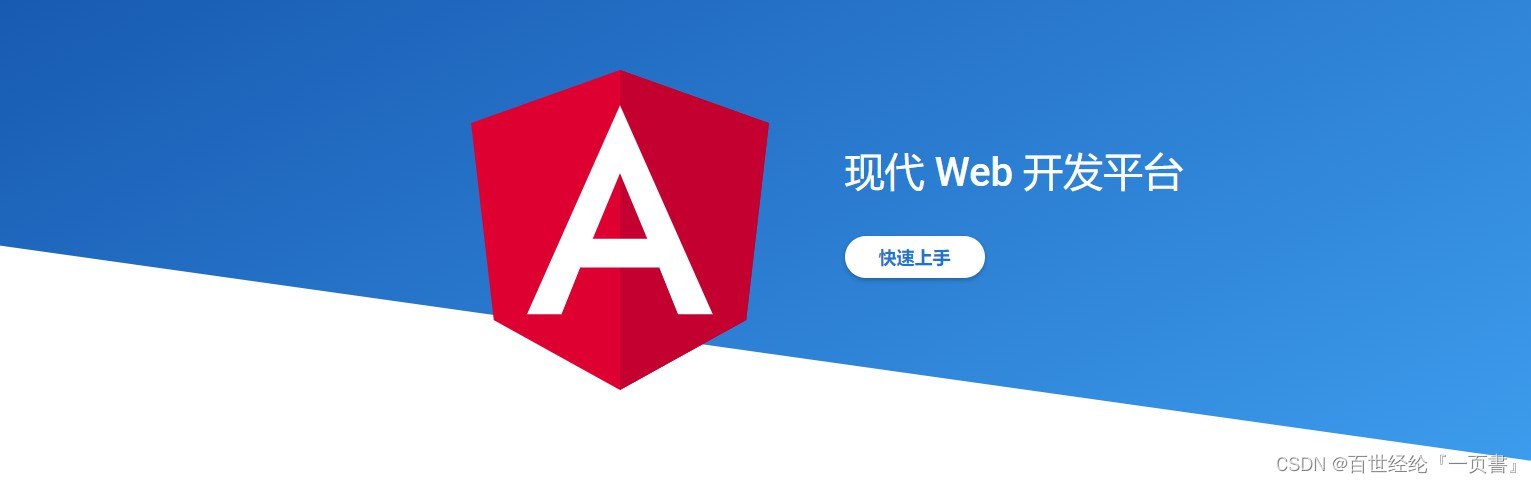
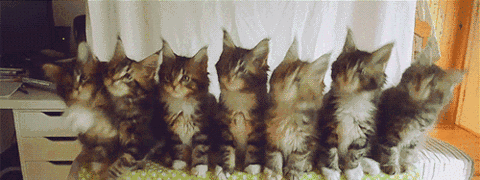 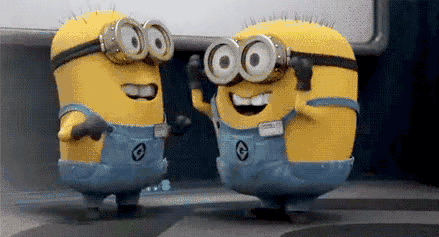
1.Angular.js VS Angular
两者其实是同一物件的不同版本: 前者的核心是组件另外可以取消双向绑定,在性能上大大提升; 后者是模板功能强大丰富,具有双向数据绑定等等;
- Angular是Google维护的一款开源javaScript框架。
- 在Anguar2.0之前的版本都叫做AngularJS,但在Angular4.0版本后就称Angular,Angular1.5到Angular4.0是完全重写。在1.x版本时使用的方法是引入AngularJS的js文件到网页中,而2.0版本之后就完全不同了。
1.1 Angular.js
Angular.js Website: https://docs.angularjs.org/guide .
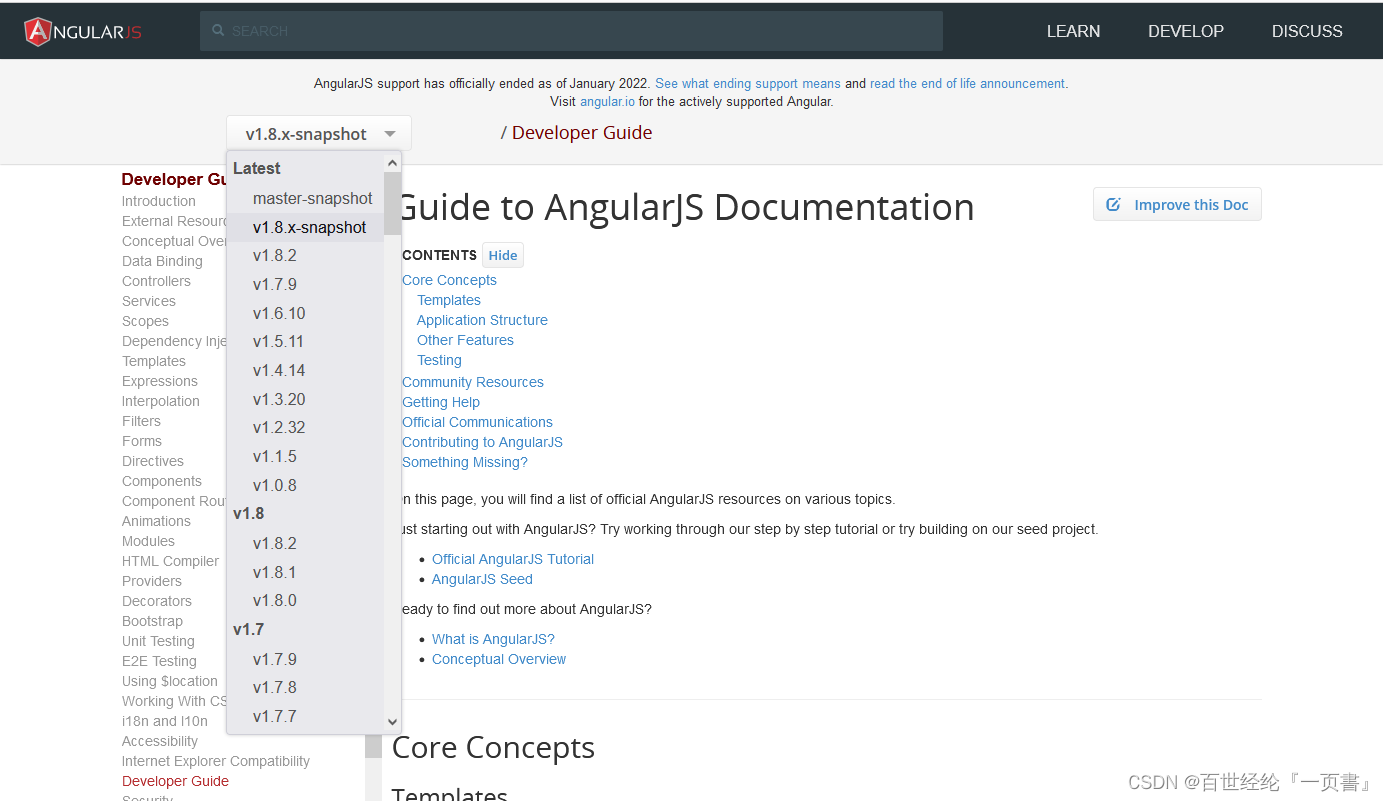
1.2 Angular
Angular Website: https://next.angular.io/docs . 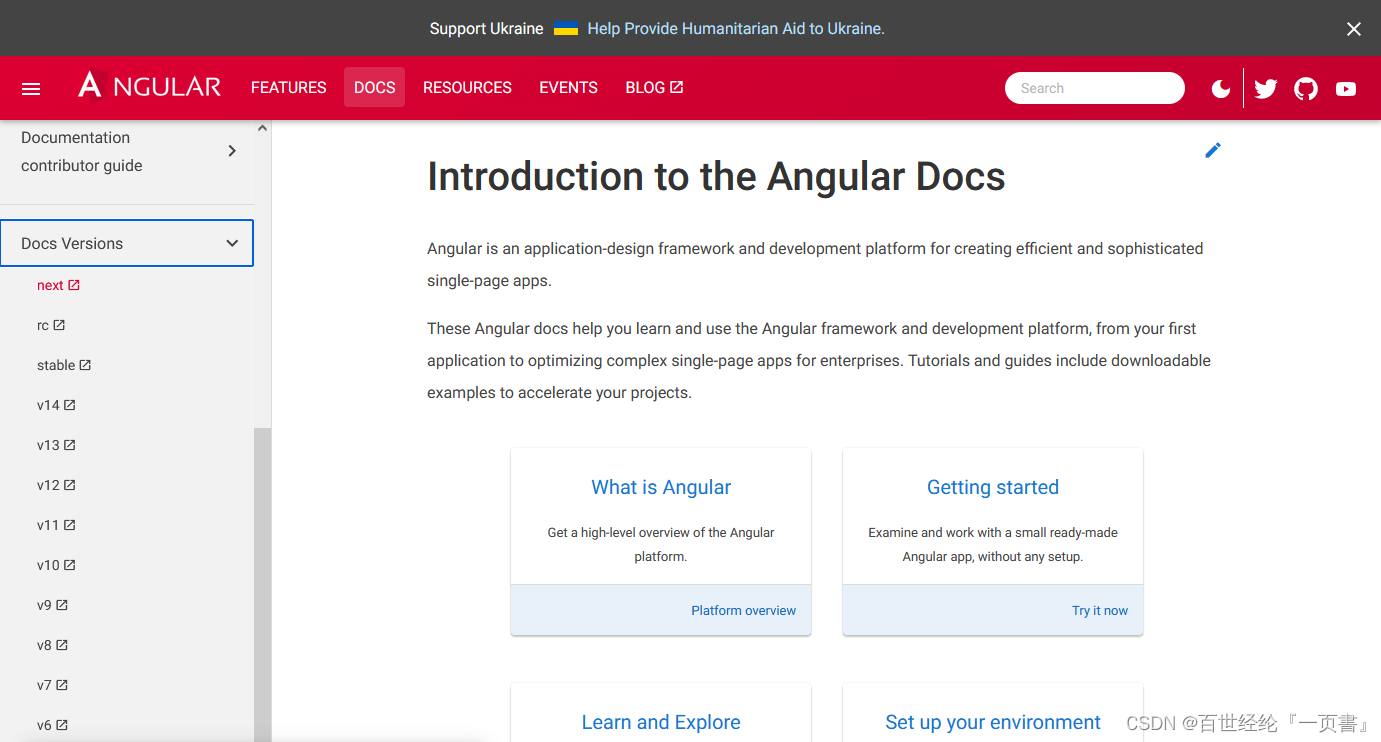
2.Angular Introduction
2.1 Install && create project
- npm install -g @angular/cli
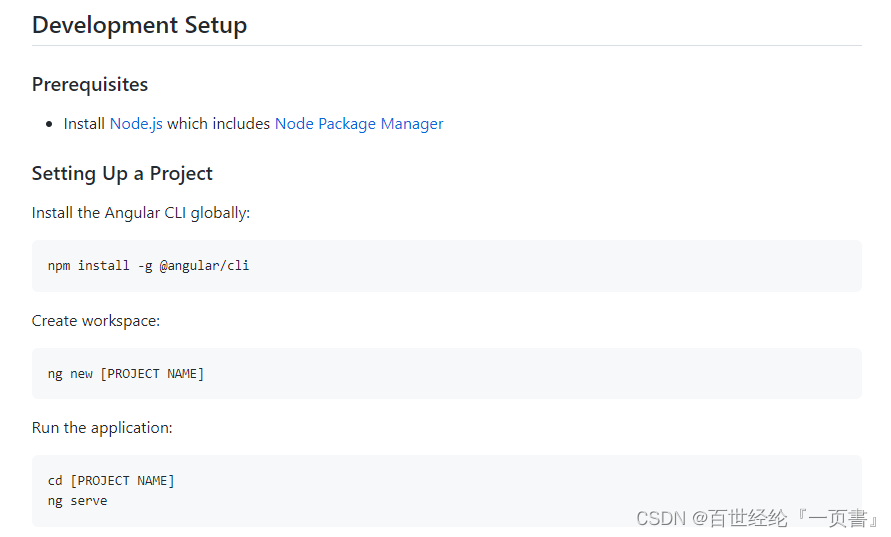
2.2 在Stackblitz上打开项目
- 官方直接建好了project
Angular Website: https://stackblitz.com. 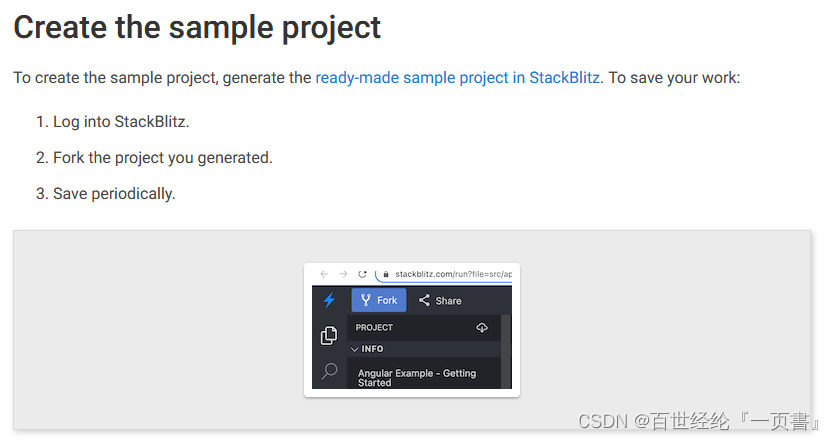 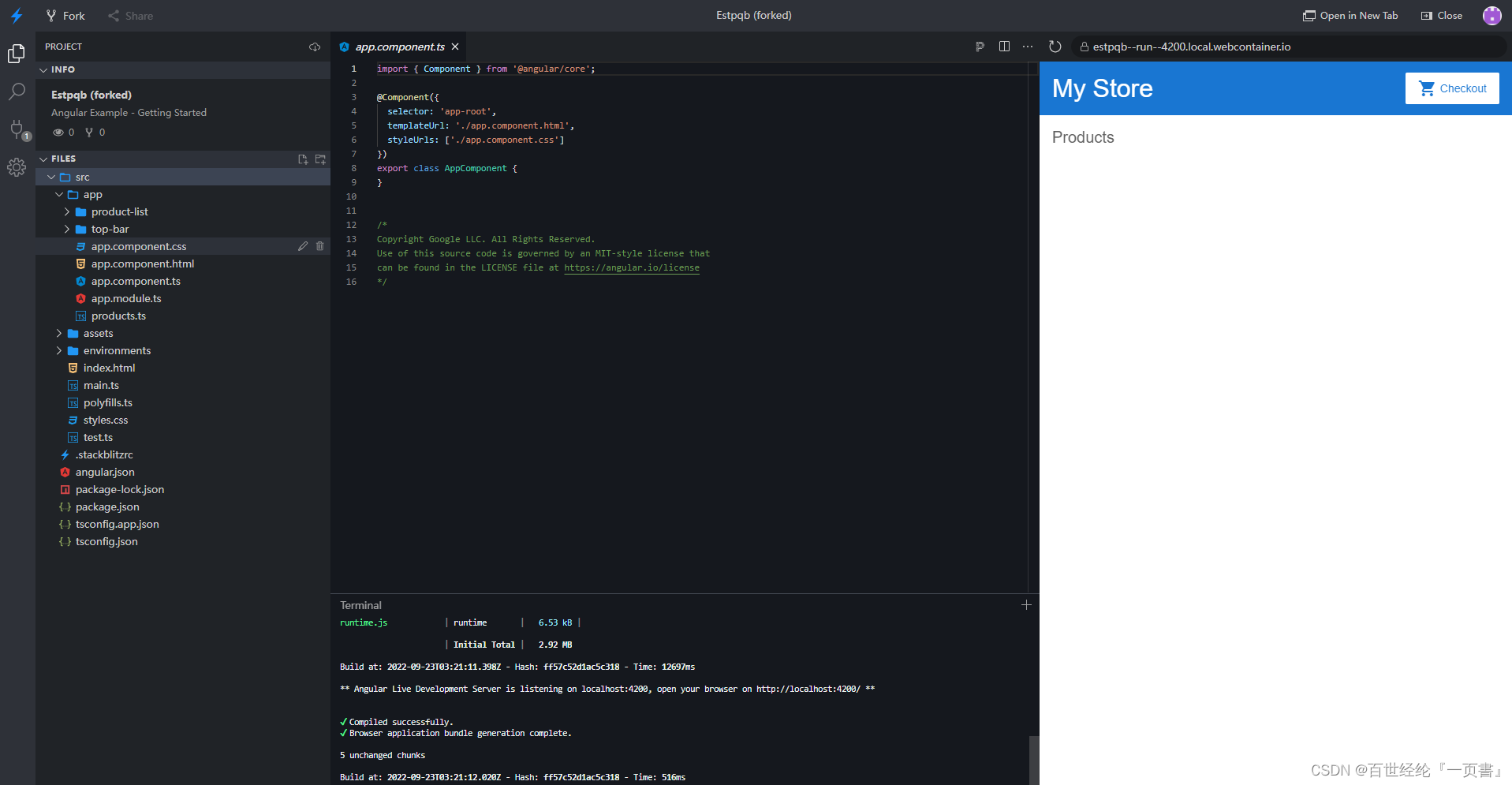 - 非常Nice,登录github,直接保存到自己的repository
第一种选择: new repository 第二种选择: import an existing repository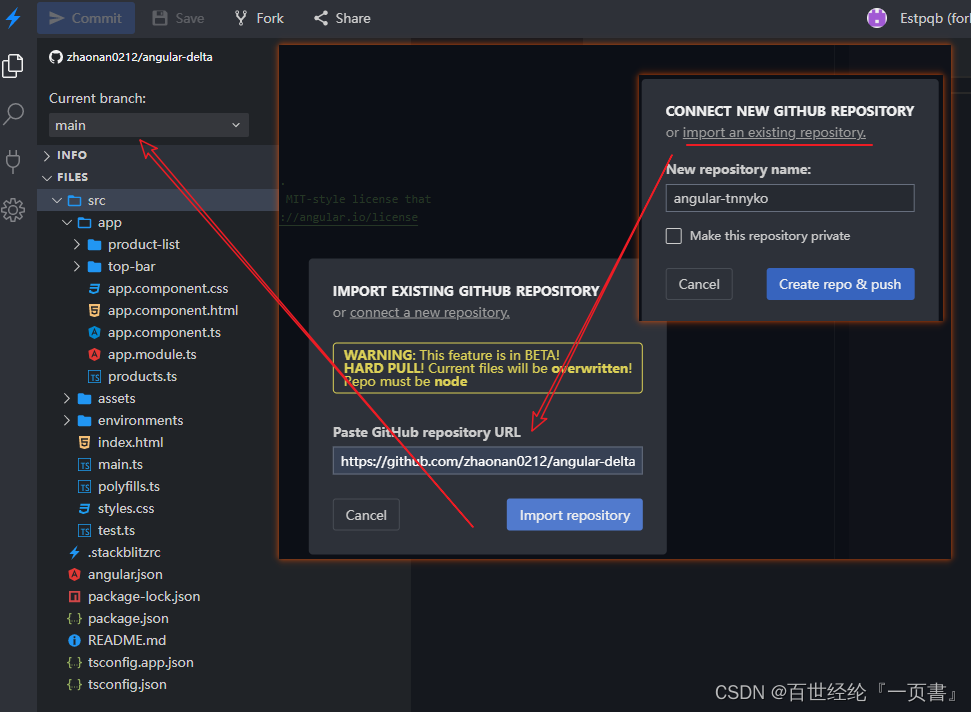
2.3 常用语法
- 在上面的项目中载打开个terminal,执行
ng generate component data-demo  - 在app.module.ts中添加DataDemoComponent的router
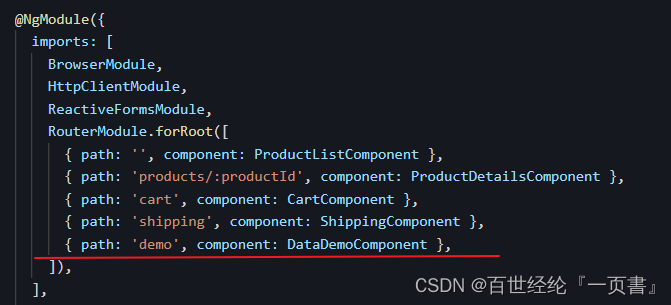 - 测试的代码都在下面,包括ts和html
- 声明基本属性的四种方式
没写就代表是public 可以指定数据类型,string、nomber,boolean any代表任何数据类型 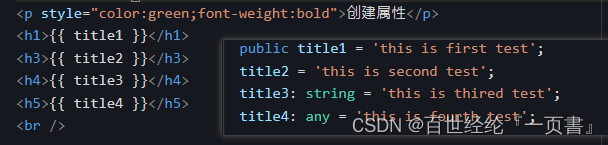 - 声明对象
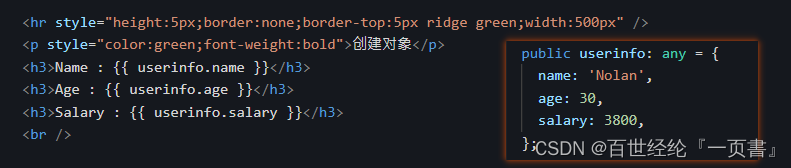 - 属性赋值[ ]
使用了[ ],意思就是后面的是变数 没有使用[ ],意思就是后面的是字符串 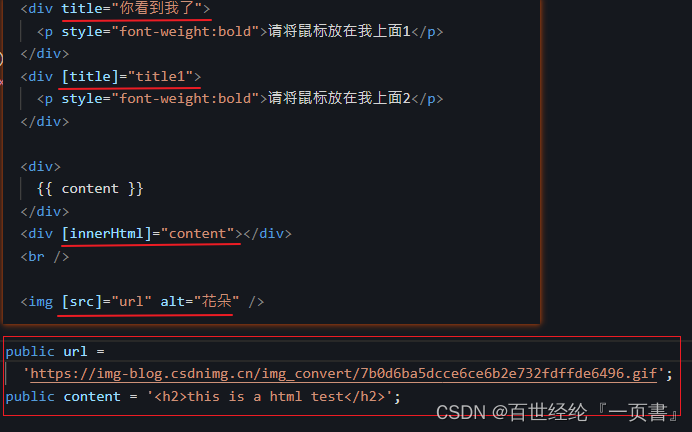 - 定义及解析数组
遍历使用*ngfor 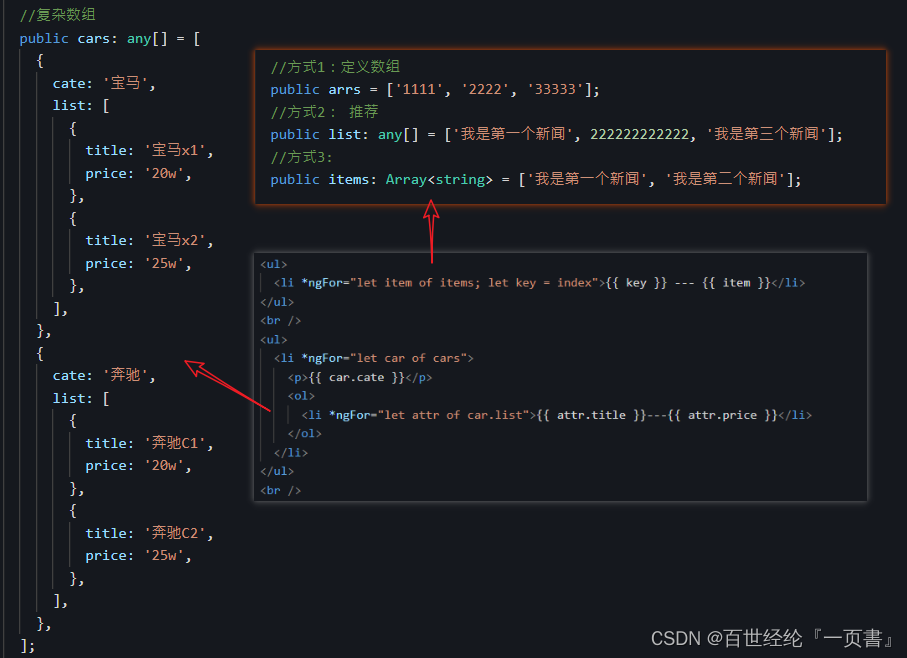 - 判断使用*ngIf
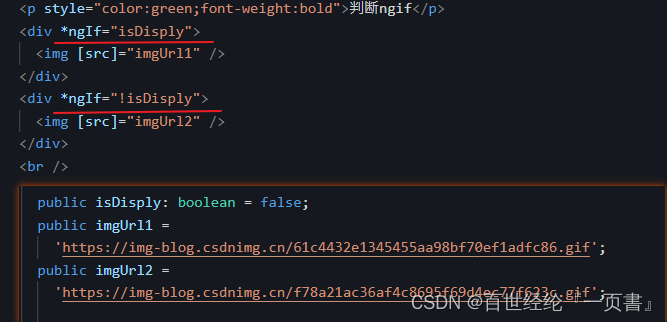 - 另一种判断ngSwitch
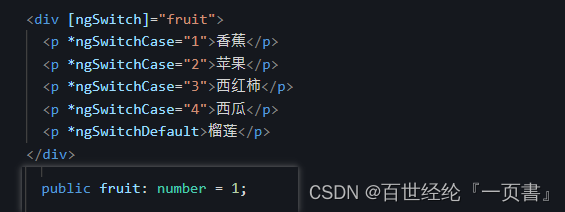 - 管道
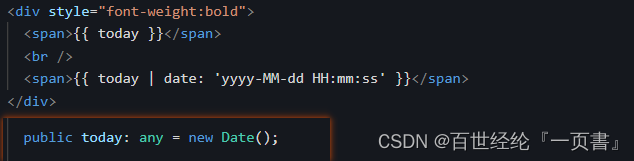 - 事件
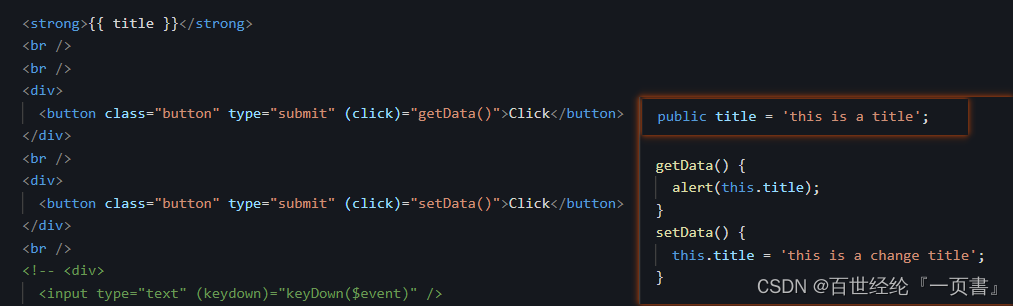 - 表单
146
import { Component, OnInit } from '@angular/core';
@Component({
selector: 'app-data-demo',
templateUrl: './data-demo.component.html',
styleUrls: ['./data-demo.component.css'],
})
export class DataDemoComponent implements OnInit {
public title1 = 'this is first test';
title2 = 'this is second test';
title3: string = 'this is thired test';
title4: any = 'this is fourth test';
public userinfo: any = {
name: 'Nolan',
age: 30,
salary: 3800,
};
public url =
'https://img-blog.csdnimg.cn/img_convert/7b0d6ba5dcce6ce6b2e732fdffde6496.gif';
public content = '<h2>this is a html test</h2>';
public arrs = ['1111', '2222', '33333'];
public list: any[] = ['我是第一个新闻', 222222222222, '我是第三个新闻'];
public items: Array<string> = ['我是第一个新闻', '我是第二个新闻'];
public cars: any[] = [
{
cate: '宝马',
list: [
{
title: '宝马x1',
price: '20w',
},
{
title: '宝马x2',
price: '25w',
},
],
},
{
cate: '奔驰',
list: [
{
title: '奔驰C1',
price: '20w',
},
{
title: '奔驰C2',
price: '25w',
},
],
},
];
public isDisply: boolean = false;
public imgUrl1 =
'https://img-blog.csdnimg.cn/61c4432e1345455aa98bf70ef1adfc86.gif';
public imgUrl2 =
'https://img-blog.csdnimg.cn/f78a21ac36af4c8695f69d4ec77f623c.gif';
public fruit: number = 1;
public attr = 'orange';
public today: any = new Date();
public title = 'this is a title';
constructor() {
this.title4 = 'this is 4th test';
console.log(this.today);
}
ngOnInit(): void {}
getData() {
alert(this.title);
}
setData() {
this.title = 'this is a change title';
}
}
<p style="color:green;font-weight:bold">this is data-demo page!</p>
<br />
<hr style="height:5px;border:none;border-top:5px ridge green;width:500px" />
<p style="color:green;font-weight:bold">创建属性</p>
<h1>{{ title1 }}</h1>
<h3>{{ title2 }}</h3>
<h4>{{ title3 }}</h4>
<h5>{{ title4 }}</h5>
<br />
<hr style="height:5px;border:none;border-top:5px ridge green;width:500px" />
<p style="color:green;font-weight:bold">创建对象</p>
<h3>Name : {{ userinfo.name }}</h3>
<h3>Age : {{ userinfo.age }}</h3>
<h3>Salary : {{ userinfo.salary }}</h3>
<br />
<hr style="height:5px;border:none;border-top:5px ridge green;width:500px" />
<p style="color:green;font-weight:bold">属性赋值[]</p>
<div title="你看到我了">
<p style="font-weight:bold">请将鼠标放在我上面1</p>
</div>
<div [title]="title1">
<p style="font-weight:bold">请将鼠标放在我上面2</p>
</div>
<div>
{{ content }}
</div>
<div [innerHtml]="content"></div>
<br />
<img [src]="url" alt="花朵" />
<hr style="height:5px;border:none;border-top:5px ridge green;width:500px" />
<p style="color:green;font-weight:bold">简单运算</p>
<div>1+2={{ 1 + 2 }}</div>
<div>2*3={{ 2 * 3 }}</div>
<br />
<hr style="height:5px;border:none;border-top:5px ridge green;width:500px" />
<p style="color:green;font-weight:bold">定义数据</p>
<div *ngFor="let arr of arrs">
{{ arr }}
</div>
<br />
<div *ngFor="let one of list">
{{ one }}
</div>
<br />
<ul>
<li *ngFor="let item of items; let key = index">{{ key }} --- {{ item }}</li>
</ul>
<br />
<ul>
<li *ngFor="let car of cars">
<p>{{ car.cate }}</p>
<ol>
<li *ngFor="let attr of car.list">{{ attr.title }}---{{ attr.price }}</li>
</ol>
</li>
</ul>
<br />
<hr style="height:5px;border:none;border-top:5px ridge green;width:500px" />
<p style="color:green;font-weight:bold">判断ngif</p>
<div *ngIf="isDisply">
<img [src]="imgUrl1" />
</div>
<div *ngIf="!isDisply">
<img [src]="imgUrl2" />
</div>
<br />
<div [ngSwitch]="fruit">
<p *ngSwitchCase="1">香蕉</p>
<p *ngSwitchCase="2">苹果</p>
<p *ngSwitchCase="3">西红柿</p>
<p *ngSwitchCase="4">西瓜</p>
<p *ngSwitchDefault>榴莲</p>
</div>
<br />
<hr style="height:5px;border:none;border-top:5px ridge green;width:500px" />
<p style="color:green;font-weight:bold">ngclass/ngstyle</p>
<div class="red" style="font-weight:bold">this is a test</div>
<div [ngClass]="{ blue: true, red: false }" style="font-weight:bold">
this is a test
</div>
<div [ngClass]="{ orange: isDisply, red: !isDisply }" style="font-weight:bold">
This div is test ngClass.
</div>
<br />
<div [ngStyle]="{ color: 'red' }" style="font-weight:bold">
This div is test ngStyle.
</div>
<div [ngStyle]="{ color: attr }" style="font-weight:bold">
This div is test ngStyle.
</div>
<br />
<hr style="height:5px;border:none;border-top:5px ridge green;width:500px" />
<p style="color:green;font-weight:bold">管道</p>
<div style="font-weight:bold">
<span>{{ today }}</span>
<br />
<span>{{ today | date: 'yyyy-MM-dd HH:mm:ss' }}</span>
</div>
<br />
<hr style="height:5px;border:none;border-top:5px ridge green;width:500px" />
<p style="color:green;font-weight:bold">事件</p>
<strong>{{ title }}</strong>
<br />
<br />
<div>
<button class="button" type="submit" (click)="getData()">Click</button>
</div>
<br />
<div>
<button class="button" type="submit" (click)="setData()">Click</button>
</div>
<br />
<!-- <div>
<input type="text" (keydown)="keyDown($event)" />
</div> -->
<br />
<hr style="height:5px;border:none;border-top:5px ridge green;width:500px" />
<p style="color:green;font-weight:bold">事件</p>
2.4 官方Demo
3.xxxxx
4.xxxxx
5.告诫自己
?????????在一秒钟内看到本质的人和花半辈子也看不清一件事本质的人,自然是不一样的命运。 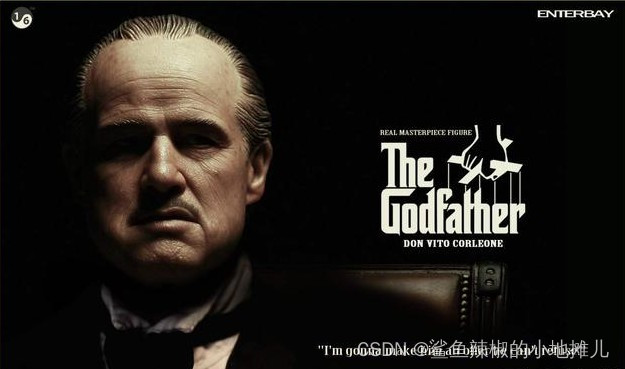
|