- css样式
transition: transition-property transition-duration transition-timing-function transition-delay;
- transition-property:设置元素中参与过渡的属性名称 如 width background 必填
- transition-duration:设置元素\过渡的持续时间; 如1s 必填
- transition-timing-function:设置元素过渡的动画类型;
- transition-delay:设置过渡效果延迟的时间,默认为 0;
transition-timing-function动画类型如下
以始终相同的速度完成整个过渡过程
值 | 描述 |
---|
linear | 以始终相同的速度完成整个过渡过程 | ease | 以慢速开始,然后变快,然后慢速结束的顺序来完成过渡过程 | ease-in | 以慢速开始过渡的过程 | ease-out | 以慢速结束过渡的过程 | ease-in-out | 以慢速开始,并以慢速结束的过渡效果 | 例子如下: | |
.transition-property {
width: 300px;
height: 300px;
border: 3px solid black;
transition-property: width background;
transition-duration: 0.9s 0.1s;
transition-timing-function: ease;
transition-delay: 2s;
}
.transition-property:hover {
width: 600px;
background-color: red;
}
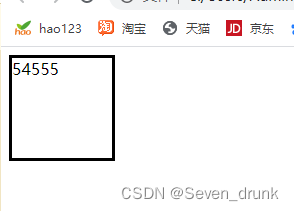 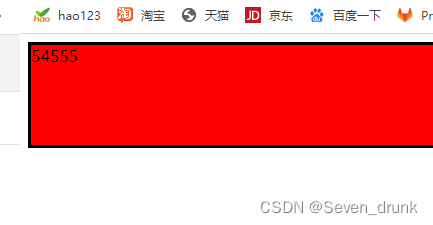
animation动画
创建 CSS 动画,要了解 @keyframes 规则。@keyframes 规则用来定义动画各个阶段的属性值
- animationName:表示动画的名称; from:定义动画的开头,相当于 0%;
- percentage:定义动画的各个阶段,为百分比值,可以添加多个; to:定义动画的结尾,相当于 100%;
- properties:不同的样式属性名称,例如 color、left、width 等等。
@keyframes ball {
0% { top: 0px; left: 0px;}
25% { top: 0px; left: 350px;}
50% { top: 200px; left: 350px;}
75% { top: 200px; left: 0px;}
100% { top: 0px; left: 0px;}
}
div {
width: 100px;
height: 100px;
border-radius: 50%;
border: 3px solid black;
position: relative;
animation-name: ball;
}
注意:要想让动画成功播放,要定义 animation-duration 属性,否则会因为 animation-duration 属性的默认值为 0,导致动画并不会播放 红圈自下往上 黑圈自上而下 移动例子:
@keyframes ball{
0%{
opacity: 0;
transform: translateY(300px);
}
100%{
opacity: 1;
}
}
@keyframes ballTwo{
0%{
opacity: 0;
transform: translateY(-150px);
}
100%{
opacity: 1;
}
}
.one {
width: 100px;
height: 100px;
border-radius: 50%;
border: 3px solid black;
position: relative;
animation-name: ball;
animation-duration:2s;
position: relative;
top: 300px;
}
.two {
width: 100px;
height: 100px;
border-radius: 50%;
border: 3px solid red;
position: relative;
animation-name: ballTwo;
animation-duration:2s;
animation-delay:2s;
position: relative;
top: 300px;
}
vue —animation
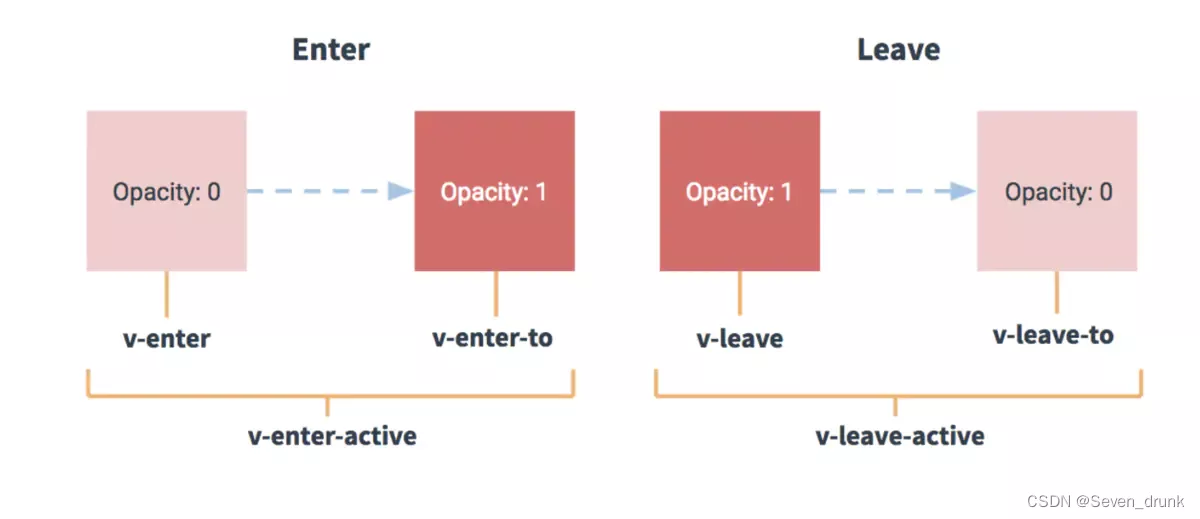 在进入/离开的过渡中,会有 6 状态; v-enter 、v-enter-active 、v-enter-to 、v-leave 、v-leave-active 、v-leave-to
- 由 v-if 所触发的切换
- 由 v-show 所触发的切换
- 由特殊元素 切换的动态组件
为过渡效果命名
可以给 组件传一个 name prop 来声明一个过渡效果名
<Transition name="fade">
...
</Transition>
对于一个有名字的过渡效果,对它起作用的过渡 class 会以其名字为前缀: 如下图:
.fade-enter-active,
.fade-leave-active {
transition: opacity 0.5s ease;
}
.fade-enter-from,
.fade-leave-to {
opacity: 0;
}
如果我们可能想要先执行离开动画,然后在其完成之后再执行元素的进入动画,可以使用mode
<Transition mode="out-in">
...
</Transition>
例子如下:
<template>
<div>
<button @click="handleAnis">Toggle</button>
<Transition name="fade" mode="out-in">
<img v-if="show" key="a" src="https://xa.hw.luckygames.team/casino/images/app/keno80.jpg" />
<img v-else key="b" src="https://www.hw.luckygames.team/casino/images/app/football.jpg" />
</Transition>
</div>
</template>
<script>
export default {
data() {
return {
show: true,
roleName: "SEER",
};
},
methods: {
handleAnis() {
this.show = !this.show;
},
},
};
</script>
<style lang="less" scoped>
.fade-enter-active,
.fade-leave-active {
transition: all 1.3s;
}
.fade-enter,
.fade-leave-to {
opacity: 0;
transform: translateY(600px);
}
</style>
|