网络编程(套接字编程)
概述
计算机网格:
? 简单的说其实就是利用通信线路将地理上分散的、具有独立功能的计算机系统和通信设备按不同的形式连接起来,以功能完善的网络软件及协议实现资源共享和信息传递的系统。
网络编程的目的:
传播交流信息,数据交换,通信。
javaWeb:网页编程 B/S
网络编程: TCP/IP C/S
网络通信要素
如何实现网络的通信:
-
知道通信双方的地址:
-
满足规则:网络通信的协议 http,ftp,smtp,tcp,udp···
IP
IP地址:inetAddress类,在 import java.net.InetAddress;net包都是与网络通信相关
端口
端口表示计算机上的一个程序进程;Java中是InetSocketAddress类,在import java.net.InetSocketAddress;包下
通信协议
TCP/IP参考模型 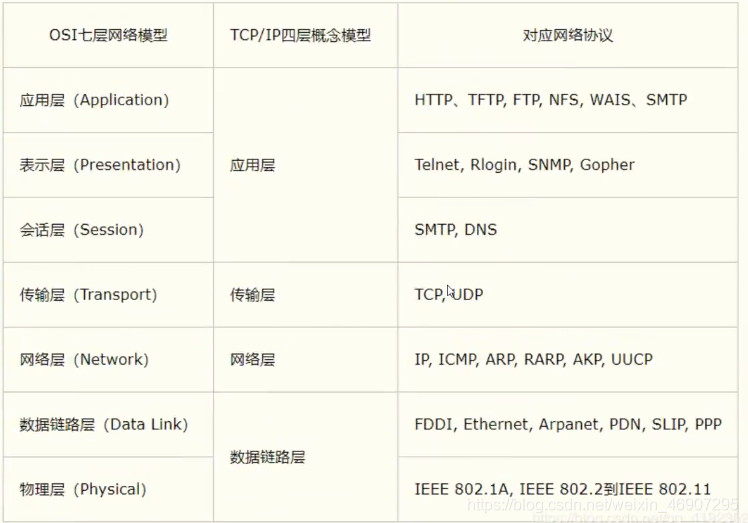
TCP/IP协议:实际上是一组协议
重要:
- TCP:用户传输协议
- UDP:用户数据报协议
- IP:网络互连协议
TCP和UDP对比
TCP:
-
连接,稳定 -
三次握手、四次挥手
-
客户端、服务端 -
传输完成,释放连接你,效率高
UDP:
- 不连接,不稳定
- 客户端、服务器:没有明确的界限
- 不管有没有准备好,都可以发过去
TCP
客户端
- 连接服务器 Socket
- 发送消息
public static void main(String[] args) {
Socket socket = null;
OutputStream os = null;
try {
InetAddress serverIp = InetAddress.getByName("127.0.0.1");
int port = 9999;
socket = new Socket(serverIp,port);
os = socket.getOutputStream();
os.write("你好,请输入姓名:".getBytes());
} catch (Exception e) {
e.printStackTrace();
}finally{
if (os!=null)
try {
os.close();
} catch (IOException e) {
e.printStackTrace();
}
if (socket!=null){
try {
socket.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
}
服务器
- 建立服务的端口 ServerSocket
- 等待用户的链接 accept
- 接收用户的消息
public class tcpServerDome01 {
public static void main(String[] args) {
ServerSocket serverSocket = null;
Socket socket = null;
InputStream is = null;
ByteArrayOutputStream baos = null;
try {
serverSocket = new ServerSocket(9999);
socket = serverSocket.accept();
is = socket.getInputStream();
baos = new ByteArrayOutputStream();
byte[] buffer = new byte[1024];
int len;
while ((len=is.read(buffer))!=-1){
baos.write(buffer,0,len);
}
System.out.println(baos.toString());
} catch (IOException e) {
e.printStackTrace();
}finally {
if (baos!=null){
try {
baos.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if (is!=null){
try {
is.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if (baos!=null){
try {
socket.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if (baos!=null){
try {
serverSocket.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
}
UDP
不需要连接,需要知道对方的地址(IP 、端口)
发送消息,发送端
package com.zhou.udp;
import java.net.DatagramPacket;
import java.net.DatagramSocket;
import java.net.InetAddress;
public class udpClientDome01 {
public static void main(String[] args) throws Exception{
DatagramSocket socket = new DatagramSocket();
String msg = "你好啊,服务器!";
InetAddress localhost = InetAddress.getByName("localhost");
int port = 9000;
DatagramPacket so = new DatagramPacket(msg.getBytes(),0,msg.getBytes().length,localhost,9000);
socket.send(so);
socket.close();
}
}
接收端
package com.zhou.udp;
import java.net.DatagramPacket;
import java.net.DatagramSocket;
public class uspServerDome01 {
public static void main(String[] args) throws Exception{
DatagramSocket socket = new DatagramSocket(9000);
byte[] beffer = new byte[1024];
DatagramPacket is = new DatagramPacket(beffer, 0, beffer.length);
socket.receive(is);
System.out.println(is.getAddress().getHostAddress());
System.out.println(new String(is.getData(),0,is.getLength()));
socket.close();
}
}
|