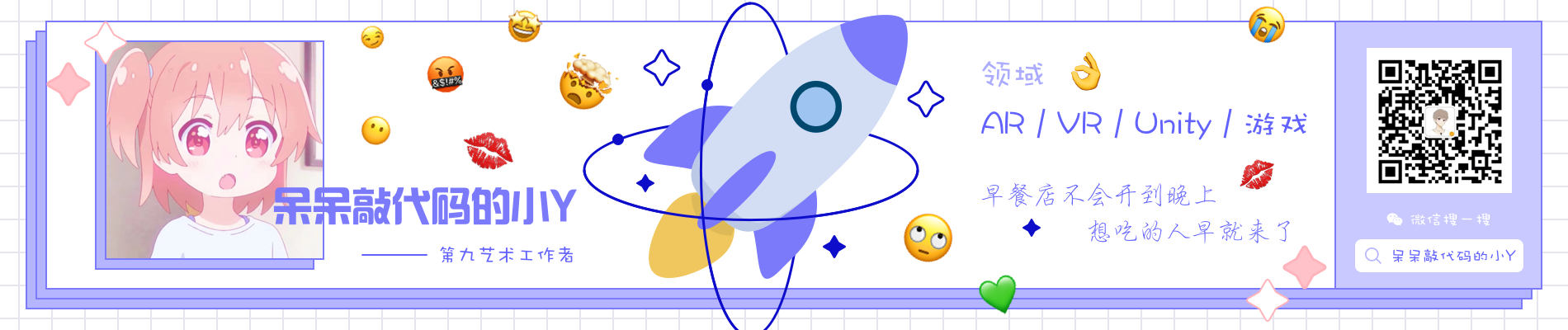
Unity 小科普
老规矩,先介绍一下 Unity 的科普小知识:
- Unity是 实时3D互动内容创作和运营平台 。
- 包括游戏开发、美术、建筑、汽车设计、影视在内的所有创作者,借助 Unity 将创意变成现实。
- Unity 平台提供一整套完善的软件解决方案,可用于创作、运营和变现任何实时互动的2D和3D内容,支持平台包括手机、平板电脑、PC、游戏主机、增强现实和虚拟现实设备。
- 也可以简单把 Unity 理解为一个游戏引擎,可以用来专业制作游戏!
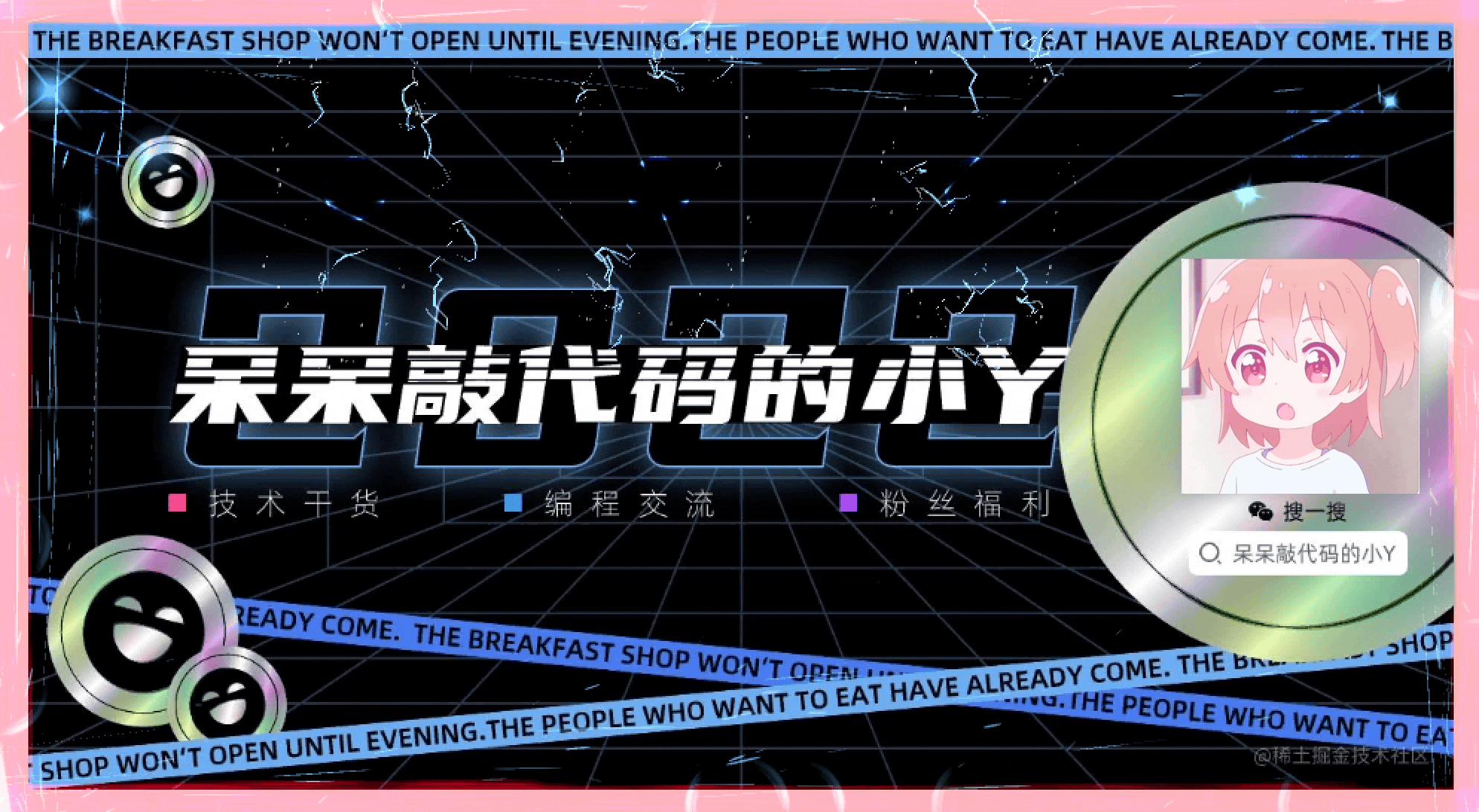
Unity 实用小技能学习
Unity InputSystem拿到触摸屏幕的坐标,鼠标的坐标等
在Unity的新输入系统InputSystem中,获取键盘鼠标的API发生了变化,不再是之前用Input.就可以拿到了。
本文将在InputSystem中获取键盘鼠标的新API做一个简单总结整理。
键盘相关 键盘事件监听
void Update()
{
if (Keyboard.current.spaceKey.wasPressedThisFrame)
{
Debug.Log("空格键按下");
}
if(Keyboard.current.aKey.wasReleasedThisFrame)
{
Debug.Log("A键抬起");
}
if(Keyboard.current.spaceKey.isPressed)
{
Debug.Log("空格按下");
}
if(Keyboard.current.anyKey.wasPressedThisFrame)
{
Debug.Log("任意键按下");
}
}
键盘事件绑定
void Start()
{
Keyboard.current.onTextInput += (c) =>
{
Debug.Log("通过Lambda表达式" + c);
};
Keyboard.current.onTextInput += KeyboardInput;
}
private void KeyboardInput(char c)
{
Debug.Log("监听" + c);
}
鼠标相关:
鼠标坐标
void Update
{
if(Mouse.current.rightButton.wasPressedThisFrame)
{
Debug.Log("鼠标右键按下");
}
if(Mouse.current.middleButton.wasPressedThisFrame)
{
Debug.Log("鼠标中建按下");
}
if(Mouse.current.forwardButton.wasPressedThisFrame)
{
Debug.Log("鼠标前键按下");
}
if(Mouse.current.backButton.wasPressedThisFrame)
{
Debug.Log("鼠标后键按下");
}
Debug.Log(Mouse.current.position.ReadValue());
Debug.Log(Mouse.current.delta.ReadValue());
Debug.Log(Mouse.current.scroll.ReadValue());
}
鼠标事件绑定
void InputTest()
{
GameInput inputAction = new GameInput();
inputAction.Enable();
inputAction.Gameplay.MouseDown.performed += ctx =>
{
Debug.Log("按下:" + UnityEngine.InputSystem.Mouse.current.position.ReadValue());
};
inputAction.Gameplay.MouseDrag.performed += ctx =>
{
Debug.Log("拖拽:" + UnityEngine.InputSystem.Mouse.current.position.ReadValue());
};
inputAction.Gameplay.MouseUp.performed += ctx =>
{
Debug.Log("抬起:" + UnityEngine.InputSystem.Mouse.current.position.ReadValue());
};
}
触摸屏相关
void Update
{
Touchscreen ts = Touchscreen.current;
if (ts == null)
{
return;
}
else
{
TouchControl tc = ts.touches[0];
if(tc.press.wasPressedThisFrame)
{
Debug.Log("按下");
}
if(tc.press.wasReleasedThisFrame)
{
Debug.Log("抬起");
}
if(tc.press.isPressed)
{
Debug.Log("按住");
}
if(tc.tap.isPressed)
{
}
Debug.Log(tc.tapCount);
Debug.Log(tc.position.ReadValue());
Debug.Log(tc.startPosition.ReadValue());
Debug.Log(tc.radius.ReadValue());
Debug.Log(tc.delta.ReadValue());
Debug.Log(tc.phase.ReadValue());
UnityEngine.InputSystem.TouchPhase tp = tc.phase.ReadValue();
switch (tp)
{
case UnityEngine.InputSystem.TouchPhase.None:
break;
case UnityEngine.InputSystem.TouchPhase.Began:
break;
case UnityEngine.InputSystem.TouchPhase.Moved:
break;
case UnityEngine.InputSystem.TouchPhase.Ended:
break;
case UnityEngine.InputSystem.TouchPhase.Canceled:
break;
case UnityEngine.InputSystem.TouchPhase.Stationary:
break;
}
}
手柄相关
Gamepad handle = Gamepad.current;
if(handle==null)
{
return;
}
Vector2 leftDir= handle.leftStick.ReadValue();
Vector2 rightDir= handle.rightStick.ReadValue();
if(Gamepad.current.leftStickButton.wasPressedThisFrame)
{
}
if (Gamepad.current.leftStickButton.wasReleasedThisFrame)
{
}
if (Gamepad.current.leftStickButton.isPressed)
{
}
if (Gamepad.current.rightStickButton.wasPressedThisFrame)
{
}
if (Gamepad.current.rightStickButton.wasReleasedThisFrame)
{
}
if (Gamepad.current.rightStickButton.isPressed)
{
}
if(Gamepad.current.dpad.left.wasPressedThisFrame)
{
}
if (Gamepad.current.dpad.left.wasReleasedThisFrame)
{
}
if (Gamepad.current.dpad.left.isPressed)
{
}
if (Gamepad.current.buttonNorth.wasPressedThisFrame)
{
}
if (Gamepad.current.buttonNorth.wasReleasedThisFrame)
{
}
if (Gamepad.current.buttonNorth.isPressed)
{
}
if(Gamepad.current.startButton.wasPressedThisFrame)
{
}
if(Gamepad.current.selectButton.wasPressedThisFrame)
{
}
if(Gamepad.current.leftShoulder.wasPressedThisFrame)
{
}
if (Gamepad.current.rightShoulder.wasPressedThisFrame)
{
}
if(Gamepad.current.leftTrigger.wasPressedThisFrame)
{
}
if(Gamepad.current.rightTrigger.wasPressedThisFrame)
{
}
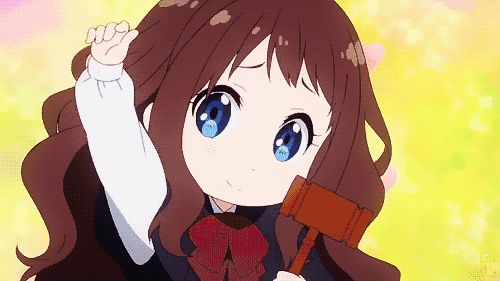
|