@作者: 风不停息丶 学习笔记 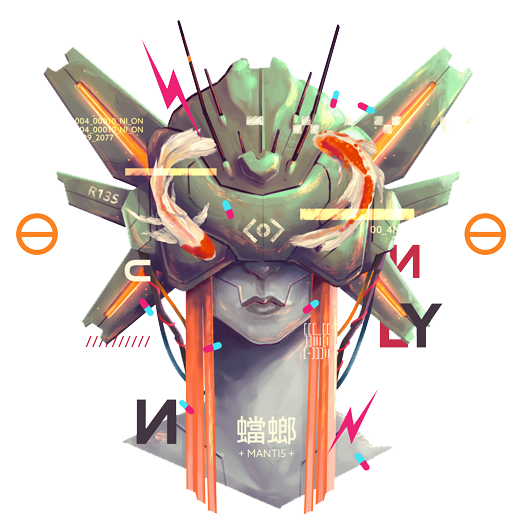
MVC基本概念
-
MVC全名是Model View Controller -
模型(model) - 视图(view) - 控制器(controller)的缩写 -
是一种软件设计规范,用一种业务逻辑、数据、界面显示分离的方法组织代码 -
将业务逻辑聚集到一个部件里面,在改进和个性化定制界面及用户交互的同时,不需要重新编写业务逻辑。 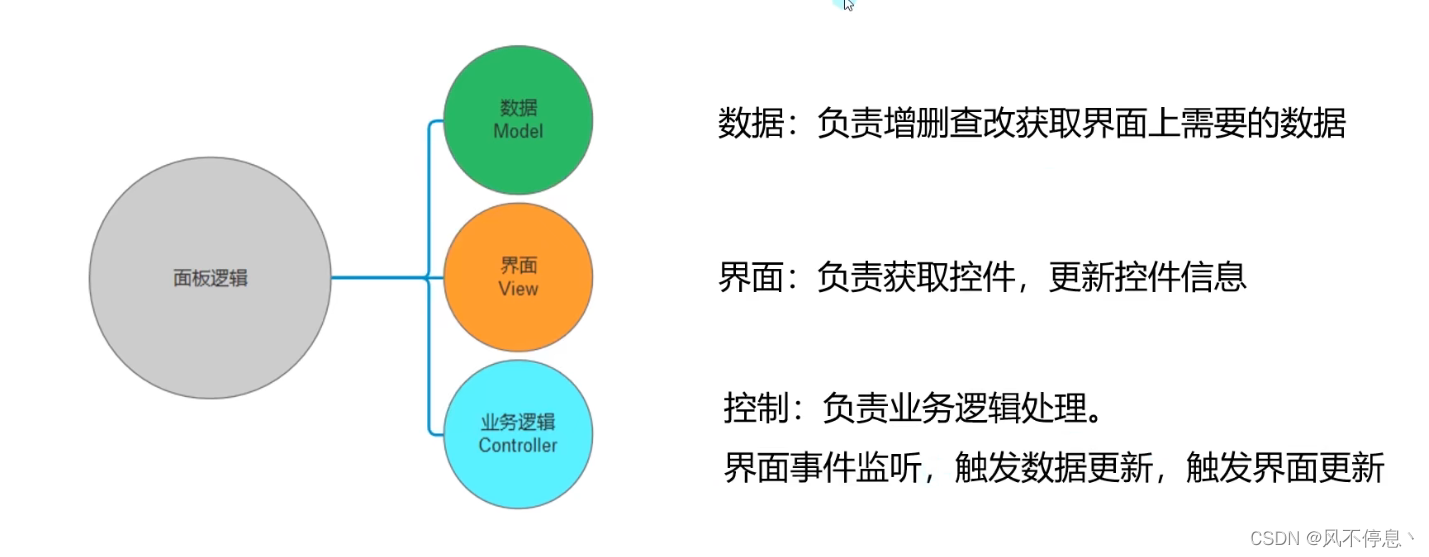 -
🐱?💻注意: -
1.在unity中它不是必备的内容 -
2.它主要用于开发游戏UI系统逻辑 -
在unity中应用于UI界面,可以将你的UI面板逻辑一分为三,数据、界面、业务逻辑 优点: 降低耦合性,方法修改,逻辑更清晰 缺点: 脚本多,体量大,流程复杂
案例:使用MVC搭建游戏UI界面
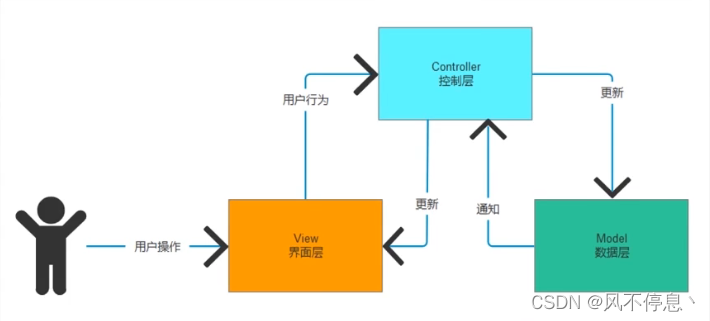
1、创建数据层Model,存储数据
public class PlayerModel {
private string playerName;
public string PlayerName
{
get
{
return PlayerName;
}
}
private int lev;
public int Lev
{
get
{
return lev;
}
}
private int money;
public int Money
{
get
{
return money;
}
}
private int gem;
public int Gem
{
get
{
return gem;
}
}
private int power;
public int Power
{
get
{
return power;
}
}
private int hp;
public int Hp
{
get
{
return hp;
}
}
private int atk;
public int Atk
{
get
{
return atk;
}
}
private int def;
public int Def
{
get
{
return def;
}
}
private int crit;
public int Crit
{
get
{
return crit;
}
}
private int miss;
public int Miss
{
get
{
return miss;
}
}
private int luck;
public int Luck
{
get
{
return luck;
}
}
private event UnityAction<PlayerModel> updateEvent;
private static PlayerModel data = null;
public static PlayerModel Data
{
get
{
if (data == null)
{
data = new PlayerModel();
data.Init();
}
return data;
}
}
public void Init()
{
playerName = PlayerPrefs.GetString("playerName", "小明");
lev = PlayerPrefs.GetInt("lev", 1);
money = PlayerPrefs.GetInt("money", 1000);
gem = PlayerPrefs.GetInt("gem", 1000);
power = PlayerPrefs.GetInt("power", 99);
hp = PlayerPrefs.GetInt("hp", 100);
atk = PlayerPrefs.GetInt("atk", 20);
def = PlayerPrefs.GetInt("def", 10);
crit = PlayerPrefs.GetInt("crit", 10);
miss = PlayerPrefs.GetInt("miss", 10);
luck = PlayerPrefs.GetInt("luck", 40);
}
public void LevUp()
{
lev += 1;
hp += lev;
atk += lev;
def += lev;
crit += lev;
miss += lev;
luck += lev;
SaveData();
}
public void SaveData()
{
PlayerPrefs.SetString("playerName", playerName);
PlayerPrefs.SetInt("playerLev", lev);
PlayerPrefs.SetInt("playerMoney", money);
PlayerPrefs.SetInt("playerGem", gem);
PlayerPrefs.SetInt("playerPower", power);
PlayerPrefs.SetInt("playerHp", hp);
PlayerPrefs.SetInt("playerAtk", atk);
PlayerPrefs.SetInt("playerDef", def);
PlayerPrefs.SetInt("playerCrit", crit);
PlayerPrefs.SetInt("playerMiss", miss);
PlayerPrefs.SetInt("playerLuck", luck);
UpdateInfo();
}
public void AddEventListener(UnityAction<PlayerModel> function)
{
updateEvent += function;
}
public void RemoveEventListener(UnityAction<PlayerModel> function)
{
updateEvent -= function;
}
private void UpdateInfo()
{
if (updateEvent != null)
{
updateEvent(this);
}
}
}
2、创建界面层View,显示UI
public class MainView : MonoBehaviour {
public Button btnRole;
public Button btnSill;
public Text textName;
public Text textLev;
public Text textMoney;
public Text textGem;
public Text textPower;
public void UpDateInfo(PlayerModel data)
{
textName.text = data.PlayerName;
textLev.text = "LV." + data.Lev;
textMoney.text = "金币:" + data.Money.ToString();
textGem.text = "钻石:" + data.Gem.ToString();
textPower.text = "体力:" + data.Power.ToString();
}
}
public class RoleView : MonoBehaviour {
public Text textLev;
public Text textHp;
public Text textAtk;
public Text textDef;
public Text textCrit;
public Text textMiss;
public Text textLuck;
public Button btnClose;
public Button btnLevUp;
public void UpdateInfo(PlayerModel data)
{
textLev.text = "LV." + data.Lev;
textHp.text = "血量:" + data.Hp.ToString();
textAtk.text = "攻击力:" + data.Atk.ToString();
textDef.text = "防御力:" + data.Def.ToString();
textCrit.text = "暴击率:" + data.Crit.ToString();
textMiss.text = "闪避率:" + data.Miss.ToString();
textLuck.text = "幸运值:" + data.Luck.ToString();
}
}
3、创建控制层Controller,处理业务逻辑
public class MainController : MonoBehaviour {
private MainView mainView;
private static MainController controller = null;
public static MainController Controller
{
get { return controller; }
}
private void Start()
{
mainView = GetComponent<MainView>();
mainView.UpDateInfo(PlayerModel.Data);
mainView.btnRole.onClick.AddListener(clickRoleBtn);
PlayerModel.Data.AddEventListener(UpdateInfo);
}
public static void ShowMe()
{
if (controller == null)
{
GameObject res = Resources.Load<GameObject>("Prefabs/MinPanel");
GameObject obj = Instantiate(res);
obj.transform.SetParent(GameObject.Find("Canvas").transform, false);
controller = obj.GetComponent<MainController>();
}
controller.gameObject.SetActive(true);
}
public static void HideMe()
{
if (controller != null)
{
controller.gameObject.SetActive(false);
}
}
private void clickRoleBtn()
{
RoleController.ShowMe();
}
private void UpdateInfo(PlayerModel data)
{
if (mainView != null)
{
mainView.UpDateInfo(data);
}
}
private void Ondestroy()
{
PlayerModel.Data.RemoveEventListener(UpdateInfo);
}
}
public class RoleController : MonoBehaviour {
private RoleView roleView;
private static RoleController controller = null;
public static RoleController Controller
{
get { return controller; }
}
void Start()
{
roleView = GetComponent<RoleView>();
roleView.UpdateInfo(PlayerModel.Data);
roleView.btnClose.onClick.AddListener(() =>
{
HideMe();
});
roleView.btnLevUp.onClick.AddListener(ClickLevUpBtn);
PlayerModel.Data.AddEventListener(UpdateInfo);
}
public static void ShowMe()
{
if (controller == null)
{
GameObject res = Resources.Load<GameObject>("Prefabs/RolePanel");
GameObject obj = Instantiate(res);
obj.transform.SetParent(GameObject.Find("Canvas").transform, false);
controller = obj.GetComponent<RoleController>();
}
controller.gameObject.SetActive(true);
}
public static void HideMe()
{
if (controller != null)
{
controller.gameObject.SetActive(false);
}
}
private void ClickLevUpBtn()
{
PlayerModel.Data.LevUp();
}
private void UpdateInfo(PlayerModel data)
{
if (roleView != null)
{
roleView.UpdateInfo(data);
}
}
private void Ondestroy()
{
PlayerModel.Data.RemoveEventListener(UpdateInfo);
}
}
最终效果
- 搭建场景,主页和角色面板
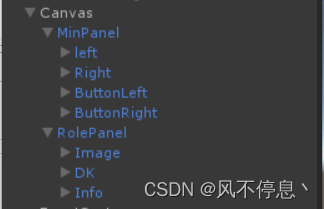
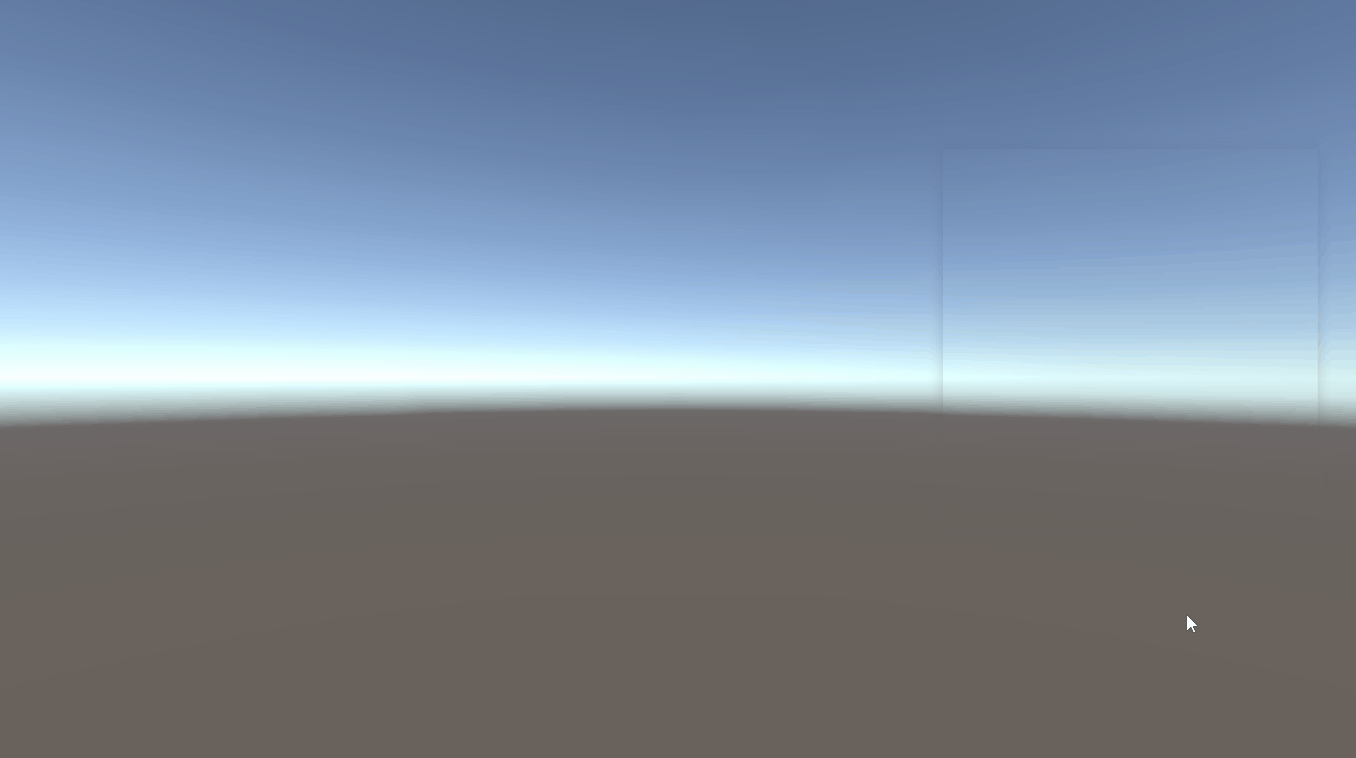
结尾总结
新手记录学习笔记,看到这篇文章的小伙伴们可以点个赞👍支持一下,谢谢! 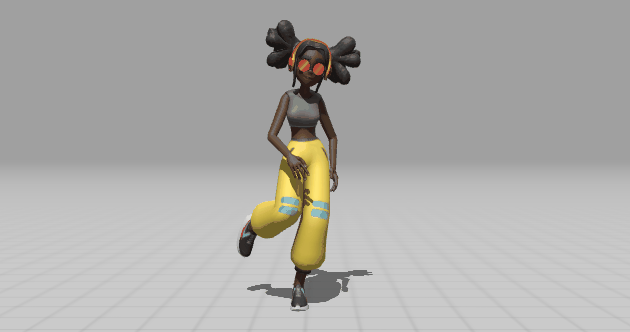
|