unity入门
递归查找子物体
void Start()
{
Transform child = FindChild("Cube1",transform);
if (child)
{
Debug.Log("子物体的名称:" + child.name);
}
}
Transform FindChild(string childName,Transform parent)
{
if(parent.name == childName)
{
return parent;
}
if(parent.childCount < 1)
{
return null;
}
Transform obj = null;
for(int i = 0; i < parent.childCount; i++)
{
Transform t = parent.GetChild(i);
obj = FindChild(childName, t);
if(obj != null)
{
break;
}
}
return obj;
}
生命周期函数
public class LifeScript : MonoBehaviour
{
private void Awake()
{
Debug.Log("Awake");
}
private void OnEnable()
{
Debug.Log("OnEnable");
}
private void OnDisable()
{
Debug.Log("OnDisable");
}
private void Start()
{
Debug.Log("Start");
}
private void FixedUpdate()
{
Debug.Log("FixedUpdate");
}
private void Update()
{
Debug.Log("Update");
}
private void LateUpdate()
{
Debug.Log("LateUpdate");
}
private void OnDestroy()
{
Debug.Log("OnDestroy");
}
}
Light 灯光组件
1、Type : ??Directional 平行光,模仿的是太阳光 ??Spot: 聚光灯 ??Point:点光源 ??Area: 区域光 2、Color: 颜色值 3、Mode: ??RealTime实时 ??Max混合 ??Baked烘焙 4、Intensity : 光照强度 5、Indirect Multiplier: 光照强度系数 6、Shadow Type: ??影子设置: ????No Shadow 没有影子 ????Hard Shadow 硬影子 ????Soft Shadow 柔和影子 7、Cookie: 灯光上贴的一张纸 ?? size:照射的大小 8、Draw halo: 辉光 9、Culling Make : 裁剪层
组件获取与控制
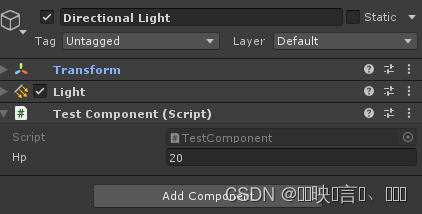 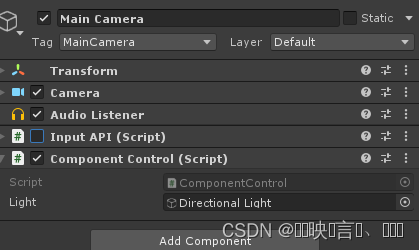
public class TestComponent : MonoBehaviour
{
public float hp;
}
public class ComponentControl : MonoBehaviour
{
public GameObject light;
void Start()
{
Light l = light.GetComponent<Light>();
l.type = LightType.Area;
l.cullingMask = 5;
TestComponent tc = light.GetComponent<TestComponent>();
Debug.Log("hp : " + tc.hp);
}
}
克隆游戏物体
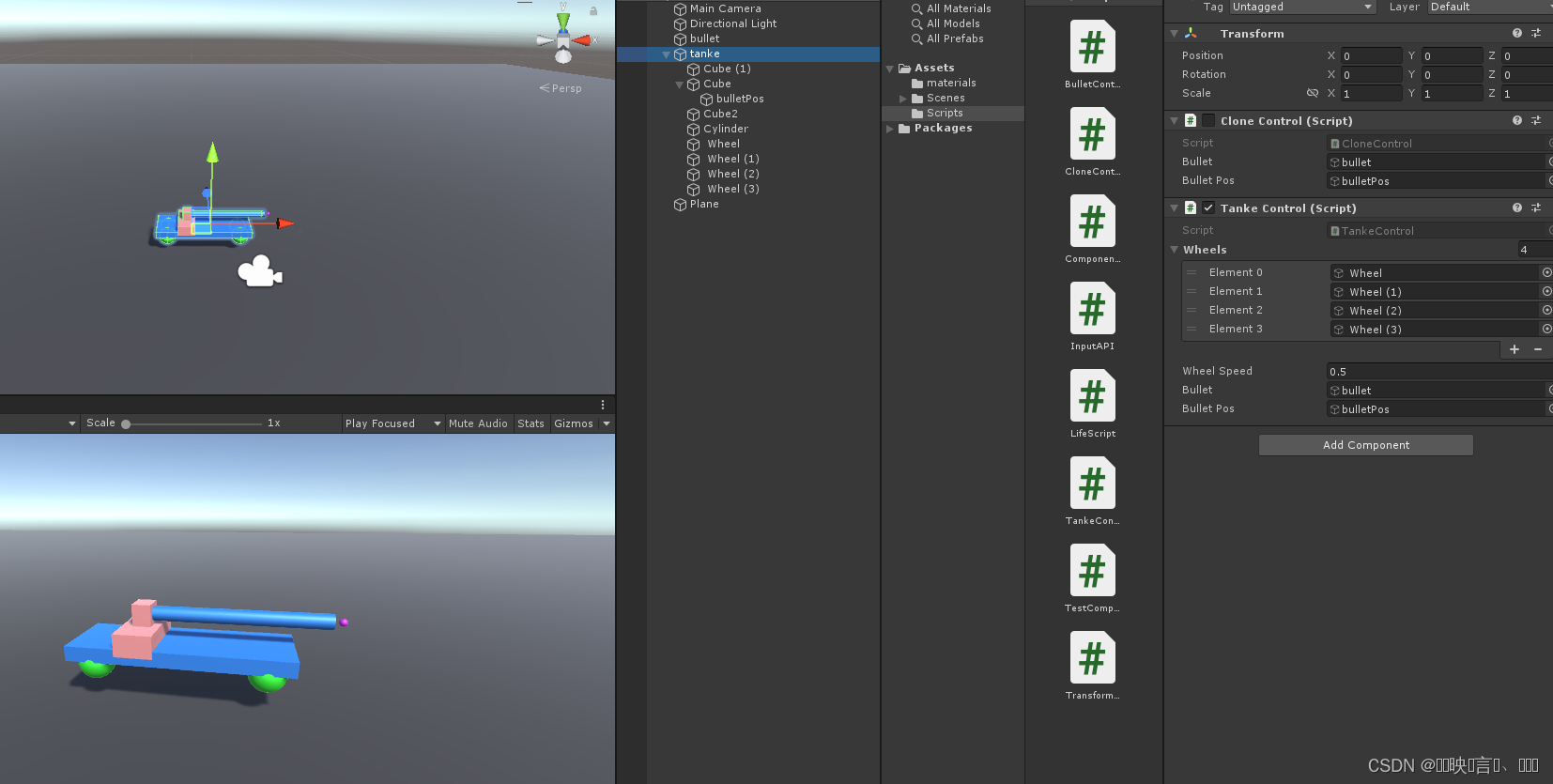 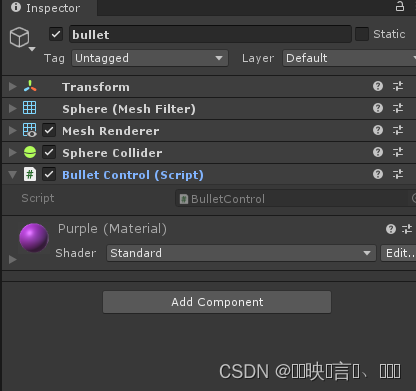
public class TankeControl : MonoBehaviour
{
public GameObject[] wheels;
public float wheelSpeed = 0.5f;
private float h, v;
public GameObject bullet;
public GameObject bulletPos;
void Update()
{
for (int i = 0; i < wheels.Length; i++)
{
wheels[i].transform.Rotate(new Vector3(0, 0, -1 * wheelSpeed), Space.Self);
}
h = Input.GetAxis("Horizontal");
v = Input.GetAxis("Vertical");
if (v < 0)
{
transform.Rotate(0, -h*0.6f, 0);
}
else
{
transform.Rotate(0, h*0.6f, 0);
}
transform.Translate(new Vector3(1, 0, 0) * v * 0.2f, Space.Self);
if (Input.GetKeyDown(KeyCode.Space))
{
GameObject go = Instantiate(bullet, bulletPos.transform.position, Quaternion.identity);
go.transform.rotation = bulletPos.transform.rotation;
}
}
}
public class BulletControl : MonoBehaviour
{
void Update()
{
transform.Translate(new Vector3(1,0,0) * 0.1f, Space.Self);
}
}
|