网络编程
网络编程和javaWeb是不一样的,Javaweb是对网页进行编程,网络编程是对通信相关的编程。本机电脑的ip为:127.0.0.1
端口
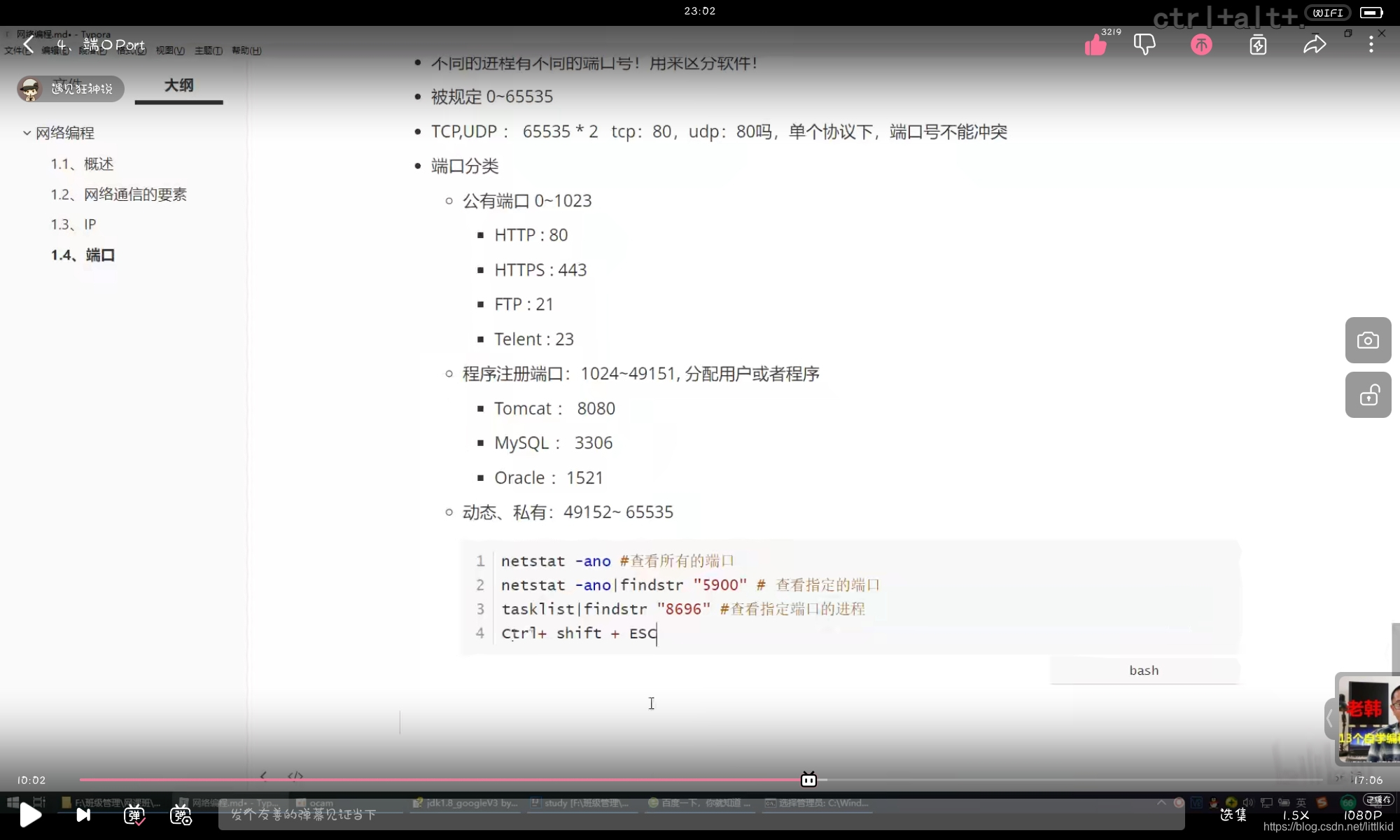
通信协议
TCP和UDP TCP:类似于打电话,即要确定对方接收到。特点:连接,稳定 UDP:类似于发短信,不用确定对方是否收的到。特点:不连接,不稳定
TCP:三次握手,四次挥手
最少需要三次才能建立稳定的连接:
- A:你瞅啥
- B:瞅你咋地
- A:干一场
四次挥手: - A:我要走了
- B:你真的要走了吗?
- B:你真的真的要走了吗?
- A:我真的要走了
TCP实现简单的聊天
服务器端实现:
public class TcpServerDemo01 {
public static void main(String[] args) throws Exception {
ServerSocket serverSocket = new ServerSocket(9999);
Socket accept = serverSocket.accept();
InputStream inputStream = accept.getInputStream();
ByteArrayOutputStream baos = new ByteArrayOutputStream();
byte[] buffer=new byte[1024];
int len;
while ((len=inputStream.read(buffer))!=-1){
baos.write(buffer,0,len);
}
System.out.println(baos.toString());
baos.close();
inputStream.close();
accept.close();
serverSocket.close();
}
}
客户端实现
public class TcpClientDemo01 {
public static void main(String[] args) throws Exception {
InetAddress byName = InetAddress.getByName("127.0.0.1");
int port=9999;
Socket socket = new Socket(byName, port);
OutputStream outputStream = socket.getOutputStream();
outputStream.write("欢迎学习网络编程".getBytes());
outputStream.close();
socket.close();
}
}
- 上面是简单的聊天
- 下面是实现文件的上传至服务器端
服务器端
public class TcpServerDemo02 {
public static void main(String[] args) throws Exception {
ServerSocket serverSocket = new ServerSocket(9999);
Socket accept = serverSocket.accept();
InputStream is = accept.getInputStream();
FileOutputStream fos = new FileOutputStream(new File("LII.png"));
byte[] buffer2 = new byte[1024];
int len;
while ((len=is.read(buffer2))!=-1){
fos.write(buffer2,0,len);
}
System.out.println(fos.toString());
fos.close();
is.close();
accept.close();
serverSocket.close();
}
}
客服端实现
public class TcpClientDemo02 {
public static void main(String[] args) throws Exception {
int port=9999;
Socket localhost = new Socket(InetAddress.getByName("127.0.0.1"), port);
OutputStream os = localhost.getOutputStream();
FileInputStream fs = new FileInputStream(new File("li.png"));
byte[] buffer2 = new byte[1024];
int len;
while ((len=fs.read(buffer2))!=-1){
os.write(buffer2,0,len);
}
System.out.println(os.toString());
fs.close();
os.close();
localhost.close();
}
}
从网站上下载资源,类似爬虫
package network01.UDPcontact;
import java.io.FileOutputStream;
import java.io.InputStream;
import java.net.HttpURLConnection;
import java.net.MalformedURLException;
import java.net.URL;
import java.net.URLConnection;
public class URLdown {
public static void main(String[] args) throws Exception {
URL url = new URL("https://m10.music.126.net/20210712150626/8b05d2d67df425e6a86ccf96c4e9e66d/yyaac/obj/wonDkMOGw6XDiTHCmMOi/2987358025/68fb/1f3f/86ea/c3b557f8c2e01aeb9daa2a64ecb09b7b.m4a");
HttpURLConnection urlConnection = (HttpURLConnection) url.openConnection();
InputStream inputStream = urlConnection.getInputStream();
FileOutputStream fileOutputStream = new FileOutputStream("f1.m4a");
byte[] buffer = new byte[1024];
int len;
while ((len=inputStream.read(buffer))!=-1){
fileOutputStream.write(buffer,0,len);
}
fileOutputStream.close();
inputStream.close();
}
}
UDP
UDP 实现“客户端”和“服务器端”的步骤
Socket之UDP套接字
UDP套接字:UDP套接字的使用是通过DatagramPacket类和DatagramSocket类,客户端和服务器端都是用DatagramPacket类来接收数据,使用DatagramSocket类来发送数据。
UDP客户端:也是主要执行三个步骤。
1.创建DatagramSocket实例;
2.使用DatagramSocket类的send()和receive()方法发送和接收DatagramPacket实例;
3.最后使用DatagramSocket类的close()方法销毁该套接字。
UDP服务器端:典型的UDP服务器要执行三个步骤,
1.创建一个指定了本地端口的DatagramSocket实例;
2.使用DatagramSocket的receive()方法接收一个来自客户端的DatagramPacket实例,而这个DatagramPacket实例在客户端创建时就包含了客户端的地址,这样我们就知道回复信息要发送到哪里了;
3.使用DatagramSocket类的send()和receive()方法来发送和接收DatagramPacket实例。
|