1.0, 0.0);
glVertex3f(0.0, 1.0, 0.0);
glColor3f(0.0, 0.0, 1.0);
glVertex3f(0.0, 0.0, 0.0);
glEnd();
glFlush();
}
int main(int argc, char** argv)
{
glutInit(&argc, argv);
glutCreateWindow("A Triangle");
glutDisplayFunc(mydisplay);
glutMainLoop();
return 0;
}
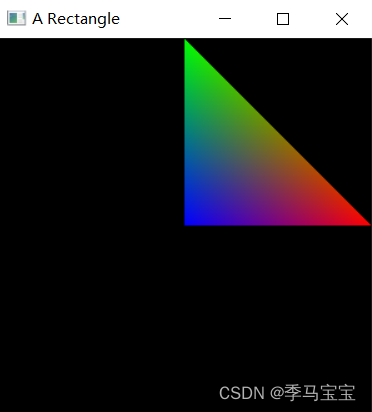
2.初始化(调整试图)
手动设置了使用RGB绘制、窗口位置及窗口大小。并且在init函数中加入了glOrtho(0.0, 1.0, 0.0, 1.0, 0.0, 1.0); 将图像变成了图中(x,y,z)分别对应范围(0-1,0-1,0-1)区间。
#include <gl/glut.h>
void mydisplay()
{
glClear(GL_COLOR_BUFFER_BIT);
glBegin(GL_TRIANGLES);
glColor3f(1.0, 0.0, 0.0);
glVertex3f(1.0, 0.0, 0.0);
glColor3f(0.0, 1.0, 0.0);
glVertex3f(0.0, 1.0, 0.0);
glColor3f(0.0, 0.0, 1.0);
glVertex3f(0.0, 0.0, 0.0);
glEnd();
glFlush();
}
void init()
{
glClearColor(0.0, 0.0, 0.0, 1.0);
glMatrixMode(GL_PROJECTION);
glLoadIdentity();
glOrtho(0.0, 1.0, 0.0, 1.0, 0.0, 1.0);
}
int main(int argc, char** argv)
{
glutInit(&argc, argv);
glutInitDisplayMode(GLUT_RGB);
glutInitWindowSize(100, 100);
glutInitWindowPosition(100, 100);
glutCreateWindow("A Triangle");
glutDisplayFunc(mydisplay);
init();
glutMainLoop();
return 0;
}
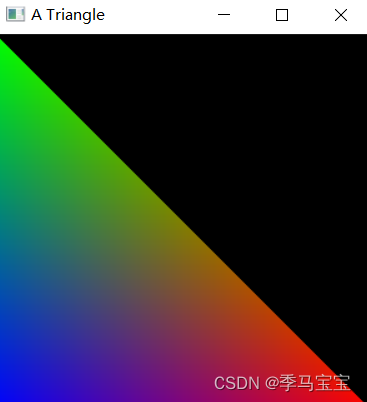
3.增加Reshape函数
在改变窗口时会调用的函数。默认的reshape函数会直接拉伸图形。 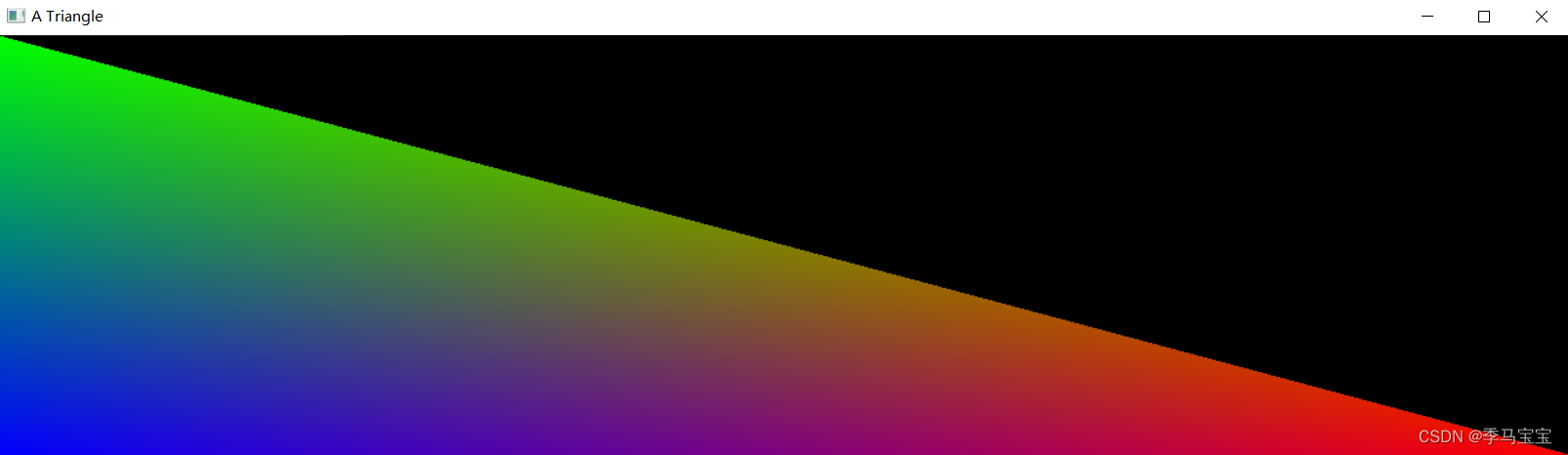 我们希望图像不被拉伸,而是保持原有大小。
#include <gl/glut.h>
int initW = 300;
int initH = 300;
void mydisplay()
{
glClear(GL_COLOR_BUFFER_BIT);
glBegin(GL_TRIANGLES);
glColor3f(1.0, 0.0, 0.0);
glVertex3f(1.0, 0.0, 0.0);
glColor3f(0.0, 1.0, 0.0);
glVertex3f(0.0, 1.0, 0.0);
glColor3f(0.0, 0.0, 1.0);
glVertex3f(0.0, 0.0, 0.0);
glEnd();
glFlush();
}
void init()
{
glClearColor(0.0, 0.0, 0.0, 1.0);
glMatrixMode(GL_PROJECTION);
glLoadIdentity();
glOrtho(0.0, 1.0, 0.0, 1.0, 0.0, 1.0);
}
void myReshape(int w, int h)
{
glViewport(0, 0, w, h);
glMatrixMode(GL_PROJECTION);
glLoadIdentity();
if (w <= h)
glOrtho(0, 1.0, 0.0, (GLfloat)h / (GLfloat)w * 1.0, 0.0, 1.0);
else
glOrtho(0, (GLfloat)w / (GLfloat)h * 1.0, 0.0, 1.0, 0.0, 1.0);
}
int main(int argc, char** argv)
{
glutInit(&argc, argv);
glutInitDisplayMode( GLUT_RGB);
glutInitWindowSize(initW, initH);
glutInitWindowPosition(100, 100);
glutCreateWindow("A Triangle");
glutDisplayFunc(mydisplay);
init();
glutReshapeFunc(myReshape);
glutMainLoop();
return 0;
}
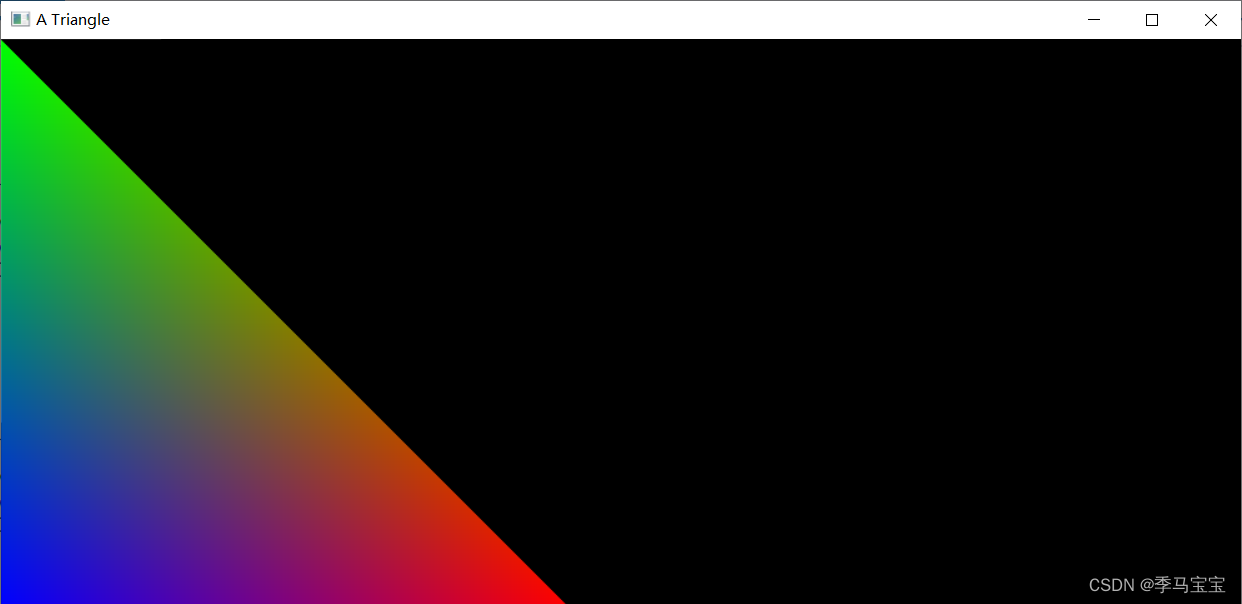
3.事件
增加了鼠标左键点击事件mouse,点击时会改变图像亮度并输出点击位置
#include <gl/glut.h>
#include <iostream>
int initW = 300;
int initH = 300;
GLfloat brightness = 1.0;
GLfloat increment = -0.1;
void mydisplay()
{
glClear(GL_COLOR_BUFFER_BIT);
glBegin(GL_TRIANGLES);
glColor3f(brightness, 0.0, 0.0);
glVertex3f(1.0, 0.0, 0.0);
glColor3f(0.0, brightness, 0.0);
glVertex3f(0.0, 1.0, 0.0);
glColor3f(0.0, 0.0, brightness);
glVertex3f(0.0, 0.0, 0.0);
glEnd();
glFlush();
}
void init()
{
glClearColor(0.0, 0.0, 0.0, 1.0);
glMatrixMode(GL_PROJECTION);
glLoadIdentity();
glOrtho(0.0, 1.0, 0.0, 1.0, 0.0, 1.0);
}
void myReshape(int w, int h)
{
glViewport(0, 0, w, h);
glMatrixMode(GL_PROJECTION);
glLoadIdentity();
if (w <= h)
glOrtho(0, 1.0, 0.0, (GLfloat)h / (GLfloat)w * 1.0, 0.0, 1.0);
else
glOrtho(0, (GLfloat)w / (GLfloat)h * 1.0, 0.0, 1.0, 0.0, 1.0);
}
void mouse(int btn, int state, int x, int y)
{
if (btn == GLUT_LEFT_BUTTON && state == GLUT_DOWN)
{
std::cout << "x: " << x << " y: " << y << std::endl;
brightness += increment;
if (brightness >= 1.0 || brightness <= 0.0)
increment = -increment;
}
}
int main(int argc, char** argv)
{
glutInit(&argc, argv);
glutInitDisplayMode( GLUT_RGB);
glutInitWindowSize(initW, initH);
glutInitWindowPosition(100, 100);
glutCreateWindow("A Triangle");
glutDisplayFunc(mydisplay);
init();
glutReshapeFunc(myReshape);
glutMouseFunc(mouse);
glutMainLoop();
return 0;
}
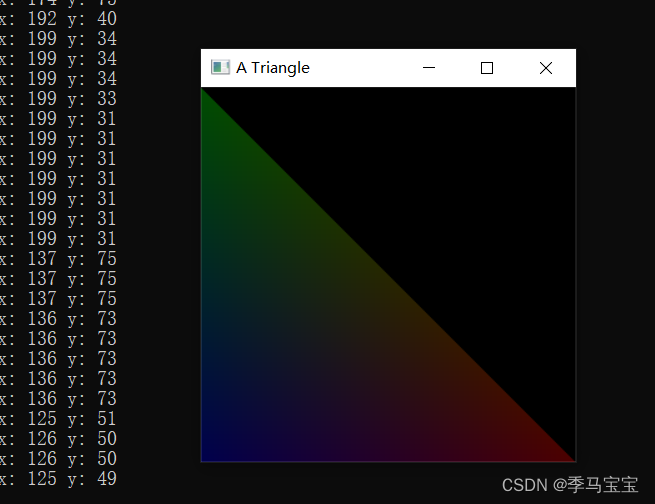
4.动画
使用定时器的一段动画,图像亮度随时间变化
#include <gl/glut.h>
#include <iostream>
int initW = 300;
int initH = 300;
GLfloat brightness = 1.0;
GLfloat increment = -0.1;
void mydisplay()
{
glClear(GL_COLOR_BUFFER_BIT);
glBegin(GL_TRIANGLES);
glColor3f(brightness, 0.0, 0.0);
glVertex3f(1.0, 0.0, 0.0);
glColor3f(0.0, brightness, 0.0);
glVertex3f(0.0, 1.0, 0.0);
glColor3f(0.0, 0.0, brightness);
glVertex3f(0.0, 0.0, 0.0);
glEnd();
glutSwapBuffers();
}
void changeColor(int x)
{
brightness += increment;
if (brightness >= 1.0 || brightness <= 0.0)
increment = -increment;
glutPostRedisplay();
glutTimerFunc(100, changeColor, 0);
}
void init()
{
glClearColor(0.0, 0.0, 0.0, 1.0);
glMatrixMode(GL_PROJECTION);
glLoadIdentity();
glOrtho(0.0, 1.0, 0.0, 1.0, 0.0, 1.0);
}
int main(int argc, char** argv)
{
glutInit(&argc, argv);
glutInitDisplayMode( GLUT_RGB|GLUT_DOUBLE);
glutInitWindowSize(initW, initH);
glutInitWindowPosition(100, 100);
glutCreateWindow("A Triangle");
glutTimerFunc(10, changeColor, 0);
glutDisplayFunc(mydisplay);
init();
glutMainLoop();
return 0;
}
|